Hello everyone! The idea for this article came a month ago, but due to being busy at work, time was sorely lacking. One evening on YouTube I came across a video about making a pixel art platformer game. And then I remembered my first computer science lessons at school, where we "drew in BASIC" and played "the crow eats letters".
Foreword
The year was 2000. The crisis of 98 is over. I was in 8th grade at a local school in a small town. With the beginning of the school year, a small event awaited everyone - a computer science lesson was introduced. Many treated this as another subject that needs to be taught, but there were also those whose eyes lit up. I was among the latter.
It should be noted that although computer science was introduced, they forgot to "introduce new computers" because there was no money for these purposes. At that time, our school was served by machines made in USSR - " Electronics MC 0511 " and several of their slightly more modern counterparts. They worked only according to their own laws, or after the arrival of a certain "Nikolai Vladimirovich" - a local master.
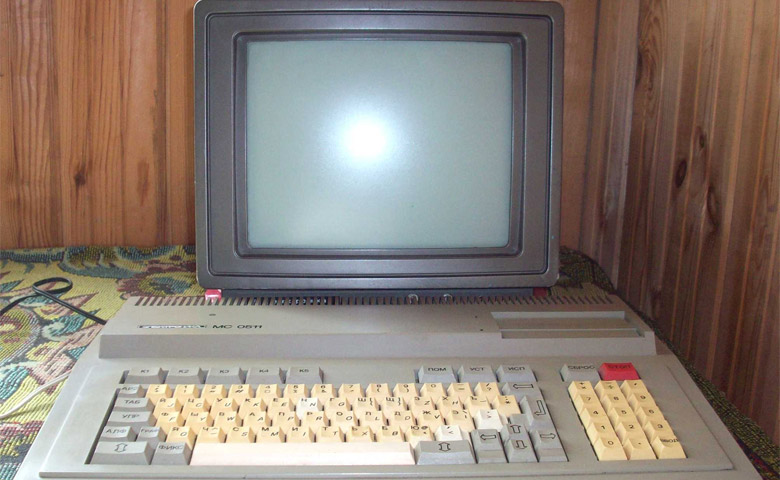
"" - 26 , . . . , . , .
, , . - , , .
BufferedImage. , .
fun drawPixel(
x:Int, y:Int, red:Int, green:Int, blue: Int,
image: BufferedImage
) {
image.setRGB(x, y, Color(red,green,blue).rgb)
}
, . , - redRng, greenRng blueRng .
fun drawRandImage(
image: BufferedImage, stepSize: Int = 1,
redRng: Int = 255, greenRng: Int = 255, blueRng: Int = 255
) {
for(posX in 0 until image.width step stepSize){
for (posY in 0 until image.height step stepSize) {
val r = if (redRng <= 0) 0 else Random.nextInt(0, redRng)
val g = if (greenRng <= 0) 0 else Random.nextInt(0, greenRng)
val b = if (blueRng <= 0) 0 else Random.nextInt(0, blueRng)
drawPixel(posX, posY, r, g, b, image)
}
}
}
stepSize , .

- . . ImageIO. - , Thread.
fun writeImage(img: BufferedImage, file: String) {
val imgthread = Thread(Runnable {
ImageIO.write(img, File(file).extension, File(file))
})
try {
imgthread.start()
} catch (ex: Exception) {
ex.printStackTrace()
imgthread.interrupt()
}
}
, "" .
ArrayList<List<Int>>. "" drawTitle, "" drawPixel, "big pixel" .
fun drawTile(
startX: Int, startY: Int, size: Int,
red: Int, green: Int, blue: Int, image: BufferedImage
) {
for (posX in startX until startX+size) {
for (posY in startY until startY+size) {
drawPixel(posX,posY,red,green,blue,image)
}
}
}
. -. when 4 …
fun drawImage(pixels: ArrayList<List<Int>>, image: BufferedImage) {
pixels.forEachIndexed { posY, row ->
row.forEachIndexed { posX, col ->
when(col) {
1 -> drawTile(posX*10,posY*10,10,255,2,0,image)
2 -> drawTile(posX*10,posY*10,10,156,25,31,image)
3 -> drawTile(posX*10,posY*10,10,255,255,255,image)
else -> drawTile(posX*10,posY*10,10,23,0,44,image)
}
}
}
}
… , (1 = , 2 = -, 3 = , 4 = )
val map = arrayListOf(
listOf(0,0,0,0,0,0,0,0,0,0,0,0,0,0,0),
listOf(0,0,0,1,1,1,0,0,0,1,2,2,0,0,0),
listOf(0,0,1,3,3,1,1,0,1,1,1,2,2,0,0),
listOf(0,1,3,3,1,1,1,1,1,1,1,1,2,2,0),
listOf(0,1,3,1,1,1,1,1,1,1,1,1,2,2,0),
listOf(0,1,1,1,1,1,1,1,1,1,1,1,2,2,0),
listOf(0,1,1,1,1,1,1,1,1,1,1,1,2,2,0),
listOf(0,0,1,1,1,1,1,1,1,1,1,2,2,0,0),
listOf(0,0,0,1,1,1,1,1,1,1,2,2,0,0,0),
listOf(0,0,0,0,1,1,1,1,1,2,2,0,0,0,0),
listOf(0,0,0,0,0,1,1,1,2,2,0,0,0,0,0),
listOf(0,0,0,0,0,0,1,2,2,0,0,0,0,0,0),
listOf(0,0,0,0,0,0,0,2,0,0,0,0,0,0,0),
listOf(0,0,0,0,0,0,0,0,0,0,0,0,0,0,0),
)
... . "" .

