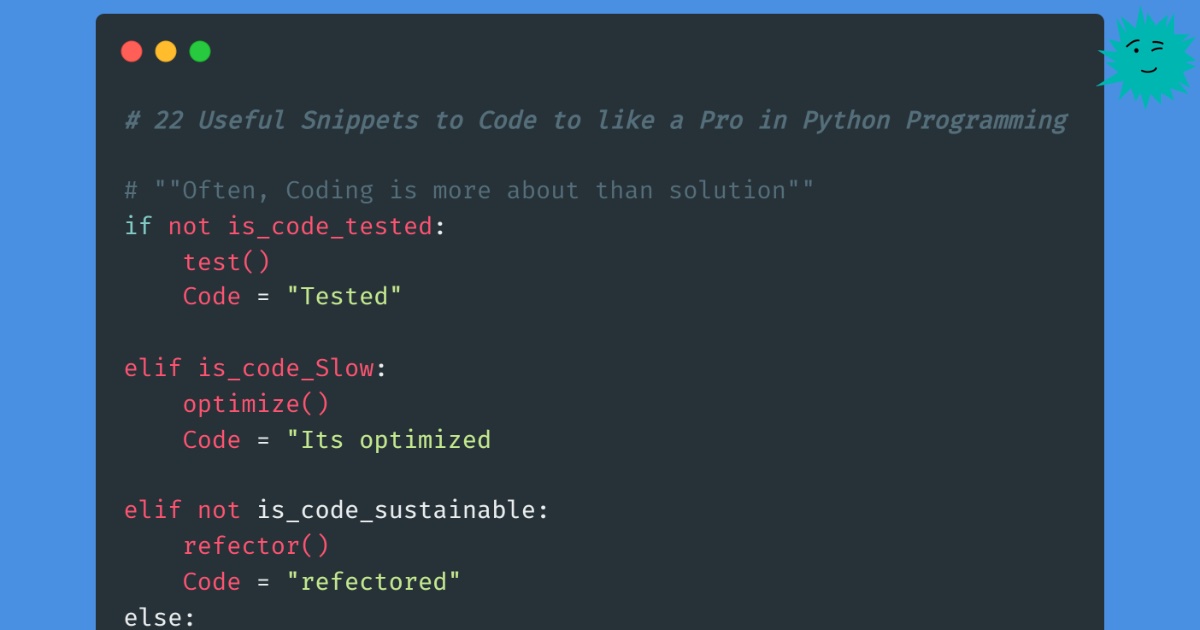
Python is one of the most popular programming languages ββand is extremely useful for solving everyday problems. In this article, I'll briefly share 22 useful code examples to take advantage of the power of Python.
You may have seen some of the examples before, while others will be new and interesting for you. All of these examples are easy to remember.
1. We get vowels
This example returns the found vowels in a string
"a e i o u"
. This can be useful when looking for or finding vowels.
def get_vowels(String):
return [each for each in String if each in "aeiou"]
get_vowels("animal") # [a, i, a]
get_vowels("sky") # []
get_vowels("football") # [o, o, a]
2. First uppercase letter
This example is used to capitalize every first letter of characters in a string. It works with a string of one or more characters and will be useful when parsing text or writing data to a file, etc.
def capitalize(String):
return String.title()
capitalize("shop") # [Shop]
capitalize("python programming") # [Python Programming]
capitalize("how are you!") # [How Are You!]
3. Print a line N times
This example can print any line n times without using Python loops.
n=5
string="Hello World "
print(string * n) #Hello World Hello World Hello World Hello World Hello World
4. Combining two dictionaries
This example merges two dictionaries into one.
def merge(dic1,dic2):
dic3=dic1.copy()
dic3.update(dic2)
return dic3
dic1={1:"hello", 2:"world"}
dic2={3:"Python", 4:"Programming"}
merge(dic1,dic2) # {1: 'hello', 2: 'world', 3: 'Python', 4: 'Programming'}
5. Calculate the execution time
This example is useful when you need to know how long it takes to execute a program or function.
import time
start_time= time.time()
def fun():
a=2
b=3
c=a+b
end_time= time.time()
fun()
timetaken = end_time - start_time
print("Your program takes: ", timetaken) # 0.0345
6. Exchange of values ββbetween variables
This is a quick way to swap two variables without using a third.
a=3
b=4
a, b = b, a
print(a, b) # a= 4, b =3
7. Checking for duplicates
This is the fastest way to check for duplicate values ββin a list.
def check_duplicate(lst):
return len(lst) != len(set(lst))
check_duplicate([1,2,3,4,5,4,6]) # True
check_duplicate([1,2,3]) # False
check_duplicate([1,2,3,4,9]) # False
8. Filtering False values
This example is used to eliminate all false values ββfrom a list eg
false, 0, None, " "
.
