Containers have become the preferred means of packaging an application with all its software and operating system dependencies, and then delivering them to different environments.
This article explores the different ways to containerize your Spring Boot application:
building a Docker image using a Dockerfile,
building an OCI image from source using Cloud-Native Buildpack,
and optimizing the image at runtime by splitting the JARs into different tiers using layered tools.
Sample code
This article is accompanied by a sample working code on GitHub .
Container terminology
We'll start with the container terminology used in the article:
Container image : a file of a specific format. We convert our application to a container image by running the build tool.
Container : An executable instance of a container image.
Container engine : A daemon process responsible for starting a container.
Container host : The host machine that runs the container engine.
(Container registry): , .
OCI: Open Container Initiative (OCI) - , Linux Foundation. OCI , , , .
, . , .
2.3 Spring Boot OCI.
Docker - , Docker , Docker.
Docker Spring Boot , Docker.
JAR , Docker, JAR JRE .
Spring Spring Initializr web
, lombok
actuator
. rest , API GET
.
Docker
, Dockerfile
:
FROM adoptopenjdk:11-jre-hotspot
ARG JAR_FILE=target/*.jar
COPY ${JAR_FILE} application.jar
EXPOSE 8080
ENTRYPOINT ["java","-jar","/application.jar"]
Docker , adoptopenjdk
, JAR, , 8080
.
Maven Gradle. Maven:
mvn clean package
JAR- . JAR Docker Docker.
JAR Docker, docker build
, Docker, :
docker build -t usersignup:v1 .
:
docker images
usersignup
, adoptopenjdk
, Docker.
REPOSITORY TAG SIZE
usersignup v1 249MB
adoptopenjdk 11-jre-hotspot 229MB
. dive, :
dive usersignup:v1
Dive:

, . .
Buildpack
(Buildpacks) - , Β« Β» (PAAS) . Heroku 2011 Cloud Foundry, Google App Engine, Gitlab, Knative .
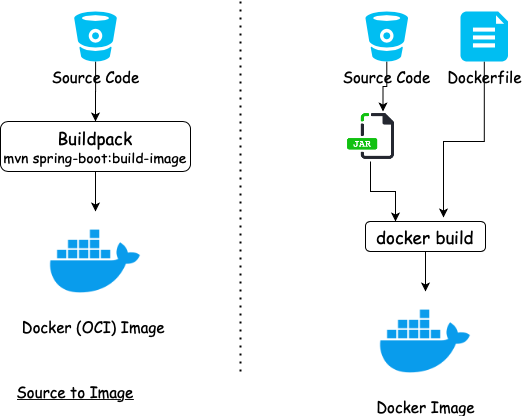
Buildpack , (builder) , builder.
. Cloud-Native Buildpacks , OCI, , Docker.
Spring Boot
Spring Boot OCI Buildpack. bootBuildImage
(Gradle) spring-boot:build-image
(Maven) Docker.
, Docker, image tag
:
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<image>
<name>docker.io/pratikdas/${project.artifactId}:v1</name>
</image>
</configuration>
</plugin>
Maven build-image
. Docker.
mvn spring-boot:build-image
:
[INFO] --- spring-boot-maven-plugin:2.3.3.RELEASE:build-image (default-cli) @ usersignup ---
[INFO] Building image 'docker.io/pratikdas/usersignup:v1'
[INFO]
[INFO] > Pulling builder image 'gcr.io/paketo-buildpacks/builder:base-platform-api-0.3' 0%
.
.
.. [creator] Adding label 'org.springframework.boot.version'
.. [creator] *** Images (c311fe74ec73):
.. [creator] docker.io/pratikdas/usersignup:v1
[INFO]
[INFO] Successfully built image 'docker.io/pratikdas/usersignup:v1'
, paketo Cloud-Native buildpack
OCI. , , Docker, :
docker images
:
REPOSITORY SIZE
paketobuildpacks/run 84.3MB
gcr.io/paketo-buildpacks/builder 652MB
pratikdas/usersignup 257MB
Jib
Jib - Google, .
jib-maven-plugin
pom.xml:
<plugin>
<groupId>com.google.cloud.tools</groupId>
<artifactId>jib-maven-plugin</artifactId>
<version>2.5.2</version>
</plugin>
Jib Maven, . , Docker:
mvn compile jib:build -Dimage=<docker registry name>/usersignup:v1
Maven :
[INFO] Containerizing application to pratikdas/usersignup:v1...
.
.
[INFO] Container entrypoint set to [java, -cp, /app/resources:/app/classes:/app/libs/*, io.pratik.users.UsersignupApplication]
[INFO]
[INFO] Built and pushed image as pratikdas/usersignup:v1
[INFO] Executing tasks:
[INFO] [==============================] 100.0% complete
, .
:
: , . . CI.
: .
Docker , Dockerfile. . Docker , .
Spring Boot Β« JARΒ» . JAR, , JAR. , . Spring Framework.
Spring Framework.
, JAR-, -.
, :

