Today we will develop a telegram bot on node js that can display statistics of coronavirus infected in all countries.
First of all, you need to add @botFather to the telegram contact list and write the / newBot command to it . Next, we set the name of our bot and, if it is not busy, we come up with a bot identifier, by which it can be found.

That's all, our telegram bot is ready and botfather has shared with us the Token API , thanks to which we will be able to control the bot.
Next, create a new project, enter npm init and add the bot.js file in which our bot will be developed.
Then I will install telegraf - this is one of the popular frameworks for creating telegram bot. We look at the telegraph documentation, copy the initial bot configuration into our project and quickly go through all the methods that are indicated in the example:
const { Telegraf } = require('telegraf')
const bot = new Telegraf(process.env.BOT_TOKEN) // , botFather
bot.start((ctx) => ctx.reply('Welcome')) // /start
bot.help((ctx) => ctx.reply('Send me a sticker')) // /help
bot.on('sticker', (ctx) => ctx.reply('')) //bot.on , ,
bot.hears('hi', (ctx) => ctx.reply('Hey there')) // bot.hears , - "hi"
bot.launch() //
Put api token in our example and zapuctim bot.
node bot
Let's check the work of our bot:
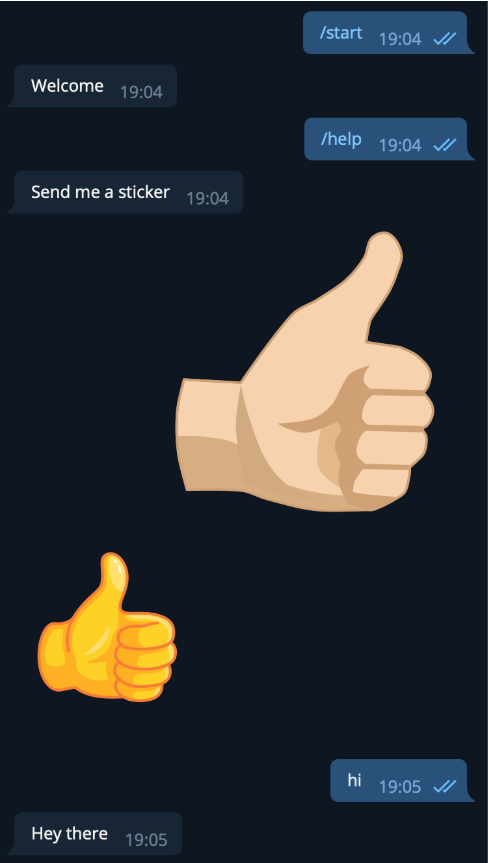
Now let's figure out what lies in ctx
For this, after declaring the bot constant, we can use log :
ctx.message.from.first_name
Restart our project, enter the / start command and in the console we get an object in which we can view the necessary user data:
{
"update_id": 375631294,
"message": {
"message_id": 11,
"from": {
"id": 222222,
"is_bot": false,
"first_name": "",
"username": "Evgenii",
"language_code": "ru"
},
"chat": {
"id": 386342082,
"first_name": "",
"username": "Evgenii",
"type": "private"
},
"date": 1593015188,
"text": "/start",
"entities": [
{
"offset": 0,
"length": 6,
"type": "bot_command"
}
]
}
}
We will be interested in the message object, from which we can get the username
ctx.message.from.first_name
And the text he sent to the bot:
ctx.message.text
We know what lies in ctx and now we can start connecting a third-party api, with which we can get statistics on the coronavirus. For this I will use a library called covid19-api . Let's install it into our project and import it into the bot.js file :
const covidApi = require('covid19-api')
Next, we will remove our sticker handler and make a new handler that tracks the text and sends a request to get the coronavirus data using the getReportsByCountries method found in the covid19-api documentation :
bot.on('text', async ctx => {
const covidData = await covidApi.getReportsByCountries(ctx.message.text) //
ctx.reply(covidData) //
})
Let's check what data we get. For example, let's write to our bot in the telegram: 'russia':
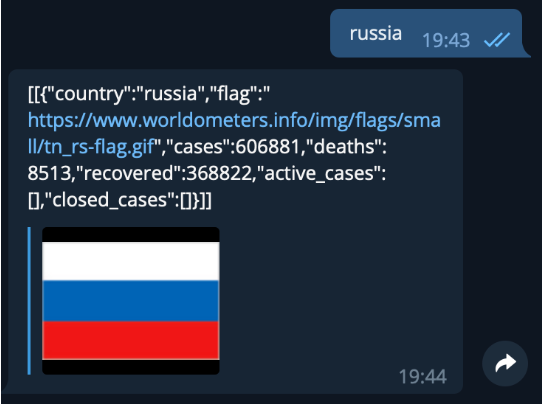
Great, we've got a lot of data. But if we enter the name of the country with a typo, our bot will stop working and give an error to the console:

To avoid this, we need to create an error handler and notify the user that such a country does not exist. We will also make a small refactoring of the code and make our bot more friendly, change the output of the message that is sent by the / start command , set up the correct display of statistics data and create a constant in a separate file that will contain a list of all countries in English and will display it when using the / help command .
As a result, we get the code:
const { Telegraf } = require('telegraf');
const covidApi = require('covid19-api');
const COUNTRIES_LIST = require('./const')
const bot = new Telegraf('1170363720:AAFJ4ALJebB8Luh5kt1DStmYYqV3TparhKc')
bot.start( ctx => ctx.reply(`
${ctx.from.first_name}!
.
.
/help."
`))
bot.help( ctx => ctx.reply(COUNTRIES_LIST)) // covid19-api
bot.on('text', async (ctx) => {
try {
const userText = ctx.message.text
const covidData = await covidApi.getReportsByCountries(userText)
const countryData = covidData[0][0]
const formatData = `
: ${countryData.country},
: ${countryData.cases},
: ${countryData.deaths},
: ${countryData.recovered}`
ctx.reply(formatData)
} catch(e) {
ctx.reply(' , /help')
}
})
bot.launch()
Which works as we want:

Congratulations! We have completed the configuration of our telegram bot, which displays statistics of patients with coronavirus.