
Here's a short list of what you'll learn:
• How to organize your code using variables.
• How functions make your code reusable.
• How to work with loops and conditions.
• What are global and local scopes.
• What are closures.
• How to write comments correctly.
• The main types of objects that you will encounter in JavaScript.
• How to work with text and perform standard string operations.
• How to use arrays to process lists.
• How to create your own objects.
ABOUT PIZZA, TYPES, PRIMITIVES AND OBJECTS
IN THIS CHAPTER:
- we will analyze the essence and features of objects;
- Let's get acquainted with basic types in JavaScript;
- Find out that pizza not only tastes great but also has educational value.
It's time to get down to serious business. Super serious! In the last few chapters, we've explored a variety of values, including: strings (text), numbers, booleans (true and false), functions, and other built-in JavaScript elements.
Here are some examples to refresh your memory:
let someText = "hello, world!";
let count = 50;
let isActive = true;
Unlike other languages, JavaScript makes it easy to define and use these built-in elements. We don't even need to make a plan for their future use. But for all its simplicity, there are many hidden details. And their knowledge is important, since it not only makes it easier to understand the code, but also speeds up the identification of the causes of its malfunctions.
As you might have guessed, inline elements are not the best way to describe the different values used in JS. There is a more formal name for such values, namely types. In this chapter, we will begin a smooth introduction to their essence and purpose.
Go!
Let's talk about pizza first
Since I constantly eat something (or think about what to eat), I will try to explain the mysterious world of types using a simpler example - the world of pizza.
If you haven't eaten it for a long time, then let me remind you how it looks:
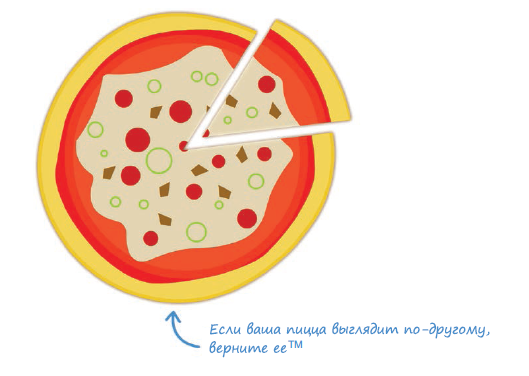
Of course, pizza doesn't come out of nowhere like this. It is created from simple and complex ingredients:
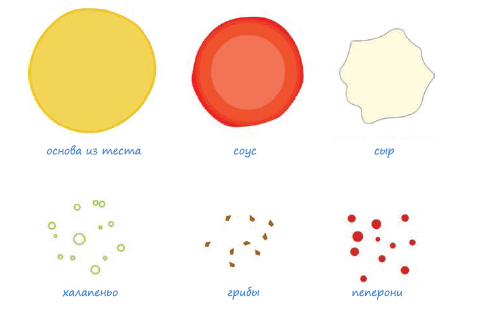
Simple ingredients are easy to identify. These are mushrooms and jalapenos. The reason we call them simple is that they cannot be decomposed into their component parts:
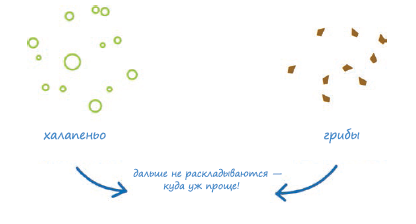
They are not manufactured or assembled from other components.
Complex ingredients include cheese, sauce, dough base and pepperoni. What makes them difficult is that they are made with other ingredients:
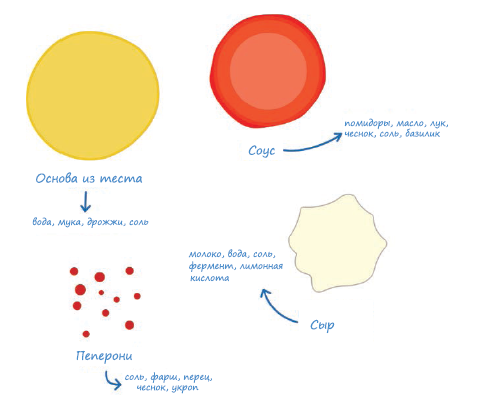
Unfortunately, ingredients like cheese and pepperoni are never easy. To prepare them, you need to mix, fry and add various components. In addition, their preparation is not limited to mixing simple ingredients, but may also require complex combinations.
From pizza to JavaScript
Everything we learned about pizza in the previous section was for good reason. The description of simple and complex ingredients is quite applicable to types in JavaScript. Each individual ingredient can be thought of as an analogue of the type that you can use (Figure 12.1).
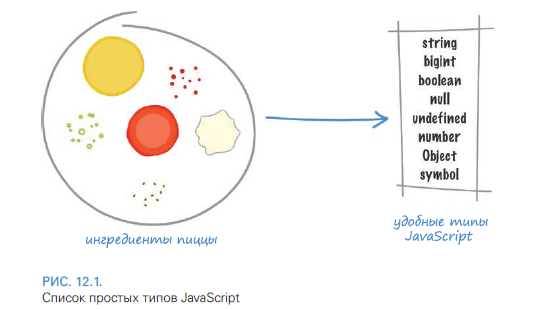
Like cheese, sauce, pepperoni, mushrooms and bacon in our pizza, types in JavaScript are string, number, boolean, null, undefined, bigint. , symbol (symbols) and Object (object). Some of these types you may already be familiar with, some you may not. We will consider them in more detail in the future, now in table. 12.1 you can see a brief description of their purpose.
