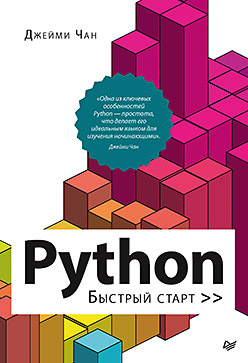
Everyone was once newbies. We often forget about this, but new generations appear who want to learn an "unfamiliar language" as quickly as possible and with a minimum of effort.
Do not judge newcomers harshly, if you are a programming guru, you do not need this book, but it may be useful for your child, brother, sister, friend or girlfriend;)
The Quick Beginnings series is a great solution, and here's why: complex concepts are broken down into simple steps - you can master the Python language, even if you have never done any programming before; all fundamental concepts are backed up by real examples; you will get a complete understanding of Python: control structures, error handling techniques, object-oriented programming concepts, and more; at the end of the book you will find an interesting project that will help you to assimilate the acquired knowledge.
Control commands
Congratulations! You have reached the most interesting chapter. Hope you enjoy it so far. In this chapter, we'll talk about how to make your program smarter - so that it can make its own choices and decisions. In particular, consider the if statement, for and while loops. They are known as control flow tools and control the flow of a program. We'll also look at the try / except construct, which determines what the program should do when an error occurs.
But before moving on to these flow control tools, let's take a look at the conditional statements.
6.1. Conditional statements
All flow control tools include condition assessment. The program will behave differently depending on whether any condition is met.
The most common conditional operator is the comparison operator. If we want to compare if two variables are the same, then we use the == (double =) operator. For example, the expression x == y asks the program to check if the value of x is equal to the value of y.
If they are equal, then the condition is satisfied and the operator will return True. Otherwise, the expression will be False.
Other comparison operators include! = (not equal), <(less than),> (greater than), <= (less or equal), and> = (greater or equal). The list below shows examples of use and shows cases that are True.
Not equal:
5! = 2
Greater than:
5> 2
Less than:
2 <5
Greater than or equal:
5> = 2
5> = 5
Less than or equal:
2 <= 5
2 <= 2
There are three logical operators: and, or, not, which can be used to combine multiple conditions.
The and operator returns True if all conditions are met. Otherwise, it will return False. For example, the expression 5 == 5 and 2> 1 will return True because both conditions are true.
The or operator returns True if at least one of thecondition. Otherwise, it will return False. The expression 5> 2 or 7> 10 or 3 == 2 will return True because the first condition 5> 2 is true.
The not operator returns True if the condition after the not keyword is false. Otherwise, it will return False. The expression not 2> 5 will return True because 2 is less than 5.
6.2. IF instructions
The if statement is one of the most commonly used control flow statements. It allows the program to evaluate whether a certain condition is met and to take the appropriate action based on the result of the evaluation. The structure of the if statement looks like this:
if 1:
A
elif 2:
B
elif 3:
C
elif 4:
D
else:
E
elif means "else if" and you can use as many elif statements as you like.
If you've written code before, for example, in C or Java, you'll be surprised to see that Python doesn't need the parentheses () after the if, elif, and else keywords. Also, Python does not use curly braces {} to define the start and end of an if statement. Python uses indentation. Anything indented is treated as a block of code that will be executed if the condition evaluates to True.
To fully understand how the if statement works, run IDLE and enter the following code:
userInput = input('Enter 1 or 2: ')
if userInput == "1":
print ("Hello World")
print ("How are you?")
elif userInput == "2":
print ("Python Rocks!")
print ("I love Python")
else:
print ("You did not enter a valid number")
The program prompts the user for input using the input () function. The result is stored in the userInput variable as a string.
Then the if userInput == "1": statement compares the userInput variable to the string "1". If the value stored in userInput is "1", the program will execute all indented statements until the indentation ends. This example will print "Hello World" followed by "How are you?"
If the value stored in user input is "2", the program will display "Python Rocks!" Followed by "I love Python".
For all other values, the message "You did not enter a valid number" appears.
Run the program three times, enter 1, 2 and 3 respectively for each run. The result will be as follows:
Enter 1 or 2: 1
Hello World
How are you?
Enter 1 or 2: 2
Python Rocks!
I love Python
Enter 1 or 2: 3
You did not enter a valid number
More details about the book can be found on the website of the publishing house
ยป Table of Contents
ยป Excerpt
For Habitants a 25% discount on coupon - Python
Upon payment for the paper version of the book, an e-book is sent to the e-mail.