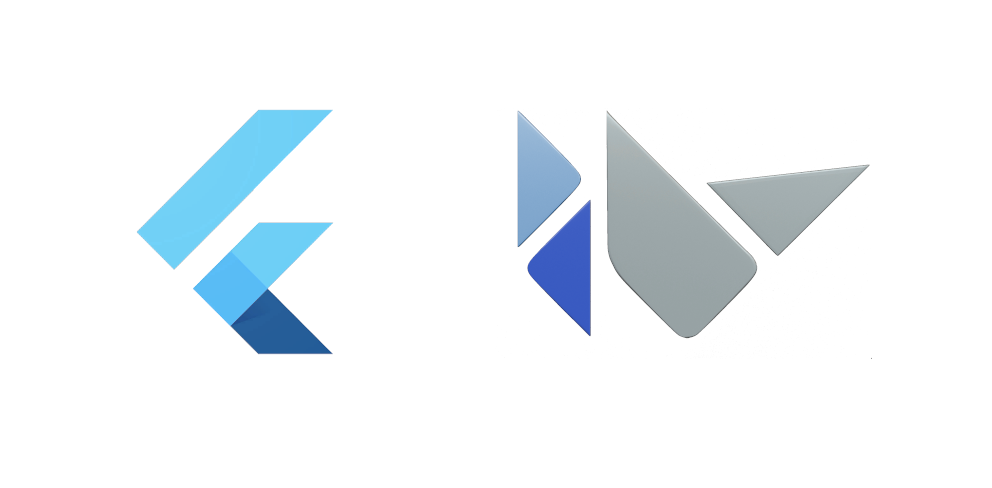
Kivy and Flutter are two open source frameworks for cross-platform development.
Flutter:
- Created by Google and released in 2017;
- uses Dart as a programming language;
- does not use native components, drawing the entire interface inside its own graphics engine;
Kivy:
- created by the Kivy community in 2010;
- uses Python as a programming language and its own declarative language for marking up UI elements - KV Language;
- does not use native components, drawing the entire interface using OpenGL ES 2.0 and SDL2;
Recently, on the open spaces of YouTube, I came across a video demonstration of the Flutter application - Facebook Desktop Redesign built with Flutter Desktop . Great demo application in material design style! And since I am one of the developers of the KivyMD library (a set of material components for the Kivy framework), I wondered how easy it would be to make the same beautiful interface. Fortunately, the author left a link to the project repository .


Which app in the above screenshots do you think is written using Flutter and which one is using Kivy? It is difficult to answer straight away, since there are no pronounced differences. The only thing that immediately catches your eye (bottom screenshot) is that there is still no normal anti-aliasing in Kivy. And this is sad, but not critical. We will compare individual elements of the application and their source code in Dart (Flutter) and Python / KV language (Kivy).
Now let's see how the components look from the inside ...
StoryCard
Kivy
Card markup in KV-Language:

Base Python class:
from kivy.properties import StringProperty
from kivymd.uix.relativelayout import MDRelativeLayout
class StoryCard(MDRelativeLayout):
avatar = StringProperty()
story = StringProperty()
name = StringProperty()
def on_parent(self, *args):
if not self.avatar:
self.remove_widget(self.ids.avatar)
Flutter:
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
class Story extends StatefulWidget {
final String name;
final String avatar;
final String story;
const Story({
Key key,
this.name,
this.avatar,
this.story,
}) : super(key: key);
@override
_StoryState createState() => _StoryState();
}
class _StoryState extends State<Story> {
@override
Widget build(BuildContext context) {
return Container(
width: 150,
margin: const EdgeInsets.only(top: 30),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(30),
boxShadow: [
BoxShadow(
color: Colors.black.withOpacity(0.3),
blurRadius: 20,
offset: Offset(0, 10),
),
],
),
child: Stack(
overflow: Overflow.visible,
fit: StackFit.expand,
children: [
ClipRRect(
borderRadius: BorderRadius.circular(30),
child: Image.network(
widget.story,
fit: BoxFit.cover,
),
),
if (widget.avatar != null)
Positioned.fill(
top: -30,
child: Align(
alignment: Alignment.topCenter,
child: Container(
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(30),
boxShadow: [
BoxShadow(
color: Colors.black.withOpacity(0.4),
blurRadius: 5,
offset: Offset(0, 3),
),
],
),
child: ClipRRect(
borderRadius: BorderRadius.circular(30),
child: Image.network(
widget.avatar,
fit: BoxFit.cover,
width: 60,
height: 60,
),
),
),
),
),
if (widget.avatar != null)
Positioned.fill(
child: Align(
alignment: Alignment.bottomCenter,
child: Row(
children: [
Expanded(
child: Container(
padding: const EdgeInsets.all(15),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(30),
gradient: LinearGradient(
begin: Alignment.topCenter,
end: Alignment.bottomCenter,
colors: [
Colors.transparent,
Colors.black,
],
),
),
child: widget.name != null ? Text(
widget.name,
textAlign: TextAlign.center,
maxLines: 1,
overflow: TextOverflow.ellipsis,
style: TextStyle(
color: Colors.white,
fontWeight: FontWeight.bold,
),
) : SizedBox(),
),
),
],
),
),
),
],
),
);
}
}
As you can see, the code in Python and KV-Language is twice as short. The source code for the Python / Kivy project discussed in this article has a total size of 31 kilobytes. 3 kilobytes of this amount are Python code, the rest is KV-Language. The source code for Flutter is 54 kilobytes. However, there seems to be nothing to be surprised at - Python is one of the most laconic programming languages ββin the world.