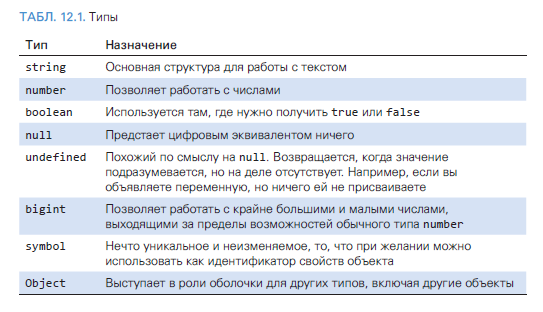
As we can see, each type has its own unique purpose. At the same time, they, similar to pizza ingredients, are also divided into simple and complex. Only in JavaScript terminology, simple and complex types are called primitives (primitive types) and objects (object types), respectively.
Primitive types include string, number, boolean, null, bigint, symbol, and undefined. Any values falling within their jurisdiction are not subject to division into parts. They are jalapenos and mushrooms in the JavaScript world. Primitives are fairly easy to define and format into easy-to-understand elements. There is no depth in them, and when we meet with them, we, as a rule, get what we see initially.
Object types, represented as Object in the above table, are more cryptic. Therefore, before proceeding to the description of the details of all the listed types, it is worth considering separately what exactly the objects are.
What is an object?
The principle of objects in programming languages like JavaScript perfectly captures their real-life analogy, in which we are all literally surrounded by objects. These include your computer, a book on a shelf, a potato (controversial), an alarm clock, a poster ordered from eBay, etc. You can go on and on.
Some objects like paperweights are of little use and can be inactive for a long time.
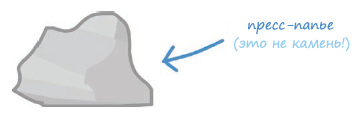
Other objects, like a TV set, are already moving beyond mere existence and performing many tasks:
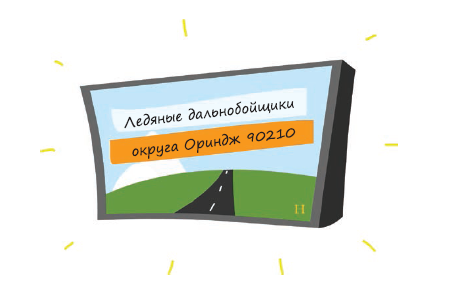
A regular TV receives a signal, allows you to turn it on and off, flip channels, adjust the volume, and more.
It is important to understand here that objects have different shapes, sizes and purposes. Despite these differences, at the top level they are all the same and represent an abstraction. They make it possible to use them without wondering about their internal structure. Even the simplest objects conceal a certain level of complexity that you don't need to worry about.
For example, it doesn't matter what exactly happens inside the TV, how the wires are soldered or what kind of glue was used to connect the parts. None of this matters. All you are interested in is for the TV to fulfill its purpose. He must regularly switch channels, allow to adjust the volume, etc. The rest is unnecessary troubles.
Basically, an object can be thought of as a black box. There are a number of predefined / described actions that he performs. Seeing how he does it is not easy enough. In fact, you are not interested in this as long as he does everything right. We'll change our mind about this later when we learn how to create the insides of an object, but for now, enjoy the simplicity of this world.
Predefined objects in JavaScript
In addition to the built-in types listed earlier, JS also comes with predefined objects out of the box. These objects let you work with anything, including datasets, dates, text, and numbers. Table 12.2 provides a list similar to the previous one, describing their purpose:

Using built-in objects is slightly different from using primitives. Each object in this regard is special in its own way. I will postpone a detailed explanation of all these features of use for later, but here I will give a short code snippet with a comment that will show the possible options:
//
let names = ["Jerry", "Elaine", "George", "Kramer"];
let alsoNames = new Array("Dennis", "Frank", "Dee", "Mac");
//
let roundNumber = Math.round("3.14");
//
let today = new Date();
// boolean
let booleanObject = new Boolean(true);
//
let unquantifiablyBigNumber = Number.POSITIVE_INFINITY;
// string
let hello = new String("Hello!");
It may be a little perplexing to you that the primitives string, boolean, symbol, bigint, and number can also exist in the form of objects. Outwardly, this object form looks very similar to the primitive one. Here's an example:
let movie = "Pulp Fiction";
let movieObj = new String("Pulp Fiction");
console.log(movie);
console.log(movieObj);
When you output both options, you will see the same result. Internally, however, movie and movieObj are quite different. The first is literally a primitive of type string, and the second is of type Object. This leads to interesting (and sometimes confusing) behavior, which I will gradually introduce as I explore the built-in types.
, , . , , . . , . , .
, . , .
More details about the book can be found on the website of the publishing house
» Table of Contents
» Excerpt
Electronic version of the book - color
For Habitants a 25% discount on coupon - JavaScript
Upon payment for the paper version of the book, an e-book is sent to the e-mail.