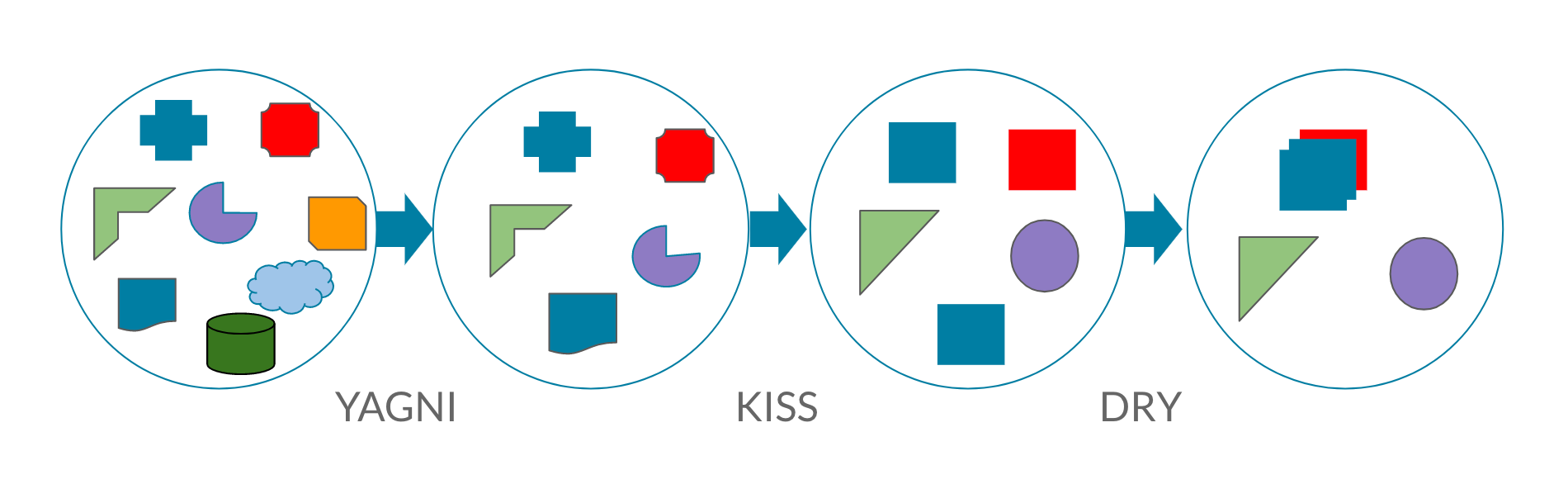
A good programmer needs to be able to combine his skills with common sense. It's all about pragmatism and the skill of choosing the best solution for your problem. When you are faced with a problem in software development, you can use basic principles to help you choose the right approach.
This text provides a set of principles that any developer should know and which should be periodically refreshed. Consider them your secret programming weapon.
Applying these principles consistently will make your transition from middle to senior easier. You may find that some (probably) you use intuitively.
There are many principles. We will focus on the seven most important ones. Using them will help you grow and become a better programmer.
1. YAGNI
You Aren't Gonna Need ItThis principle is simple and obvious, but not everyone follows it. If you are writing code, be sure that you will need it. Don't write code if you think it will come in handy later.
This principle applies to refactoring. If you're refactoring a method, class, or file, don't be afraid to remove unnecessary methods. Even if they were useful before, now they are not needed.
The day may come when they are needed again - then you can use the git repository to resurrect them from the dead.
2. DRY
Don't Repeat YourselfThis concept was first articulated in Andy Hunt and Dave Thomas's book The Pragmatic Programmer: The Journey from Apprentice to Master.
The idea revolves around a single source of truth (SSOT). What is this anyway?
In information systems design and theory, the Single Source of Truth (SSOT) is the practice of structuring information models and data schemas, which means that all pieces of data are processed (or edited) in only one place ... SSOTs provide data that is valid, relevant, and usable.
- Wikipedia
Using SSOT will create a more robust and comprehensible codebase.
Duplicating code is a waste of time and resources. You will have to maintain the same logic and test the code in two places at once, and if you change the code in one place, you will need to change it in another.
In most cases, code duplication occurs due to ignorance of the system. Before you write anything, be pragmatic: take a look around. Perhaps this function is implemented somewhere. Perhaps this business logic exists elsewhere. Code reuse is always a smart decision.
3. KISS
Keep It Simple, StupidThis principle was developed by the US Navy in 1960. This principle states that simple systems will perform better and more reliably.
This principle has much in common with the reinvention of the wheel, which was done in the 1970s. Then it sounded like a business and advertising metaphor.
