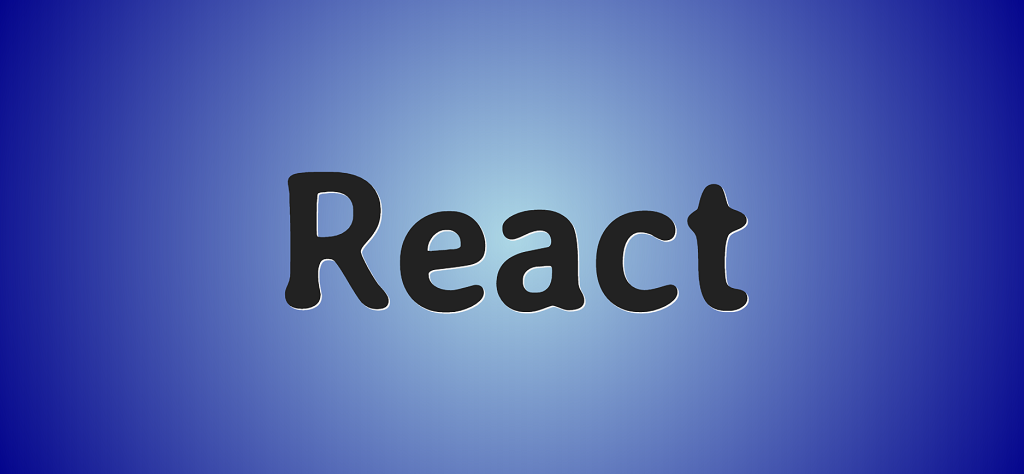
Good day, friends!
Here's a guide to the main React hooks: useState, useEffect, useLayoutEffect, useContext, useReducer, useCallback, useMemo, and UseRef.
Inspiration: React Hooks cheat sheet: Unlock solutions to common problems .
The purpose of this guide is to provide a brief overview of the purpose and capabilities of each hook. After the description of the hook, there is an example code for its use and a sandbox for your experiments.
The full set of hooks is available in this repository .
- Downloading the repository
- Install dependencies: npm i
- Run: npm start
Hooks are located in the "hooks" directory. The main file is index.js. To execute a specific hook, you need to uncomment the corresponding import and render lines.
Without further preface.
useState
useState allows you to work with the state of variables inside a functional component.
Variable state
To determine the state of the variable, call useState with the initial state as an argument: useState (initialValue).
const DeclareState = () => {
const [count] = useState(1);
return <div> - {count}.</div>;
};
Updating the state of a variable
To update the state of a variable, call the update function returned by useState: const [state, updater] = useState (initialValue).
The code:
const UpdateState = () => {
const [age, setAge] = useState(19);
const handleClick = () => setAge(age + 1);
return (
<>
<p> {age} .</p>
<button onClick={handleClick}> !</button>
</>
);
};
Sandbox:
Multiple variable states
In one functional component, you can define and update the states of several variables.
The code:
const MultStates = () => {
const [age, setAge] = useState(19);
const [num, setNum] = useState(1);
const handleAge = () => setAge(age + 1);
const handleNum = () => setNum(num + 1);
return (
<>
<p> {age} .</p>
<p> {num} .</p>
<button onClick={handleAge}> !</button>
<button onClick={handleNum}> !</button>
</>
);
};
Sandbox:
Using an object to determine the state of a variable
In addition to strings and numbers, objects can be used as an initial value. Note that useStateUpdater needs to be passed the entire object because it is being replaced rather than merged with the previous one.
