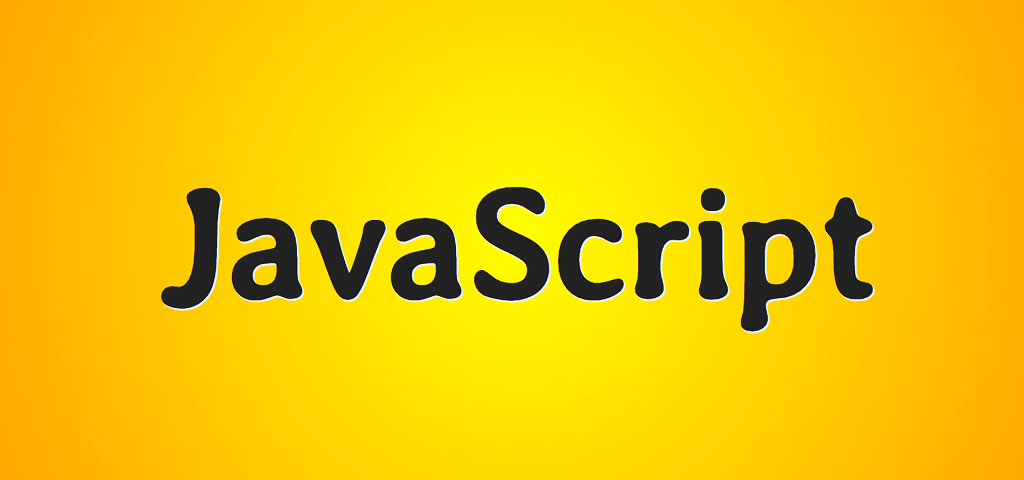
Good day, friends!
Here's a list of the second hundred JavaScript basics questions from this repository with short answers and links to Ilya Kantor's Modern JavaScript Tutorial (JSR) and MDN.
This list, along with 300+ practice questions, are available in my app .
The application implements a mechanism for memorizing the studied question, and also provides offline work.
I apologize for any possible errors and typographical errors. Any form of feedback is appreciated.
Edition of 14.09.
See the first 100 questions here .
101. What is stopPropagation () used for?
This method is used to prevent the event from bubbling up or up the chain from the ancestors of the target element. In other words, it stops sending the event from the target element to its ancestors. Let's consider an example:
<div onclick="f2()">
<div onclick="f1()"></div>
</div>
function f1(event) {
event.stopPropagation()
console.log(' ')
}
function f2() {
console.log(' ')
}
When you click on a nested container, the message "Inner container" is displayed in the console. If you remove event.stopPropagation (), then when you click on the nested container, both messages will be displayed in the console.
JSR
MDN
102. What does return false do?
This statement is used in event handlers to:
- Canceling the default browser behavior
- Preventing event propagation through the DOM
- Stopping the execution of the callback and returning control to the calling function
Note that without specifying a return value, the return statement returns undefined.
JSR
MDN
103. What is BOM?
The BOM or (Browser Object Model) allows JavaScript to interact with the browser. This model includes such objects as navigator, location, history, screen, XMLHttpRequest, etc. In other words, BOMs are additional objects provided by the browser to work with everything but the document.
Please note that the BOM is not standardized, so its implementation may differ in different browsers.

JSR
104. What is setTimeout () used for?
This method is used for deferred execution of a task. In other words, it allows you to run the execution of a function or the evaluation of an expression after a certain time (in milliseconds). In the following example, we print a message to the console after 2 seconds:
setTimeout(() => console.log('!'), 2000)
// ,
const timer = setTimeout(() => {
console.log('!')
clearTimeout(timer)
}, 2000)
JSR
MDN
105. What is setInterval () used for?
This method is used to periodically execute a task. In other words, it allows you to run execution of a function or evaluation of an expression after a certain period of time (in milliseconds). In the following example, we print a message to the console every 2 seconds:
setInterval(() => console.log('!'), 2000)
// ,
//
let i = 0
const timer = setInterval(() => {
console.log('!')
i++
if (i == 2) {
clearInterval(timer)
}
}, 2000)
In the second example, the message "Hello!" will print to the console twice, after which the timer will be stopped
JSR
MDN
106. Why is JavaScript called single threated?
JavaScript is a single threaded or synchronous programming language. This means that only one task can be executed at a time. If the task is complex, it may take a long time to complete and the main thread of code execution will be blocked during this time. Blocking a stream, in turn, means there is no interactivity on the page. The browser stops responding to user actions and other events. To solve this problem, callbacks, promises, async / await, workers and other tools for working with asynchronous code are used. In JavaScript, unlike, for example, Java, Go or C ++, there is no way to create additional threads or processes.
