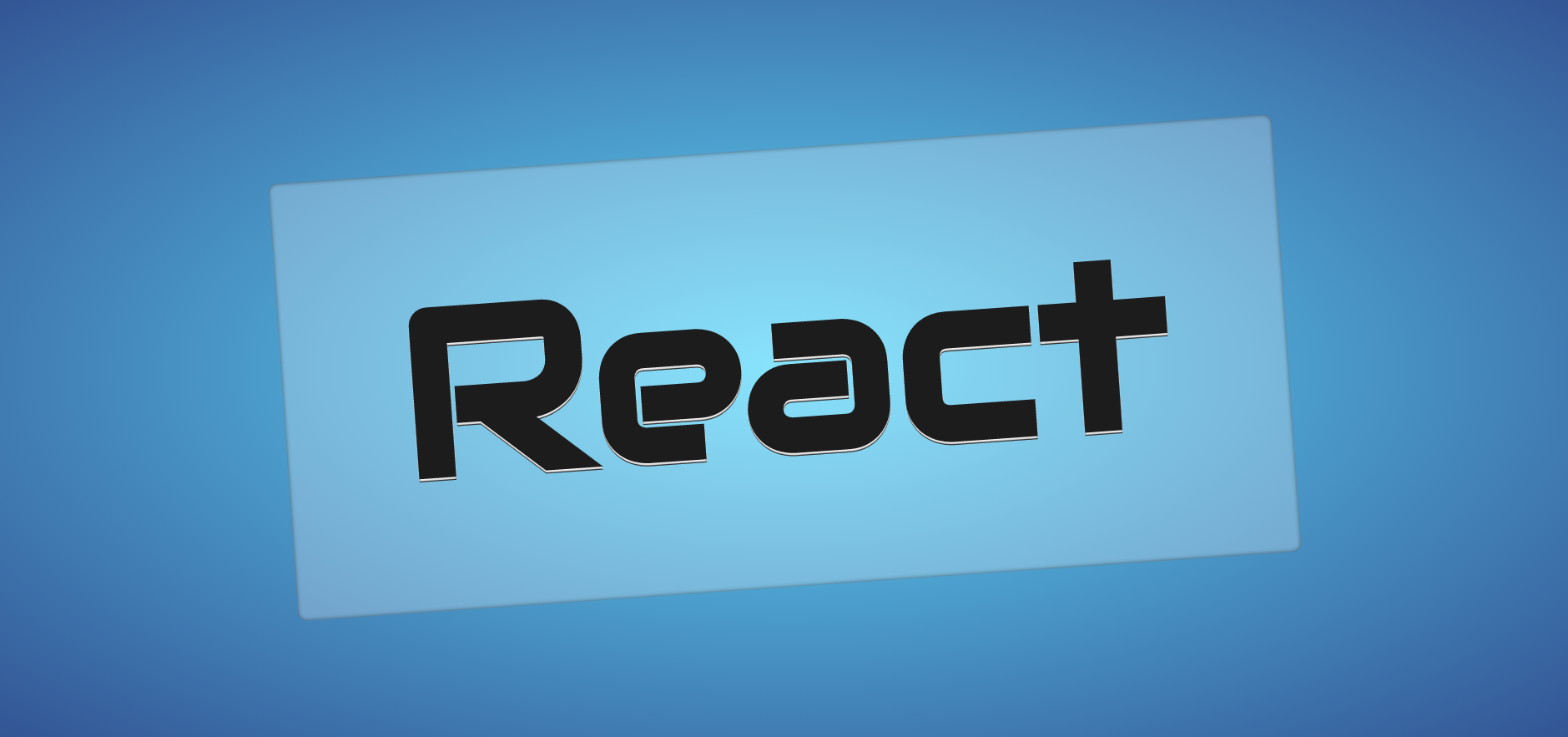
Good day, friends!
I would like to share with you my experience of developing a simple chat in React using the Socket.IO library .
It is assumed that you are familiar with the named library. If you are not familiar, here is a related guide with examples of creating a tudushka and chat in vanilla JavaScript .
It also assumes that you are at least superficially familiar with Node.js .
In this article, I'll focus on the practicalities of using Socket.IO, React, and Node.js.
Our chat will have the following main features:
- Room selection
- Sending messages
- Delete messages by the sender
- Storing messages in a local database in JSON format
- Storing the username and ID in the browser's local storage
- Displaying the number of active users
- Displaying a list of users with an online indicator
We will also implement the ability to send emoji .
If you are interested, please follow me.
