Translated for Hexlet.io
In computer programming, a naming convention is a set of rules for choosing a sequence of characters to be used for identifiers that denote variables, types, functions, and other objects in source code and documentation - Wikipedia
Coming up with names is hard!
In this article, we will focus on the (P) A / HC / LC naming technique in order to improve the readability of the code. These guidelines can be applied to any programming language, the article uses JavaScript for code examples.
What does (P) A / HC / LC mean?
This practice uses the following pattern for naming a function:
? (P) + (A) + (HC) + ? (LC)
What does the prefix (P) stand for?
The prefix expands the meaning of the function.
- is
Describes a property or state of the current context (usually a boolean value).
const color = 'blue';
const isBlue = (color === 'blue'); //
const isPresent = true; //
if (isBlue && isPresent) {
console.log('Blue is present!');
}
- has
Indicates whether the current context has a specific value or state (usually a boolean value).
/* */
const isProductsExist = (productsCount > 0);
const areProductsPresent = (productsCount > 0);
/* */
const hasProducts = (productsCount > 0);
- should
Reflects a positive conditional operator (usually a Boolean value) associated with a specific action.
const shouldUpdateUrl = (url, expectedUrl) => url !== expectedUrl;
Action is the heart of function
Action is the verb part of the function name. This is the most important part in describing what a function does.
- get
( getter ).
function getFruitsCount() {
return this.fruits.length;
}
- set
A B.
let fruits = 0;
const setFruits = (nextFruits) => {
fruits = nextFruits;
};
setFruits(5);
console.log(fruits); // 5
- reset
.
const initialFruits = 5;
let fruits = initialFruits;
setFruits(10);
console.log(fruits); // 10
const resetFruits = () => {
fruits = initialFruits;
};
resetFruits();
console.log(fruits); // 5
- fetch
, (, ).
const fetchPosts = (postCount) => fetch('https://api.dev/posts', {...});
- remove
- -.
, , — removeFilter
, deleteFilter
( ):
const removeFilter = (filterName, filters) => filters.filter((name) => name !== filterName);
const selectedFilters = ['price', 'availability', 'size'];
removeFilter('price', selectedFilters);
- delete
-. .
, , , . delete-post, CMS deletePost
, removePost
.
const deletePost = (id) => database.find({ id }).delete();
- compose
. , .
const composePageUrl = (pageName, pageId) => `${pageName.toLowerCase()}-${pageId}`;
- handle
. .
const handleLinkClick = () => {
console.log('Clicked a link!');
};
link.addEventListener('click', handleLinkClick);
— , .
— -. , , , .
/* , */
const filter = (list, predicate) => list.filter(predicate);
/* , */
const getRecentPosts = (posts) => filter(posts, (post) => post.date === Date.now());
/*
.
, JavaScript (Array).
filterArray .
*/
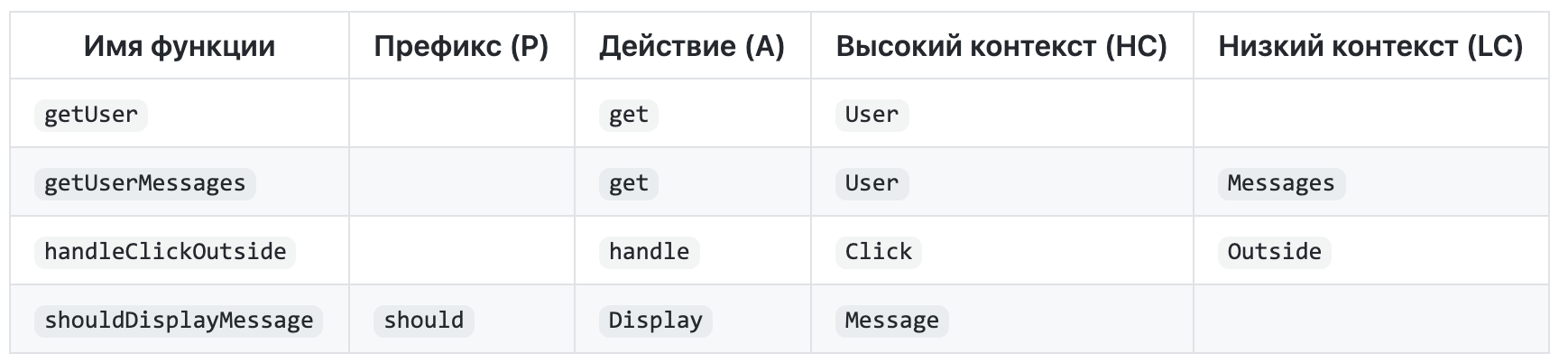
, .
1. S-I-D
(Short), (Intuitive) (Descriptive).
/* */
const a = 5; // "a"
const isPaginatable = (postsCount > 10); // "Paginatable"
const shouldPaginatize = (postsCount > 10); // - !
/* */
const postsCount = 5;
const hasPagination = (postsCount > 10);
const shouldDisplayPagination = (postsCount > 10); //
2.
Don't use abbreviations. Usually they only worsen the readability of the code. Finding a short, descriptive name can be difficult, but abbreviations cannot be an excuse not to. For example:
/* */
const onItmClk = () => {};
/* */
const onItemClick = () => {};
3. Avoid duplicate context
Always remove context from a name if it does not impair readability.
class MenuItem {
/* ( "MenuItem") */
handleMenuItemClick(event) {
...
}
/* MenuItem.handleClick() */
handleClick(event) {
...
}
}
4. Reflect the expected result in the name
/* */
const isEnabled = (itemsCount > 3);
return <Button disabled={!isEnabled} />
/* */
const isDisabled = (itemsCount <= 3);
return <Button disabled={isDisabled} />
5. Consider the singular / plural
Like the prefix, variable names can be singular or plural depending on whether they have one or more meaning.
/* */
const friends = 'Bob';
const friend = ['Bob', 'Tony', 'Tanya'];
/* */
const friend = 'Bob';
const friends = ['Bob', 'Tony', 'Tanya'];
6. Use meaningful and pronounced names
/* */
const yyyymmdstr = moment().format("YYYY/MM/DD");
/* */
const currentDate = moment().format("YYYY/MM/DD");