// setState ( ) - useState ( )
// , - {name: "Igor"}
setState({ age: 30 });
//
// {name: "Igor", age: 30} -
useStateUpdater({ age: 30 });
//
// {age: 30} -
The code:
const StateObject = () => {
const [state, setState] = useState({ age: 19, num: 1 });
const handleClick = (val) =>
setState({
...state,
[val]: state[val] + 1,
});
const { age, num } = state;
return (
<>
<p> {age} .</p>
<p> {num} .</p>
<button onClick={() => handleClick('age')}> !</button>
<button onClick={() => handleClick('num')}> !</button>
</>
);
Sandbox:
Initializing the state of a variable using a function
The initial value of the state of a variable can be determined by a function.
const StateFun = () => {
const [token] = useState(() => {
const token = localStorage.getItem("token");
return token || "default-token";
});
return <div> - {token}</div>;
};
Function instead of setState
The update function returned by useState can be more than just setState.
const [value, updateValue] = useState(0);
// , ,
updateValue(1);
updateValue((prevVal) => prevVal + 1);
The second method is suitable for cases where the update depends on the previous state.
The code:
const CounterState = () => {
const [count, setCount] = useState(0);
return (
<>
<p> {count}.</p>
<button onClick={() => setCount(0)}></button>
<button onClick={() => setCount((prevVal) => prevVal + 1)}>
(+)
</button>
<button onClick={() => setCount((prevVal) => prevVal - 1)}>
(-)
</button>
</>
);
};
Sandbox:
useEffect
useEffect accepts a function responsible for additional (side) effects.
Basic use
The code:
const BasicEffect = () => {
const [age, setAge] = useState(19);
const handleClick = () => setAge(age + 1);
useEffect(() => {
document.title = ` ${age} !`;
});
return (
<>
<p> .</p>
<button onClick={handleClick}> !</button>
</>
);
};
Sandbox:
Removing (canceling) an effect
A common practice is to remove the effect after a while. This can be done using the function returned by the effect passed to useEffect. Below is an example with addEventListener.
The code:
const CleanupEffect = () => {
useEffect(() => {
const clicked = () => console.log("!");
window.addEventListener("click", clicked);
return () => {
window.removeEventListener("click", clicked);
};
}, []);
return (
<>
<p> .</p>
</>
);
};
Sandbox:
Multiple effects
Multiple useEffects can be used in a functional component.
The code:
const MultEffects = () => {
//
useEffect(() => {
const clicked = () => console.log("!");
window.addEventListener("click", clicked);
return () => {
window.removeEventListener("click", clicked);
};
}, []);
//
useEffect(() => {
console.log(" .");
});
return (
<>
<p> .</p>
</>
);
};
Sandbox:
Note that the call to useEffect on re-rendering can be skipped by passing it an empty array as the second argument.
Effect dependencies
The code:
const EffectDependency = () => {
const [randomInt, setRandomInt] = useState(0);
const [effectLogs, setEffectLogs] = useState([]);
const [count, setCount] = useState(1)
useEffect(() => {
setEffectLogs((prevEffectLogs) => [
...prevEffectLogs,
` ${count}.`,
]);
setCount(count + 1)
}, [randomInt]);
return (
<>
<h3>{randomInt}</h3>
<button onClick={() => setRandomInt(~~(Math.random() * 10))}>
!
</button>
<ul>
{effectLogs.map((effect, i) => (
<li key={i}>{" ".repeat(i) + effect}</li>
))}
</ul>
</>
);
};
Sandbox:
In this case, we are passing the randomInt dependency to useEffect as the second argument, so the function is called on the initial render, as well as whenever randomInt changes.
Skip effect (empty array dependency)
In the example below, useEffect is passed an empty array as a dependency, so the effect will only work on initial rendering.
The code:
const SkipEffect = () => {
const [randomInt, setRandomInt] = useState(0);
const [effectLogs, setEffectLogs] = useState([]);
const [count, setCount] = useState(1);
useEffect(() => {
setEffectLogs((prevEffectLogs) => [
...prevEffectLogs,
` ${count}.`,
]);
setCount(count + 1);
}, []);
return (
<>
<h3>{randomInt}</h3>
<button onClick={() => setRandomInt(~~(Math.random() * 10))}>
!
</button>
<ul>
{effectLogs.map((effect, i) => (
<li key={i}>{" ".repeat(i) + effect}</li>
))}
</ul>
</>
);
};
Sandbox:
When the button is clicked, useEffect is not called.