JSR
MDN
107. What is event delegation?
Event delegation is a technique where an event is registered on a parent to handle events raised by children.
Often used to handle button clicks in a grouping container or to modify text entry fields in forms, for example:
<form>
<input type="text" class="first-input">
<input type="text" class="second-input">
</form>
<div>
<button class="first-button">click</button>
<button class="second-button">click</button>
</div>
const form = document.querySelector('form')
const buttons = document.querySelector('div')
form.addEventListener('input', event => {
console.log(event.target.className)
})
buttons.addEventListener('click', event => {
console.log(event.target.className)
})
In the above example, instead of registering handlers on the child elements, we register them on the parent elements. Entering text in a field or pressing a button results in the output of the class name of the corresponding element to the console.
JSR
108. What is ECMAScript?
ECMAScript is the programming language behind JavaScript. This is a kind of pattern or blueprint that JavaScript is "built" by. ECMAScript is standardized in the ECMA-262 specification by the standards organization Ecma International.
JSR
MDN
109. Name the features of the JSON syntax
JSON syntax has the following features:
- Data is key / value pairs
- Key and value are wrapped in double quotes, unless the value is a number ("key": "value")
- Data are separated by commas
- Objects are wrapped in curly braces
- Arrays - to square
JSR
MDN
110. What does JSON.stringify () do?
When sending data to the server, it must have a special string format. The JSON.stringify () method is used to convert an object to a JSON string:
const user = { name: '', age: 30 }
const str = JSON.stringify(user)
console.log(str) // {"name":"","age":30}
JSR
MDN
111. What does JSON.parse () do?
When receiving data from the server, they have a special string format. The JSON.parse () method is used to convert this data to a JavaScript object:
const str = { "name":"","age":30 }
const user = JSON.parse(str)
console.log(user) // {name: "", age: 30}
JSR
MDN
112. What is JSON for?
When exchanging data between client and server, this data can only be strings. Since JSON is text, it is perfect for this. It can also be used as a data format by any programming language, along with other formats such as XML or Protobuf.
JSR
MDN
113. What is PWA (Progressive Web Application - progressive web application)?
In short, PWAs are websites that behave like native applications. They can be installed on a phone or computer, and, as a rule, they work offline. For the latter, service workers and a caching interface are used. The advantage of PWAs over mobile applications is their size and relative ease of development. Also, you do not need to spend resources on creating two applications for one site - web and mobile. It also maintains a good user experience.
MDN
114. What is clearTimeout () used for?
This method is used to stop a timer started by setTimeout (). To do this, the timer identifier is written to a variable, which is then passed to clearTimeout () as an argument.
const timer = setTimeout(() => {
console.log('!')
clearTimeout(timer)
}, 2000)
In the above example, two seconds later, the message βHello!β Is displayed on the console, after which the timer stops. This is done so that the garbage collector can remove the timer that has run.
JSR
MDN
115. What is clearInterval () used for?
This method is used to stop a timer started with setInterval (). To do this, the timer identifier is written to a variable, which is then passed to clearInterval () as an argument.
let i = 1
const timer = setInterval(() => {
console.log(i)
i++
if (i === 3) clearInterval(timer)
}, 1000)
In the above example, the value of the variable i is printed to the console every second, which is incremented by 1 (1, 2) each time. When i becomes 3, the timer stops.
JSR
MDN
116. How do I redirect?
To do this, you can use the location property of the window object:
location.href = 'newPage.html'
//
location.replace('newPage.html')
//
location.assign('newPage.html')
MDN
117. How to check if a substring exists in a string?
There are at least three ways to do this.
