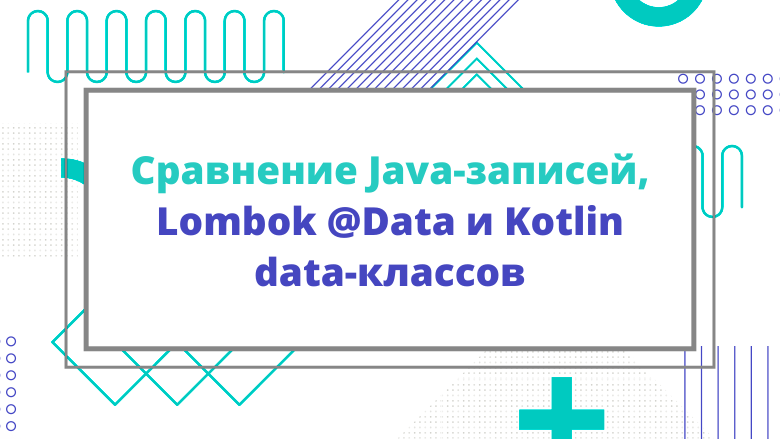
Despite the fact that all three solutions allow you to deal with boilerplate code, there is very little in common between them. Records have stronger semantics from which they have important advantages. Which often makes them the best choice, though not always.
I'm sure you've already seen examples of how to turn a regular POJO using records ...
class Range {
private final int low;
private final int high;
public Range(int low, int high) {
this.low = low;
this.high = high;
}
public int getLow() {
return low;
}
public int getHigh() {
return high;
}
@Override
public boolean equals(Object o) {
if (this == o)
return true;
if (o == null || getClass() != o.getClass())
return false;
Range range = (Range) o;
return low == range.low &&
high == range.high;
}
@Override
public int hashCode() {
return Objects.hash(low, high);
}
@Override
public String toString() {
return "[" + low + "; " + high + "]";
}
}
... in one line of code:
// (components)
record Range (int low, int hight) { }
, @Data
@Value
Lombok , :
@Data
class Range {
private final int low;
private final int high;
}
Kotlin, , , data-:
data class Range(val low: Int, val high: Int)
, ? . , .
, . , , . โ . JEP 395 , - , . .
(records)
JEP 395 :
(records) โ , .
, , , , , . , () . โ .
, . (, , @Data / @Value
data-), , , . .
( , .)
(transparency). ( Project Amber):
API , , .
:
( ) , , , ( API )
, ( ; API )
( API )
( API , )
Lombok data- Kotlin , "" ( Java, Kotlin ). Java ? , .
(set) โ . , , C โ {, , ...}, N โ {0, 1, ...}. {-2147483648, ..., 0, ..., 2147483647} โ , Java int
. null
, Integer
. ( null
) โ String
.
, , โ , . , โ " , " ( ), โ โ " " (), โ .
class Pair {
private final int first;
private final int second;
}
Pair
, . , . , , . int ร int ( ).
// given: bijective function from int to int
IntUnaryOperator increment =
i -> i == Integer.MAX_VALUE ? Integer.MIN_VALUE : ++i;
// then: combining two `increment`s yields a bijective function
// (this requires no additional proof or consideration)
UnaryOperator<Pair> incrementPair =
pair -> new Pair(
increment.applyAsInt(pair.first()),
increment.applyAsInt(pair.second()));
Pair::first
Pair::second
? , . / , Pair
. , , , , pair
.
, , , . , "-" ( ), .
. JEP 395 :
.
"" , , . , , int ร int
, , Pair(int first, int second)
Range(int low, int high)
. (range.get1()
), (record.low()
).
: - , , . , , . .
, :
( ) .
.
.
.
.
, , , .
if (range instanceof Range(int low, int high) && high < low)
return new Range(high, low);
, . , range โ , : low high โ .
with
Range range = new Range(5, 10);
// SYNTAX IS MADE UP!
Range newRange = range with { low = 0; }
// range: [5; 10]
// newRange: [0; 10]
, , , newRange
, range
low
: , . :
(,
low
,high
)
with
( , .)
, JSON / XML- , , . , . , , Reflection API .
, , Inside Java Podcast, episode 14 ( Spotify). , .
. , -, :
( )
, , (0, 0) = (0, 0), equals
, hashCode
.
( toString
) , , , .
. , , , , . , final
. ( ).
, ? . 10% , 90% , .
Lombok @Data/@Value
Lombok . , . , , Lombok, , .
( Lombok. API , , , , , Java. , , .)
data- Kotlin
, . .
, , , , . , data- , ( "", , ...), Lombok ( , copy, ...). , data- , Kotlin . .
@JvmRecord Kotlin : ", data- โ " ( , ). , . ?
Data- , , , . Kotlin @JvmRecord
data- , data-. , data- โ .
@JvmRecord
? . proposal:
Kotlin JVM-, :
Java- Kotlin ABI;
Kotlin - , Java reflection .
, case- Scala. , , , , , , , .
. , .
"Java Developer. Professional" : ยซ ยป.