For those who are only interested in the code: here is the link to the repository .
Sandbox:
Project structure and dependencies
Let's start creating a project:
mkdir react-chat
cd react-chat
Create a client using Create React App :
yarn create react-app client
#
npm init react-app client
#
npx create-react-app client
In the future, I will use yarn : to install dependencies
yarn add = npm i, yarn start = npm start, yarn dev = npm run dev
.
Go to the "client" directory and install additional dependencies:
cd client
yarn add socket.io-client react-router-dom styled-components bootstrap react-bootstrap react-icons emoji-mart react-timeago
- socket.io-client - Socket.IO client side
- react-router-dom - routing
- styled-components - styling (CSS-in-JS)
- bootstrap , react-bootstrap - styling
- react-icons - icons
- emoji-mart - emoji
- react-timeago - date and time formatting
The "dependencies" section of the "package.json" file:
{
"bootstrap": "^4.6.0",
"emoji-mart": "^3.0.0",
"react": "^17.0.1",
"react-bootstrap": "^1.5.0",
"react-dom": "^17.0.1",
"react-icons": "^4.2.0",
"react-router-dom": "^5.2.0",
"react-scripts": "4.0.1",
"react-timeago": "^5.2.0",
"socket.io-client": "^3.1.0",
"styled-components": "^5.2.1"
}
Go back to the root directory (react-chat), create the "server" directory, go to it, initialize the project and install the dependencies:
cd ..
mkdir server
cd server
yarn init -yp
yarn add socket.io lowdb supervisor
- socket.io - Socket.IO backend
- lowdb - local database in JSON format
- supervisor - development server (alternative to nodemon , which does not work correctly with the latest stable version of Node.js; it has something to do with incorrect starting / stopping of child processes)
Add the "start" command to start the production server and the "dev" command to start the development server. package.json:
{
"name": "server",
"version": "1.0.0",
"main": "index.js",
"license": "MIT",
"private": true,
"dependencies": {
"lowdb": "^1.0.0",
"socket.io": "^3.1.0",
"supervisor": "^0.12.0"
},
"scripts": {
"start": "node index.js",
"dev": "supervisor index.js"
}
}
Go back to the root directory (react-chat), initialize the project and install the dependencies:
cd .. yarn init -yp yarn add nanoid concurrently
- nanoid - generating identifiers (will be used both on the client and on the server)
- concurrently - simultaneous execution of two or more commands
react-chat / package.json (note the commands for npm look different; see the concurrently docs):
{
"name": "react-chat",
"version": "1.0.0",
"main": "index.js",
"license": "MIT",
"private": true,
"dependencies": {
"concurrently": "^6.0.0",
"nanoid": "^3.1.20"
},
"scripts": {
"server": "yarn --cwd server dev",
"client": "yarn --cwd client start",
"start": "concurrently \"yarn server\" \"yarn client\""
}
}
Great, we are done with the formation of the main structure of the project and the installation of the necessary dependencies. Let's start implementing the server.
Server implementation
The structure of the "server" directory:
|--server |--db - |--handlers |--messageHandlers.js |--userHandlers.js |--index.js ...
In the "index.js" file, we do the following:
- Building an HTTP server
- We connect Socket.IO to it
- We start the server on port 5000
- Registering Event Handlers When Connecting a Socket
index.js:
// HTTP-
const server = require('http').createServer()
// Socket.IO
const io = require('socket.io')(server, {
cors: {
origin: '*'
}
})
const log = console.log
//
const registerMessageHandlers = require('./handlers/messageHandlers')
const registerUserHandlers = require('./handlers/userHandlers')
// (, = )
const onConnection = (socket) => {
//
log('User connected')
// ""
const { roomId } = socket.handshake.query
//
socket.roomId = roomId
// ( )
socket.join(roomId)
//
//
registerMessageHandlers(io, socket)
registerUserHandlers(io, socket)
// -
socket.on('disconnect', () => {
//
log('User disconnected')
//
socket.leave(roomId)
})
}
//
io.on('connection', onConnection)
//
const PORT = process.env.PORT || 5000
server.listen(PORT, () => {
console.log(`Server ready. Port: ${PORT}`)
})
In the file "handlers / messageHandlers.js" we do the following:
- Setting up a local database in JSON format using lowdb
- We write initial data to the database
- Creating functions for receiving, adding and deleting messages
- We register the processing of the corresponding events:
- message: get - receive messages
- message: add - add a message
- message: remove - delete a message
Messages are objects with the following properties:
- messageId (string) - message identifier
