
1. Use relative paths in configuration
Do not specify an absolute path in
settings.py
, this will be useful if you will later run the project from different locations, for example, when deploying to another web server with different paths. Use the following code in settings.py if your templates and static files are located inside the Django project directory:
# settings.py
import os
PROJECT_DIR = os.path.dirname(__file__)
...
STATIC_DOC_ROOT = os.path.join(PROJECT_DIR, "static")
...
TEMPLATE_DIRS = (
os.path.join(PROJECT_DIR, "templates"),
)
2. Use the {% url%} tag
Instead of hardcoding your links, try using a backward compatible tag
{% url %}
. This will give you an absolute URL, but if the project is moved, the links will remain valid.
Basically it
{% url %}
takes the name of the view and its parameters and does a reverse lookup to return the requested URL. If you make changes to urls.py, the links won't break.
3. Use the Django admin for your PHP applications
One of Django's strengths is its user authentication system, which is built into the core of Django. It is very easy to configure and contains a convenient system for user authorization and setting the necessary settings.
This authorization system is so good that many suggest using it as an admin area for PHP applications.
4. Use a separate server for static processing
Django allows you to host static files in your dev environment, but not in your production environment.
Django is designed to save you from yourself. If you try to use static files from the same Apache instance serving Django, you will lose performance.
Apache reuses processes between each request, so once a process has cached all the code and libraries for Django, they remain in memory. And if you don't use this process to
process Django's request, all this memory will be wasted.
In case you use a separate server (or virtualhost) for static handling, your application performance will not be affected.
5. Use the Django debug toolbar.
Debugging tools are invaluable in any language. They speed up the development process by helping to find bugs and potential problems that may arise.
The Django debug toolbar allows you to see all executed SQL queries while the view is being rendered, and you can also view the stacktrace for any of them.
6. Django unit testing
Unit testing is a good way to make sure that your code changes work as expected and don't break previous code. One of the great things about Django is that writing unit tests is incredibly easy. Django offers the ability to use doctest or unittest out of the box, and the Django documentation has great tutorials and code examples on how to set up unit tests to make finding bugs even easier.
7. Visualization of models
Install Django Command Extensions and pygraphviz and then use the following command to get a nice visualization of project models in Django:
./manage.py graph_models -a -g -o my_project.png
8. Virtualenv
Virtualenv + Python = magic wand. Virtualenv will isolate Python / Django settings for each individual project. This means that changes to one site will not affect other sites. It can also be useful when different versions of Django or python need to be kept on the server.
9. Use Memcache
When poor performance can be a problem, you will most likely want to install some sort of caching system. Django offers many options for caching, but memcached is by far the best.
Installing and using memcached is very easy if you use the cmemcache module. After the module is installed, you need to add one line to the configuration so that your pages will start serving even faster.
10. Autoloading custom tags that can be used in all templates
from django import template
template.add_to_builtins('project.app.templatetags.custom_tag_module')
If you add this to the module that is loaded by default (settings.py, urls.py, every app models.py), you will have access to all tags and filters from your custom module in any template, without using
{% load custom_tag_module %}
.
The argument to
template.add_to_builtins()
can be a path to any module; your custom module doesn't have to be tied to any specific application.
For example, it can also be a module located in the root of the project (for example: '
project.custom_tag_module
').
Is it worth learning Django in 2020?
If you are a beginner programmer wondering: Should I learn Django? The short answer is yes.
If you need to implement your idea with minimal costs and quickly, Django is the right tool. It is free, open source and makes developing web projects even easier. Django is the best solution for prototyping applications because it includes everything you need to create the functionality you need right out of the box. For example, the admin panel can be connected with one line of code.
If you are a developer already familiar with Python and are looking for a framework for the backend of your application or website, Django is also a good choice.
The framework contains libraries and tools that allow you to carry out various operations and efficiently process a large number of user requests in real time.
Therefore, the development process becomes fast as you do not have to create every component from scratch.
Django benefits
1. Fast
The developers made the framework in mind that it would be simple and at the same time fast. It contains libraries that will allow you not to reinvent the wheel or create a project from scratch.
2. Safe
When it comes to security, the framework allows you to avoid stepping on a rake and avoid many security problems, such as SQL injection, XSS, CSRF due to possible programmer errors.
3. Administration panel
The framework immediately has an administration panel and an authorization system, which allows you to save time on user management and create a separate administration panel for the backend.
4. Scalable
The framework is suitable for projects with varying degrees of workload, and if a small project may grow in the future, no problem - Django scales perfectly under heavy workloads.
5. Data science and analytics
We already know that Django is built in Python, and Python is known for being a great tool for building projects using artificial intelligence and machine learning. Hence, if you are planning to integrate machine learning into your project or analyze and process large amounts of information, then you should definitely try Django.
6. Community support
There is a fairly large and active community around Django, and the framework itself has rich and user-friendly documentation.
Fast start
In order to immediately quickly start coding on Django with a team and testing, I built and uploaded a VPS image in the marketplace with an empty Django 3.1.3 project and
- http server Gunicorn 20.0.4
- Nginx 1.14.1 as a reverse proxy
- and a Postgresql 12.1 database server.

The cost of such a server will be 769 rubles per month - 538 rubles, if paid for a year.
And write your favorite life hacks while working with Django in the comments - let's try to collect a post of tips.
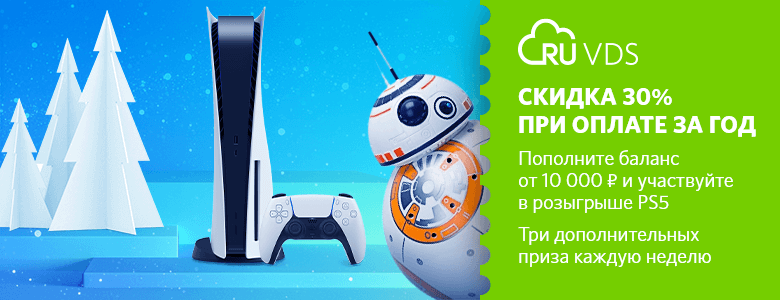
