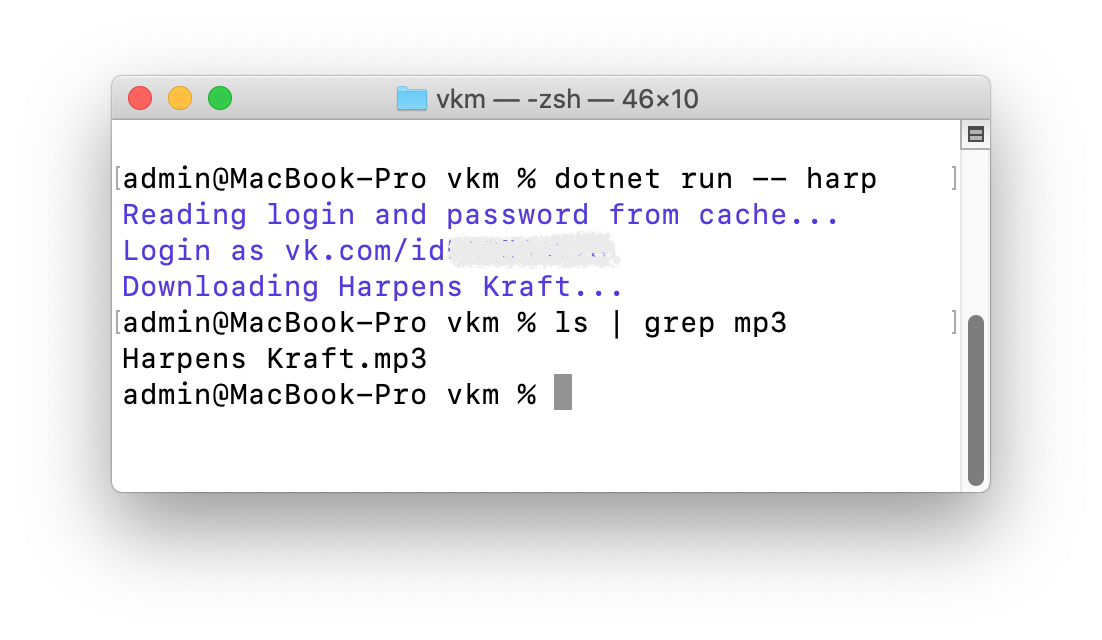
In 2020, we use different music services, but as a relic of a bygone era, in a forgotten VK profile, many have music. There is no download function, but what if you desperately need to save the audio recording?
Since such software was not found in the public domain, except for a couple of web services requiring authorization via VK (which is not very safe), under the cut we will consider the process of creating a self-hosted utility in modern C # for uploading your audio, which does not merge profile data to third parties. services.
One of the values โโof a programmer's work is simplicity and, if possible, conciseness of the code. Therefore, we will glue several existing libraries to get the desired solution.
The utility will work like this:
dotnet vkm [login] [password] [audio-lemma]
First of all, let's create a repository and describe the project dependencies in one csproj file
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<!-- -->
<OutputType>Exe</OutputType>
<TargetFramework>netcoreapp3.1</TargetFramework>
<!-- null , NRE -->
<Nullable>enable</Nullable>
<TreatWarningsAsErrors>true</TreatWarningsAsErrors>
<!-- C# 9 top-level -->
<LangVersion>9</LangVersion>
</PropertyGroup>
<ItemGroup>
<!-- VK API -->
<PackageReference Include="VkNet" Version="1.56.0" />
<!-- , -->
<PackageReference Include="VkNet.AudioBypassService" Version="1.7.0" />
</ItemGroup>
</Project>
After that, with a clear conscience, you can start writing code. We will need authorization of the utility in VK with full access to our profile. And as we can see, thanks to the .NET ecosystem, it's incredibly easy to do this:
static class Vk
{
internal static VkApi LoginToVkApi(string login, string password)
{
// ,
var api = new VkApi(new ServiceCollection().AddAudioBypass());
api.Authorize(new ApiAuthParams
{
ApplicationId = 1980660,
Login = login,
Password = password,
Settings = All
});
$"Login as vk.com/id{api.UserId}".Println(DarkBlue);
return api;
}
}
Let's describe the entry point and filter of the loaded audio recordings. We use top-level programs for this and validate the arguments right in the Application.cs file, while initializing the api
var vk = args.Length switch
{
3 => LoginToVkApi(args[0], args[1]),
_ => throw new ArgumentException("Invalid arguments. Usage:\n" +
" dotnet vkm [login] [password] [audio]\n" +
)
};
Bringing the lemma to search for an audio recording to upper-case
var lemma = args.Last().ToUpperInvariant();
And we use Linq for all audio recordings with its entry. Special thanks to habrauserSuperHackerVkfor a way to get an mp3-link with a regular .
var audios = vk.Audio.Get(new AudioGetParams { Count = 6000 })
.Where(x => x.Title.ToUpperInvariant().Contains(lemma))
.Select(x => (x.Title, Url: Regex.Replace(
x.Url.ToString(),
@"/[a-zA-Z\d]{6,}(/.*?[a-zA-Z\d]+?)/index.m3u8()",
@"$1$2.mp3"
)));
Finally, all that remains is to upload your found audio:
using var http = new HttpClient();
foreach (var (title, url) in audios)
{
$"Downloading {title}...".Println(DarkBlue);
await WriteAllBytesAsync($"{title}.mp3", await http.GetByteArrayAsync(url));
}
That's all! The utility is written and ready for personal use. It's noticeable how C # is turning more and more into a good multitool every year that allows you to solve any range of tasks. Enhancements to syntactic capabilities that seem to clutter the language when announced, in practice, on the contrary, allow you to shorten the code and make it simple and understandable.
GitHub repository with minor additions and launch documentation .
Have a great day everyone!