- userId (string) - user ID
- senderName (string) - sender name
- messageText (string) - message text
- createdAt (date) - creation date
handlers / messageHandlers.js:
const { nanoid } = require('nanoid')
//
const low = require('lowdb')
const FileSync = require('lowdb/adapters/FileSync')
// "db" "messages.json"
const adapter = new FileSync('db/messages.json')
const db = low(adapter)
//
db.defaults({
messages: [
{
messageId: '1',
userId: '1',
senderName: 'Bob',
messageText: 'What are you doing here?',
createdAt: '2021-01-14'
},
{
messageId: '2',
userId: '2',
senderName: 'Alice',
messageText: 'Go back to work!',
createdAt: '2021-02-15'
}
]
}).write()
module.exports = (io, socket) => {
//
const getMessages = () => {
//
const messages = db.get('messages').value()
// ,
// - , ,
io.in(socket.roomId).emit('messages', messages)
}
//
//
const addMessage = (message) => {
db.get('messages')
.push({
// nanoid, 8 - id
messageId: nanoid(8),
createdAt: new Date(),
...message
})
.write()
//
getMessages()
}
//
// id
const removeMessage = (messageId) => {
db.get('messages').remove({ messageId }).write()
getMessages()
}
//
socket.on('message:get', getMessages)
socket.on('message:add', addMessage)
socket.on('message:remove', removeMessage)
}
In the file "handlers / userHandlers.js" we do the following:
- Create a normalized structure with users
- We create functions for getting, adding and removing users
- We register the processing of the corresponding events:
- user: get - get users
- user: add - add a user
- user: leave - delete a user
We could also use lowdb to work with the list of users. You can do this if you like. I, with your permission, will confine myself to the object.
The normalized structure (object) of users has the following format:
{ id (string) - : { username (string) - , online (boolean) - } }
In fact, we are not deleting users, but transferring their status to offline (assigning the "online" property to "false").
handlers / userHandlers.js:
//
//
const users = {
1: { username: 'Alice', online: false },
2: { username: 'Bob', online: false }
}
module.exports = (io, socket) => {
//
// "roomId" ,
// ,
//
const getUsers = () => {
io.in(socket.roomId).emit('users', users)
}
//
// id
const addUser = ({ username, userId }) => {
// ,
if (!users[userId]) {
// ,
users[userId] = { username, online: true }
} else {
// ,
users[userId].online = true
}
//
getUsers()
}
//
const removeUser = (userId) => {
// ,
// (O(1))
// ()
// redux, , immer,
users[userId].online = false
getUsers()
}
//
socket.on('user:get', getUsers)
socket.on('user:add', addUser)
socket.on('user:leave', removeUser)
}
We start the server to check its performance:
yarn dev
If we see the message “Server ready. Port: 5000 ", and the" messages.json "file with initial data appeared in the" db "directory, which means that the server is working as expected, and you can proceed to the implementation of the client part.
Client implementation
With the client, everything is somewhat more complicated. The structure of the "client" directory:
|--client |--public |--index.html |--src |--components |--ChatRoom |--MessageForm |--MessageForm.js |--package.json |--MessageList |--MessageList.js |--MessageListItem.js |--package.json |--UserList |--UserList.js |--package.json |--ChatRoom.js |--package.json |--Home |--Home.js |--package.json |--index.js |--hooks |--useBeforeUnload.js |--useChat.js |--useLocalStorage.js App.js index.js |--jsconfig.json ( src) ...
As the name implies, the "components" directory contains application components (parts of the user interface, modules), and the "hooks" directory contains user ("custom") hooks, the main of which is useChat ().
Files "package.json" in the component directories have a single field "main" with the value of the path to the JS file, for example:
{
"main": "./Home"
}
This allows you to import a component from a directory without specifying a file name, for example:
import { Home } from './Home'
//
import { Home } from './Home/Home'
The "components / index.js" and "hooks / index.js" files are used to aggregate and re-export components and hooks, respectively.
components / index.js:
export { Home } from './Home'
export { ChatRoom } from './ChatRoom'
hooks / index.js:
export { useChat } from './useChat'
export { useLocalStorage } from './useLocalStorage'
export { useBeforeUnload } from './useBeforeUnload'
This again allows you to import components and hooks by directory and at the same time. Aggregation and re-export causes the use of named component exports (React documentation recommends using the default export).