String.prototype.includes ()
const mainStr = 'hello'
const subStr = 'hel'
mainStr.includes(subStr) // true
String.prototype.indexOf ()
const mainStr = 'hello'
const subStr = 'hel'
mainStr.indexOf(subStr) !== -1 // true
RegExp
const mainStr = 'hello'
const regex = /hel/
regex.test(mainStr) // true
JSR
MDN - includes
MDN - indexOf
MDN - test
118. How to check the correctness of the email address?
This can be done using HTML by setting the type of the input field to the value email (<input type = "email">). However, this method is not considered very reliable. Therefore, usually, email is validated using a regular expression. It is recommended to do this on the server side as JavaScript can be disabled on the client:
const validateEmail = email =>
/\S+@\S+.\S+/
.test(email.toString()
.toLowerCase())
const email = 'myemail@example.com'
validateEmail(email) // true
This example uses one of the simplest regular expressions to validate an email address. A more reliable expression looks like this (RFC 2822): [a-z0-9! # $% & '* + / =? ^ _ \ `{|} ~ -] + (?:. [A-z0-9! # $% & '* + / =? ^ _ \ `{|} ~ -] +) * @ (?: [a-z0-9] (?: [a-z0-9 -] * [a-z0- 9])?.) + [A-z0-9] (?: [A-z0-9 -] * [a-z0-9])?
119. How do I get the current URL?
To do this, you can use the location property of the window object or the URL property of the document object:
console.log(' URL', location.href)
console.log(' URL', document.URL) // Chrome " URL chrome-search://local-ntp/local-ntp.html"
MDN - location
MDN - document.URL
120. What properties does the location object have?
The properties of the location object can be used to get parts of the URL of the current page:
- href - full URL
- origin - protocol, host and port (origin, used in Common Origin Policy (SOP) and Resource Sharing (CORS))
- protocol
- host - host and port
- hostname - host
- port
- pathname - path
- search - the query string after?
- hash - query string after # (anchor)
- username - username before domain
- password - password before the domain
MDN
121. How to get the query string?
You can use the URL constructor for this:
const url = new URL('https://example.com?foo=1&bar=2')
console.log(url.search) // ?foo=1&bar=2
console.log(url.searchParams.get('foo')) // 1
MDN
122. How to check if a property exists in an object?
There are at least three ways to do this.
In operator
const user = { name: '' }
console.log('name' in user) // true
console.log(!('age' in user)) // true
HasOwnProperty () method
const user = { name: '' }
console.log(user.hasOwnProperty('name')) // true
console.log(!user.hasOwnProperty('age')) // true
Comparison with undefined
const user = { name: '' }
console.log(user.name !== undefined) // true
console.log(user.age === undefined) // true
JSR
MDN - for ... in
MDN - hasOwnProperty
123. How to iterate over the enumerated properties of an object?
To do this, you can use a for ... in loop with the hasOwnProperty () method to exclude inherited properties.
const user = {
name: '',
age: 30
}
for (key in user) {
if (user.hasOwnProperty(key)) {
console.log(\`${key}: ${user[key]}\`) // name: age: 30
}
}
JSR
MDN
124. How to check that the object is empty?
There are at least three ways to do this.
Object.entries () method
const obj = {}
console.log(Object.entries(obj).length === 0) // true
// Date
const obj2 = new Date()
console.log(Object.entries(obj2).length === 0 && obj2.constructor === Object) // false
Object.keys () method
const obj = {}
console.log(Object.keys(obj).length === 0) // true
// Date
const obj2 = new Date()
console.log(Object.keys(obj2).length === 0 && obj2.constructor === Object) // false
For ... in loop and Object.hasOwnProperty () method
const obj = {}
const obj2 = {key: 'value'}
const isEmpty = obj => {
for (key in obj) {
if (obj.hasOwnProperty(key)) return false
}
return true
}
console.log(isEmpty(obj)) // true
console.log(isEmpty(obj2)) // false
JSR - JSR Objects
- Object.keys, values, entries
MDN - Object.entries
MDN - Object.keys
MDN - for ... in
MDN - Object.hasOwnProperty
125. What is the arguments object?
arguments is an array-like object (pseudo-array) containing the arguments passed to the function:
function sum () {
let total = 0
for (let i = 0; i < arguments.length; i++) {
total += arguments[i]
}
return total
//
let total = 0
for (const i of arguments) {
total += i
}
return total
//
return Array.from(arguments).reduce((acc, cur) => acc + cur)
}
sum(1, 2, 3) // 6
Note that arrow functions do not have arguments. Instead of arguments, it is recommended to use the rest operator ... (other parameters), which works in both ordinary and arrow functions:
const sum = (...rest) => rest.reduce((acc, cur) => acc + cur)
