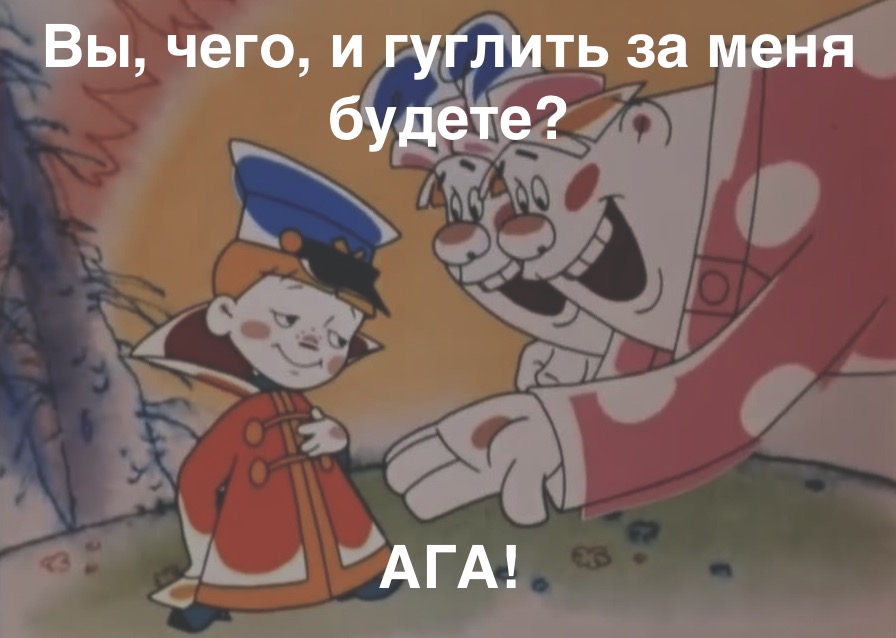
I am publishing the continuation of the collection of interview questions and answers for Backend-Java-developer. In the first part, we walked through Java and Spring. And in this one we'll talk about Hibernate, databases, patterns and development practices, one popular library, support and maintenance of our applications, and also look at alternative cheat sheets and summarize.
A GitHub repository with a full cheat sheet is here , and Habr is still a cake.
Questions
Hibernate
3 levels of caching:
- First-level cache. Enabled by default.
- Second-level cache. Disabled by default.
- (Query cache). .
:
- Baeldung
- First Level Cache Second Level Cache
Hibernate Vlad Mihalcea:
- High-Performance Java Persistence: 2, 16 โ Caching
- How does Hibernate Query Cache work
- How does Hibernate Collection Cache work
- How does Hibernate store second-level cache entries
- How does Hibernate NONSTRICT_READ_WRITE CacheConcurrencyStrategy work
- How does Hibernate TRANSACTIONAL CacheConcurrencyStrategy work
- How does Hibernate READ_ONLY CacheConcurrencyStrategy work
- How does Hibernate READ_WRITE CacheConcurrencyStrategy work
- Eager Loading โ , . (
@OneToOne
,@ManyToOne
,@OneToMany
,@ManyToMany
)fetch = FetchType.EAGER
.@OneToOne
@ManyToOne
. - Lazy Loading โ , . , (
@OneToOne
,@ManyToOne
,@OneToMany
,@ManyToMany
)fetch = FetchType.LAZY
.@OneToMany
,@ManyToMany
. proxy-, . LAZY- Hibernate, LazyInitializationException.
"N+1" .
N+1 Hibernate, .
N+1 , N , . N . slow query log
, , .
plain sql (jdbc, JOOQ), ( ) . , . " !?". - , - . ? JOIN
. .
, FetchType.EAGER
. N+1. @ManyToOne
. JPQL JOIN FETCH
. , FetchType.LAZY
.
FetchType.LAZY
, , N+1. , . โ . JOIN FETCH
.
JOIN FETCH
, bonjour. @OneToMany
FetchMode.SUBSELECT
โ 2 , . .. .
โ . @BatchSize
.
:
N+1, db-util Vlad Mihalcea. .
JOOQ N+1 , 17- (01:16:36) +. JOOQ.