The jsconfig.json file looks like this:
{
"compilerOptions": {
"baseUrl": "src"
}
}
This "tells" the compiler that the import of modules starts from the "src" directory, so components, for example, can be imported like this:
//
import { Home, ChatRoom } from 'components'
//
import { Home, ChatRoom } from './components'
Let's start by looking at custom hooks.
You can use ready-made solutions. For example, here are the hooks offered by the react-use library :
#
yarn add react-use
#
import { useLocalStorage } from 'react-use'
import { useBeforeUnload } from 'react-use'
The useLocalStorage () hook allows you to store (write and retrieve) values in the browser's local storage. We will use it to store the username and user ID between browser sessions. We do not want to force the user to enter their name every time, but the ID is needed to determine the messages belonging to this user. The hook takes a name for the key and, optionally, an initial value.
hooks / useLocalstorage.js:
import { useState, useEffect } from 'react'
export const useLocalStorage = (key, initialValue) => {
const [value, setValue] = useState(() => {
const item = window.localStorage.getItem(key)
return item ? JSON.parse(item) : initialValue
})
useEffect(() => {
const item = JSON.stringify(value)
window.localStorage.setItem(key, item)
// , key, useEffect, ,
// useEffect
// eslint-disable-next-line
}, [value])
return [value, setValue]
}
The "useBeforeUnload ()" hook is used to display a message or execute a function when the page (browser tab) is reloaded or closed. We will use it to send a "user: leave" event to the server to toggle the user's status. An attempt to implement dispatch of the specified event using the callback returned by the "useEffect ()" hook was unsuccessful. The hook takes one parameter, a primitive or a function.
hooks / useBeforeUnload.js:
import { useEffect } from 'react'
export const useBeforeUnload = (value) => {
const handleBeforeunload = (e) => {
let returnValue
if (typeof value === 'function') {
returnValue = value(e)
} else {
returnValue = value
}
if (returnValue) {
e.preventDefault()
e.returnValue = returnValue
}
return returnValue
}
useEffect(() => {
window.addEventListener('beforeunload', handleBeforeunload)
return () => window.removeEventListener('beforeunload', handleBeforeunload)
// eslint-disable-next-line
}, [])
}
The useChat () hook is the main hook for our application. It will be easier if I comment it line by line.
hooks / useChat.js:
import { useEffect, useRef, useState } from 'react'
// IO
import io from 'socket.io-client'
import { nanoid } from 'nanoid'
//
import { useLocalStorage, useBeforeUnload } from 'hooks'
//
// -
const SERVER_URL = 'http://localhost:5000'
//
export const useChat = (roomId) => {
//
const [users, setUsers] = useState([])
//
const [messages, setMessages] = useState([])
//
const [userId] = useLocalStorage('userId', nanoid(8))
//
const [username] = useLocalStorage('username')
// useRef() DOM-,
//
const socketRef = useRef(null)
useEffect(() => {
// ,
// ""
// socket.handshake.query.roomId
socketRef.current = io(SERVER_URL, {
query: { roomId }
})
// ,
// id
socketRef.current.emit('user:add', { username, userId })
//
socketRef.current.on('users', (users) => {
//
setUsers(users)
})
//
socketRef.current.emit('message:get')
//
socketRef.current.on('messages', (messages) => {
// , ,
// "userId" id ,
// "currentUser" "true",
// ,
const newMessages = messages.map((msg) =>
msg.userId === userId ? { ...msg, currentUser: true } : msg
)
//
setMessages(newMessages)
})
return () => {
//
socketRef.current.disconnect()
}
}, [roomId, userId, username])
//
//
const sendMessage = ({ messageText, senderName }) => {
// id
socketRef.current.emit('message:add', {
userId,
messageText,
senderName
})
}
// id
const removeMessage = (id) => {
socketRef.current.emit('message:remove', id)
}
// "user:leave"
useBeforeUnload(() => {
socketRef.current.emit('user:leave', userId)
})
// ,
return { users, messages, sendMessage, removeMessage }
}
By default, all client requests are sent to localhost: 3000 (the port on which the development server is running). To redirect requests to the port on which the "server" server is running, proxying must be performed. To do this, add the following line to the "src / package.json" file:
"proxy": "http://localhost:5000"
It remains to implement the application components.
