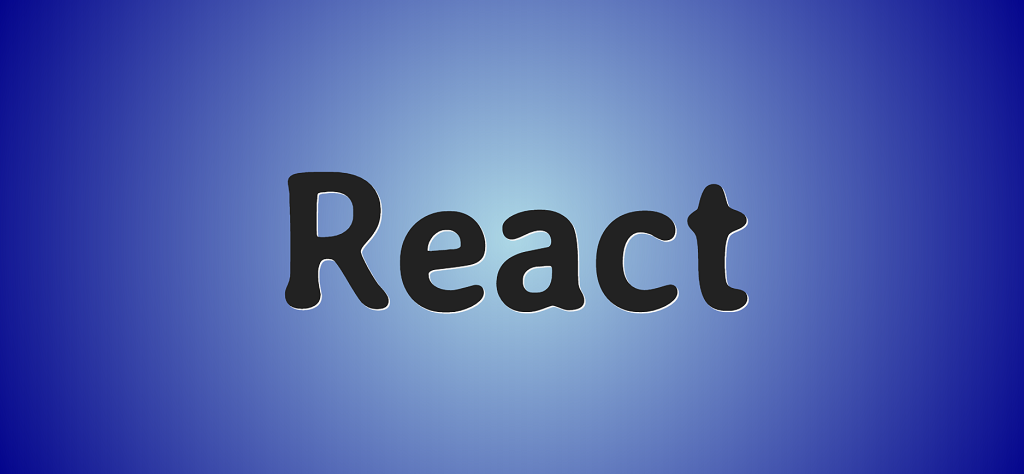
Good day, friends!
I present to your attention the top ten custom hooks .
Table of contents
- useMemoCompare
- useAsync
- useRequireAuth
- useRouter
- useAuth
- useEventListener
- useWhyDidYouUpdate
- useDarkMode
- useMedia
- useLocalStorage
useMemoCompare
This hook is similar to useMemo, but instead of an array of dependencies, it is passed a function that compares the previous and new values. A function can compare nested properties, call methods on objects, or do something else for comparison purposes. If the function returns true, the hook returns a reference to the old object. It should be noted that this hook, unlike useMemo, does not imply the absence of repeated complex calculations. He needs to pass the calculated value for comparison. This can come in handy when you want to share the library with other developers and don't want to force them to remember the object before submitting. If an object is created in the body of a component (in the case where it depends on props), then it will be new on every render. If the object is a dependency of useEffect then the effect will be triggered on every render,which can lead to problems, up to an endless loop. This hook allows you to avoid this development of events by using the old object reference instead of the new one if the function recognized the objects as the same.
import React, { useState, useEffect, useRef } from "react";
//
function MyComponent({ obj }) {
const [state, setState] = useState();
// , "id"
const objFinal = useMemoCompare(obj, (prev, next) => {
return prev && prev.id === next.id;
});
// objFinal
// obj , , obj
// ,
// , ,
// -> -> -> ..
useEffect(() => {
//
return objFinal.someMethod().then((value) => setState(value));
}, [objFinal]);
// [obj.id] ?
useEffect(() => {
// eslint-plugin-hooks , obj
// eslint-disable-next-line
//
return obj.someMethod().then((value) => setState(value));
}, [obj.id]);
}
//
function useMemoCompare(next, compare) {
// ref
const prevRef = useRef();
const prev = prevRef.current;
//
//
const isEqual = compare(prev, next);
// , prevRef
//
// , true,
useEffect(() => {
if (!isEqual) {
prevRef.current = next;
}
});
// ,
return isEqual ? prev : next;
}
useAsync
It is good practice to display the status of an asynchronous request. An example
would be fetching data from an API and displaying a loading indicator before rendering the results. Another example is disabling the button while the form is being submitted and then displaying the result. Instead of polluting the component with lots of useState calls to track the state of the asynchronous function, we can use this hook, which takes an asynchronous function and returns value, error, and status as needed to update the user interface. Possible values ββfor the "status" property are "idle", "pending", "success", and "error". Our hook allows you to execute a function immediately or late using the execute function.