:
- Vlad Mihalcea:
- Hibernate โ
- DOU JPA Hibernate
- JPoint " Hibernate "
4 JPA (Hibernate):
- MappedSuperclass โ . . @MappedSuperClass,
@Entity
. , , @AttributeOverride . . . - Single table โ . , -.
@Entity
-@Inheritance(strategy = InheritanceType.SINGLE_TABLE)
@DiscriminatorColumn(name = "YOUR_DISCRIMINATOR_COLUMN_NAME")
(DTYPE
VARCHAR
).@DiscriminatorValue("ThisChildName")
, - . , @DiscriminatorFormula,CASE...WHEN
โ JPA, Hibernate. . . โNULL
. - Joined table โ , . , ( ) .
@Entity
-@Inheritance(strategy = InheritanceType.JOINED)
.JOIN
,CASE...WHEN
,_clazz
, (0 (), 1, 2 ..) Hibernate . - Table per class โ
MappedSuperclass
, . . JPA 2.2 ( 2.12) , Hibernate , .@Entity
@Inheritance(strategy = InheritanceType.TABLE_PER_CLASS)
. (@Id
) .@AttributeOverride
โ .UNION
. , Hibernate_clazz
, (1, 2 ..).NULL AS some_field
. .
:
- : ,
- Vlad Mahalcea: MappedSuperclass
, JavaDoc :
JPA 2.2. pdf.
Postgresql. Postgres Pro:
WHERE HAVING , WHERE , ( , ), HAVING . , WHERE ; . HAVING, , . ( , HAVING, , . WHERE.)
:
- A โ (Atomicity). , .
- C โ (Consistency). .
- I โ (Isolation). .
- D โ (Durability). (, - ).
Read uncommitted
( ). (, ).Read committed
( ). . ยซยป ( , , ).Repeatable read
( ). (, ).Serializable
(). , , โ (, ).
:
- MSSQL: , ,
- PostgreSQL. Postgres Professional, egorov PostgreSQL (, , ..). Postgres Professional .
- MySQL: ,
:
- โ , . B-.
- โ , . .
: - โ . ID , ; ID ; ; . .
- โ , . , .
:
- Oracle, MySQL, PostgreSQL, MS SQL
- PostgreSQL: 1, 2, 3, 4, 5, 6, 7, 8, 9, 10
- ยซยป MySQL (InnoDB)
- ยซ ยป Postgres
- , , ?
- :
- : ,
- Database Indexes Explained
- Bitmap- B*tree-: ?
- MySQL
- PostgreSQL Java-
- 14 SQL Server,
- ,
- PostgreSQL
- Anatomy of an SQL Index
- SQL Indexing and Tuning e-Book
- Deep dive into Hash indexes for In-Memory OLTP tables
- Indexing based on Hashing
- SQL Server Indexes Interview Questions and Answers
- Top 25 SQL interview questions and answers about indexes
- An in-depth look at Database Indexin
โ , . , . . , , .
:
- shared (read) โ , . , , .
- exclusive (write) โ , . . , .
โ , ( VERSION
0
). , , . โ (, ) , .
, , . , .
Vlad Mihalcea:
- A beginnerโs guide to Java Persistence locking
- A beginnerโs guide to database locking and the lost update phenomena
- How do LockModeType.PESSIMISTIC_READ and LockModeType.PESSIMISTIC_WRITE work in JPA and Hibernate
- How does LockModeType.PESSIMISTIC_FORCE_INCREMENT work in JPA and Hibernate
- How does LockModeType.OPTIMISTIC work in JPA and Hibernate
- How does LockModeType.OPTIMISTIC_FORCE_INCREMENT work in JPA and Hibernate
- How to prevent OptimisticLockException with Hibernate versionless optimistic locking
- How does database pessimistic locking interact with INSERT, UPDATE, and DELETE SQL statements
- How does MVCC (Multi-Version Concurrency Control) work
- How does the 2PL (Two-Phase Locking) algorithm work
- How to prevent lost updates in long conversations
:
:
- Pessimistic Locking in JPA
- Optimistic Locking in JPA
- Enabling Transaction Locks in Spring Data JPA
- Deadlock
- Enum LockModeType
- Postgresql
- PostgreSQL Concurrency with MVCC
- Locking Hibernate
- Optimistic locking JOOQ
- Optimistically Locking Your Spring Boot Web Services
- Optimistic and pessimistic locking with SQL
, ,
, . . , . , , , .
