
Process manager for production with load balancer. Wrappers for debugging and Git hooks, securing express applications with HTTP headers, a utility with over 180 functions for working with dates, and other tools that will save you time. This collection is especially useful for novice NodeJS developers, but may please seasoned skeptics.
Whenever you need to fix a problem with your code, chances are that a package already exists to solve your problem. Here is a list of packages that I think every developer should know about. Consider these npm packages as a time saver.
1. Husky
Husky makes it easy to implement hooks in Git. It allows you to require everyone to automatically lint and test your code before committing or pushing to the repository.

Sources husky
Installation
yarn add husky
Application
Example of hooks with
husky
:
// package.json
{
"husky": {
"hooks": {
"pre-commit": "npm lint",
"pre-push": "npm test"
}
}
}
pre-commit
runs before commit.
pre-push
before submitting to the repository.
2. Dotenv
Dotenv is a module without dependencies. It loads environment variables from file
.env
into process.env . Keeping the configuration in an environment separate from the code is based on the twelve-factor application methodology . Sources dotenv .
Installation
yarn add dotenv
Application
As early as possible in your application, write
require
and set the values dotenv
:
require('dotenv').config()
Create a
.env
file in the root directory of your project. Add environment variables on new lines as NAME=VALUE
. For instance:
DB_HOST=localhost
DB_USER=root
DB_PASS=s1mpl3
Now
process.env
contains the keys and values from the file .env
:
const db = require('db')
db.connect({ host: process.env.DB_HOST, username: process.env.DB_USER, password: process.env.DB_PASS})
3.date-fns
Date-fns is similar to lodash, but designed to work with dates. Provides a complete yet simple and consistent set of JavaScript date manipulation tools in the browser and Node.JS.

Sources date-fns
Installation
yarn add date-fns
Application
Example with date-fns:
import { compareAsc, format } from 'date-fns'
format(new Date(2014, 1, 11), 'yyyy-MM-dd')
//=> '2014-02-11'
const dates = [
new Date(1995, 6, 2),
new Date(1987, 1, 11),
new Date(1989, 6, 10),
]
dates.sort(compareAsc)
//=> [
// Wed Feb 11 1987 00:00:00,
// Mon Jul 10 1989 00:00:00,
// Sun Jul 02 1995 00:00:00
// ]
Documentation with examples and use cases.
4. Bunyan
Bunyan is an easy-to-use and powerful JSON logging library for Node.

Sources bunyan
Installation
yarn add bunyan
Please note: the CLI is
bunyan
written to be compatible (within reason) with all versions of Bunyan logs. Therefore, you can install it globally: yarn add global bunyan
to get this tool in PATH
and then use local Bunyan installations for applications.
Application
Bunyan is a simple and fast JSON logging library for Node.js services.
// hi.jsconst bunyan = require('bunyan');
const log = bunyan.createLogger({name: "myapp"});
log.info("hi");
Here's what gets returned to the console if you run
node hi.js
.

5. Ramda
Ramda is a practical and useful functional style library. It emphasizes pure functional style. Immutability and side-effect-free functionality is at the heart of Ramda's design philosophy. This approach can help you write simple, elegant code.

Sources ramda
Installation
yarn add ramda
Application
import * as R from 'ramda'
const greet = R.replace('{name}', R.__, 'Hello, {name}!');
greet('Alice'); //=> 'Hello, Alice!'
Code examples
6. Debug
Debug is a tiny JavaScript debugging utility modeled after the Node.JS debugging technique.

Debug sources
Installation
yarn add debug
Application
Debug provides a function. Simply pass the name of your module to this function, and it will return a decorated version
console.error
for passing debug statements.
const debug = require('debug');
const log = debug('http:server');
const http = require('http');
const name = 'My App name';
log('booting %o', name);
http.createServer((req, res) => {
log(req.method + ' ' + req.url);
res.end('debug examplen');
}).listen(3200, () => {
log('listening');
});
//
// DEBUG=http:server node app.js
Shows and hides debug output for a module as a whole or parts of it.