The Home component is the first thing the user sees when they launch the application. It has a form in which the user is asked to enter his name and select a room. In reality, in the case of a room, the user has no choice, only one option (free) is available. The second (disabled) option (job) is the ability to scale the application. The display of the button to start a chat depends on the field with the user's name (when this field is empty, the button is not displayed). The button is actually a link to the chat page.
components / Home.js:
import { useState, useRef } from 'react'
// react-router-dom
import { Link } from 'react-router-dom'
//
import { useLocalStorage } from 'hooks'
// react-bootstrap
import { Form, Button } from 'react-bootstrap'
export function Home() {
//
//
const [username, setUsername] = useLocalStorage('username', 'John')
//
const [roomId, setRoomId] = useState('free')
const linkRef = useRef(null)
//
const handleChangeName = (e) => {
setUsername(e.target.value)
}
//
const handleChangeRoom = (e) => {
setRoomId(e.target.value)
}
//
const handleSubmit = (e) => {
e.preventDefault()
//
linkRef.current.click()
}
const trimmed = username.trim()
return (
<Form
className='mt-5'
style={{ maxWidth: '320px', margin: '0 auto' }}
onSubmit={handleSubmit}
>
<Form.Group>
<Form.Label>Name:</Form.Label>
<Form.Control value={username} onChange={handleChangeName} />
</Form.Group>
<Form.Group>
<Form.Label>Room:</Form.Label>
<Form.Control as='select' value={roomId} onChange={handleChangeRoom}>
<option value='free'>Free</option>
<option value='job' disabled>
Job
</option>
</Form.Control>
</Form.Group>
{trimmed && (
<Button variant='success' as={Link} to={`/${roomId}`} ref={linkRef}>
Chat
</Button>
)}
</Form>
)
}
The UserList component, as the name suggests, is a list of users. It has an accordion, the list itself, and indicators of users' online presence.
components / UserList.js:
//
import { Accordion, Card, Button, Badge } from 'react-bootstrap'
// -
import { RiRadioButtonLine } from 'react-icons/ri'
// -
export const UserList = ({ users }) => {
//
const usersArr = Object.entries(users)
// ( )
// [ ['1', { username: 'Alice', online: false }], ['2', {username: 'Bob', online: false}] ]
//
const activeUsers = Object.values(users)
//
// [ {username: 'Alice', online: false}, {username: 'Bob', online: false} ]
.filter((u) => u.online).length
return (
<Accordion className='mt-4'>
<Card>
<Card.Header bg='none'>
<Accordion.Toggle
as={Button}
variant='info'
eventKey='0'
style={{ textDecoration: 'none' }}
>
Active users{' '}
<Badge variant='light' className='ml-1'>
{activeUsers}
</Badge>
</Accordion.Toggle>
</Card.Header>
{usersArr.map(([userId, obj]) => (
<Accordion.Collapse eventKey='0' key={userId}>
<Card.Body>
<RiRadioButtonLine
className={`mb-1 ${
obj.online ? 'text-success' : 'text-secondary'
}`}
size='0.8em'
/>{' '}
{obj.username}
</Card.Body>
</Accordion.Collapse>
))}
</Card>
</Accordion>
)
}
The MessageForm component is a standard form for sending messages. Picker is an emoji component provided by the emoji-mart library. This component is shown / hidden by pressing a button.
components / MessageForm.js:
import { useState } from 'react'
//
import { Form, Button } from 'react-bootstrap'
//
import { Picker } from 'emoji-mart'
//
import { FiSend } from 'react-icons/fi'
import { GrEmoji } from 'react-icons/gr'
//
export const MessageForm = ({ username, sendMessage }) => {
//
const [text, setText] = useState('')
//
const [showEmoji, setShowEmoji] = useState(false)
//
const handleChangeText = (e) => {
setText(e.target.value)
}
// /
const handleEmojiShow = () => {
setShowEmoji((v) => !v)
}
//
// ,
const handleEmojiSelect = (e) => {
setText((text) => (text += e.native))
}
//
const handleSendMessage = (e) => {
e.preventDefault()
const trimmed = text.trim()
if (trimmed) {
sendMessage({ messageText: text, senderName: username })
setText('')
}
}
return (
<>
<Form onSubmit={handleSendMessage}>
<Form.Group className='d-flex'>
<Button variant='primary' type='button' onClick={handleEmojiShow}>
<GrEmoji />
</Button>
<Form.Control
value={text}
onChange={handleChangeText}
type='text'
placeholder='Message...'