sum(1, 2, 3) // 6
JSR
MDN
126. How to capitalize the first letter of a string?
This can be done using the charAt (), toUpperCase () and slice () methods:
String.prototype.capitilize = function () {
return this.charAt(0).toUpperCase() + this.slice(1)
}
console.log('hello'.capitilize()) // Hello
JSR
MDN - charAt
MDN - toUpperCase
MDN - slice
127. How to get the current date?
This can be done using a Date object or the Intl.DateTimeFormat constructor:
console.log(new Date().toLocaleDateString()) // 02.09.2020
console.log(new Intl.DateTimeFormat(
'ru-Ru',
{
weekday: 'long',
day: 'numeric',
month: 'long',
year: 'numeric'
}
).format(new Date())) // , 2 2020 .
JSR - Date
JSR = Intl
MDN - Date
MDN - Intl.DateTimeFormat
128. How to compare two Date objects?
To do this, you should not compare the objects themselves, but, for example, the values ββreturned by the getTime () method:
const d1 = new Date()
const d2 = new Date(d1)
console.log(d1.getTime() === d2.getTime()) // true
console.log(d1 === d2) // false
JSR
MDN
129. How to check that a line starts on a different line?
You can use the built-in startsWith () method to do this:
console.log('Good morning'.startsWith('Good')) // true
console.log('Good morning'.startsWith('morning')) // false
According to CanIUse, almost 94% of
JSR MDN browsers support this method
130. How to remove problems in a line?
To do this, you can use the built-in methods trimStart () (start of line), trimEnd () (end of line), and trim () (start and end of line):
console.log(' hello world '.trim()) // hello world
trim does not work for spaces between words. In this case, you can use the replace () method and regular expression:
console.log('hello world'.replace(/s+/, ' ')) // hello world
//
console.log('hello world'.replace(/s{2,}/, ' ')) // hello world
console.log('key value'.replace(/s{2,}/, ' -> ')) // key -> value
According to CanIUse , trimStart () and trimEnd () are supported by 93% of browsers.
MDN
131. How to add a new property to an object?
There are two ways to do this. Let's assume we have an object like this:
const obj = {
name: '',
age: 30
}
We can add a new property to it using either dot or bracket notation:
obj.job = ''
obj['job'] = ''
One of the differences between these methods is that when using parenthesis notation, the added key can be a number:
const obj = {}
obj[1] = ''
console.log(obj) // { 1: '' }
obj.2 = '' // SyntaxError: Unexpected number
JSR
MDN
132. Is the expression! - a special operator?
No, it's not. It is a combination of two operators: operator! (logical not) and operator - (decrement). If you use the specified expression with any value, then first this value will be decreased by one, then converted to a boolean type and inverted:
const fun = val => !--val
const a = 1
const b = 2
console.log(fun(a)) // !0 -> not false -> true
console.log(fun(b)) // !1 -> not true -> false
JSR
MDN - Logical NOT
MDN - Decrement
133. How to assign a default value to a variable?
To do this, you can use the || (boolean or):
const a = b || 'default'
In this case, the variable a will be assigned the default value if the value of the variable b is false (false, undefined, null, NaN, 0, '').
If we are talking about the standard values ββof the function parameters, then they can be assigned as follows:
const greet = (name = '') => \`, ${name}!\`
console.log(greet('')) // , !
console.log(greet()) // , !
Moreover, subsequent parameters can use the values ββof the previous ones as standard values:
const sum = (a = 1, b = a + 2) => a + b
console.log(sum()) // 4
JSR
MDN
134. How to create a multiline string?
Previously, it was done something like this (concatenation and line break control characters):
const str =
' ' + ' ' +
' ' + ' ' +
'!'
