Good day.
Today we will look at the disassembled code of instructions if, for, while, switch
that are written in the C language.

If statement
This instruction is fairly easy to distinguish in disassembled form from other instructions. Its distinguishing feature is the single conditional jump instructions je, jne, and other jump commands.


radare2. IDA PRO radare2 , radare2. IDA PRO.
IDA PRO


radare2

#include <stdio.h>
void main() {
int x = 1;
int y = 2;
if(x == y) {
printf("x = y\n");
}
else{
printf("x != y\n");
}
}
gcc. gcc -m32 prog_if.c -o prog_if
. -m32 , x86.
radare2, r2 prog_if
. aaa
main s main
. pdf
.
radare2
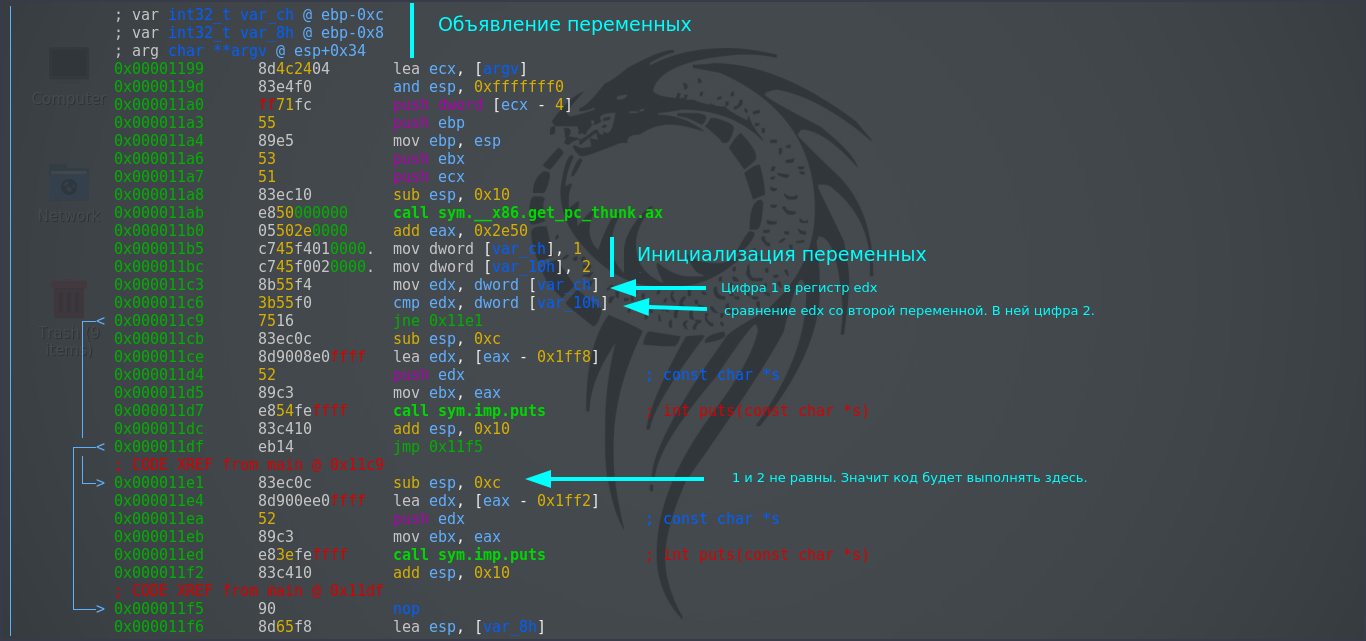
( int x; int y ), 1 var_ch ( x) 2 var10h ( y). (cmp) 1 2 (cmp edx, dword [var_10h]
). . jne ( jump if noe equal) 0x000011e1. if ( VV
radare2 IDA).

. . .
#include <stdio.h>
void main() {
int x = 0;
int y = 1;
int z = 2;
if(x == y) {
if(z == 0) {
printf("z = 0; x = y\n");
}
else{
printf("z = 0; x != y\n");
}
}
else {
if(z == 0) {
printf("z = zero and x != y.\n");
} else {
printf("z non-zero and x != y.\n");
}
}
}
radare2


.
for
for : , , /. for .
#include <stdio.h>
void main() {
int x;
for(x = 0; x < 100; x++) {
printf("x = %d", x);
}
}
radare2

1 - var_ch (x = 0)
2 - , jle. ( x 2, .)
3 - (printf)
4 - var_ch (++x)

while
while , - , . while for, . for, .
#include <stdio.h>
int func_1(int x);
int change_status();
int main() {
int status = 0;
while(status == 0) {
printf("int e = %d", func_1(5) );
status = change_status();
}
return 0;
}
int change_status() {
return 1;
}
int func_1(int x) {
int c;
int e;
int l;
c = 1 + 2;
e = x / 5;
l = 4 - 2;
return e;
}
radare2

1 - var_4h (status = 0)
2 - , je. ( x 0, .)
3 - (func1, printf, change_status)

switch
switch : .
#include <stdio.h>
int main() {
int i = 3;
switch(i) {
case 1:
printf("CASE_1 i = %d", i+4);
break;
case 2:
printf("CASE_2 i = %d", i+9);
break;
case 3:
printf("CASE_3 i = %d", i+14);
break;
}
return 0;
}
radare2

1 - var_4h (i = 3)
2 - (add, printf)
"case" , (cmp, je, jne) i case.



, ( ) , — switch if . , cmp je jne.
switch. case 4 .
#include <stdio.h>
int main() {
int i = 3;
switch(i) {
case 1:
printf("CASE_1 i = %d", i+4);
break;
case 2:
printf("CASE_2 i = %d", i+9);
break;
case 3:
printf("CASE_3 i = %d", i+14);
break;
case 4:
printf("CASE_3 i = %d", i+19);
break;
default:
break;
}
return 0;
}
radare2


1 - var_4h (i = 3)
2 - (add, printf)
c if . .




Graph mode is your friend in disassembly :)
That's all. I recommend trying to write C programs yourself, compile and study the disassembled code. Practice and practice again!
Thanks for attention. Do not be ill.