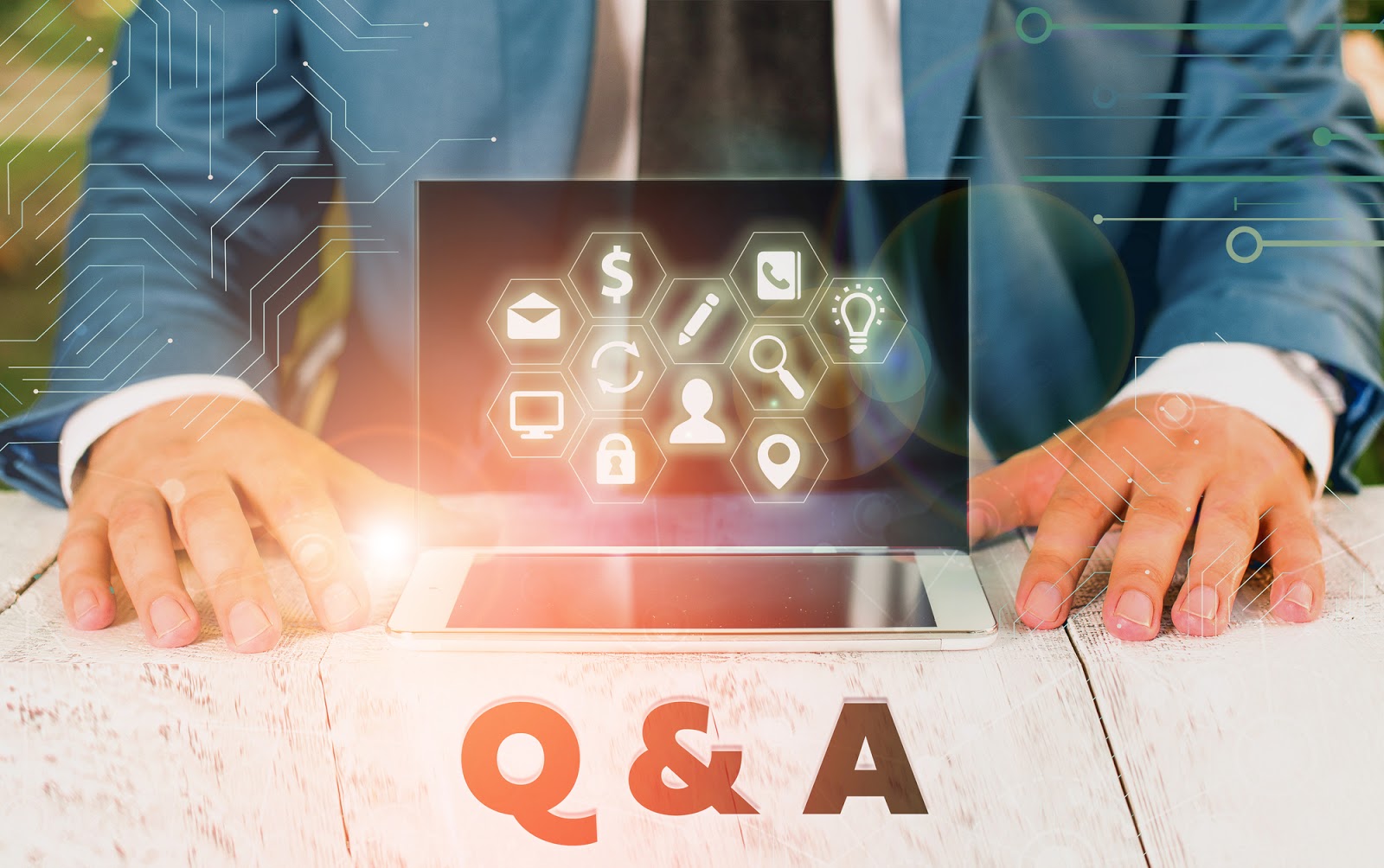
Hello, Habr! The number of services in the Huawei Mobile Services (HMS) ecosystem per year. grew from 9 to 31, and developers began to have more and more questions about the support of hybrid applications, interaction with AppGallery, the use of individual services and whales. The main platforms for our communication with the global community are Stackoverflow , Reddit , XDA-Developers and the support section on the Huawei developer portal. Especially for those who are interested in our platform, we have collected 10 questions about working with Huawei Mobile Services from these sites.
1. Will React-native and Firebase SDK work on Huawei phones without Google Service and no code changes?
Yes, the React-native app will work without modification, just submit the APK to upload to the Huawei App Gallery. The Firebase SDK is a little more complicated. The performance of your application depends on the services you are trying to include in your application. For example, logging into Google using the Firebase authentication module will not be supported on phones that do not have Google Mobile Services, such as the Huawei Mate 30 Pro.