//
const str = ' a
!'
console.log(str)
/*
!
*/
Now they do it like this (template literal):
const str =
\`
!\`
JSR
MDN
135. Can we add properties to functions?
Since functions are objects too, we can easily add properties to them. The function property value can be another function:
function someFun () {}
someFun.somePropName = 'somePropValue'
console.log(someFun.somePropName) // somePropValue
//
console.log(someFun.name) // someFun
const sum = (x, y) => x + y
console.log(sum(1, 2)) // 3
sum.curry = x => y => x + y
console.log(sum.curry(1)(2)) // 3
JSR
MDN
136. How to find out how many arguments a function expects to receive?
The length property can be used for this:
const sum = (a, b, c) => +a + +b + +c
console.log(sum(1, '1', true)) // 3
console.log(sum(0, '', [])) // 0
console.log(sum.length) // 3
MDN
137. What is a polyfill?
Polyfills are used to make modern JavaScript work in older browsers. This is done by implementing new features of the language using the old syntax. The very process of converting new code into old code is called transpilation. The most popular transpiler for JavaScript code is Babel.
For example, one of the latest JavaScript features is the Promise.allSettled () method, which, unlike Promise.all (), does not terminate when any of the promises passed to it are rejected.
However, as of today, its browser support according to CanIUse data is 80%, so a polyfill is needed:
const promise1 = Promise.resolve('promise1')
const promise2 = Promise.reject('promise2')
const promise3 = Promise.resolve('promise3')
// Promise.allSettled()
Promise
.allSettled([promise1, promise2, promise3])
.then(console.log)
/*
[
{status: "fulfilled", value: "promise1"},
{status: "rejected", reason: "promise2"},
{status: "fulfilled", value: "promise3"},
]
*/
//
// Promise.all() = 94%
const allSettled = promises => {
const wrappedPromises = promises
.map(p => Promise.resolve(p)
.then(
val => ({
status: 'fulfilled',
value: val
}),
err => ({
status: 'rejected',
reason: err
})))
return Promise.all(wrappedPromises)
}
allSettled([promise1,promise2,promise3])
.then(console.log)
JSR
MDN
138. What are the continue and break statements used for?
The break statement is used to break out of the loop. After stopping the iteration, the code continues:
const obj = {
1: 'Everything',
2: 'is',
3: 'impossible'
}
for (key in obj) {
if (obj[key] === 'impossible') break
console.log(obj[key]) // Everything is
}
console.log('possible') // possible
The continue statement is used to skip iteration:
const obj = {
1: 'Everything',
2: 'is',
3: 'impossible',
4: 'possible'
}
for (key in obj) {
if (obj[key] === 'impossible') continue
console.log(obj[key]) // Everything is possible
}
MDN - break
MDN - continue
139. What is a label?
Labels let you name loops and code blocks. They can be used, for example, to exit a loop or as a condition for executing code:
loop1:
for (let i = 0; i < 3; i++) {
loop2:
for (let j = 0; j < 3; j++) {
if (i === j) continue loop1
console.log(\`i = ${i}, j = ${j}\`)
}
}
/*
i = 1, j = 0
i = 2, j = 0
i = 2, j = 1
*/
It is considered bad practice to use labels.
MDN
140. What are the advantages of declaring variables at the beginning of the code?
It is recommended to declare variables at the beginning of each script or function. This provides the following benefits:
- Keeps the code clean
- All variables are in one place
- Avoids accidentally creating global variables
- Prevents unwanted variable overrides
JSR
MDN
141. What are the advantages of initializing a variable when declared?
It is recommended to initialize all variables at the time of declaration. This provides the following benefits:
- Keeps the code clean
- Variables and their values ββare in one place
- Prevents uninitialized variables from being assigned undefined
JSR
MDN
142. What are the main recommendations for creating an object
To create an object, instead of the object constructor new Object (), it is recommended to use the parenthesis notation {}. Also, depending on the value type, it is recommended to use the following:
- string like '' instead of new String ()
- a number, for example 0 instead of new Number ()
- boolean value like false instead of new Boolean ()
- [] instead of new Array ()
- // instead of new RegExp ()
- function () {} instead of new Function ()
