How it all started
Very often, when working with Enterprise applications, we have to deal with such screens in the absence of data received from the backend, when we simply need to display a list on the screen.
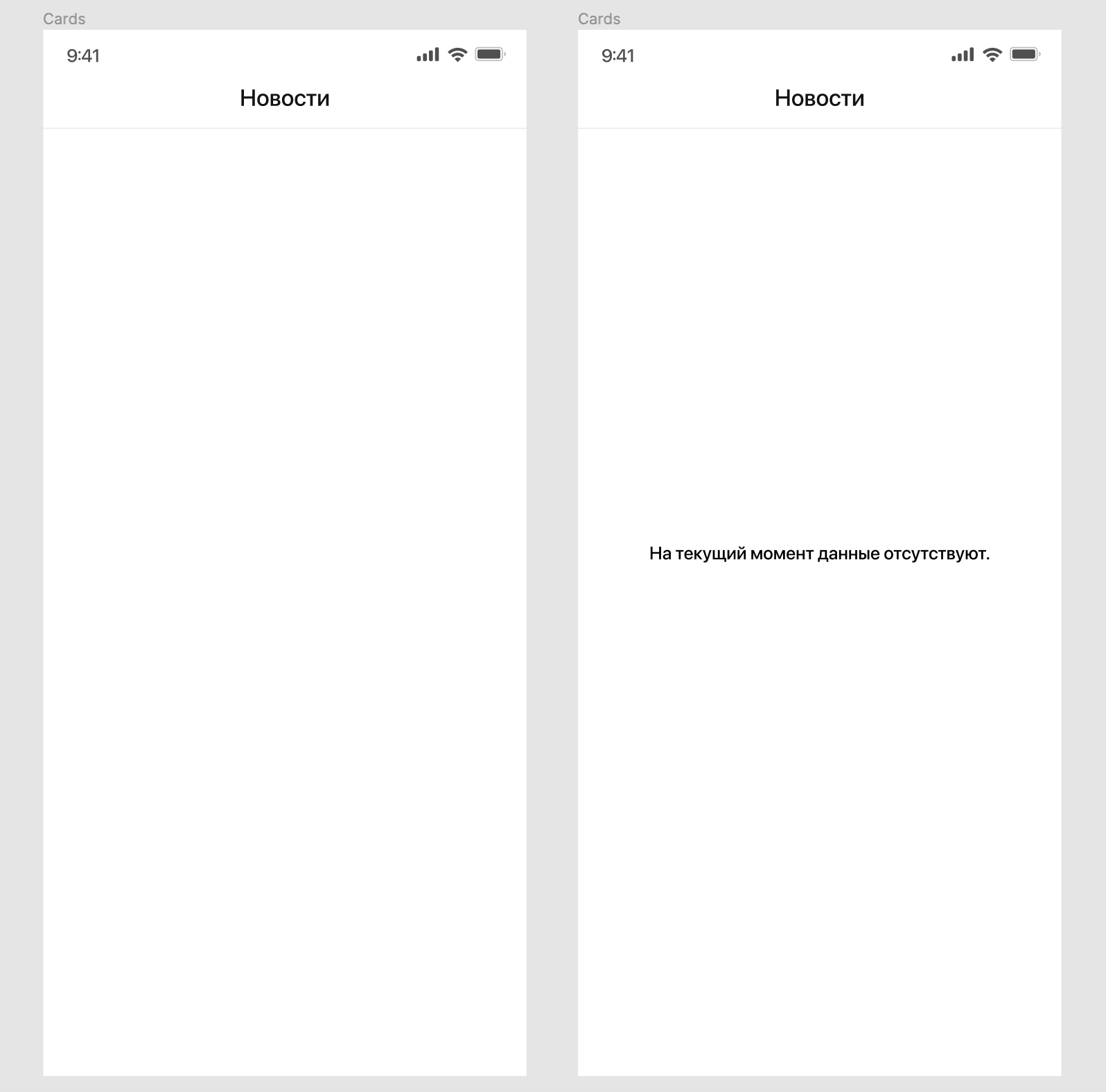
, , , , , .
, , . , .

-, . -, UI- - .
public class EmptyStateViewModel : ViewModel
{
public EmptyStateViewModel(string image, string title, string description)
{
Image = image;
Title = title;
Description = description;
}
public string Image { get; }
public string Title { get; }
public string Description { get; }
}
( xaml Xamarin Forms) Bindings , , mvvm- .
?
- , - . - EmptyStateView, Retry, . EmptyStateViewModel, , .

