Recently, I got an internship at a new IT company and our (my team's) project was a telegram bot that automates part of the work of hr managers. The first week was given to us to self-study whatever we deem necessary (and I am convinced that the best way to learn something is practice), so I started to act. The programming language was chosen python (probably it is clear from the cover why), so in this article I will analyze an example with it.
BotFather
To create a cable-bot, you can write a user @BotFather command / newbot . It will ask for the name and @username for the future bot. Nothing complicated here - it tells you everything (the main thing is that @username is not busy and ends with "bot"). BotFather will send an HTTP API token, which we will use to work with the bot.
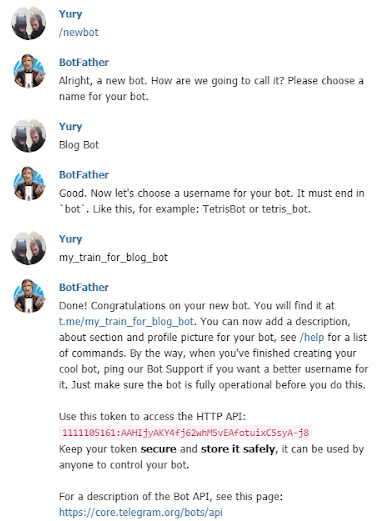
Telebot and the power of python
It always seemed to me that creating a bot is not so easy. To be honest, I wanted to try for a long time, but either I didn’t have enough time (I thought it would take more than one evening), or I could not choose the technology (I somehow watched the tutorial for c #), but most likely it was just laziness. But then I needed it to work, so I dared not put it off any longer.
python . , , . ( ) 6 (!) . :
import telebot
bot = telebot.TeleBot('1111105161:AAHIjyAKY4fj62whM5vEAfotuixC5syA-j8')
@bot.message_handler(commands=['start'])
def start_command(message):
bot.send_message(message.chat.id, "Hello!")
bot.polling()
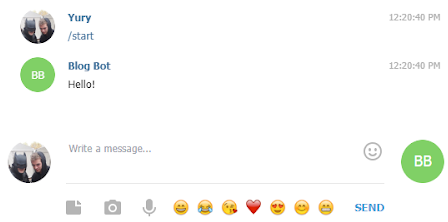
/start, . : telebot ( pyTelegramBotAPI pip install pyTelegramBotAPI
( pip install telebot
!), , , BotFather. , ( “/start”), , , “Hello!”. , , , . - , "" .
Flask & Requests
Telebot, , , “”. , hr- (), / / . , . python - flask. , 5000- (http://localhost:5000/):
from flask import Flask
app = Flask(__name__)
@app.route("/", methods=["GET"])
def index():
return "Hello, World!"
if __name__ == "__main__":
app.run()
, . (habr), . , “Hello!” :
from flask import Flask, request
import requests
app = Flask(__name__)
def send_message(chat_id, text):
method = "sendMessage"
token = "1111105161:AAHIjyAKY4fj62whM5vEAfotuixC5syA-j8"
url = f"https://api.telegram.org/bot{token}/{method}"
data = {"chat_id": chat_id, "text": text}