JSR
MDN
143. How to define an array in JSON format?
JSON array is an array of JSON objects, for example:
[
{ "name": "", "age": 30 },
{ "name": "", "age": 20 }
]
JSR
MDN
144. How to implement a function that returns a random integer in a given range?
Such a function can be implemented using the Math.random () and Math.floor () methods of the Math object:
const getRandomInteger = (min, max) => Math.floor(min + Math.random() * (max + 1 - min))
JSR
MDN - Math.random ()
MDN - Math.floor ()
145. What is tree shaking?
Tree shaking is the removal of the code of unused modules. Such modules are not included in the final assembly (bundle). In order for the module builder (bundler) to determine which modules are in use and which are not, the program structure must be based on ES6 modules. This technique was popularized by the Rollup bundler.
MDN
146. What is tree shaking used for?
Tree shaking can significantly reduce the size of an assembly (bundle) by removing the code of unused modules from it. The smaller the assembly size, the better the application performance. Tree shaking is implemented in module builders such as Rollup and Webpack.
MDN
147. What is a regular expression?
A regular expression is a sequence of characters that form a search pattern. This pattern can be used to search for data in text, such as substrings in a string. Regular expressions are widely used by many programming languages ββfor text search and replace operations. The general regex pattern looks like this:
//
Example:
const regex = /java/i
const str = 'JavaScript'
console.log(regex.test(str)) // true
You can also use the RegExp constructor to create a regular expression:
const regex = new RegExp('java', 'i')
const str = 'JavaScript'
console.log(regex.test(str)) // true
JSR
MDN
148. What methods are used in regular expressions?
There are two main methods used in regular expressions: exec () and test ().
The exec () method searches for a regular expression match in the string passed to it as an argument. The behavior of this method depends on whether the regular expression has the g flag. If not, the first match is returned. If the g flag is present, then:
- The exec () call returns the first match and stores the position after it in the lastIndex property.
- The next such call starts the search at position lastIndex, returns the next match, and remembers the position after it in lastIndex.
- If there are no more matches, exec () returns null and lastIndex is set to 0.
const str = 'Java JavaScript - '
const regex = /Java/g
let result
while (result = regex.exec(str)) {
console.log(
\` ${result[0]} ${result.index}\`
)
}
/*
Java 0
Java 7
*/
The test () method returns a boolean value depending on whether a match is found in the string:
const str = ' JavaScript'
console.log(
/ /.test(str) // true
)
JSR
MDN
149. What flags are used in regular expressions?
Flag | Description |
---|---|
g | global comparison |
i | ignore case when matching |
m | matching across multiple lines |
const regex = /([-]+)s([-]+)/i
const str = ' '
const newStr = str.replace(regex, '$2 $1')
console.log(newStr) //
JSR
MDN
150. What special characters are used in regular expressions?
The special characters used in regular expressions can be divided into several groups.
Basic character classes:
Symbol | Value |
---|---|
\. | any character with some exceptions |
\ d | numeral |
\ D | not a number |
\ w | Latin character and underscore |
\ W | not latin character and underscore |
\ s | whitespace character |
\ S | no whitespace character |
\ | escaping like \. Is the point |
Character sets:
Symbol | Value |
---|---|
[a-yayo-yo] | any letter of the Russian alphabet |
[^ a-yyoA-yyo] | any character except the letters of the Russian alphabet |
Borders:
Symbol | Value |
---|---|
^ | start of line |
$ | end of line |
\ b | zero-width word boundary |
\ B | non-zero-width word boundary |
Grouping:
Symbol | Value |
---|---|
(x) | matches x, the match is remembered |
(?: x) | matches x, the match is not remembered |
Quantifiers:
Symbol | Value |
---|---|
* | zero or more characters |
+ | one or more characters |
*? and +? | similar to * and +, but looking for the minimum match |
? | zero or one character |
x (? = y) | matches x if x is followed by y |
x (?! y) | matches x if x is not followed by y |
(? <= y) x | matches x if x precedes y |
(?! y) x | matches x if x does not precede y |
x | y | x or y |
x {n} | n is the exact number of x |
x {n,} | n - minimum number of x |
x {n, m} | n - minimum number x, m - maximum (from, to) |
JSR
MDN
151. How do I change the styles of an HTML element?
This can be done either by using the style property or by assigning an appropriate class to the element:
document
.querySelector(selector)
.style.property = value
document
.querySelector('title')
.fontSize = '2rem'
document.querySelector(selector)
.className = 'class-name'
document.querySelector(selector)
.classList.add('class-name')
document.querySelector('button')
.classList.add('active')
JSR
MDN - style
MDN - className
MDN - classList
152. What is a debugger?
The debugger expression provides access to any debugging functionality available in a specific environment, for example, setting breakpoints (breakpoints, breakpoints). If debugging functionality is not available at runtime, this expression will have no effect:
const fun = () => {
//
debugger //
//
}
JSR
MDN
153. What are debugger breakpoints used for?
Checkpoints are used to pause the execution of a function or other code at a specific place in order to find out why a program is not working properly. Once stopped, the function can be continued.
JSR
MDN
154. Can reserved words be used as identifiers?
No, you cannot use reserved words as names for variables, labels, functions, or objects:
const class = ' ' // SyntaxError: Unexpected token 'class'
155. How to determine the width and height of the image?
This can be done in a number of ways. Here is one of them:
const getImgSize = src => {
const img = new Image()
img.src = src
img.addEventListener('load', () => console.log(\`${img.width} x ${img.height}\`)) // 276 x 110
document.body.append(img)
}
getImgSize('http://www.google.com/ intl/en_ALL/images/logo.gif')
MDN
156. How to send a synchronous HTTP request?
To do this, you can use the XMLHttpRequest object by passing it to the open () method with a third optional argument with the value false:
const getUsers = url => {
const xhr = new XMLHttpRequest()
xhr.open('GET', url, false)
xhr.send()
console.table(xhr.response)
const response = JSON.parse(xhr.response)
const template = \`
<table>
${response.reduce((html, user) => html += \`
<tr>
<td>${user.name}</td>
<td>${user.username}</td>
<td>${user.email}</td>
</tr>\`, '')}
<table>
\`
document.body
.insertAdjacentHTML('beforeend', template)
}
getUsers('https://jsonplaceholder. typicode.com/users')
JSR
MDN
157. How to make an asynchronous HTTP request?
The fetch () method can be used for this:
const getUsers = async url => {
const response = await fetch(url)
const data = await response.json()
console.table(data)
const template = \`
<table>
${data.reduce((html, user) => html += \`
<tr>
<td>${user.name}</td>
<td>${user.username}</td>
<td>${user.email}</td>
</tr>\`, '')}
<table>
\`
document.body.insertAdjacentHTML('beforeend', template)
}
getUsers('https://jsonplaceholder. typicode.com/users')
JSR
MDN
158. How to get the date in the required format?
The toLocaleString () method can be used for this:
console.log(
new Date().toLocaleString('ru-Ru', {
weekday: 'long',
year: 'numeric',
month: 'long',
day: 'numeric'
})
) // , 6 2020 .
MDN
159. How to get the maximum page sizes?
To do this, you need to find the maximum values ββof the properties scrollWidth, offsetWidth, clientWidth and scrollHeight, offsetHeight, clientHeight of the document.body and document.documentElement objects:
const pageWidth = Math.max(
document.body.scrollWidth, document.documentElement.scrollWidth,
document.body.offsetWidth, document.documentElement.offsetWidth,
document.body.clientWidth, document.documentElement.clientWidth
)
const pageHeight = Math.max(
document.body.scrollHeight, document.documentElement.scrollHeight,
document.body.offsetHeight, document.documentElement.offsetHeight,
document.body.clientHeight, document.documentElement.clientHeight
)
const pageSize = {
width: pageWidth,
heigth: pageHeight
}
console.log(pageSize)
const pageCenter = {
centerX: pageWidth / 2,
centerY: pageHeight / 2
}
console.log(pageCenter)
JSR
160. What is a conditional or ternary operator?
The ternary operator is a shorthand way of writing the if ... else block:
let accesAllowed
const age = propmt(' ?')
