Usually, articles of this kind give full answers to questions, but if you are interviewing for a rather serious position, a brief summary will obviously not be enough and you need to understand the question itself rather deeply, so I mainly inserted links to documentation or articles on the issues described.
PHP
- What's new in PHP 7. *
- Data types
- Type hinting + strict mode
- Strong versus loose comparison
- Working with links
- Copy-on-write
- Run time error handling
- Closures
- Array functions
- list()
- //
- Final
- Reflection API
- Autoloader
- SPL
- APC/APCu
- OPcache
- PSR standards
- Composer
- XDebug
- Rest API
OOP
Databases
Docker
HTTP
JS
PHP
PHP 7.*
Eh, I remember the times when I was telling the difference between versions 4 and 5, never seeing the 4th in my eyes, old traditions must be observed, the question occurs in almost all interviews, and although no one expects you to remember literally in which version which changes have occurred, in general, this information is worth knowing:
- New in php 7.0 ( habr / documentation )
- New in php 7.1 ( habr / documentation )
- New in php 7.2 ( habr / documentation )
- New in php 7.3 ( habr / documentation )
- New in php 7.4 ( habr / documentation )
- And since we started, new in php 8.0 habr
Data types
Although PHP is a dynamic language, all data in it is of distinct types:
Scalar types:
- bool
- int
- float (sometimes the name double is found, which for php does not make a difference)
- string
Mixed types:
- array
- object
- callable
- iterable
Special types:
- resource
- null
Read more about types and pseudo-types in the documentation .
Type hinting + strict mode
As a logical continuation of the topic of types and strong typing, which came with php 7. *, you need to know about the difference between strong and weak typing, see the documentation for details .
Strong versus loose comparison
You need to understand the difference between loose comparison (comparison of values ​​without regard to data type) and strict comparison (taking into account data type), which this table reflects quite well . We should also highlight the strict and loose comparison of objects .
Working with links
References in PHP are roughly a way to access the same variable (memory area) under different names.
The main operations that can be performed with links:
It is important to understand the specifics of passing objects. You may have read that in php all objects are passed by reference
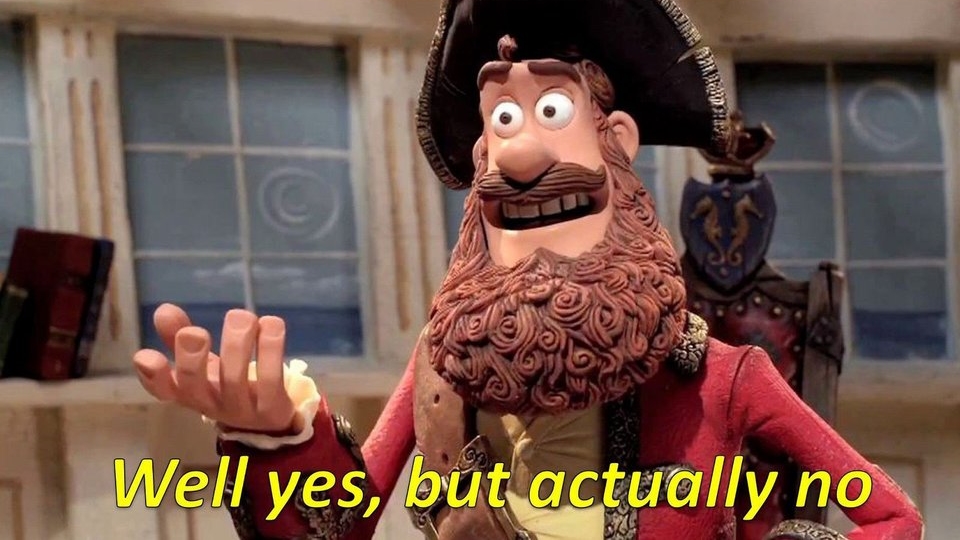
This was true before php5, but now only the object identifier is passed ( documentation ).
In short, if you created an object, and passed it to a function, inside which you did
$object = null;
this does not mean that the original object will also be reset to zero, you can still work with it. But if you explicitly passed an object by reference, then this action will "zero" the original object as well.
Copy-on-write
Each time a variable is passed to a function, its value (in theory) should be copied. This might not be a problem for integer or float data, but imagine you are passing an array containing ten million values ​​to a function. To avoid this, PHP uses the copy-on-write paradigm. So, in fact, when you transfer an array, its copying does not occur until the moment when you do not want to change anything in this array. An article with a more detailed explanation.