import React, { useState, useEffect, useCallback } from 'react'
//
function App() {
const {execute, status, value, error } = useAsync(myFunction, false)
return (
<div>
{status === 'idle' && <div> </div>}
{status === 'success' && <div>{value}</div>}
{status === 'error' && <div>{error}</div>}
<button onClick={execute} disabled={status === 'pending'}>
{status !== 'pending' ? ' ' : '...'}
</button>
</div>
)
}
//
// 50%
const myFunction = () => {
return new Promise((resolve, reject) => {
setTimeout(() => {
const random = Math.random() * 10
random <=5
? resolve(' ')
: reject(' ')
}, 2000)
})
}
//
const useAsync = (asyncFunction, immediate = true) => {
const [status, setStatus] = useState('idle')
const [value, setValue] = useState(null)
const [error, setError] = useState(null)
// "execute" asyncFunction
// pending, value error
// useCallback useEffect
// useEffect asyncFunction
const execute = useCallback(() => {
setStatus('pending')
setValue(null)
setError(null)
return asyncFunction()
.then(response => {
setValue(response)
setStatus('success')
})
.catch(error => {
setError(error)
setStatus('error')
})
}, [asyncFunction])
// execute
// , execute
// ,
useEffect(() => {
if (immediate) {
execute()
}
}, [execute, immediate])
return { execute, status, value, error }
}
useRequireAuth
The purpose of this hook is to redirect the user to the login page when logging out of the account. Our hook is a composition of the "useAuth" and "useRouter" hooks. Of course, we can implement the necessary functionality in the "useAuth" hook, but then we have to include it with the routing scheme. With composition, we can keep useAuth and useRouter simple by implementing a redirect with a custom hook.
import Dashboard from "./Dahsboard.js";
import Loading from "./Loading.js";
import { useRequireAuth } from "./use-require-auth.js";
function DashboardPage(props) {
const auth = useRequireAuth();
// auth null ( )
// false ( )
//
if (!auth) {
return <Loading />;
}
return <Dashboard auth={auth} />;
}
// (use-require-auth.js)
import { useEffect } from "react";
import { useAuth } from "./use-auth.js";
import { useRouter } from "./use-router.js";
function useRequireAuth(redirectUrl = "./signup") {
const auth = useAuth();
const router = useRouter();
// auth.user false,
// , ,
useEffect(() => {
if (auth.user === false) {
router.push(redirectUrl);
}
}, [auth, router]);
return auth;
}
useRouter
If you are using React Router in your work, you may have noticed that several useful hooks have recently appeared, such as "useParams", "useLocation", "useHistory" and "useRouterMatch". Let's try to wrap them up in a single hook that returns the data and methods we need. We'll show you how to combine multiple hooks and return a single object containing their states. For libraries like React Router, it makes sense to provide a selection of the desired hook. This avoids unnecessary rendering. But sometimes we need all or most of the named hooks.
import { useMemo } from "react";
import {
useParams,
useLocation,
useHistory,
useRouterMatch,
} from "react-router-dom";
import queryString from "query-string";
//
function MyComponent() {
//
const router = useRouter();
// (?postId=123) (/:postId)
console.log(router.query.postId);
//
console.log(router.pathname);
// router.push()
return <button onClick={(e) => router.push("./about")}>About</button>;
}
//
export function useRouter() {
const params = useParams();
const location = useLocation();
const history = useHistory();
const match = useRouterMatch();
//
// ,
return useMemo(() => {
return {
// push(), replace() pathname
push: history.push,
replace: history.replace,
pathname: location.pathname,
// "query"
// ,
// : /:topic?sort=popular -> { topic: 'react', sort: 'popular' }
query: {
...queryString.parse(location.search), //
...params,
},
// "match", "location" "history"
// React Router
match,
location,
history,
};
}, [params, match, location, history]);
}
useAuth
It is common to have several components that are rendered depending on whether the user is logged into an account. Some of these components call authentication methods like signin, signout, sendPasswordResetEmail, etc. The "useAuth" hook is perfect for this, as it ensures that the component receives the authentication state and redraws the component when changes are present. Instead of instantiating useAuth for each user, our hook calls useContext to get data from the parent component. The real magic happens in the ProvideAuth component, where all authentication methods (in the example we are using Firebase) are wrapped in a useProvideAuth hook. The context is then used to pass the current authentication object to the child components calling useAuth.This will make more sense after reading the example. Another reason I like this hook is because it abstracts the real authentication provider (Firebase), which makes it easier to make changes.