If you want to use the same APK for both GMS and HMS, you need to check the service availability first.
For GMS:
val gmsAvailable = GooglePlayServicesUtil.getInstance().isGooglePlayServicesAvailable(mContext)
For HMS:
val hmsAvailable = HuaweiApiAvailability.getInstance().isHuaweiMobileServicesAvailable(mContext)
When trying to use Google Login, or Huawei Login, or any other services:
if gmsAvailable {
// execute GMS Code
} else if hmsAvailable {
// execute HMS Code
}
2. What are the real hidden costs of supporting an additional ecosystem?
The cost of maintaining an application in HMS depends on how you design the system and what services your application requires. On average, integration times can vary from a few hours to several weeks, depending on the application and the number of Google and Firebase services in the original application.
If your application does not have integrated GMS, then you can download it without any modifications - Facebook, Yandex and others services will work.
3. What mashups does HMS support?
Since HMS Core 5.0.0, the number of whales supported by third-party platforms has been increased:
Apache Cordova:
- Account Kit plugins for Cordova
- Ads Kit plugins for Cordova
- Analytics Kit plugin for Cordova
- In-App Purchases Kit plugin for Cordova
- Location Kit plugin for Cordova
- Map Kit plugins for Cordova
- ML Kit plugins for Cordova
- Push Kit plugin for Cordova
- Site Kit plugins for Cordova
React Native:
- Account Kit plugins for React Native
- Ads Kit plugins for React Native
- Analytics Kit plugin for React Native
- Health Kit plugin for React Native
- In-App Purchases Kit plugin for React Native
- Location Kit plugin for React Native
- Map Kit plugin for React Native
- ML Kit plugins for React Native
- Push Kit plugin for React Native
- Site Kit plugins for React Native
Xamarin:
- Account Kit plugins for Xamarin
- Ads Kit plugins for Xamarin
- Analytics Kit plugins for Xamarin
- Map Kit plugins for Xamarin
- Location Kit plugins for Xamarin
- Push Kit plugins for Xamarin
- Site Kit plugins for Xamarin
Flutter:
- Account Kit plugins for Flutter
- Ads Kit plugins for Flutter
- Analytics Kit plugins for Flutter
- In-App Purchases Kit plugin for Flutter
- Location Kit plugins for Flutter
- Map Kit plugins for Flutter
- Push Kit plugins for Flutter
- Site Kit plugins for Flutter
4. Can you read the depth sensor (TOF) data on Huawei phones?
Yes, this is possible using the AR Engine SDK . Huawei AR Engine provides real-time rendering of the scene grid, and the result includes the position of the mobile phone in space. The 3D grid of the current camera view only supports Honor V20 and P30Pro models, which can receive depth information, and the supported scan scene is static.
TOF is supported on the following devices:
- P: P30 / P30Pro / P40 / P40Pro / P40Pro +
- Mate: Mate20 / Mate20Pro / Mate20RS / Mate 20X / Mate20X (5G) / Mate30 / Mate30Pro / Mate30RS / Mate30 (5G) / Mate30Pro (5G) / Mate X / Mate XS
- Nova: Nova6 / Nova6-5G / Nova7 / Nova7Pro
- Honor: Honor V20 / Honor 20 / Honor 20Pro / Honor V30 / Honor V30Pro / Honor 30S / Honor 30 Pro / Honor 30 Pro +
- : Tablet M6
To receive data from TOF, you need to use the ARSceneMesh class using the following methods:
public ShortBuffer getSceneDepth()
// Get the depth image of current frame(optimized).
public int getSceneDepthHeight()
// Get the height of the depth image.
public int getSceneDepthWidth()
// Get the width of the depth image.
There are other options for how to calculate the depth. You can get ARFrame class object and use its methods hitTest, acquireDepthImage. The GetSceneDepth method from the ARSceneMesh class also returns the processed depth map. It is more accurate, but only works up to 2.5 meters.