Run time error handling
In the 7th version of php there were a number of changes that relate to the error hierarchy (more information on the hierarchy ), otherwise it is expected that the applicant will know how to catch exceptions , or even be able to write his own error_handler .
Closures
They are also anonymous functions, most useful as values ​​for callback parameters, documentation . There is a good, albeit a little old article about working with closures in Habré .
Array functions
Often asked to name all the functions for working with arrays that you remember from memory. And although, in my opinion, this question is a little more than meaningless, the list of functions can be repeated in the documentation .
List () function
Thanks to changes in PHP 7.1, the function has become more convenient to use, as a result, it is used more often, all information can be found in the documentation .
Variable scope
In most cases, all variables have only one scope , with the exception of global / superglobals, which, as the name implies, are available everywhere, as well as static / constants.
Class property / method / constant scope
It is defined using the keywords public (visible to everyone), protected (available only to the current class or its descendants) or private (available only to the current class), and can be used both for properties / methods and since PHP 7.1 for class constants. Read more in the documentation .
Final classes and methods
By placing the final keyword in front of class method declarations, you can prevent them from being overridden in child classes, and if the class itself is declared final, it cannot be inherited. Such classes and methods are designed to protect sensitive code from changes, and the code itself becomes easier to maintain.
Read more in the documentation .
Late static linking
Expressed as the difference between calling self :: and static ::. "Late binding" means that static :: will be evaluated at runtime and can be redefined in derived classes. Read more in the documentation .
Magic methods
In PHP, there is absolutely nowhere without magic, so we have methods that allow us to implement additional functionality for an object, override the standard behavior of an object (for example, __serialize () / __ unserialize ()), or the behavior of an object in atypical situations (__toString ()) and others.
Documentation , and a good, albeit slightly outdated article on habr .
Dynamic variables
You can pass the name of one variable to another and then use that variable “dynamically”.
They should be used with great care, as they can significantly complicate both reading and maintaining the code (it is often impossible to understand what is in a variable without running the code), but they can be very useful in abstractions. Read more in the documentation .
Generators
Generators (yield keyword) create simple iterators to save memory in situations where you need to iterate over a large amount of data. They can be a bit tricky to understand right before you write your generator.
The description is in the documentation , and there is a rather old one (however, the syntax of generators has not changed since that moment) and a good article on habr .
Reflection API
In short, it is the ability to look inside your own (or someone else's) code, find out what variables, methods, constants a class has, and make them public if desired. This ability is actively used by frameworks (for example, in a symphony for auto-generation of dependency injection) and libraries. Description in the documentation and, of course, an article on Habré .
Autoloader
Usually, on projects it is very rarely necessary to implement autoloading of classes yourself, but for companies that write all solutions on their own (for example, large corporations that do not want to work with open source), or some special projects, this is relevant. It can also be helpful to understand how it works.
- github.com/codedokode/pasta/blob/master/php/autoload.md
- www.php.net/manual/ru/function.spl-autoload-register.php
- www.php.net/manual/ru/language.oop5.autoload.php
SPL
Or Standart PHP Library - a set of classes (data structures, iterators, exceptions, classes for processing files), interfaces and functions for solving standard problems. As a rule, knowing the library by heart is not required, but understanding what it consists of is worth at least for personal development. More details in the documentation - www.php.net/manual/ru/book.spl.php
APC / APCu
Alternative PHP cache (APC) or alternative cache without opcode (APCu) is a free open source cache designed for caching and optimizing PHP intermediate code. It is mainly used to save computations between requests. Documentation:
OPcache
Improves PHP performance by storing compiled script bytecode in shared memory, thereby eliminating the need for PHP to load and parse scripts on every request. Official documentation , as well as a very good article on how it all works on Habré .
PSR standards
PHP Standards Recommendations - standards for coding, as well as interfaces for common tasks. These standards are used in all (known to me) frameworks, and facilitate interactions between different libraries. Official documentation as well as a good article on Habré .
Composer
Dependency manager , as well as a tool that implements autoloading of library code and the application itself. Can help optimize and speed up your production app .
XDebug
Most developers use this extension to make it easier to work with the code or to optimize it, the basic knowledge here is how to set it up and run it, the following article blog.denisbondar.com/post/phpstorm_docker_xdebug will help with this .