With regard to software development, it means the following - do not come up with a more complex solution to the problem than it needs.
Sometimes the smartest decision turns out to be the simplest. Writing efficient, efficient, and simple code is great.
One of the most common mistakes of our time is using new tools solely because they shine. Developers should be motivated to use the latest technology, not because it is new, but because it is right for the job.
4. Big Design Up Front
Global Design FirstThis approach to software development is very important and often overlooked. Make sure everything is well thought out before moving on to implementation.
… . , . , . , BDUF, , . , .
—
Many developers feel that if they don't write code, then they are not making progress. This is the wrong approach. By making a plan, you save yourself the trouble of starting from scratch over and over again.
Sometimes other people have to be involved in the flaws and processes of the architecture development. The sooner you discuss all this, the better it will be for everyone.
A very common counter-argument is that the cost of solving problems is often less than the cost of planning time. The fewer errors the user encounters, the better the experience will be. You may not have another chance to deal with these errors.
5. SOLID
This is the most well-known principle of software development. Solid is an abbreviation for:
S) Single-responsibility principle
Its importance cannot be overemphasized. Each object, class and method should be responsible for only one thing. If your object / class / method does too much, you end up with spaghetti code. Here's an example:
const saveTodo = async () => {
try {
response = await saveTodoApi();
showSuccessPop('Success');
window.location.href = '/successPage';
} catch (error) {
showErrorPopup(`Error: ${error} `);
}
}
This method seems harmless, but it actually does too much:
- Saves the object
- Handles notification in UI
- Performs navigation
Another side effect of this code is testing issues. Complicated functionality is difficult to test.
O) Open – closed principle
Program objects should be open for extension, but closed for modification. The point is that you cannot override methods or classes, simply adding additional functions as needed.
A good way to deal with this problem is by using inheritance. JavaScript solves this problem with composition.
A simple rule of thumb: if you change an entity to make it extensible, you are breaking this principle for the first time.
L) Liskov substitution principle
This principle states that objects of higher classes should be replaceable with objects of subclasses, and the application should perform as expected when such replacements are made.
I) Interface segregation principle
This principle was formulated by Robert Martin when he consulted Xerox, and it is obvious.
Objects should not depend on interfaces they don't use
The software should be divided into independent parts. Side effects must be minimized to ensure independence.
Make sure you don't force objects to implement methods they never need. Here's an example:
interface Animal {
eat: () => void;
walk: () => void;
fly: () => void;
swim: () => void;
}
Not all animals can fly, walk or swim, so these methods should not be part of the interface or should be optional.
D) Dependency inversion principle
This principle cannot be overemphasized. We must rely on abstractions, not concrete implementations. Software components should have low cohesion and high consistency.
You need to worry not about how something works, but about how it works. A simple example is using dates in JavaScript. You can write your own abstraction layer for them. Then if you change the source of obtaining dates, you will need to make changes in one place, and not in a thousand.
Sometimes it takes effort to add this layer of abstraction, but it pays off in the end.
Take a look at date-io as an example , this library has a layer of abstraction that allows you to use it with different date sources.
6. Avoid Premature Optimization
Avoid Premature OptimizationThis practice encourages developers to optimize their code before the optimization is proven necessary. I think that if you follow KISS or YAGNI, you will not fall into this hook.
Get it right, it is good to anticipate that something bad will happen. But before you dive into the implementation details, make sure these optimizations are really useful.
A very simple example is scaling. You will not buy 40 servers on the assumption that your new application will become very popular. You will add servers as needed.
Premature optimization can lead to delays in the code and, therefore, increase the time required to bring functions to market.
Many consider premature optimization to be the root of all evil.
7. Occam's Razor
Brithva Okkama (sometimes Lezvie Okkam) is a methodological principle that briefly states: “You should not multiply things unnecessarily” [1] (or “You should not attract new entities unless absolutely necessary”).
- Wikipedia
What does this mean in the programming world? Don't create unnecessary entities unnecessarily. Be pragmatic - consider if they are needed as they may end up complicating your codebase.
Conclusion
These principles are not very difficult. In fact, it's the simplicity that makes them beautiful. If you are confused, do not try to apply all of them at once. Just try to work with awareness and try to gradually incorporate these principles into your workflow.
Using basic yet powerful principles will allow you to become a better programmer and have a clearer understanding of why you are doing something.
If you are applying most of the principles intuitively, it is worthwhile to think and understand why you are doing something in a certain way.
All the best.
- Russia's first serial control system for a dual-fuel engine with functional separation of controllers
- There are more lines of code in a modern car than ...
- - Automotive, Aerospace, (50+)
- McKinsey: automotive