We will not argue about which is better: to describe the UI using DSL languages ββor directly in the code. In Kivy, by the way, you can also build Python widgets with code, but this is not a very good solution.
TopBar
Flutter:

Kivy:

The implementation of this bar, including animation, in Python / Kivy took only 88 lines of code. Dart / Flutter has 325 lines and 9 kilobytes of disk space. Let's see what this widget is:
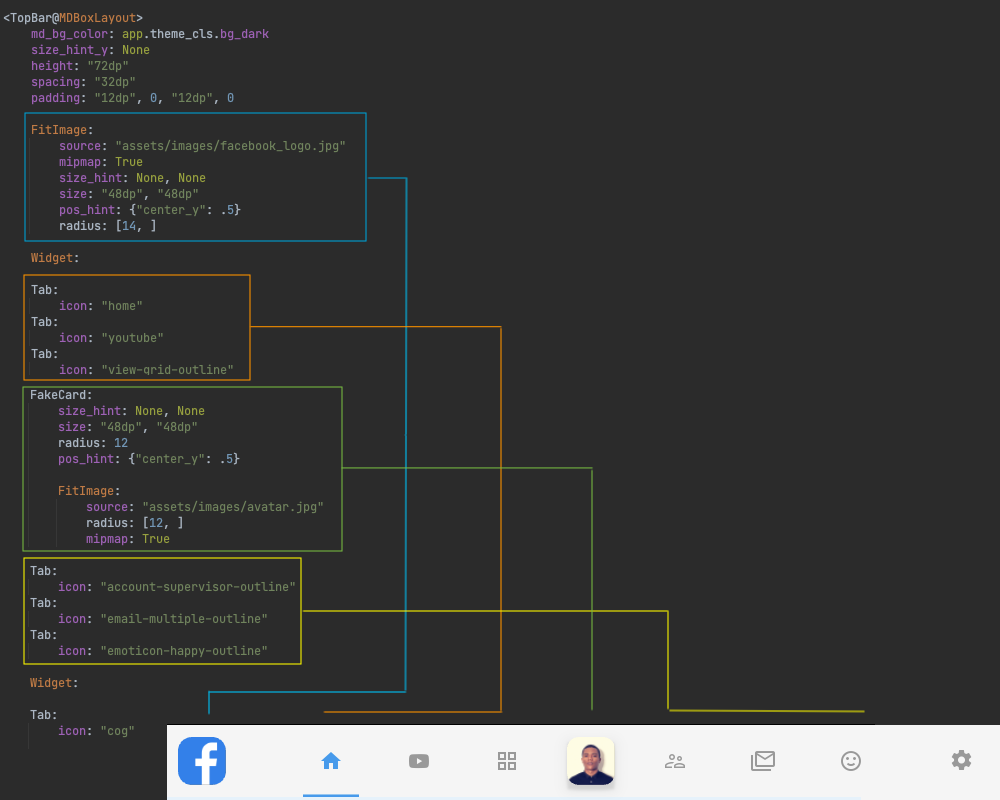
Logo, three tabs, an avatar, three tabs and one tab - the settings button. Implementation of a tab with an animated indicator:

Animation of the indicator and changing the type of the mouse cursor is implemented in a Python file in the class of the same name with the markup rule:
from kivy.animation import Animation
from kivy.properties import StringProperty, BooleanProperty
from kivy.core.window import Window
from kivymd.uix.boxlayout import MDBoxLayout
from kivymd.uix.behaviors import FocusBehavior
class Tab(FocusBehavior, MDBoxLayout):
icon = StringProperty()
active = BooleanProperty(False)
def on_enter(self):
Window.set_system_cursor("hand")
def on_leave(self):
Window.set_system_cursor("arrow")
def on_active(self, instance, value):
Animation(
opacity=value,
width=self.width if value else 0,
d=0.25,
t="in_sine" if value else "out_sine",
).start(self.ids.separator)
We will simply animate the width and opacity of the indicator depending on the state of the button (active). The state of the button is set in the main class of the application screen:
class FacebookDesktop(ThemableBehavior, MDScreen):
def set_active_tab(self, instance_tab):
for widget in self.ids.tab_box.children:
if issubclass(widget.__class__, MDBoxLayout):
if widget == instance_tab:
widget.active = True
else:
widget.active = False
Learn more about animation in Kivy:
Material Design. Creating animations in Kivy
Development of mobile applications in Python. Creating animations in Kivy. Part 2
Implementation in Dart / Flutter.
Since there is a lot of code, I hid everything under spoilers:
app_logo.dart
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
class AppLogo extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Container(
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(10),
boxShadow: [
BoxShadow(
color: Colors.blue.withOpacity(.6),
blurRadius: 5,
spreadRadius: 1,
),
],
),
child: ClipRRect(
borderRadius: BorderRadius.circular(10),
child: Image.asset(
'assets/images/facebook_logo.jpg',
width: 30,
height: 30,
),
),
);
}
}
avatar.dart
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
class TopBarAvatar extends StatefulWidget {
@override
_TopBarAvatarState createState() => _TopBarAvatarState();
}
class _TopBarAvatarState extends State<TopBarAvatar>
with SingleTickerProviderStateMixin {
Animation<Color> _animation;
AnimationController _animationController;
@override