Rest API
REpresentational State Transfer is an architectural approach that allows you to organize interaction between applications using all the capabilities of HTTP. Good article on this topic and also the differences between REST and SOAP .
In the context of an API, you need to understand the basic data exchange formats, most often XML and JSON .
Tests
All unit tests are based on PHPUnit (as far as I know), and today, being able and knowing how to write tests is a mandatory part of development. Many frameworks (like Symfony) offer their own wrappers and testing solutions. Here's a good article on the best testing tools .
OOP
Encapsulation / inheritance / polymorphism
- . , , , . , . .. . ( ) . , , .
- . , , , .
- Polymorphism . This is a property that allows the same name to be used to solve several technically different problems. In simple terms, the concept of polymorphism is the idea of ​​“one interface, many implementations”. This means that you can create a common interface for a group of related actions.
Abstract classes / methods
- Doesn't involve instantiation.
- They implement in practice one of the principles of OOP - polymorphism.
- May contain abstract methods and properties. An abstract method is not implemented for the class in which it is described, but must be implemented for its non-abstract descendants.
- They represent the most general abstractions, that is, having the greatest volume and the least content.
Implementation nuances in the documentation .
Interfaces
An interface, unlike an abstract class, cannot contain fields and methods that have an implementation - it only describes the method signatures that its inheritors must implement. Unlike the same abstract class, a class can implement many interfaces simultaneously.
Official documentation , and a good article on Habré .
Traits
The trait is very similar to a class, but it is intended for grouping functionality and its further reuse in a set of classes that cannot be linked by one abstract class. It is also sometimes said that a trait is a way to implement multiple inheritance in PHP.
Since each class can implement many traits, conflicts can arise when the same method is implemented in different traits and / or in the class itself. Read more about traits in the documentation .
GRASP templates
General Responsibility Assignment Software Patterns - design patterns for solving common problems. Unlike patterns from the Gang of Four, GRAPS patterns do not have a pronounced structure, a clear scope and a specific problem to be solved, but only represent generalized approaches / recommendations / principles used in the design of system design.
A good article on the topic - habr.com/ru/post/92570
Gang of four patterns
The topic of patterns is vast, many articles have already been written on it, and it is simply impossible to try to describe it in a few sentences. I like this resource - refactoring.guru/ru/design-patterns/catalog , but you can use this link if necessary .
SOLID
Everyone asks him, but they ask him differently.
Bad option - you are asked to read the principles and their definitions from memory.
Good - they tell you a principle, and ask you to explain it, describe examples of use, come up with a real situation when this or that principle will help in some way. This option, in my opinion, makes it much better to understand the level of the candidate and his real understanding of the principles.
On Habré you can find dozens of really good articles on these principles, so I link to medium .
Kiss
Keep it simple, stupid is a good principle, the whole point of which is already contained in the title, you can learn more here .
Dry
Don't repeat yourself - the principle that you should reuse your code wherever possible. More details here .
Databases
A good article on SQL databases can be found here . Questions about NoSql databases in my case are usually found on the example of MongoDB . In the case of ElasticSearch, wrapper libraries are usually used, general information can be obtained from the article on Habré .
In memory storages (those that store values ​​in RAM) are memcached and / or redis (most often), as a rule, work with them is carried out through wrapper libraries, if you want to understand the issue in more detail, of course there is an article on Habré .
Docker
A very important tool that has been used on almost all projects (in my case). And although it is often configured once per project, you need to know the basic concepts and the principle of working with it. Personally, I love this article for a job interview .
HTTP and other protocols
Of course, any web developer should know and understand how data transfer protocols work (at least top-level protocols). The topic is large and it is difficult to fit it into several sentences, there is an extensive article on this topic on Habré .
Authorization and authentication
Authorization is the granting of rights to perform certain actions, as well as the process of verifying rights when trying to perform these actions.
Authentication is an authentication procedure, such as verifying the identity of a user by comparing the password entered by him.
It is important to understand the difference between authorization and authentication , how they are implemented using session and cookies , JSON Web Tokens ( JWT ), etc.
Js
I don't really know this language, so I never pretend to be positions where you need to know js frameworks and be full-stack, perhaps that's why for all interviews there was enough knowledge described in the article - habr.com/ru/post/486820