- S โ (The Single Responsibility Principle). .
- O โ / (The Open Closed Principle). , .
- L โ (The Liskov Substitution Principle). . , .
- I โ (The Interface Segregation Principle). , .
- D โ (The Dependency Inversion Principle). , .
, , " " , . ? 3 " ". , , . , :)
, , , . Baeldung.
GoF. , , โ refactoring.guru. , (, , , ), , . . - .
(scale cube, The Art of Scalability) : sharding, mirrorring microservices.
- Sharding (data partioning) โ .
- Mirroring (horizontal duplication) โ .
- Microservices โ , -.
:
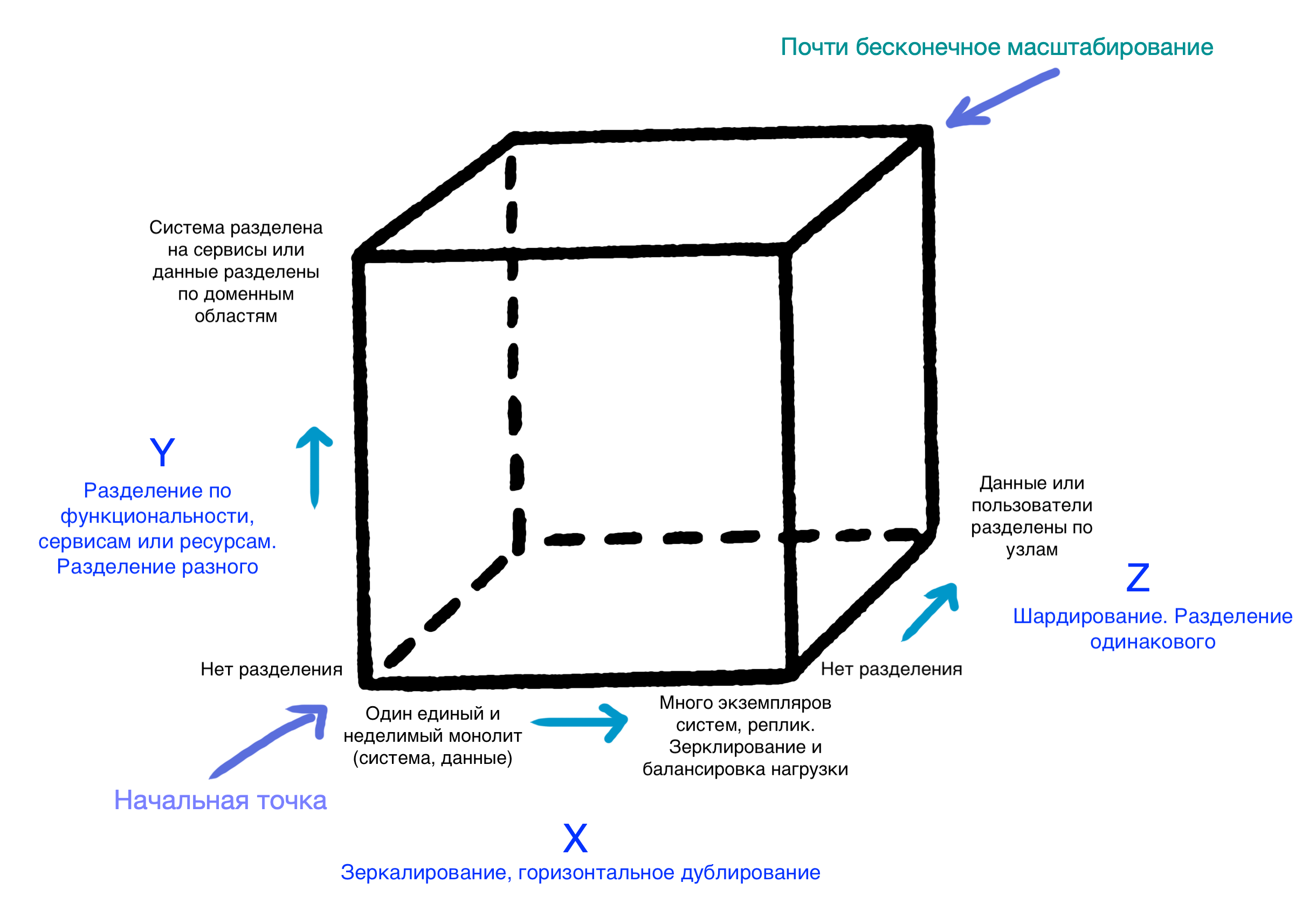
:
- .