void initState() {
_animationController = AnimationController(
vsync: this,
duration: Duration(milliseconds: 150),
);
_animation = ColorTween(
begin: Colors.grey.withOpacity(.4),
end: Colors.blue.withOpacity(.6),
).animate(_animationController);
_animation.addListener(() {
setState(() {});
});
super.initState();
}
@override
Widget build(BuildContext context) {
return MouseRegion(
onHover: (event) {
setState(() {
_animationController.forward();
});
},
onExit: (event) {
setState(() {
_animationController.reverse();
});
},
cursor: SystemMouseCursors.click,
child: Padding(
padding: const EdgeInsets.symmetric(horizontal: 15),
child: Container(
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(15),
boxShadow: [
BoxShadow(
color: _animation.value,
blurRadius: 10,
spreadRadius: 0,
),
],
),
child: ClipRRect(
borderRadius: BorderRadius.circular(15),
child: Image.asset(
'assets/images/avatar.jpg',
width: 50,
height: 50,
),
),
),
),
);
}
}
button.dart
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
class TopBarButton extends StatefulWidget {
final IconData icon;
final bool isActive;
final Function onTap;
const TopBarButton({
Key key,
this.icon,
this.isActive = false,
this.onTap,
}) : super(key: key);
@override
_TopBarButtonState createState() => _TopBarButtonState();
}
class _TopBarButtonState extends State<TopBarButton>
with SingleTickerProviderStateMixin {
Animation<Color> _animation;
AnimationController _animationController;
@override
void initState() {
_animationController = AnimationController(
vsync: this,
duration: Duration(milliseconds: 150),
);
_animation = ColorTween(
begin: Colors.grey.withOpacity(.6),
end: Colors.blue.withOpacity(.6),
).animate(_animationController);
_animation.addListener(() {
setState(() {});
});
super.initState();
}
@override
void didUpdateWidget(TopBarButton oldWidget) {
if (widget.isActive) {
_animationController.forward();
} else {
_animationController.reverse();
}
super.didUpdateWidget(oldWidget);
}
@override
Widget build(BuildContext context) {
return GestureDetector(
onTap: widget.onTap,
child: MouseRegion(
cursor: SystemMouseCursors.click,
child: Container(
height: 80,
child: Stack(
alignment: Alignment.center,
children: [
Padding(
padding: const EdgeInsets.symmetric(horizontal: 30),
child: Icon(
widget.icon,
color: _animation.value,
),
),
Positioned(
bottom: -1,
child: Align(
alignment: Alignment.bottomCenter,
child: AnimatedContainer(
duration: Duration(milliseconds: 50),
curve: Curves.easeInOut,
decoration: BoxDecoration(
color: _animation.value,
borderRadius: BorderRadius.circular(5),
boxShadow: [
BoxShadow(
color: _animation.value,
blurRadius: 5,
offset: Offset(0, 2),
),
],
),
width: widget.isActive ? 50 : 0,
height: 4,
),
),
),
],
),
),
),
);
}
@override
void dispose() {
_animationController.dispose();
super.dispose();
}
}
widget.dart