Skipping effect (no dependencies)
If there is no dependency array, the effect will be triggered every time the page is rendered.
useEffect(() => {
console.log(
" ."
);
});
useContext
useContext removes the need to rely on the context consumer. It has a simpler interface compared to MyContext.Consumer and renders props. Below is a comparison of using context using useContext and Context.Consumer.
// Context
const ThemeContext = React.createContext("dark")
//
function Button() {
return (
<ThemeContext.Consumer>
{theme => <button className={thene}> !</button>}
</ThemeContext.Consumer>
}
// useContext
import { useContext } from "react"
function ButtonHook() {
const theme = useContext(ThemeContext)
return <button className={theme}> !</button>
}
The code:
const ChangeTheme = () => {
const [mode, setMode] = useState("light");
const handleClick = () => {
setMode(mode === "light" ? "dark" : "light");
};
const ThemeContext = React.createContext(mode);
const theme = useContext(ThemeContext);
return (
<div
style={{
background: theme === "light" ? "#eee" : "#222",
color: theme === "light" ? "#222" : "#eee",
display: "grid",
placeItems: "center",
minWidth: "320px",
minHeight: "320px",
borderRadius: "4px",
}}
>
<p> : {theme}.</p>
<button onClick={handleClick}> </button>
</div>
);
};
Sandbox:
useLayoutEffect
The behavior of useLayoutEffect is similar to that of useEffect, with a few exceptions, which we'll talk about later.
useLayoutEffect(() => {
//
}, []);
Basic use
Here is an example using useEffect, but with useLayoutEffect.
The code:
const [randomInt, setRandomInt] = useState(0);
const [effectLogs, setEffectLogs] = useState([]);
const [count, setCount] = useState(1);
useLayoutEffect(() => {
setEffectLogs((prevEffectLogs) => [
...prevEffectLogs,
` ${count}.`,
]);
setCount(count + 1);
}, [randomInt]);
return (
<>
<h3>{randomInt}</h3>
<button onClick={() => setRandomInt(~~(Math.random() * 10))}>
!
</button>
<ul>
{effectLogs.map((effect, i) => (
<li key={i}>{" ".repeat(i) + effect}</li>
))}
</ul>
</>
);
};
Sandbox:
useLayoutEffect and useEffect
The function passed to useEffect is called after the page has been rendered, i.e. after the formation of the layout and rendering of the elements. This is suitable for most additional effects that should not block the flow. However, if you want to do some DOM manipulation as an additional effect, for example, useEffect is not the best choice. To prevent the user from seeing the changes, useLayoutEffect should be used. The function passed to useLayoutEffect is called before the page is rendered.
useReducer
useReducer can be used as an alternative to useState, however, its purpose is to encapsulate complex logic for working with states, when the state depends on the previous value or there are several states.
Basic use
In the example below, useReducer is used instead of useState. The useReducer call returns a state value and a dispatch function.
The code:
const initialState = { width: 30 };
const reducer = (state, action) => {
switch (action) {
case "plus":
return { width: Math.min(state.width + 30, 600) };
case "minus":
return { width: Math.max(state.width - 30, 30) };
default:
throw new Error(" ?");
}
};
const BasicReducer = () => {
const [state, dispath] = useReducer(reducer, initialState);
const color = `#${((Math.random() * 0xfff) << 0).toString(16)}`;
return (
<>
<div
style={{
margin: "0 auto",
background: color,
height: "100px",
width: state.width,
}}
></div>
<button onClick={() => dispath("plus")}>
.
</button>
<button onClick={() => dispath("minus")}>
.
</button>
</>
);
};
Sandbox:
Deferred ("lazy") state initialization
useReducer takes a third optional argument, a function that returns a state object. This function is called with initialState as the second argument.