/>
<Button variant='success' type='submit'>
<FiSend />
</Button>
</Form.Group>
</Form>
{/* */}
{showEmoji && <Picker onSelect={handleEmojiSelect} emojiSize={20} />}
</>
)
}
The MessageListItem component is a message list item. TimeAgo is a component for formatting date and time. It takes a date and returns a string like "1 month ago". This line is updated in real time. Only the user who sent them can delete messages.
components / MessageListItem.js:
//
import TimeAgo from 'react-timeago'
//
import { ListGroup, Card, Button } from 'react-bootstrap'
//
import { AiOutlineDelete } from 'react-icons/ai'
//
export const MessageListItem = ({ msg, removeMessage }) => {
//
const handleRemoveMessage = (id) => {
removeMessage(id)
}
const { messageId, messageText, senderName, createdAt, currentUser } = msg
return (
<ListGroup.Item
className={`d-flex ${currentUser ? 'justify-content-end' : ''}`}
>
<Card
bg={`${currentUser ? 'primary' : 'secondary'}`}
text='light'
style={{ width: '55%' }}
>
<Card.Header className='d-flex justify-content-between align-items-center'>
{/* TimeAgo */}
<Card.Text as={TimeAgo} date={createdAt} className='small' />
<Card.Text>{senderName}</Card.Text>
</Card.Header>
<Card.Body className='d-flex justify-content-between align-items-center'>
<Card.Text>{messageText}</Card.Text>
{/* */}
{currentUser && (
<Button
variant='none'
className='text-warning'
onClick={() => handleRemoveMessage(messageId)}
>
<AiOutlineDelete />
</Button>
)}
</Card.Body>
</Card>
</ListGroup.Item>
)
}
The MessageList component is a list of messages. It uses the "MessageListItem" component.
components / MessageList.js:
import { useRef, useEffect } from 'react'
//
import { ListGroup } from 'react-bootstrap'
//
import { MessageListItem } from './MessageListItem'
// (inline styles)
const listStyles = {
height: '80vh',
border: '1px solid rgba(0,0,0,.4)',
borderRadius: '4px',
overflow: 'auto'
}
//
// "MessageListItem"
export const MessageList = ({ messages, removeMessage }) => {
// ""
const messagesEndRef = useRef(null)
// ,
useEffect(() => {
messagesEndRef.current?.scrollIntoView({
behavior: 'smooth'
})
}, [messages])
return (
<>
<ListGroup variant='flush' style={listStyles}>
{messages.map((msg) => (
<MessageListItem
key={msg.messageId}
msg={msg}
removeMessage={removeMessage}
/>
))}
<span ref={messagesEndRef}></span>
</ListGroup>
</>
)
}
The App component is the main component of the application. It defines routes and assembles the interface.
src / App.js:
//
import { BrowserRouter as Router, Switch, Route } from 'react-router-dom'
//
import { Container } from 'react-bootstrap'
//
import { Home, ChatRoom } from 'components'
//
const routes = [
{ path: '/', name: 'Home', Component: Home },
{ path: '/:roomId', name: 'ChatRoom', Component: ChatRoom }
]
export const App = () => (
<Router>
<Container style={{ maxWidth: '512px' }}>
<h1 className='mt-2 text-center'>React Chat App</h1>
<Switch>
{routes.map(({ path, Component }) => (
<Route key={path} path={path} exact>
<Component />
</Route>
))}
</Switch>
</Container>
</Router>
)
Finally, the "src / index.js" file is the JavaScript entry point for Webpack. It does global styling and rendering of the App component.
src / index.js:
import React from 'react'
import { render } from 'react-dom'
import { createGlobalStyle } from 'styled-components'
//
import 'bootstrap/dist/css/bootstrap.min.css'
import 'emoji-mart/css/emoji-mart.css'
//
import { App } from './App'
// ""
const GlobalStyles = createGlobalStyle`
.card-header {
padding: 0.25em 0.5em;
}
.card-body {
padding: 0.25em 0.5em;
}
.card-text {
margin: 0;
}
`
const root = document.getElementById('root')
render(
<>
<GlobalStyles />
<App />
</>,
root
)
Well, we have finished developing our little application.
It's time to make sure it works. To do this, in the root directory of the project (react-chat), execute the command "yarn start". After that, in the browser tab that opens, you should see something like this:



Instead of a conclusion
If you have a desire to improve the application, here are a couple of ideas:
- Add DB for users (using the same lowdb)
- Add a second room - for this it is enough to implement separate processing of message lists on the server
- ( ) —
- — MongoDB Cloud Mongoose; Express
- : (, , ..) — react-filepond, — multer; WebRTC
- From the more exotic: add voice over to text and translate voice messages into text - you can use react-speech-kit for this
Some of these ideas are included in my plans to improve the chat.
Thank you for your attention and have a nice day.