7. Flat
Flat takes an object and flattens it. You can also collapse a key-separated object. [Note. transl. - not only a period can be a separator].
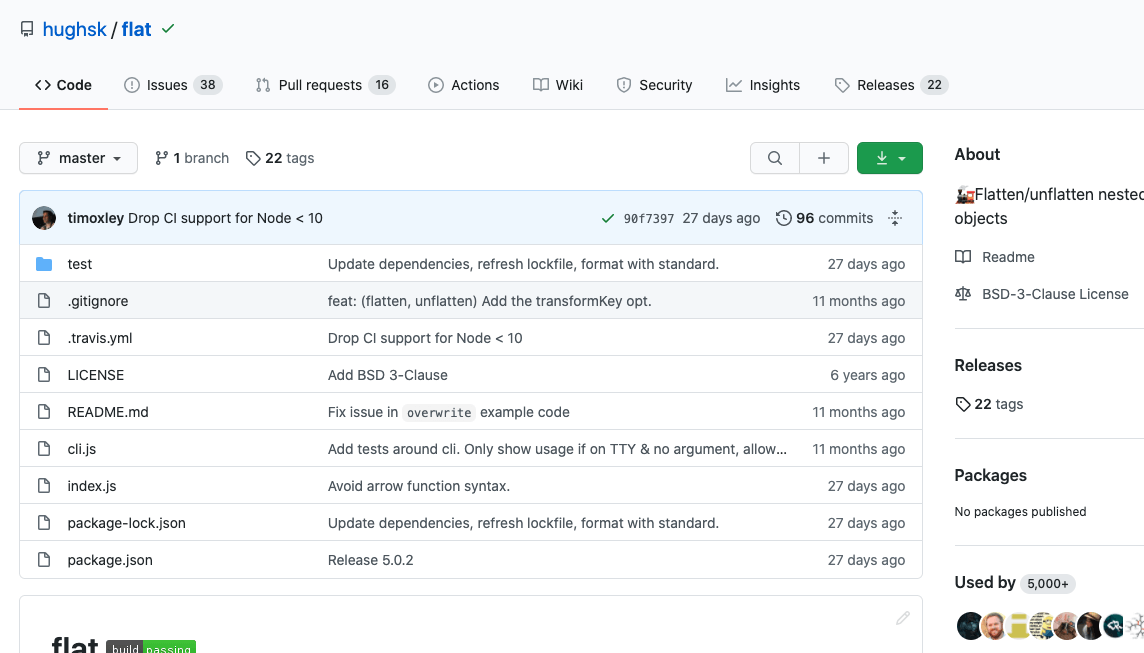
Sources Flat
Installation
yarn add flat
Application
const flatten = require('flat')
flatten({
key1: {
keyA: 'valueI'
},
key2: {
keyB: 'valueII'
},
key3: { a: { b: { c: 2 } } }
})
// {
// 'key1.keyA': 'valueI',
// 'key2.keyB': 'valueII',
// 'key3.a.b.c': 2
// }
8. JSON5
The JSON5 Data Interchange Format is a superset of JSON that aims to alleviate some of the limitations of JSON by extending its syntax to include some ECMAScript 5.1 features .

JSON5 sources
Installation
yarn add json5
const JSON5 = require('json5')
Application
Pay attention to the file extension. JSON5 is a superset, an extension of JSON.
{
//
unquoted: 'and you can quote me on that',
singleQuotes: 'I can use "double quotes" here',
lineBreaks: "Look, Mom! \
No \\n's!",
hexadecimal: 0xdecaf,
leadingDecimalPoint: .8675309, andTrailing: 8675309.,
positiveSign: +1,
trailingComma: 'in objects', andIn: ['arrays',],
"backwardsCompatible": "with JSON",
}
9. ESLint
ESLint is a great tool for avoiding mistakes and setting coding standards for development teams. ESLint is a tool for identifying and representing patterns found in ECMAScript / JavaScript code.

ESLint sources
Installation and application
yarn add eslint
Then set up the config file:
./node_modules/.bin/eslint --init
After that, you can run ESLint in any file or directory, for example:
./node_modules/.bin/eslint yourfile.js
Documentation with many examples of getting started and setting up.
10. PM2
PM2 is a production process manager with built-in load balancer. It allows you to keep applications running at all times, reload them without downtime, and ease common system administration tasks.

Sources pm2
Installation
yarn add global pm2
Application launch
You can run any application (Node.js, Python, Ruby, binaries,
$PATH
and so on) like this:
pm2 start app.js
Your application is now running under the control of a daemon and continuously monitored and maintained. Learn more about process management, quick start .
And here's how you can list all running applications:
pm2 ls

Check out the official documentation for a complete list of features and capabilities.
11. Helmet
Helmet helps secure express applications through HTTP headers. It's not a silver bullet, but it can help.

Helmet sources
Installation
yarn add helmet
Application
Helmet
is written in Connect style and is compatible with frameworks like Express . If you need Koa support, see koa-helmet
.)
const express = require("express");
const helmet = require("helmet");
const app = express();
app.use(helmet());
The helmet function is a wrapper around 11 smaller middleware. In other words, equivalent to
// ...
app.use(helmet());
// ... :
app.use(helmet.contentSecurityPolicy());
app.use(helmet.dnsPrefetchControl());
app.use(helmet.expectCt());
app.use(helmet.frameguard());
app.use(helmet.hidePoweredBy());
app.use(helmet.hsts());
app.use(helmet.ieNoOpen());
app.use(helmet.noSniff());
app.use(helmet.permittedCrossDomainPolicies());
app.use(helmet.referrerPolicy());
app.use(helmet.xssFilter());
12. Compression
Compression is a data compression tool.

Compression sources
Installation
yarn add compression
Application
When using this module along with express, or
connect
just call compression()
in middleware. Requests passing through the middleware will be compressed.
const compression = require('compression')
const express = require('express')
const app = express()
//
app.use(compression())// ...
What tools do you use to work with NodeJS?
Find out the details of how to get a high-profile profession from scratch or Level Up in skills and salary by taking online SkillFactory courses:
- JavaScript Course (12 months)
- - (8 )
- Java- (18 )
E