So, a drop of theory
FTP (File Transfer Protocol) is a network file transfer protocol.
FTP requires two: an FTP server and an FTP client.
The server provides access by login and password to the necessary files and, accordingly, shows the user only those files and folders to which he has access.
Client is a program for FTP connection. The main idea is that the user can operate on the file data on the server side: view, edit, copy, download and delete.
Everything is logical.
Unlike, say, the same HTTP, FTP uses a double connection as a connection model. In this case, one channel is the control one, through which commands are received to the server and its responses are returned (usually through TCP port 21), and through the rest the actual data transfer takes place, one channel for each transfer. Therefore, within the framework of one session via the FTP protocol, several files can be simultaneously transferred, and in both directions.
If you describe the process of establishing a connection and further transferring data point by point, you get something like the following:
- The user activates the client application and connects to the server by entering a username and password.
- — .
- , FTP- ( , FTP — , , ), , (, , , ..).
- ( , ), , FPT — , .
. - Upon completion of the transfer, this connection is closed, but the control channel between the interpreters remains open, as a result of which the user can reopen the data transfer within the same session.
FTP - the default connection is port 21, unless another port is set.
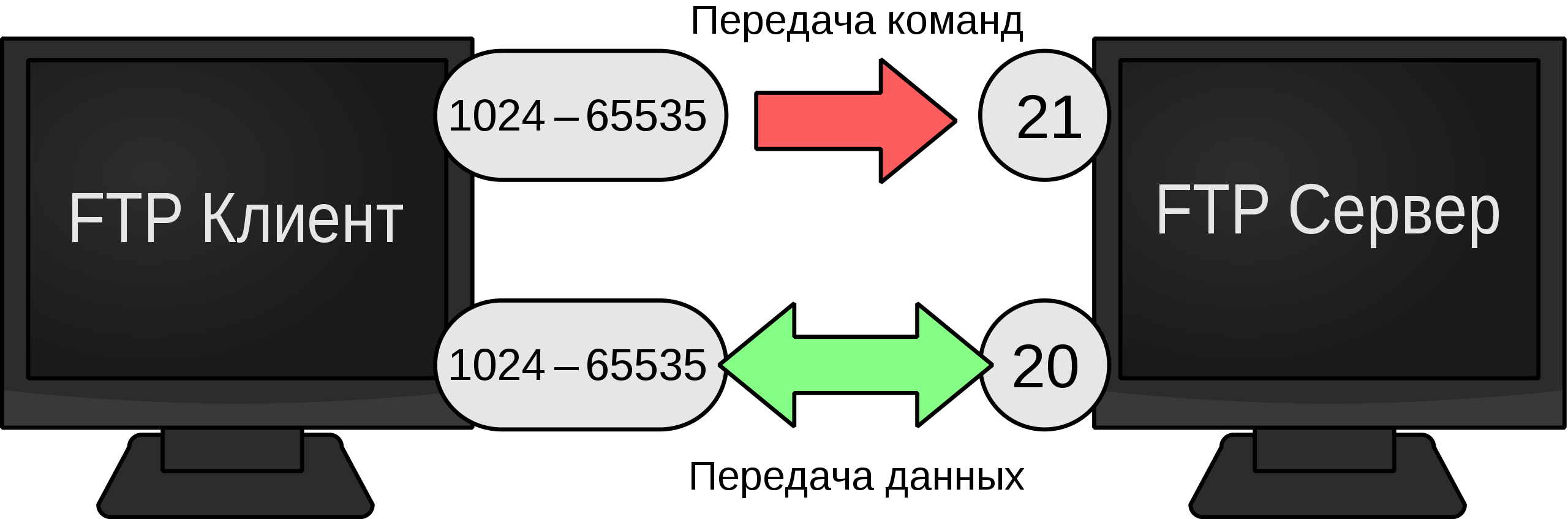
Implementation
In this case, we will use the FTPClient class from the Apache library (org.apache.commons.net.ftp.FTPClient). We create an object of the class, set a timeout. Then we must first connect to the server using connect before doing anything (and remember to disconnect after we're done). In connect, as the first parameter, we write the local address of the host, the second argument is the port for connection. You also need to check the FTP response code (too obvious, but still) to make sure the connection was successful.
import org.apache.commons.net.ftp.FTP
import org.apache.commons.net.ftp.FTPClient
import org.apache.commons.net.ftp.FTPReply
...
val con = FTPClient()
con.connectTimeout =
con.connect("192.168.0.105", 21)
if (FTPReply.isPositiveCompletion(con.replyCode)) {
//
}
Ok, we are connected to the server. What's next? Now you need to go through authorization under the username, which we, in fact, created for this. But before that, you need to set the current data connection mode to PASSIVE_LOCAL_DATA_CONNECTION_MODE using the enterLocalPassiveMode () command. This means that all transfers will occur between the client and the server, and that the server is in passive mode, waiting for the client to connect to initiate the transfer. (for example, ACTIVE_LOCAL_DATA_CONNECTION_MODE implies that the server will initiate the connection).
After that, in login, write the username and password. storeFile () stores the file on the server using the given name and accepting input from the given InputStream. I had a task to periodically write to a specific file on the server, so it looks like this:
con.enterLocalPassiveMode()
if (con.login("nad", "nad")) { // (, )
con.setFileType(FTP.BINARY_FILE_TYPE)
val msg = "your_msg"
val msg_bytes: InputStream = ByteArrayInputStream(msg.toByteArray())
val result: Boolean = con.storeFile("/sms.txt", msg_bytes)
msg_bytes.close()
if (result) Log.v("upload result", "succeeded")
con.logout()
con.disconnect()
}
}
catch (ex: IOException) {
ex.printStackTrace()
return Result.failure()
}
If you want to copy your file to the server in its entirety, you can do this (the essence does not change much, but suddenly someone needs it):
val file = File(" ")
val msg_bytes = FileInputStream(file)
All in all, this is all you might need. And, of course, a little about setting up a local FTP server. I have this, as I wrote earlier, FileZilla. We leave everything by default. Create a user and assign him a folder on the host, from which he can read / write / delete, etc.

That's all. Launch and view the logs on the server. And here's what you should get:

Good luck!