// App
import React from "react";
import { ProvideAuth } from "./use-auth.js";
function App(props) {
return (
<ProvideAuth>
{/*
,
Next.js, : /pages/_app.js
*/}
</ProvideAuth>
);
}
// ,
import React from "react";
import { useAuth } from "./use-auth.js";
function NavBar(props) {
// auth
const auth = useAuth();
return (
<NavbarContainer>
<Logo />
<Menu>
<Link to="/about">About</Link>
<Link to="/contact">Contact</Link>
{auth.user ? (
<Fragment>
<Link to="/account">Account ({auth.user.email})</Link>
<Button onClick={() => auth.signout()}>Signout</Button>
</Fragment>
) : (
<Link to="/signin">Signin</Link>
)}
</Menu>
</NavbarContainer>
);
}
// (use-auth.js)
import React, { useState, useEffect, useContext, createContext } from "react";
import * as firebase from "firebase/app";
import "firebase/auth";
// Firebase
firebase.initializeApp({
apiKey: "",
authDomain: "",
projectId: "",
appID: "",
});
const authContext = createContext();
// Provider, "auth"
// , useAuth
export const useAuth = () => {
return useContext(authContext);
};
// "auth"
//
export const useAuth = () => {
return useContext(authContext);
};
// , "auth"
function useProviderAuth() {
const [user, setUser] = useState(null);
// Firebase,
//
const signin = (email, password) => {
return firebase
.auth()
.signInWithEmailAndPassword(email, password)
.then((response) => {
setUser(response.user);
return response.user;
});
};
const signup = (email, password) => {
return firebase
.auth()
.createUserWithEmailAndPassword(email, password)
.then((response) => {
setUser(response.user);
return response.user;
});
};
const signout = () => {
return firebase
.auth()
.signOut()
.then(() => {
setUser(false);
});
};
const sendPasswordResetEmail = (email) => {
return firebase
.auth()
.sendPasswordResetEmail(email)
.then(() => true);
};
const confirmPasswordReset = (code, password) => {
return firebase
.auth()
.confirmPasswordReset(code, password)
.then(() => true);
};
//
//
// ,
// "auth"
useEffect(() => {
const unsubscribe = firebase.auth().onAuthStateChange((user) => {
if (user) {
setUser(user);
} else {
setUser(false);
}
});
//
return () => unsubscribe();
}, []);
// "user"
return {
user,
signin,
signup,
signout,
sendPasswordResetEmail,
confirmPasswordReset,
};
}
useEventListener
If you have to deal with a large number of event handlers that register with useEffect, you might want to separate them into separate hooks. In the example below, we create a useEventListener hook that checks for addEventListener support, adds handlers and removes them on exit.
import { useState, useRef, useEffect, useCallback } from "react";
//
function App() {
//
const [coords, setCoords] = useState({ x: 0, y: 0 });
// useCallback,
//
const handler = useCallback(
({ clientX, clientY }) => {
//
setCoords({ x: clientX, y: clientY });
},
[setCoords]
);
//
useEventListener("mousemove", handler);
return <h1> : ({(coords.x, coords.y)})</h1>;
}
//
function useEventListener(eventName, handler, element = window) {
// ,
const saveHandler = useRef();
// ref.current
//
//
//
useEffect(() => {
saveHandler.current = handler;
}, [handler]);
useEffect(
() => {
// addEventListener
const isSupported = element && element.addEventListener;
if (!isSupported) return;
// , , ref
const eventListener = (event) => saveHandler.current(event);
//
element.addEventListener(eventName, eventListener);
//
return () => {
element.removeEventListener(eventName, eventListener);
};
},
[eventName, element] //
);
}
useWhyDidYouUpdate
This hook allows you to determine which props changes lead to re-rendering. If the function is βcomplexβ and you are sure that it is clean, i.e. returns the same results for the same props, you can use the higher order component "React.memo" as we do in the example below. If after that unnecessary renders have not stopped, you can use useWhyDidYouUpdate, which outputs to the console the props that change during rendering, indicating the previous and current values.
import { useState, useEffect, useRef } from "react";
// , <Counter>
// React.memo,
// useWhyDidYouUpdate
const Counter = React.memo((props) => {
useWhyDidYouUpdate("Counter", props);
return <div style={props.style}>{props.count}</div>;
});
function App() {
const [count, setCount] = useState(0);
const [userId, setUserId] = useState(0);
// , , <Counter>
// , userId
// "switch user". ,
//
// ,
//
const counterStyle = {
fontSize: "3rem",
color: "red",
};
}
return (
<div>
<div className="counter">
<Counter count={count} style={counterStyle} />
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
<div className="user">
<img src={`http://i.pravatar.cc/80?img=${userId}`} />
<button onClick={() => setUserId(userId + 1)}>Switch User</button>
</div>
</div>
);
//
function useWhyDidYouUpdate(name, props) {
// "ref"
//
const prevProps = useRef();
useEffect(() => {
if (prevProps.current) {
//
const allKeys = Object.keys({ ...prevProps.current, ...props });
//
const changesObj = {};
//
allKeys.forEach((key) => {
//
if (prevProps.current[key] !== props[key]) {
// changesObj
changesObj[key] = {
from: prevProps.current[key],
to: props[key],
};
}
});
// changesObj - ,
if (object.keys(changesObj).length) {
console.log("why-did-you-update", name, changesObj);
}
}
// , prevProps
prevProps.current = props;
});
}
useDarkMode
This hook implements the logic for switching the site's color scheme (light and dark). It uses local storage to store the user-selected scheme, the default mode set in the browser using the "prefers-color-scheme" media query. To enable dark mode, use the "dark-mode" class of the "body" element. Hook also demonstrates the power of composition. State synchronization with localStorage is implemented using the "useLocalStorage" hook, and defining the user's preferred schema using the "useMedia" hook, which are designed for different purposes. However, composing these hooks results in an even more powerful hook of just a few lines of code. This is almost the same as the "compositional" power of hooks in relation to component state.