- microservices.io.
- wiki pros cons.
- SCALING APPLICATIONS: THE SCALE CUBE.
- .
- .
- Microservice Patterns and Best Practices Microservices Patterns ( ).
-, . . - "".
โ Microservice Patterns and Best Practices. Medium .
. โ Enterprise Integration Patterns .
CAP โ , , :
- Consistency () โ โ . โ - , , .
- Availability () โ , .
- Partition tolerance ( ) โ , ( ) - ( ).
MongoDB, (), โ CP, โฆ , . .
.
:
- : , , , ,
- Visual Guide to NoSQL Systems CAP
- The CAP FAQ
- bigdataschool
- MongoDB stackoverflow
- CAP Theorem IBM
- CAP Theorem and Distributed Database Management Systems
- What is the CAP Theorem? medium
- (SOA) โ , , . SOA โ , . SOA - .
:
, MSA , SOA โ . . โ , . MSA SOA . , .
:
- SOA
- , SOA API ibm SOA MSA
- Monolith vs SOA vs MSA medium
- Microservices vs SOA DZone
- MSA SOA
- SOA MSA
:
- () -> , , , , , ( )
- ->
- ,
:
- () โ (/, ..)
- ,
:
- . : , , , , , 2018
- Wikipedia
- Microservices โ Not A Free Lunch!
- โ
- What are microservices?
- To go or not to go micro: the pros and cons of microservices
- Microservices in a Nutshell. Pros and Cons
:
(Test Double) โ ,
:
- Dummy โ , , . : , null
- Fake โ , , production-. : in-memory (fake database)
- Stub โ (system under test โ SUT)
- Spy โ Stub, , , ..
- Mock โ ,
Mock , โ .
:
- Mock vs Stub
- Mocks Aren't Stubs
- Test Double
- Test Stub
- Test Spy
- Mock Object
- Fake Object
- Interaction Based Testing Spock Framework
- Mockito
- Mockito Mock vs. Spy in Spring Boot Tests
- Tag: Mockito Bealdung
โโ , .
:
- . :
- โ , , . (Generic).
- (inclusive) โ , . .
- ( ad-hoc) โ () . :
- Java Challengers #3:
- JVM
- Java
- Java Virtual Machine Specification: Invoking Methods, invokevirtual, Linking
- Java Language Specification: Inheritance, Overriding, and Hiding, Conversions and Contexts, Type Inference
REST โ , web-.
REST, :
- URI
- โ JSON, XML
- โ HTTP. โ , . .
- HTTP . GET: ; POST: ; PUT: ; DELETE: . โ PUT vs PATCH vs POST.
- HTTP . HTTP- .
- HATEOAS , REST,
, , HTTP- URI, API RESTful. , . , , REST โ , . โ .
REST- :
- - (Clientโserver)
- (Stateless) โ
- (Cacheable) โ
- (Uniform interface) โ , , "" , HATEOAS
- (Layered system) โ , .
- ( ) (Code on demand (optional)) โ
SOAP โ .
SOAP:
- โ XML (SOAP-XML โ Envelope, Header, Body, Fault)
- SOAP- WSDL( XML)
- SOAP โ (TCP, UDP, HTTP, SMTP, FTP ..)
- HTTP, GET POST
, web-, .
-:
- REST โ , REST vs SOAP, Contract First, Code First, HATEOAS, Java Spring
- REST vs SOAP. 1. REST vs SOAP. 2. ?
- . ( !)