requests.post(url, data=data)
@app.route("/", methods=["POST"])
def receive_update():
chat_id = request.json["message"]["chat"]["id"]
send_message(chat_id, "Hello!")
return "ok"
if __name__ == "__main__":
app.run()
, . , . , , . telegram API setWebhook. , url, ( http://localhost:5000/). , : " localhost", localhost . , setWebhook https url-. ngrok, . ngrok, “ngrok http 5000”. :
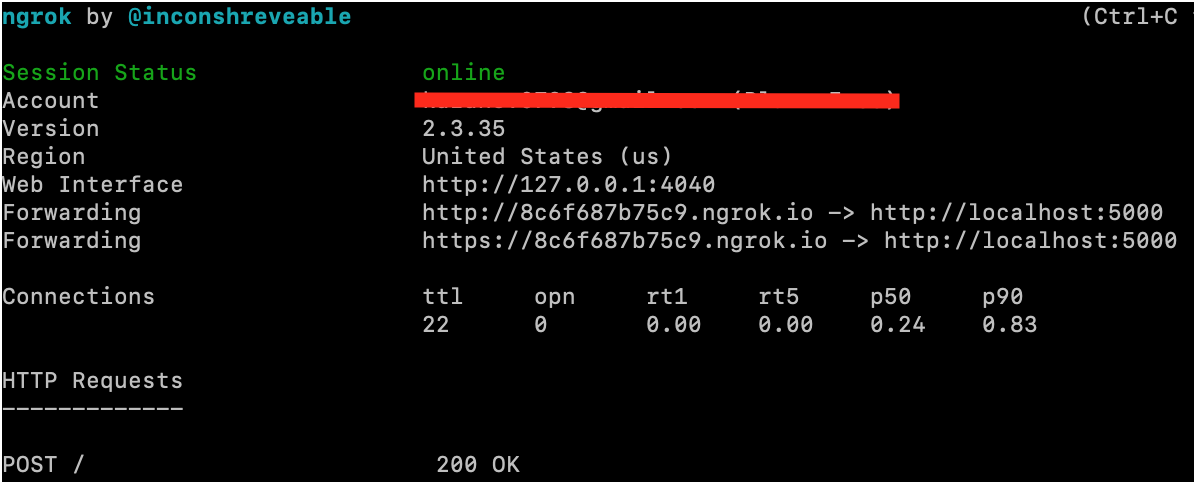
setWebhook, , postman. post https://api.telegram.org/bot<>/setWebhook url. :
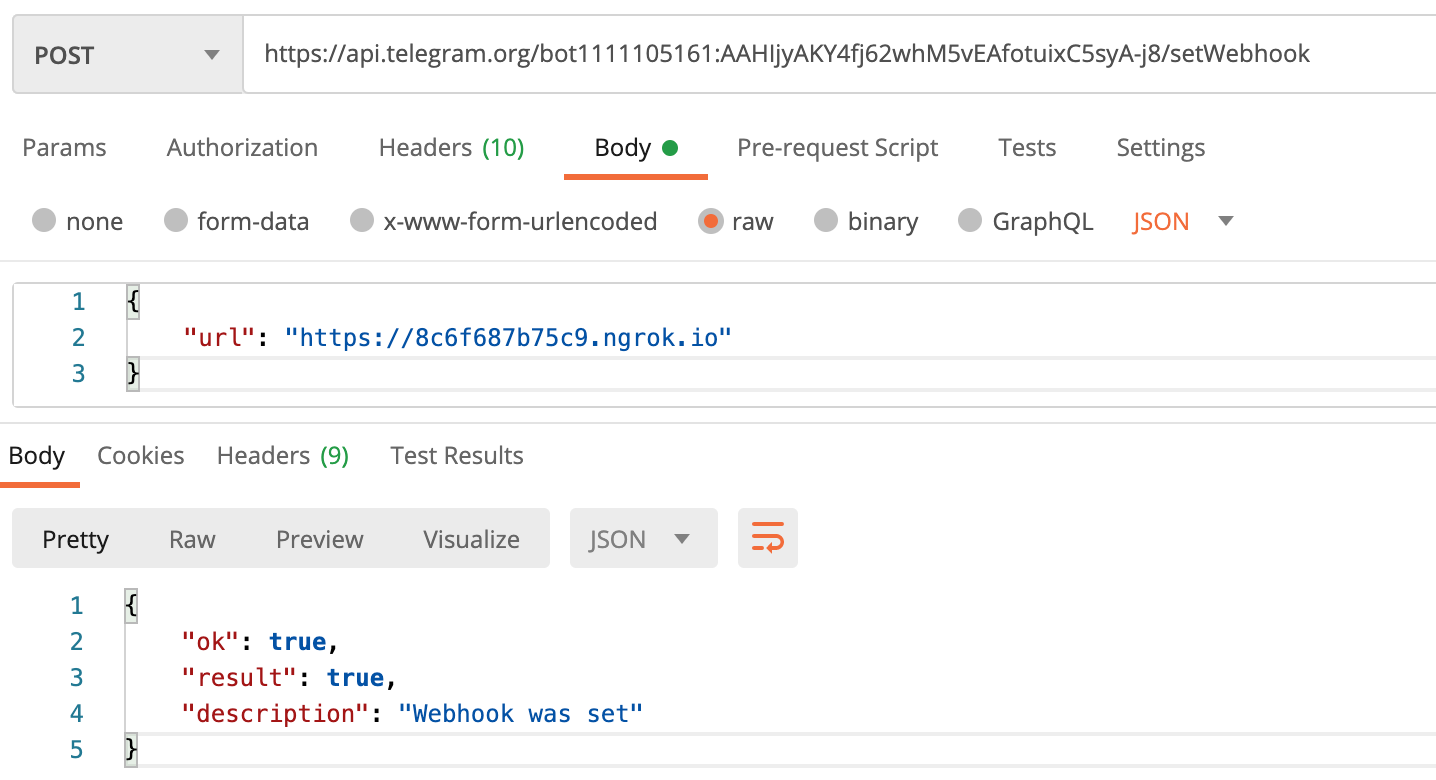
telebot, . , flask’e, . ? -, send_message . :
from flask import Flask, request
import telebot
app = Flask(__name__)
bot = telebot.TeleBot('1111105161:AAHIjyAKY4fj62whM5vEAfotuixC5syA-j8')
@app.route("/", methods=["POST"])
def receive_update():
chat_id = request.json["message"]["chat"]["id"]
bot.send_message(chat_id, "Hello!")
return "ok"
if __name__ == "__main__":
app.run()
, , , , @bot.message_handler - , (, , , . .). , flask , telebot. bot.polling(), “ ”. , - . , :
from flask import Flask, request
import telebot
bot = telebot.TeleBot('1111105161:AAHIjyAKY4fj62whM5vEAfotuixC5syA-j8')
bot.set_webhook(url="https://8c6f687b75c9.ngrok.io")
app = Flask(__name__)
@app.route('/', methods=["POST"])
def webhook():
bot.process_new_updates(
[telebot.types.Update.de_json(request.stream.read().decode("utf-8"))]
)
return "ok"
@bot.message_handler(commands=['start'])
def start_command(message):
bot.send_message(message.chat.id, 'Hello!')
if __name__ == "__main__":
app.run()
Here we use the set_webhook method, similar to how we did it earlier through postman, and on an empty route we write "a little magic" to successfully receive bot updates. Of course, this is not a very good way, and in the future it is better to write the functionality for processing incoming messages yourself. But first, I think this is the best solution.
Conclusion
It's really not that difficult to write a telegram bot in python, but there are also pitfalls that I tried to talk about in this article. Of course, this is just the beginning, but the last piece of code can be used as a template for the server side, which will work with the telegram bot. Then it remains only to increase the functionality at your discretion.