import 'package:facebook_desktop/screens/home/components/top_bar/app_logo.dart';
import 'package:facebook_desktop/screens/home/components/top_bar/avatar.dart';
import 'package:facebook_desktop/screens/home/components/top_bar/button.dart';
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_feather_icons/flutter_feather_icons.dart';
class TopBar extends StatefulWidget {
@override
_TopBarState createState() => _TopBarState();
}
class _TopBarState extends State<TopBar> {
int _selectedPage = 0;
@override
Widget build(BuildContext context) {
return Container(
color: Colors.white,
padding: const EdgeInsets.symmetric(
horizontal: 30,
),
child: Row(
children: [
Expanded(
flex: 1,
child: Align(
alignment: Alignment.centerLeft,
child: AppLogo(),
),
),
Expanded(
flex: 6,
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
TopBarButton(
icon: FeatherIcons.home,
isActive: _selectedPage == 0,
onTap: () {
setState(() {
_selectedPage = 0;
});
},
),
TopBarButton(
icon: FeatherIcons.youtube,
isActive: _selectedPage == 1,
onTap: () {
setState(() {
_selectedPage = 1;
});
},
),
TopBarButton(
icon: FeatherIcons.grid,
isActive: _selectedPage == 2,
onTap: () {
setState(() {
_selectedPage = 2;
});
},
),
TopBarAvatar(),
TopBarButton(
icon: FeatherIcons.users,
isActive: _selectedPage == 3,
onTap: () {
setState(() {
_selectedPage = 3;
});
},
),
TopBarButton(
icon: FeatherIcons.zap,
isActive: _selectedPage == 4,
onTap: () {
setState(() {
_selectedPage = 4;
});
},
),
TopBarButton(
icon: FeatherIcons.smile,
isActive: _selectedPage == 5,
onTap: () {
setState(() {
_selectedPage = 5;
});
},
),
],
),
),
Expanded(
flex: 1,
child: Align(
alignment: Alignment.centerRight,
child: IconButton(
color: Colors.grey.withOpacity(.6),
icon: Icon(FeatherIcons.settings),
onPressed: () {},
),
),
),
],
),
);
}
}
ChatCard (Kivy, Flutter)
![]() |
![]() |
The animation of the shift of the card occurs relative to the parent widget (parent) when receiving focus and unfocused events (on_enter, on_leave):
on_enter: Animation(x=root.parent.x + dp(12), d=0.4, t="out_cubic").start(root)
on_leave: Animation(x=root.parent.x + dp(24), d=0.4, t="out_cubic").start(root)
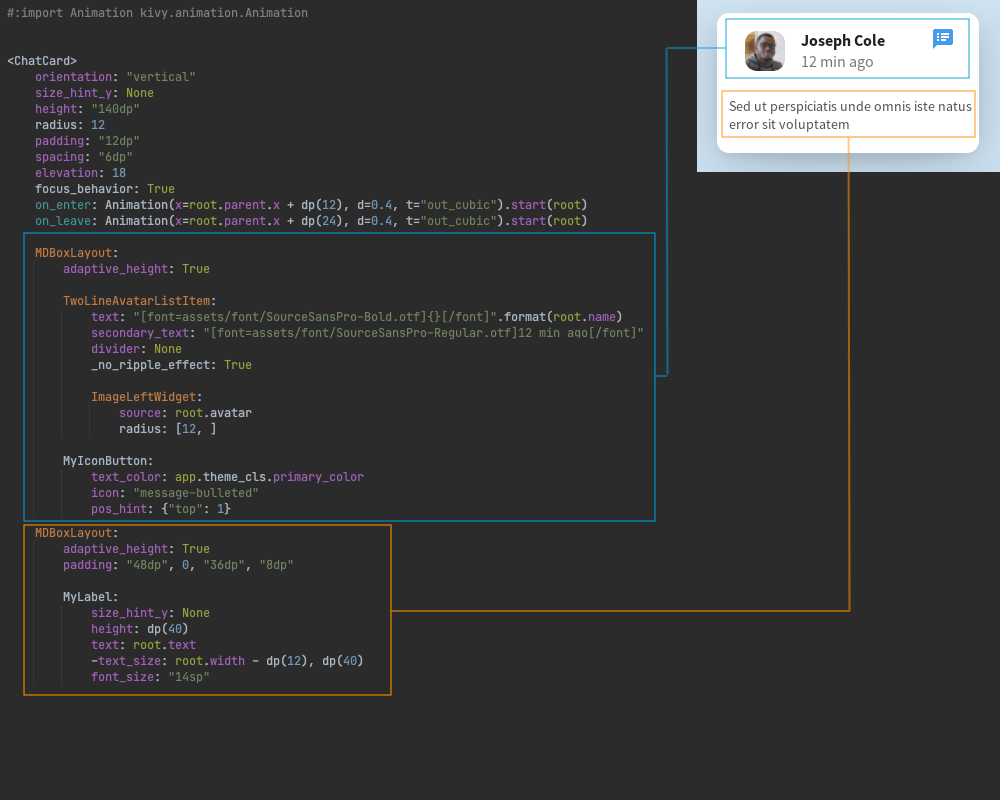
And the base Python class:
from kivy.core.window import Window
from kivy.properties import StringProperty
from FacebookDesktop.components.cards.fake_card import FakeCard
class ChatCard(FakeCard):
avatar = StringProperty()
text = StringProperty()
name = StringProperty()
def on_enter(self):
Window.set_system_cursor("hand")
def on_leave(self):
Window.set_system_cursor("arrow")
Python / Kivy implementation - 60 lines of code, Dart / Flutter implementation - 182 lines of code.
chat_card.dart
import 'package:ezanimation/ezanimation.dart';
import 'package:facebook_desktop/components/user_tile.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_feather_icons/flutter_feather_icons.dart';
class ChatCard extends StatefulWidget {