, Spring Boot.
Spring Boot Buildpack
Spring Boot 2.3 JAR- . , Spring Boot Maven:
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<layers>
<enabled>true</enabled>
</layers>
</configuration>
</plugin>
Buildpack, Docker .
build-image
Maven :
mvn spring-boot:build-image
Dive, , , ( ) , JAR:

Spring Boot Docker
Maven Gradle JAR Docker Docker.
Docker, .
JAR Maven :
META-INF/
.
BOOT-INF/lib/
.
BOOT-INF/lib/spring-boot-jarmode-layertools-2.3.3.RELEASE.jar
BOOT-INF/classpath.idx
BOOT-INF/layers.idx
JAR spring-boot-jarmode-layertools
layersfle.idx
. JAR- , .
JAR, -Djarmode=layertools
spring-boot-jarmode-layertools
JAR :
java -Djarmode=layertools -jar target/usersignup-0.0.1-SNAPSHOT.jar
, :
Usage:
java -Djarmode=layertools -jar usersignup-0.0.1-SNAPSHOT.jar
Available commands:
list List layers from the jar that can be extracted
extract Extracts layers from the jar for image creation
help Help about any command
list
, extract
help
help
. list
:
java -Djarmode=layertools -jar target/usersignup-0.0.1-SNAPSHOT.jar list
dependencies
spring-boot-loader
snapshot-dependencies
application
, .
:
| |
---|---|
| , SNAPSHOT |
| JAR |
| , SNAPSHOT |
|
|
layers.idx
, Docker. , . , - .
,
Docker :
# the first stage of our build will extract the layers
FROM adoptopenjdk:14-jre-hotspot as builder
WORKDIR application
ARG JAR_FILE=target/*.jar
COPY ${JAR_FILE} application.jar
RUN java -Djarmode=layertools -jar application.jar extract
# the second stage of our build will copy the extracted layers
FROM adoptopenjdk:14-jre-hotspot
WORKDIR application
COPY --from=builder application/dependencies/ ./
COPY --from=builder application/spring-boot-loader/ ./
COPY --from=builder application/snapshot-dependencies/ ./
COPY --from=builder application/application/ ./
ENTRYPOINT ["java", "org.springframework.boot.loader.JarLauncher"]
- Dockerfile2
.
Docker :
docker build -f Dockerfile2 -t usersignup:v1 .
:
Sending build context to Docker daemon 20.41MB
Step 1/12 : FROM adoptopenjdk:14-jre-hotspot as builder
14-jre-hotspot: Pulling from library/adoptopenjdk
.
.
Successfully built a9ebf6970841
Successfully tagged userssignup:v1
, Docker , .
, Dive, , Docker. Dive:
dive userssignup:v1
, , , 11 , .

, , , yml
layers.idx
:
- "dependencies":
- "BOOT-INF/lib/"
- "spring-boot-loader":
- "org/"
- "snapshot-dependencies":
- "custom-dependencies":
- "io/myorg/"
- "application":
- "BOOT-INF/classes/"
- "BOOT-INF/classpath.idx"
- "BOOT-INF/layers.idx"
- "META-INF/"
layers.idx
, io.myorg
, .
Cloud-Native Buildpacks . Docker : JAR, , Docker.
, , , , .
, Github .
, .
:
docker system prune -a
Docker:
docker build -f <Docker file name> -t <tag> .
( Dockerfile):
mvn spring-boot:build-image
. JAR- , spring-boot-maven-plugin:
java -Djarmode=layertools -jar application.jar list
. JAR- , spring-boot-maven-plugin:
java -Djarmode=layertools -jar application.jar extract
docker images
(, ):
dive <image ID or image tag>