def Filtering(lst):
return list(filter(None,lst))
lst=[None,1,3,0,"",5,7]
Filtering(lst) #[1, 3, 5, 7]
9. Size in bytes
This example returns the length of a string in bytes, which is handy when you need to know the size of a string variable.
def ByteSize(string):
return len(string.encode("utf8"))
ByteSize("Python") #6
ByteSize("Data") #4
10. Occupied memory
The example allows you to get the amount of memory used by any variable in Python.
import sys
var1="Python"
var2=100
var3=True
print(sys.getsizeof(var1)) #55
print(sys.getsizeof(var2)) #28
print(sys.getsizeof(var3)) #28
11. Anagrams
This code is useful for checking if a string is an anagram. Anagram is a word obtained by rearranging the letters of another word.
from collections import Counter
def anagrams(str1, str2):
return Counter(str1) == Counter(str2)
anagrams("abc1", "1bac") # True
12. Sorting the list
This example sorts the list. Sorting is a commonly used task that can be done in many lines of code with a loop, but you can speed up your work with the built-in sort method.
my_list = ["leaf", "cherry", "fish"]
my_list1 = ["D","C","B","A"]
my_list2 = [1,2,3,4,5]
my_list.sort() # ['cherry', 'fish', 'leaf']
my_list1.sort() # ['A', 'B', 'C', 'D']
print(sorted(my_list2, reverse=True)) # [5, 4, 3, 2, 1]
13. Sorting the dictionary
orders = {
'pizza': 200,
'burger': 56,
'pepsi': 25,
'Coffee': 14
}
sorted_dic= sorted(orders.items(), key=lambda x: x[1])
print(sorted_dic) # [('Coffee', 14), ('pepsi', 25), ('burger', 56), ('pizza', 200)]
14. Getting the last item in the list
my_list = ["Python", "JavaScript", "C++", "Java", "C#", "Dart"]
#method 1
print(my_list[-1]) # Dart
#method 2
print(my_list.pop()) # Dart
15. Convert comma separated list to string
This code converts a comma separated list into a single string. It is useful when you need to concatenate the entire list with a string.
my_list1=["Python","JavaScript","C++"]
my_list2=["Java", "Flutter", "Swift"]
#example 1
"My favourite Programming Languages are" , ", ".join(my_list1)) # My favourite Programming Languages are Python, JavaScript, C++
print(", ".join(my_list2)) # Java, Flutter, Swift
16. Checking palindromes
This example shows how to quickly check for palindromes.
def palindrome(data):
return data == data[::-1]
palindrome("level") #True
palindrome("madaa") #False
17. List Shuffle
from random import shuffle
my_list1=[1,2,3,4,5,6]
my_list2=["A","B","C","D"]
shuffle(my_list1) # [4, 6, 1, 3, 2, 5]
shuffle(my_list2) # ['A', 'D', 'B', 'C']
18. Convert string to lower and upper case
str1 ="Python Programming"
str2 ="IM A PROGRAMMER"
print(str1.upper()) #PYTHON PROGRAMMING
print(str2.lower()) #im a programmer
19. String formatting
This code allows you to format the string. Formatting in Python means joining data from variables to a string.
#example 1
str1 ="Python Programming"
str2 ="I'm a {}".format(str1) # I'm a Python Programming
#example 2 - another way
str1 ="Python Programming"
str2 =f"I'm a {str1}" # I'm a Python Programming
20. Search for a substring
This example will be useful for finding a substring in a string. I am implementing it in two ways to avoid writing a lot of code.
programmers = ["I'm an expert Python Programmer",
"I'm an expert Javascript Programmer",
"I'm a professional Python Programmer"
"I'm a beginner C++ Programmer"
]
#method 1
for p in programmers:
if p.find("Python"):
print(p)
#method 2
for p in programmers:
if "Python" in p:
print(p)
21. Print on one line
We know that the print function does the output on each line, and if you use two print functions, they print on two lines. This example will show you how to execute output on the same line without moving to a new one.
# fastest way
import sys
sys.stdout.write("Call of duty ")
sys.stdout.write("and Black Ops")
# output: Call of duty and Black Ops
#another way but only for python 3
print("Python ", end="")
print("Programming")
# output: Python Programming
22. Splitting into fragments
This example will show you how to split a list into chunks and split it into smaller parts.
def chunk(my_list, size):
return [my_list[i:i+size] for i in range(0,len(my_list), size)]
my_list = [1, 2, 3, 4, 5, 6]
chunk(my_list, 2) # [[1, 2], [3, 4], [5, 6]]
Advertising
Servers for developers - a choice among an extensive list of preinstalled operating systems, the ability to use your own ISO to install the OS, a huge selection of tariff plans and the ability to create your own configuration in a couple of clicks, activate any server within a minute. Try it!
Subscribe to our chat on Telegram .