final String image;
final String name;
final String message;
final EdgeInsets padding;
const ChatCard({
Key key,
this.image,
this.name,
this.message,
this.padding,
}) : super(key: key);
@override
_ChatCardState createState() => _ChatCardState();
}
class _ChatCardState extends State<ChatCard> {
EzAnimation _animation;
@override
void initState() {
_animation = EzAnimation(
0.0,
-5.0,
Duration(milliseconds: 200),
curve: Curves.easeInOut,
context: context,
);
_animation.addListener(() {
setState(() {});
});
super.initState();
}
@override
Widget build(BuildContext context) {
return Transform.translate(
offset: Offset(_animation.value, 0),
child: MouseRegion(
cursor: SystemMouseCursors.click,
onEnter: (event) {
_animation.start();
},
onExit: (event) {
_animation.reverse();
},
child: Padding(
padding: widget.padding ?? const EdgeInsets.all(15),
child: Container(
width: 250,
padding: const EdgeInsets.all(15),
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(10),
boxShadow: [
BoxShadow(
color: Colors.black.withOpacity(.1),
blurRadius: 15,
offset: Offset(0, 8),
),
],
),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
UserTile(
name: widget.name,
image: widget.image,
trailing: Icon(
FeatherIcons.messageSquare,
color: Colors.blue,
size: 14,
),
),
SizedBox(
height: 10,
),
Text(
widget.message,
style: TextStyle(color: Colors.grey, fontSize: 12),
maxLines: 3,
overflow: TextOverflow.ellipsis,
),
],
),
),
),
),
);
}
@override
void dispose() {
_animation.dispose();
super.dispose();
}
}
user_tile.dart
import 'package:facebook_desktop/screens/home/components/section.dart';
import 'package:flutter/material.dart';
class UserTile extends StatelessWidget {
final String name;
final String image;
final Widget trailing;
const UserTile({
Key key,
this.name,
this.image,
this.trailing,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return Row(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Container(
margin: const EdgeInsets.only(right: 10),
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(10),
boxShadow: [
BoxShadow(
color: Colors.black.withOpacity(.1),
blurRadius: 5,
offset: Offset(0, 2),
),
],
),
child: ClipRRect(
borderRadius: BorderRadius.circular(5),
child: Image(
image: NetworkImage(
image,
),
fit: BoxFit.cover,
height: 50,
width: 50,
),
),
),
Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
SectionTitle(
title: name,
),
SizedBox(
height: 5,
),
Text(
'12 min ago',
style: TextStyle(color: Colors.grey),
),
],
),
if (trailing != null)
Expanded(
child: Align(
alignment: Alignment.topRight,
child: trailing
),
),
],
);
}
}
But not everything is as simple as it seems. In the process, I discovered that the KivyMD library was missing buttons with the "badge" type. By the way, the Flutter project also used custom buttons. Therefore, to create the right toolbar, I had to make such buttons myself.