function App() {
const [darkMode, setDarkMode] = useDarkMode();
return (
<div>
<div className="navbar">
<Toggle darkMode={darkMode} setDarkMode={setDarkMode} />
</div>
<Content />
</div>
);
}
//
function useDarkMode() {
// "useLocalStorage"
const [enabledState, setEnableState] = useLocalStorage("dark-mode-enabled");
//
// "usePrefersDarkMode" "useMedia"
const prefersDarkMode = usePrefersDarkMode();
// enabledState , , , prefersDarkMode
const enabled =
typeof enabledState !== "undefined" ? enabledState : prefersDarkMode;
// /
useEffect(
() => {
const className = "dark-mode";
const element = window.document.body;
if (enabled) {
element.classList.add(className);
} else {
element.classList.remove(className);
}
},
[enabled] // enabled
);
//
return [enabled, setEnableState];
}
// "useMedia"
// , ,
// -
//
function usePrefersDarkMode() {
return useMedia(["(prefers-color-scheme: dark)"], [true], false);
}
useMedia
This hook encapsulates the logic for defining media queries. In the example below, we render a different number of columns depending on the media query based on the current screen width and then place an image above the columns so that it levels out the difference in column heights (we don't want one column to be taller than the other) ... You can create a hook that directly determines the width of the screen, however our hook allows you to combine media queries specified in JS and a stylesheet.
import { useState, useEffect } from "react";
function App() {
const columnCount = useMedia(
// -
["(min-width: 1500px)", "(min-width: 1000px)", "(min-width: 600px)"],
//
[5, 4, 3],
//
2
);
// ( 0)
let columnHeight = new Array(columnCount).fill(0);
// ,
let columns = new Array(columnCount).fill().map(() => []);
data.forEach((item) => {
//
const shortColumntIndex = columnHeight.indexOf(Math.min(...columnHeight));
//
columns[shortColumntIndex].push(item);
//
columnHeight[shortColumntIndex] += item.height;
});
//
return (
<div className="App">
<div className="columns is-mobile">
{columns.map((column) => (
<div className="column">
{column.map((item) => (
<div
className="image-container"
style={{
// aspect ratio
paddingTop: (item.height / item.width) * 100 + "%",
}}
>
<img src={item.image} alt="" />
</div>
))}
</div>
))}
</div>
</div>
);
}
//
function useMedia(queries, values, defaultValue) {
// -
const mediaQueryList = queries.map((q) => window.matchMedia(q));
//
const getValue = () => {
//
const index = mediaQueryList.findIndex((mql) => mql.matches);
//
return typeof values[index] !== "undefined"
? values[index]
: defaultValue;
};
//
const [value, setValue] = useState(getValue);
useEffect(
() => {
//
// : getValue useEffect,
//
//
const handler = () => setValue(getValue);
// -
mediaQueryList.forEach((mql) => mql.addEventListener(handler));
//
return () =>
mediaQueryList.forEach((mql) => mql.removeEventListener(handler));
},
[] //
);
return value;
}
useLocalStorage
This hook is designed to synchronize state with local storage to persist state across page reloads. Using this hook is similar to using useState, except that we pass the local storage key as the default on page load, instead of defining an initial value.
import { useState } from "react";
//
function App() {
// useState, ,
const [name, setName] = useLocalStorage("name", "Igor");
return (
<div>
<input
type="text"
placeholder="Enter your name"
value={name}
onChange={(e) => setName(e.target.value)}
/>
</div>
);
}
//
function useLocalStorage(key, initialValue) {
//
// useState
const [storedValue, setStoredValue] = useState(() => {
try {
//
const item = window.localStorage.getItem(key);
// initialValue
return item ? JSON.parse(item) : initialValue;
} catch (error) {
// ,
console.error(error);
return initialValue;
}
});
// useState,
//
const setValue = (value) => {
try {
//
const valueToStore =
value instanceof Function ? value(storedValue) : value;
//
setStoredValue(valueToStore);
//
window.localStorage.setItem(key, JSON.stringify(valueToStore));
} catch (error) {
//
console.error(error);
}
};
return [storedValue, setValue];
}
That's all for today. Hope you found something helpful. Thank you for attention.