The code:
const initializeState = () => ({
width: 90,
});
// , initializeState
const initialState = { width: 0 };
const reducer = (state, action) => {
switch (action) {
case "plus":
return { width: Math.min(state.width + 30, 600) };
case "minus":
return { width: Math.max(state.width - 30, 30) };
default:
throw new Error(" ?");
}
};
const LazyState = () => {
const [state, dispath] = useReducer(reducer, initialState, initializeState);
const color = `#${((Math.random() * 0xfff) << 0).toString(16)}`;
return (
<>
<div
style={{
margin: "0 auto",
background: color,
height: "100px",
width: state.width,
}}
></div>
<button onClick={() => dispath("plus")}>
.
</button>
<button onClick={() => dispath("minus")}>
.
</button>
</>
);
};
Sandbox:
Simulating the behavior of this.setState
useReducer uses a less strict reducer than Redux. For example, the second argument passed to a reducer doesn't need the type property. This provides us with interesting opportunities.
The code:
const initialState = { width: 30 };
const reducer = (state, newState) => ({
...state,
width: newState.width,
});
const NewState = () => {
const [state, setState] = useReducer(reducer, initialState);
const color = `#${((Math.random() * 0xfff) << 0).toString(16)}`;
return (
<>
<div
style={{
margin: "0 auto",
background: color,
height: "100px",
width: state.width,
}}
></div>
<button onClick={() => setState({ width: 300 })}>
.
</button>
<button onClick={() => setState({ width: 30 })}>
.
</button>
</>
);
};
Sandbox:
useCallback
useCallback returns the saved (cached) callback.
Starter template
The code:
const CallbackTemplate = () => {
const [age, setAge] = useState(19);
const handleClick = () => setAge(age < 100 ? age + 1 : age);
const someValue = "some value";
const doSomething = () => someValue;
return (
<>
<Age age={age} handleClick={handleClick} />
<Guide doSomething={doSomething} />
</>
);
};
const Age = ({ age, handleClick }) => {
return (
<div>
<p> {age} .</p>
<p> </p>
<button onClick={handleClick}> !</button>
</div>
);
};
const Guide = React.memo((props) => {
const color = `#${((Math.random() * 0xfff) << 0).toString(16)}`;
return (
<div style={{ background: color, padding: ".4rem" }}>
<p style={{ color: color, filter: "invert()" }}>
.
</p>
</div>
);
});
Sandbox:
In the above example, the Age component is updated and re-rendered when the button is clicked. The Guide component is also re-rendered as a new callback is passed to the doSomething props. Even though Guide uses React.memo to optimize performance, it still gets redrawn. How can we fix this?
Basic use
The code:
const BasicCallback = () => {
const [age, setAge] = useState(19);
const handleClick = () => setAge(age < 100 ? age + 1 : age);
const someValue = "some value";
const doSomething = useCallback(() => someValue, [someValue]);
return (
<>
<Age age={age} handleClick={handleClick} />
<Guide doSomething={doSomething} />
</>
);
};
const Age = ({ age, handleClick }) => {
return (
<div>
<p> {age} .</p>
<p> </p>
<button onClick={handleClick}> !</button>
</div>
);
};
const Guide = React.memo((props) => {
const color = `#${((Math.random() * 0xfff) << 0).toString(16)}`;
return (
<div style={{ background: color, padding: ".4rem" }}>
<p style={{ color: color, filter: "invert()" }}>
.
</p>
</div>
);
});
Sandbox:
Built-in useCallback
useCallback can be used as a built-in function.
The code:
const InlineCallback = () => {
const [age, setAge] = useState(19);
const handleClick = () => setAge(age < 100 ? age + 1 : age);
const someValue = "some value";
return (
<>
<Age age={age} handleClick={handleClick} />
<Guide doSomething={useCallback(() => someValue, [someValue])} />
</>
);
};
const Age = ({ age, handleClick }) => {
return (
<div>
<p> {age} .</p>
<p> </p>
<button onClick={handleClick}> !</button>
</div>
);
};
const Guide = React.memo((props) => {
const color = `#${((Math.random() * 0xfff) << 0).toString(16)}`;
return (
<div style={{ background: color, padding: ".4rem" }}>
<p style={{ color: color, filter: "invert()" }}>
.