- REST API Best Practices
- RESTful
- RESTful API
- REST-like API
- web API
- RESTful API โ ( 1) RESTful API โ ( 2)
- : REST
- 7 REST API
- JAX-WS XSD Restrictions
- REST API Java
- Spring Boot: REST API Java
- RESTful Kotlin Spring boot
- SOAP- Java Apache CXF Spring
- SOAP Web- Spring-WS
-:
, ! !
Lombok โ , , , , . Lombok .
? ? , . โ .
โ : " - ?". . , -, .
, , Lombok โ IDEA. , . ! 2020.3 Lombok- IDEA.
Lombok , . , Hibernate ( ) Lombok entity-. @EqualsAndHashCode, @ToString @Data, equals
, hashCode
toString
, entities. entities .
, , "+" 7-8 โ . asm0dey Lombok Lombok . : " , , @SneakyThrows ?" ( ), " @Data. equals ?", " @EqualsAndHashCode StackOverflowException ?".
Lombok โ โ , . โ . Lombok โ Lombok, . - , , .
P.S. Lombok onX โ (@__
Java 7) . , Spring-.
:
.
:
- Apache JMeter โ , opensource, HTTP, HTTPS, FTP, LDAP, SOAP, TCP, shell-. , java-based , JDBC, Message-oriented middleware (MOM) JMS Java Objects.
- LoadRunner โ . , Enterprise.
- LoadNinja โ , - . , .
- WebLOAD โ . , , , CI/CD.
- LoadUI Pro โ SOAP UI, . - , API.
- K6 โ opensource , . JavaScript. , , , Kafka, Datadog, InfluxDB, JSON StatsD. CI-.
- . โ , โ hit-based- HTTP- Phantom jMeter. , , , .
- Gatling โ opensource Scala Netty Akka. Scala, Jenkins.
:
(Virtual Machine โ VM) โ / , . โ , . .. , , . . VM , . backup' VM .
VM .
โ / , , .
KVM QEMU, XEN, VMWare Hyper-V.
Docker โ , , , .. .
, . , . linux namespaces. cgroups.
, Dockerfile
, () , . , , . .
Docker- CI/CD.
Docker- โ Docker-Hub
Docker, .
Docker:
- VM Docker?
- " Docker": 1, 2, 3, 4, 5, 6
- Docker.
- docker- ( docker run runc)
- Docker: AWS
- : Docker .
- docker
- Linux namespaces
- Docker
- Docker
- Docker Y
- Docker , ,
- Docker-
- Docker
- 10 Docker: 1, 2
- Docker:
- Docker:
- containerd Docker
- : containerd "" CNCF
:
, :
- Containers vs. virtual machines
- Play with Docker
- Docker
- The Docker EcoSystem
- Hardware-assisted virtualization
- Full virtualization
- : KVM, Hyper-V VMware?
- KVM
- KVM
- Unix. 1: Kernel-based Virtual Machine (KVM)
- Linux/Cgroups
- : cgroups
- , VM Docker
- containerd
- awesome-compose
- Learn Docker & Containers using Interactive Browser-Based Scenarios
- What Is Docker & Docker Container? A Deep Dive Into Docker!
- Docker for beginners
โ - Java- โ , URL- URL ..
( -, ( Java EE)):
- โ Apache Tomcat. : (TomEE)
- Jetty
- Undertow
โ , Java EE ( Jakarta EE).
: - Wildfly
- Apache TomEE
- IBM WebSphere
- Eclipse GlassFish
-
- Java-backend, Java core (60 )
- Spring:
- Java 1: JPA Hibernate
- JavaSobes telegram
- Awesome-interview-questions/Java
- Java Developer
- Java Developer
- Java
- JBook
- Telegram- Java: fill the gaps
- Telegram- middlejava
- Telegram- Java Developer
- Telegram- microJUG miniJUG
- Telegram- javaswag
- 50 Docker, ,
- โ Java-
- Every Programmer Should Know
- : 1, 2, 3
Backend-Java- . , , , " " . , JUG.RU.
, , PR GitHub .
! ! ( ) . , โ .
In addition, memorizing the answers to the questions above does not guarantee successful employment, good work, and silkiness of hair.