, " " , - , . , , .
Excel
. JS (React JS), , JavaScript . - …
, Excel. - . . " " - , Apache POI - word, excel, pdf. , .
hex rgba, Color.
val toRGBA = { hex: String ->
val red = hex.toLong(16) and 0xff0000 shr 16
val green = hex.toLong(16) and 0xff00 shr 8
val blue = hex.toLong(16) and 0xff
val alpha = hex.toLong(16) and 0xff000000 shr 24
Color(red.toInt(),green.toInt(),blue.toInt(),alpha.toInt())
}
, .
fun getPixelColors(file: String, listName: String): ArrayList<List<String>> {
val table = FileInputStream(file)
val sheet = WorkbookFactory.create(table).getSheet(listName)
val rowIterator: Iterator<Row> = sheet.iterator()
val rowArray: ArrayList<Int> = ArrayList()
val cellArray: ArrayList<Int> = ArrayList()
while (rowIterator.hasNext()) {
val row: Row = rowIterator.next()
rowArray.add(row.rowNum)
val cellIterator = row.cellIterator()
while (cellIterator.hasNext()) {
val cell = cellIterator.next()
cellArray.add(cell.address.column)
}
}
val rowSize = rowArray.maxOf { el->el }
//...
//...
return pixelMatrix
}
( ). , , . .
, , . ...
val rows = sheet.lastRowNum
val cells = sheet.getRow(rows).lastCellNum // + rows
val pixArray = Array(rows+1) {Array(ccc+1) {""} }
... OutOfBounds. (row) , , . , "", . iterator.hasNext(), .
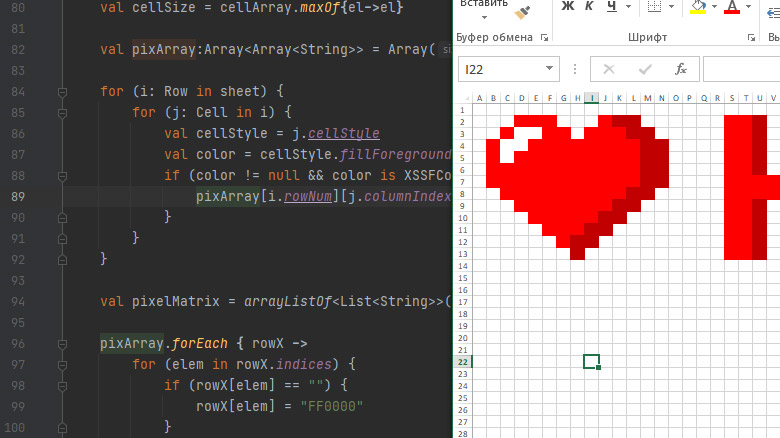
- " " BufferedImage. , - TYPE_INT_ARGB, .
fun renderImage(pixels: ArrayList<List<String>>): BufferedImage {
val resultImage = BufferedImage(
pixels[0].size*10,
pixels.size*10,
BufferedImage.TYPE_INT_ARGB
)
pixels.forEachIndexed { posY, row ->
row.forEachIndexed { posX, col ->
drawTile(
(posX)*10,(posY)*10, 10,
toRGBA(col).red, toRGBA(col).green,toRGBA(col).blue,
toRGBA(col).alpha, resultImage
)
}
}
return resultImage
}
, .
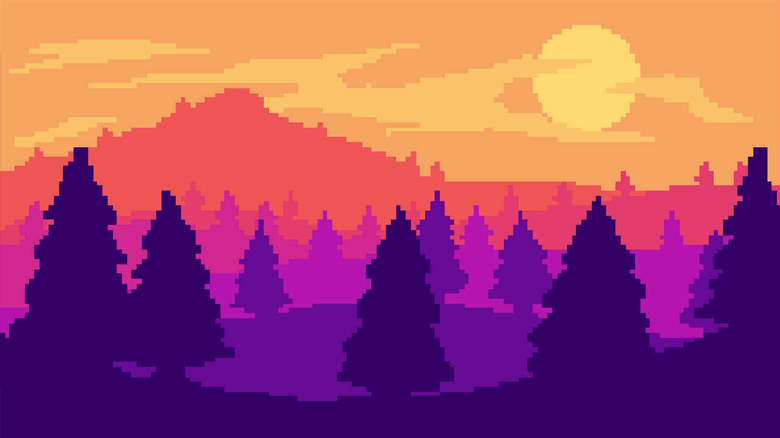
github. ? svg, (blur, glitch, glow, etc..), “ ” , xls (HSSF Color) . - , , - .
, " " (ctrl+c, ctrl+v), "" . , : , , - " ". , , , .
14 , , .
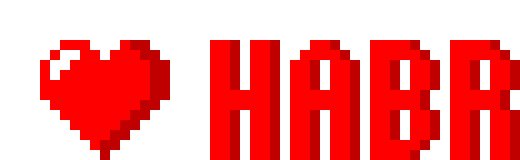
Even if in a couple of years "Electronics MS" was replaced by its modern Pentium-based counterparts, those first lessons on old computers will forever remain with me, because it was they who put my love for computers and everything connected with them into me ...
How did computer science begin at your school?
Thanks to all! Bye everyone!