</p>
</div>
);
});
Sandbox:
useMemo
useMemo returns the stored (cached) value.
Starter template
The code:
const MemoTemplate = () => {
const [age, setAge] = useState(19);
const handleClick = () => setAge(age < 100 ? age + 1 : age);
const someValue = { value: "some value" };
const doSomething = () => someValue;
return (
<>
<Age age={age} handleClick={handleClick} />
<Guide doSomething={doSomething} />
</>
);
};
const Age = ({ age, handleClick }) => {
return (
<div>
<p> {age} .</p>
<p> </p>
<button onClick={handleClick}> !</button>
</div>
);
};
const Guide = React.memo((props) => {
const color = `#${((Math.random() * 0xfff) << 0).toString(16)}`;
return (
<div style={{ background: color, padding: ".4rem" }}>
<p style={{ color: color, filter: "invert()" }}>
.
</p>
</div>
);
});
Sandbox:
This template is identical to the starting useCallback template, except that someValue is an object, not a string. The Guide component is also re-rendered despite using React.memo.
But why is this happening? After all, objects are compared by reference, and the reference to someValue changes with each rendering. Any ideas?
Basic use
The value returned by doSomething can be stored using useMemo. This will prevent unnecessary rendering.
The code:
const BasicMemo = () => {
const [age, setAge] = useState(19);
const handleClick = () => setAge(age < 100 ? age + 1 : age);
const someValue = () => ({ value: "some value" });
const doSomething = useMemo(() => someValue, []);
return (
<>
<Age age={age} handleClick={handleClick} />
<Guide doSomething={doSomething} />
</>
);
};
const Age = ({ age, handleClick }) => {
return (
<div>
<p> {age} .</p>
<p> </p>
<button onClick={handleClick}> !</button>
</div>
);
};
const Guide = React.memo((props) => {
const color = `#${((Math.random() * 0xfff) << 0).toString(16)}`;
return (
<div style={{ background: color, padding: ".4rem" }}>
<p style={{ color: color, filter: "invert()" }}>
.
</p>
</div>
);
});
Sandbox:
useRef
useRef returns a ref object. The values ββof this object are available through the "current" property. This property can be assigned an initial value: useRef (initialValue). A ref object exists for the life of a component.
Accessing the DOM
The code:
const DomAccess = () => {
const textareaEl = useRef(null);
const handleClick = () => {
textareaEl.current.value =
" - , . , !";
textareaEl.current.focus();
};
const color = `#${((Math.random() * 0xfff) << 0).toString(16)}`;
return (
<>
<button onClick={handleClick}>
.
</button>
<label htmlFor="msg">
.
</label>
<textarea ref={textareaEl} id="msg" />
</>
);
};
Sandbox:
Instance-like variables (generics)
A ref object can contain any value, not just a pointer to a DOM element.
The code:
const StringVal = () => {
const textareaEl = useRef(null);
const stringVal = useRef(
" - , . , !"
);
const handleClick = () => {
textareaEl.current.value = stringVal.current;
textareaEl.current.focus();
};
const color = `#${((Math.random() * 0xfff) << 0).toString(16)}`;
return (
<>
<button onClick={handleClick}>
.
</button>
<label htmlFor="msg">
.
</label>
<textarea ref={textareaEl} id="msg" />
</>
);
};
Sandbox:
useRef can be used to store the ID of the timer so that it can be stopped later.
The code:
const IntervalRef = () => {
const [time, setTime] = useState(0);
const setIntervalRef = useRef();
useEffect(() => {
const id = setInterval(() => {
setTime((time) => (time = new Date().toLocaleTimeString()));
}, 1000);
setIntervalRef.current = id;
return () => clearInterval(setIntervalRef.current);
}, [time]);
return (
<>
<p> :</p>
<time>{time}</time>
</>
);
};
Sandbox:
I hope you enjoyed the article. Thank you for attention.