In anticipation of the start of the "C # ASP.NET Core developer" course, we have prepared a traditional translation of useful material.
We also recommend watching the webinar on "Differences in Structural Design Patterns by Examples" . In this open-ended lesson, participants and an expert instructor will explore three structural design patterns: Proxy, Adapter, and Decorator.

Introduction
ASP.NET Core. (exception handling) — , . — , . , .
ASP.NET Core , , , . API- . (Global Exception handler), . , . , , . , , ASP.NET Core Web API.
ASP.NET Core Web API Visual Studio 2019
, , ASP.NET Web API. , .
Microsoft Visual Studio «Create a New Project» ( ).
«Create New Project» «ASP.NET Core Web Application for C#» (- ASP.NET Core C#) «Next» ().
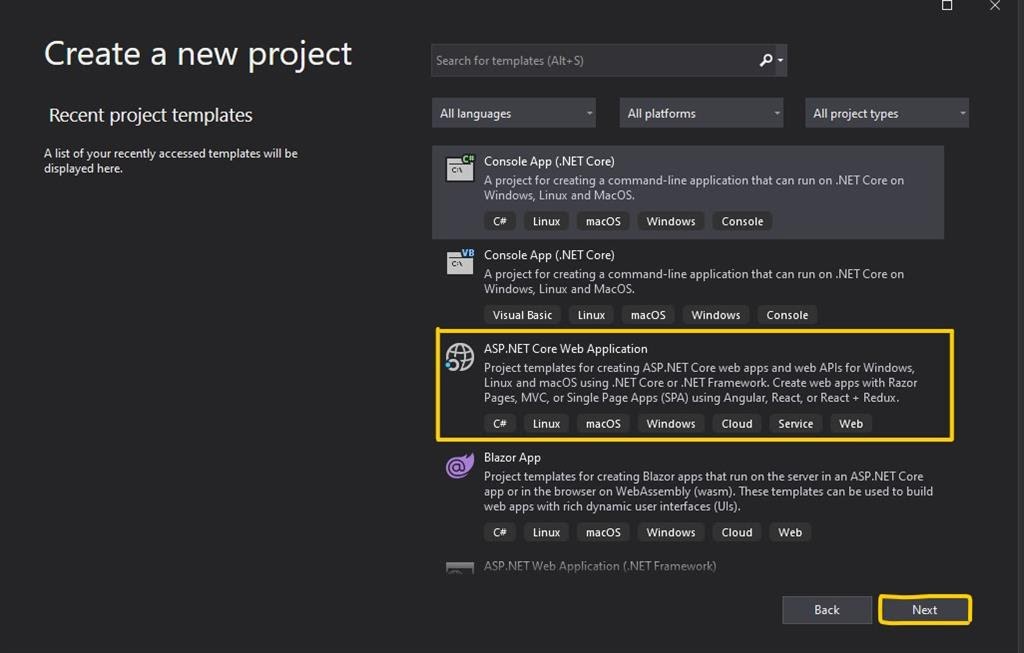
«Configure your new project» ( ) «Create» ().
«Create a New ASP.NET Core Web Application» ( - ASP.NET Core) «API» «Create».
, «Enable Docker Support» ( Docker) «Configure for HTTPS» ( HTTPS) . .
, «No Authentication» ( ), .
.
UseExceptionHandler middleware ASP.NET Core.
, Middleware ASP.NET Core. Middleware , , - . middleware ASP.NET Core UseExceptionHandler . ASP.NET Core middleware-. , , , . middleware- , , .
middleware , , , . ., API . middleware configure()
startup.cs
. - MVC, middleware , . , middleware UseExceptionHandler
.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseExceptionHandler("/Home/Error");
app.UseMvc();
}
. WeatherForecastController.cs
-, :
[Route("GetExceptionInfo")]
[HttpGet]
public IEnumerable<string> GetExceptionInfo()
{
string[] arrRetValues = null;
if (arrRetValues.Length > 0)
{ }
return arrRetValues;
}
, , , . ., middleware —
app.UseExceptionHandler(
options =>
{
options.Run(
async context =>
{
context.Response.StatusCode = (int)HttpStatusCode.InternalServerError;
context.Response.ContentType = "text/html";
var exceptionObject = context.Features.Get<IExceptionHandlerFeature>();
if (null != exceptionObject)
{
var errorMessage = $"<b>Exception Error: {exceptionObject.Error.Message} </b> {exceptionObject.Error.StackTrace}";
await context.Response.WriteAsync(errorMessage).ConfigureAwait(false);
}
});
}
);
API :

Middleware API ASP.NET Core
, middleware
. , middleware
. middleware
. , , - , . middleware
:
using Microsoft.AspNetCore.Http;
using Newtonsoft.Json;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Threading.Tasks;
namespace API.DemoSample.Exceptions
{
public class ExceptionHandlerMiddleware
{
private readonly RequestDelegate _next;
public ExceptionHandlerMiddleware(RequestDelegate next)
{
_next = next;
}
public async Task Invoke(HttpContext context)
{
try
{
await _next.Invoke(context);
}
catch (Exception ex)
{
}
}
}
}
middleware
. Middleware
, middleware
. , , HandleExceptionMessageAsync
catch
. , Invoke
, :
public async Task Invoke(HttpContext context)
{
try
{
await _next.Invoke(context);
}
catch (Exception ex)
{
await HandleExceptionMessageAsync(context, ex).ConfigureAwait(false);
}
}
HandleExceptionMessageAsync
, :
private static Task HandleExceptionMessageAsync(HttpContext context, Exception exception)
{
context.Response.ContentType = "application/json";
int statusCode = (int)HttpStatusCode.InternalServerError;
var result = JsonConvert.SerializeObject(new
{
StatusCode = statusCode,
ErrorMessage = exception.Message
});
context.Response.ContentType = "application/json";
context.Response.StatusCode = statusCode;
return context.Response.WriteAsync(result);
}
, , ExceptionHandlerMiddlewareExtensions
,
using Microsoft.AspNetCore.Builder;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace API.DemoSample.Exceptions
{
public static class ExceptionHandlerMiddlewareExtensions
{
public static void UseExceptionHandlerMiddleware(this IApplicationBuilder app)
{
app.UseMiddleware<ExceptionHandlerMiddleware>();
}
}
}
, middleware Configure startup, :
app.UseExceptionHandlerMiddleware();
— . . ASP.NET Core, , , . , , . , , , .