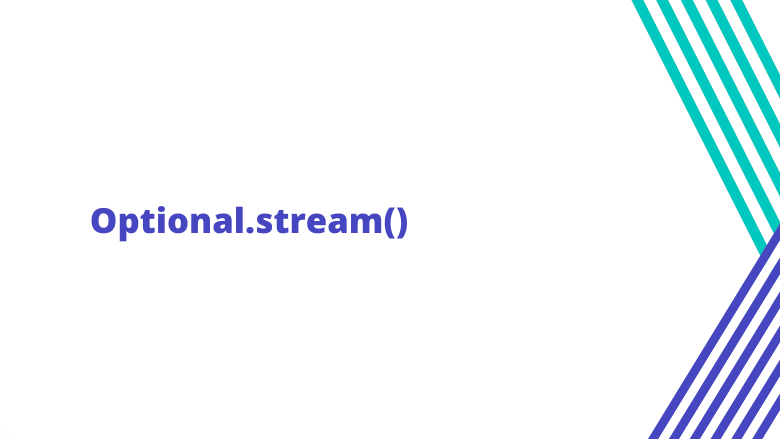
This week I learned about an interesting "new" feature of Optional that I want to talk about in this post. It has been available since Java 9, so the newness is relative.
Let's start with the following sequence for calculating the total order price:
public BigDecimal getOrderPrice(Long orderId) {
List<OrderLine> lines = orderRepository.findByOrderId(orderId);
BigDecimal price = BigDecimal.ZERO;
for (OrderLine line : lines) {
price = price.add(line.getPrice());
}
return price;
}
Provide variable-accumulator for price
Add the price of each line to the total price
, , . :
public BigDecimal getOrderPrice(Long orderId) {
List<OrderLine> lines = orderRepository.findByOrderId(orderId);
return lines.stream()
.map(OrderLine::getPrice)
.reduce(BigDecimal.ZERO, BigDecimal::add);
}
orderId
: null
.
null
, - :
public BigDecimal getOrderPrice(Long orderId) {
if (orderId == null) {
throw new IllegalArgumentException("Order ID cannot be null");
}
List<OrderLine> lines = orderRepository.findByOrderId(orderId);
return lines.stream()
.map(OrderLine::getPrice)
.reduce(BigDecimal.ZERO, BigDecimal::add);
}
, orderId
Optional. Optional:
public BigDecimal getOrderPrice(Long orderId) {
return Optional.ofNullable(orderId)
.map(orderRepository::findByOrderId)
.flatMap(lines -> {
BigDecimal sum = lines.stream()
.map(OrderLine::getPrice)
.reduce(BigDecimal.ZERO, BigDecimal::add);
return Optional.of(sum);
}).orElse(BigDecimal.ZERO);
}
orderId
Optional
flatMap()
,Optional<BigDecimal>
;map()
Optional<Optional<BigDecimal>>
Optional
, .
Optional
,0
Optional
! , .
, Optional
stream()
( Java 9). :
public BigDecimal getOrderPrice(Long orderId) {
return Optional.ofNullable(orderId)
.stream()
.map(orderRepository::findByOrderId)
.flatMap(Collection::stream)
.map(OrderLine::getPrice)
.reduce(BigDecimal.ZERO, BigDecimal::add);
}
:

, . , , , .
"Java Developer. Basic". , , .