public class ErrorStateViewModel : EmptyStateViewModel
{
public ErrorStateViewModel(string image, string title, string description, string actionTitle, Command actionCommand)
: base(image, title, description)
{
ActionTitle = actionTitle;
ActionCommand = actionCommand;
}
public string ActionTitle { get; }
public Command ActionCommand { get; }
}
?
- . . , -. None, null.
public static class OverlayFactory
{
public static T None<T>()
where T : EmptyStateViewModel
{
return null;
}
public static EmptyStateViewModel CreateCustom(string image, string title, string description)
{
return new EmptyStateViewModel(image, title, description);
}
public static ErrorStateViewModel CreateCustom(string image, string title, string description, string actionTitle, Command actionCommand)
{
return new ErrorStateViewModel(image, title, description, actionTitle, actionCommand);
}
}
- -, , ,
public class SomeViewModel : BaseViewModel
{
private IItemsLoadingService _itemsLoadingService;
public SomeViewModel(IItemsLoadingService itemsLoadingService)
{
_itemsLoadingService = itemsLoadingService;
}
public ObservableCollection<ItemViewModel> Items { get; } = new ObservableCollection<ItemViewModel>();
public EmptyStateViewModel EmptyState { get; protected set; }
public ErrorStateViewModel ErrorState { get; protected set; }
public override async Task InitializeAsync()
{
await base.InitializeAsync();
await LoadItemsAsync();
}
private async Task LoadItemsAsync()
{
try
{
var result = await _itemsLoadingService.GetItemsAsync();
var items = result.ToList();
ErrorState = OverlayFactory.None<ErrorStateViewModel>();
if (items.Count == 0)
{
EmptyState = OverlayFactory.CreateCustom("img_empty_state", "Title", "Description");
}
else
{
EmptyState = OverlayFactory.None<ErrorStateViewModel>();
// Add items to list
}
}
catch
{
ErrorState = OverlayFactory.CreateCustom("img_error_state", "Title", "Description", "Retry", new Command(() => LoadItemsAsync));
}
}
}
Binding EmptyState/ErrorState , mvvm-, , EmptyStateViewModel/ErrorStateViewModel null, . SetViewModel.
, View ViewModel View ViewState . ViewModel null - ViewState Gone, - Visible:
public void SetViewModel(EmptyStateViewModel viewModel)
{
ViewModel = viewModel;
View.Visibility = viewModel != null ? ViewStates.Visible : ViewStates.Gone;
}
iOS - constraints , - . enum, Android.
public void SetViewModel(EmptyStateViewModel viewModel)
{
ViewModel = viewModel;
View.SetVisibility(viewModel != null ? ViewStates.Visible : ViewStates.Gone);
}
extension
public static void SetVisibility(this UIView view, ViewVisibility visibility)
{
var constraints = GetViewConstraints(view) ?? new NSLayoutConstraint[] {};
if (visibility == ViewVisibility.Gone)
{
SaveViewConstraints(view, constraints);
NSLayoutConstraint.DeactivateConstraints(constraints);
view.Hidden = true;
return;
}
if (visibility == ViewVisibility.Visible)
{
SaveViewConstraints(view, null);
NSLayoutConstraint.ActivateConstraints(constraints);
view.Hidden = false;
return;
}
}
Here, in the case of setting ViewVisibility.Gone, we pre-save the constraints of our view and deactivate them, and when the visibility is turned on, on the contrary, we get the previously saved constraints, reset the preservation, and then activate them.
private static NSLayoutConstraint[] GetViewConstraints(UIView view)
{
return view.GetAssociatedObject<NSMutableArray<NSLayoutConstraint>>(Key)?.ToArray() ??
view.Superview?.Constraints
.Where(constraint => (constraint.FirstItem?.Equals(view) == true) || constraint.SecondItem.Equals(view))
.ToArray();
}
private static void SaveViewConstraints(UIView view, NSLayoutConstraint[] constraints)
{
NSMutableArray<NSLayoutConstraint> viewConstraints = null;
if (constraints.Length > 0)
{
viewConstraints = new NSMutableArray<NSLayoutConstraint>();
viewConstraints.AddObjects(constraints);
}
view.SetAssociatedObject(Key, viewConstraints, AssociationPolicy.RetainNonAtomic);
}
The first method allows you to get previously saved constraints, if any, or, if not, get the current ones. If there is no parent view, then null will be returned.
The second method will save the current constraints so that they can be restored later.
Thus, it turned out to make more pleasant screens with missing data, or error status screens.
PS - the first article on Habré, so don't judge strictly. But you need to start somewhere.