5. How can I open AppGallery directly from the application?
AppGallery from the application opens in the same way as the Google Play Store. Please note that AppGallery uses its own appmarket: // scheme:
- Scheme: appmarket: //
- Package: com.huawei.appmarket
Here is a snippet from AppGallery
private void startHuaweiAppGallery() {
Intent intent = new Intent(Intent.ACTION_VIEW, Uri.parse("appmarket://details?id=" + getPackageName()));
List<ResolveInfo> otherApps = getPackageManager().queryIntentActivities(intent, 0);
boolean agFound = false;
for (ResolveInfo app : otherApps) {
if (app.activityInfo.applicationInfo.packageName.equals("com.huawei.appmarket")) {
ComponentName psComponent = new ComponentName(app.activityInfo.applicationInfo.packageName, app.activityInfo.name);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK | Intent.FLAG_ACTIVITY_RESET_TASK_IF_NEEDED | Intent.FLAG_ACTIVITY_CLEAR_TOP);
intent.setComponent(psComponent);
startActivity(intent);
agFound = true;
break;
}
}
//Optional, Or copy the Google Play Store URL here (See below)
if (!agFound) {
//Your Huawei app ID can be found in the Huawei developer console
final string HUAWEI_APP_ID = "100864605";
//ex. https://appgallery.cloud.huawei.com/marketshare/app/C100864605
intent = new Intent(Intent.ACTION_VIEW, Uri.parse("https://appgallery.cloud.huawei.com/marketshare/app/C" + HUAWEI_APP_ID));
startActivity(intent);
}
}
6. How to create Huawei Android Emulator?
Huawei provides Huawei developers with cloud debugging as a free service. If you are using the Huawei SDK, you must have a Huawei developer account. Just log into Huawei Developer Console and follow the instructions .
The cloud debugging feature is really easy to use. It allows remote debugging on real devices. In the process, you can view device information, download and install APKs to remote devices, receive operation logs, and save the logs to your local computer for analysis.
7. How to access payload of HMS push notifications?
To access payload, you need to implement the HmsMessageService class and override the onMessageReceived method. You can access the payload from the RemoteMessage object. To access the token, override the onNewToken method.
Java code:
import android.util.Log;
import com.huawei.hms.push.HmsMessageService;
import com.huawei.hms.push.RemoteMessage;
public class HService extends HmsMessageService {
@Override
public void onMessageReceived(RemoteMessage remoteMessage) {
super.onMessageReceived(remoteMessage);
if (remoteMessage != null) {
if (!remoteMessage.getData().isEmpty()) {
Log.d("HMS", "Payload" + remoteMessage.getData());
}
if (remoteMessage.getNotification() != null) {
Log.d("HMS", "Message Notification Body: " + remoteMessage.getNotification().getBody());
}
}
}
}
Kotlin code:
override fun onMessageReceived(remoteMessage: RemoteMessage?) {
super.onMessageReceived(remoteMessage)
if (remoteMessage!!.data.isNotEmpty()) {
Log.i(TAG, "Message data payload: " + remoteMessage.data)
}
if (remoteMessage.notification != null) {
Log.i(TAG, "Message Notification Body: " + remoteMessage.notification.body)
}
}
Make sure you register your service:
<service
android:name=".service.HService"
android:enabled="true"
android:exported="true"
android:permission="${applicationId}.permission.PROCESS_PUSH_MSG"
android:process=":HmsMessageService">
<intent-filter>
<action android:name="com.huawei.push.action.MESSAGING_EVENT" />
</intent-filter>
</service>
8. What tools to use when developing Android application for Huawei mobile phone?
For application development, you can use both Android Studio and other IDEs such as Eclipse, Intelliji IDEA. If you already have an application that uses GMS, use the HMS Toolkit to convert your code that works with GMS to work with HMS. It should be borne in mind that the HMS Toolkit does not support the conversion of all services, and before using it, it is better to clarify which services it can transfer.
9. How to initialize HMS services without agconnect-services.json?
So far HMS does not provide a single code-based initialization solution. Initialization without a json file is possible when working with the following services:
- Push Kit:
<meta-data
android:name="com.huawei.hms.client.appid"
<!-- Replace value xxx with the actual appid.-->
android:value="appid=xxx">
</meta-data>
- Map Kit:
MapsInitializer.setApiKey("Your API Key");
- Site Kit οΌ
SearchService searchService = SearchServiceFactory.create(this, "API key");
- ML Kit:
MLApplication.getInstance().setApiKey("your ApiKey");
10. What can a product management system (PMS) do in HMS In-App Purchase?
The Product Management System (PMS) API allows you to create and manage product information. Through it you can:
- Create products: including subscriptions with automatic renewal.
- Query product information: For example, you can query for a specific product based on the App ID and Product ID, or query for all products that match your specified criteria.
- Update product information: product name, language, price and status. You can work with one or several products at the same time.
- Promote Products: The API allows you to categorize advertising campaigns by region, set promotion times and set prices.
That's all for now, if you have any questions about working with HMS, ask them in the comments.