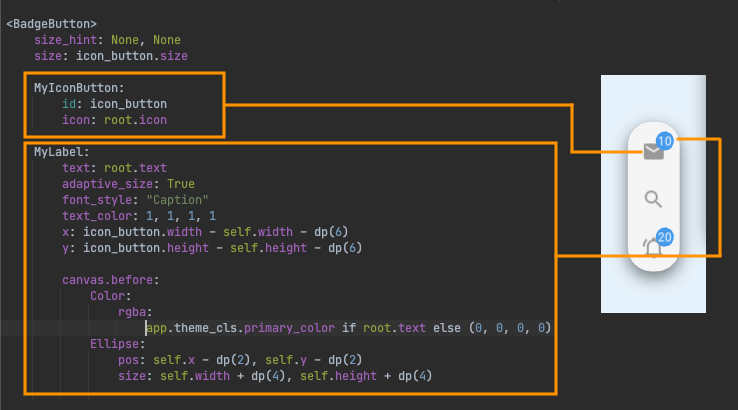
Base Python class:
from kivy.properties import StringProperty
from kivymd.uix.relativelayout import MDRelativeLayout
class BadgeButton(MDRelativeLayout):
icon = StringProperty()
text = StringProperty()
And already create the left toolbar:

Even considering that I had to create custom buttons like "badge", the code of the left toolbar in Python / Kivy turned out to be shorter - 58 lines of code, implementation in Dart / Flutter - 97 lines.
button.dart
import 'package:flutter/material.dart';
class LeftBarButton extends StatelessWidget {
final IconData icon;
final String badge;
const LeftBarButton({
Key key,
this.icon,
this.badge,
}) : super(key: key);
@override
Widget build(BuildContext context) {
return GestureDetector(
child: Stack(
children: [
Container(
padding: const EdgeInsets.all(10),
child: Icon(
icon,
color: Colors.grey.withOpacity(.6),
),
),
if (badge != null)
Positioned(
top: 5,
right: 2,
child: Container(
padding: const EdgeInsets.all(3),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(100),
color: Colors.blue,
),
child: Text(
badge,
style: TextStyle(
color: Colors.white,
fontSize: 10,
),
),
),
)
],
),
);
}
}
widget.dart
import 'package:facebook_desktop/screens/home/left_bar/button.dart';
import 'package:flutter/material.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter_feather_icons/flutter_feather_icons.dart';
class LeftBar extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Container(
margin: const EdgeInsets.all(30),
padding: const EdgeInsets.all(5),
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(50),
boxShadow: [
BoxShadow(
color: Colors.grey.withOpacity(.1),
blurRadius: 2,
offset: Offset(0, 4),
)
],
),
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
LeftBarButton(
icon: FeatherIcons.mail,
badge: '10',
),
SizedBox(
height: 5,
),
LeftBarButton(
icon: FeatherIcons.search,
),
SizedBox(
height: 5,
),
LeftBarButton(
icon: FeatherIcons.bell,
badge: '20',
),
],
),
);
}
}
Of course, I am not belittling the merits of the Flutter framework. The tool is wonderful! I just wanted to show Python developers that they can do the same things as in Flutter, but in their favorite programming language using the Kivy framework and the KivyMD library. As for mobile platforms, here it is worth recognizing that Flutter surpasses Kivy in terms of speed. But that's already another article ... Link to the repository of the Facebook Desktop Redesign built with Flutter Desktop project in the Python / Kivy / KivyMD implementation.