This is my first post where I want to tell you about the most famous cellular automaton "Game of Life", and also write it in Python using Pygame graphics.
Conways Game of life (in Russian 'Game of life') is a cellular automaton invented by John Conway back in 1970.
The rules are very simple, the whole game takes place in 2D space (plane) on which there can be 2 types of cells "Living" - 0 and "Empty" -1. The basic rules for the life of a cell are Birth3 Survive23, which means a cell is born with three neighbors and survives with two or three, otherwise it dies.
Determination of the number of neighbors occurs in the Moore neighborhood.
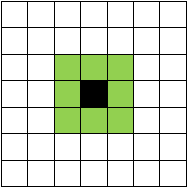
A little background history from Wikipedia.
John Conway became interested in a problem proposed in the 1940s by the famous mathematician John von Neumann, who was trying to create a hypothetical machine that could reproduce itself. John von Neumann managed to create a mathematical model of such a machine with very complex rules. Conway tried to simplify the ideas proposed by Neumann, and in the end he managed to create the rules that became the rules of the game "Life".
The description of this game was first published in the October (1970) issue of Scientific American, under the heading "Math Games" by Martin Gardner
I'm sure you are tired of all this theory, let's start writing a simulation in Python / Pygame
Python, .
pygame "pip install pygame" "pip3 install pygame" ( "pip " , PATH Python)
,
#
import pygame as p
from pygame.locals import *
# RGB
BLACK = (0 , 0 , 0)
WHITE = (255 , 255 , 255)
#
root = p.display.set_mode((1000 , 500))
#
while 1:
#
root.fill(WHITE)
#
for i in range(0 , root.get_height() // 20):
p.draw.line(root , BLACK , (0 , i * 20) , (root.get_width() , i * 20))
for j in range(0 , root.get_width() // 20):
p.draw.line(root , BLACK , (j * 20 , 0) , (j * 20 , root.get_height()))
# " "
for i in p.event.get():
if i.type== QUIT:
quit()
p.display.update()
-
-
system
count
"", counter.
count
# 2
cells=[ [0 for j in range(root.get_width()//20)] for i in range(root.get_height()//20)]
cells2=cells
# -
def near(pos: list , system=[[-1 , -1] , [-1 , 0] , [-1 , 1] , [0 , -1] , [0 , 1] , [1 , -1] , [1 , 0] , [1 , 1]]):
count = 0
for i in system:
if cells[(pos[0] + i[0]) % len(cells)][(pos[1] + i[1]) % len(cells[0])]:
count += 1
return count
, .
#
for i in range(len(cells)):
for j in range(len(cells[0])):
#
if cells[i][j]:
# 2 3
if near([i , j]) not in (2 , 3):
cells2[i][j] = 0
continue
#
cells2[i][j] = 1
continue
# 3
if near([i , j]) == 3:
cells2[i][j] = 1
continue
#
cells2[i][j] = 0
cells = cells2
#
import time
import pygame as p
import random
from pygame.locals import *
# RGB
BLACK = (0 , 0 , 0)
WHITE = (255 , 255 , 255)
#
root = p.display.set_mode((1000 , 500))
# 2
cells = [[random.choice([0 , 1]) for j in range(root.get_width() // 20)] for i in range(root.get_height() // 20)]
# -
def near(pos: list , system=[[-1 , -1] , [-1 , 0] , [-1 , 1] , [0 , -1] , [0 , 1] , [1 , -1] , [1 , 0] , [1 , 1]]):
count = 0
for i in system:
if cells[(pos[0] + i[0]) % len(cells)][(pos[1] + i[1]) % len(cells[0])]:
count += 1
return count
#
while 1:
#
root.fill(WHITE)
#
for i in range(0 , root.get_height() // 20):
p.draw.line(root , BLACK , (0 , i * 20) , (root.get_width() , i * 20))
for j in range(0 , root.get_width() // 20):
p.draw.line(root , BLACK , (j * 20 , 0) , (j * 20 , root.get_height()))
# " "
for i in p.event.get():
if i.type == QUIT:
quit()
#
for i in range(0 , len(cells)):
for j in range(0 , len(cells[i])):
print(cells[i][j],i,j)
p.draw.rect(root , (255 * cells[i][j] % 256 , 0 , 0) , [i * 20 , j * 20 , 20 , 20])
#
p.display.update()
cells2 = [[0 for j in range(len(cells[0]))] for i in range(len(cells))]
for i in range(len(cells)):
for j in range(len(cells[0])):
if cells[i][j]:
if near([i , j]) not in (2 , 3):
cells2[i][j] = 0
continue
cells2[i][j] = 1
continue
if near([i , j]) == 3:
cells2[i][j] = 1
continue
cells2[i][j] = 0
cells = cells2
Everything worked out, the speed does not frustrate either.
In the next articles we will try to implement modifications of the game "Life".