Some essential tools for a rock-star developer
Hello, Habr. As part of the recruitment for the " React.js Developer " course , we prepared a translation of the material.
We invite everyone to the open demo lesson "Webpack and babel" . In this lesson, we will look at modern and powerful JavaScript chips - Webpack and Babel. We will show you how to create a React project from scratch using Webpack. Join us!
ReactJS has high performance by default. But from time to time you have a chance to make it even better. And the wonderful React community has come up with some fantastic libraries for that.
Today we're going to talk about seven such libraries that can improve the quality of your code while improving performance.
Let's start.
... ... ...
1. React Query
, React Query, React.
. , . . , Redux.
React Query
. .
const useFetch = (url) => {
const [data, setData] = useState();
const [isLoading, setIsLoading] = useState(false);
const [error, setError] = useState(false);
useEffect(() => {
const fetchData = async () => {
setIsError(false);
setIsLoading(true);
try {
const result = await fetch(url);
setData(result.data);
} catch (error) {
setError(error);
}
setIsLoading(false);
};
fetchData();
}, [url]);
return {data , isLoading , isError}
}
() React Query
, React Query. , .
import { useQuery } from 'react-query'
const { isLoading, error, data } = useQuery('repoData', () =>
fetch(url).then(res =>res.json()
)
)
, .
. . .
2. React Hook Form
React Hook Form - , .
-.
(UI)
, , React Hook Form .
React Hook Form
.
function LoginForm() {
const [email, setEmail] = React.useState("");
const [password, setPassword] = React.useState("");
const handleSubmit = (e: React.FormEvent) => {
e.preventDefault();
console.log({email, password});
}
return (
<form onSubmit={handleSubmit}>
<input
type="email"
id="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
/>
<input
type="password"
id="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
/>
</form>
);
}
React Form
React Hook Form.
function LoginForm() {
const { register, handleSubmit } = useForm();
const onSubmit = data => console.log(data);
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input {...register("email")} />
<input {...register("password")} />
<input type="submit" />
</form>
);
}
. .
. . .
3. React Window
React Window . , 1 000 . , 1000 .
. .
1 000
import React, {useEffect, useState} from 'react';
const names = [] // 1000 names
export const LongList = () => {
return <div>
{names.map(name => <div> Name is: {name} </div>)}
<div/>
}
1000 , 10-20 .
React Window
React Window.
import { FixedSizeList as List } from 'react-window';
const Row = ({ index, style }) => <div style={style}> Name is {names[index]}</div>
const LongList = () => (
<List
height={150}
itemCount={1000}
itemSize={35}
width={300}
>
{Row}
</List>
);
, . , , .
. . .
4. React LazyLoad
- , , . , , .
React LazyLoad - , . , .
LazyLoad
, .
import React from 'react';
const ImageList = () => {
return <div>
<img src ='image1.png' />
<img src ='image2.png' />
<img src ='image3.png' />
<img src ='image4.png' />
<img src ='image5.png' />
</div>
}
LazyLoad
LazyLoad.
import React from 'react';
import LazyLoad from 'react-lazyload';
const ImageList = () => {
return <div>
<LazyLoad> <img src ='image1.png' /> <LazyLoad>
<LazyLoad> <img src ='image2.png' /> <LazyLoad>
<LazyLoad> <img src ='image3.png' /> <LazyLoad>
<LazyLoad> <img src ='image4.png' /> <LazyLoad>
<LazyLoad> <img src ='image5.png' /> <LazyLoad>
</div>
}
. . .
5. (Why Did You Render)
React-. , .
, Why Did You Render, . , , .
.
import React, {useState} from 'react'
const WhyDidYouRenderDemo = () => {
console.log('render')
const [user , setUser] = useState({})
const updateUser = () => setUser({name: 'faisal'})
return <>
<div > User is : {user.name}</div>
<button onClick={updateUser}> Update </button>
</>
}
export default WhyDidYouRenderDemo
.
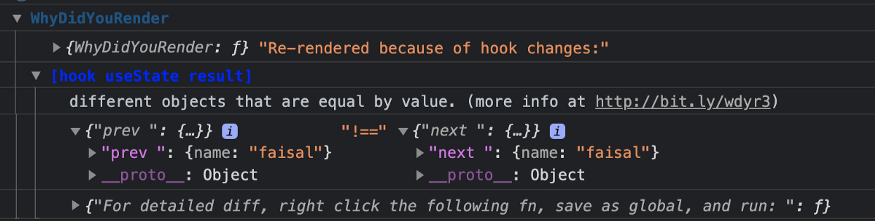
, , .
. . .
6. Reselect
Redux, . , Redux , - , , , - .
Reselect , , .
, Redux .
. , .
. .
.
import { createSelector } from 'reselect'
const shopItemsSelector = state => state.shop.items
const subtotalSelector = createSelector(
shopItemsSelector,
items => items.reduce((subtotal, item) => subtotal + item.value, 0)
)
const exampleState = {
shop: {
items: [
{ name: 'apple', value: 1.20 },
{ name: 'orange', value: 0.95 },
]
}
}
. . .
7. Deep Equal
Deep Equal - , . . JavaScript, , , , .
.
const user1 = {
name:'faisal'
}
const user2 ={
name:'faisal'
}
const normalEqual = user1 === user2 // false
( ), ( ) .
Deep Equal, 46 . , .
var equal = require('deep-equal');
const user1 = {
name:'faisal'
}
const user2 ={
name:'faisal'
}
const deepEqual = equal(user1 , user2); // true -> exactly what we wanted!
. . .
. , React.
, . !