// if...else
if (age > 18) {
accesAllowed = true
} else {
accessAllowed = false
}
//
(age > 18)
? accesAllowed = true
: accessAllowed = false
JSR
MDN
161. Can a chain of ternary operators be used?
Yes, in this case the ternary operator is an alternative to the if ... else if ... else block:
let accessAllowed
const getAge = () => prompt(' ?')
// -
// if...else if...else
const checkAge = (age = getAge()) => {
console.log(age)
if (isNaN(age)) {
Promise.resolve(alert(' ')).then(accessAllowed = false).then(checkAge)
} else if (age === null || age === '') {
Promise.resolve(alert(' ')).then(accessAllowed = false).then(checkAge)
} else if (age < 0) {
Promise.resolve(alert(' 0')).then(accessAllowed = false).then(checkAge)
} else if (age > 100) {
Promise.resolve(alert(' 100')).then(accessAllowed = false).then(checkAge)
} else if (age < 18) {
Promise.resolve(alert(', ')).then(accessAllowed = false)
} else {
Promise.resolve(alert(' !')).then(accessAllowed = true)
}
console.log(accessAllowed)
}
//
const checkAge = (age = getAge()) => {
isNaN(age)
? Promise.resolve(alert(' ')).then(accessAllowed = false).then(checkAge)
: (age === null || age === '')
? Promise.resolve(alert(' ')).then(accessAllowed = false).then(checkAge)
: (age < 0)
? Promise.resolve(alert(' 0')).then(accessAllowed = false).then(checkAge)
: (age > 100)
? Promise.resolve(alert(' 100')).then(accessAllowed = false).then(checkAge)
: (age < 18)
? Promise.resolve(alert(', ')).then(accessAllowed = false)
: Promise.resolve(alert(' !')).then(accessAllowed = true)
console.log(accessAllowed)
}
JSR
MDN
162. How to start executing the code after the page is fully loaded?
This can be done in several ways.
Place the script tag before the closing body tag or add a defer attribute to it:
<body>
...
<script src="script.js"></script>
</body>
<!-- -->
<head>
...
<script src="script.js" defer></script>
</head>
If your script is a module, then instead of the defer attribute, you need to specify the type attribute with the value module:
<script src="script.js" type="module"></script>
Add an onload attribute to the body tag:
<body onload="script()"></body>
Add code as a handler for the load event of the window object:
window.onload = () => console.log(' ')
//
window.addEventListener('load', () => console.log(' '))
Do the same for document.body:
document.body.onload = () => console.log(' ')
163. What is the difference between __proto__ and prototype?
The __proto__ property ([[Prototype]] internal hidden property) is an object from which an instance inherits fields and methods. And prototype is an object that is used to create __proto__ when instantiated using the new keyword:
class Person {
constructor(firstName, secondName) {
this.firstName = firstName
this.secondName = secondName
}
getFullName() {
return \`${this.firstName} ${this.secondName}\`
}
}
const user = new Person('', '')
console.log(user.getFullName()) //
console.log(user.__proto__.getFullName === Person.prototype.getFullName) // true
console.log(Person.prototype) // {constructor: Ζ, getFullName: Ζ}
console.log(user.prototype === undefined) // true
JSR
MDN
164. Give an example of the mandatory use of a semicolon
One of the mandatory use of semicolons is the use of IIFE (Immediately Invoked Fuction Expression):
For example, the following code:
try {
const x = ''
(() => {
console.log(x)
})()
} catch {
console.log(' ')
}
Will be interpreted like this:
try {
const x = ''(() => {
console.log(x)
})()
} catch {
console.log(' ')
}
Therefore, in the try block, we get a TypeError: "To be" is not a function, control is passed to the catch block, and "Not to be" is output to the console.
For the code to work as expected, it should look like this:
try {
//
const x = '';
//
;(() => {
console.log(x)
})()
} catch {
console.log(' ')
}
Also, don't forget about cases of automatic semicolon placement.
165. What is the freeze () method used for?
This method, as its name implies, serves to "freeze" an object. A frozen object is immutable (immutable). This means that you cannot add new properties to such an object, delete or modify existing ones. Also this method sets configurable: false and writable: false for existing properties. The method returns a frozen object.
'use strict'
const obj = {
mission: 'possible'
}
Object.freeze(obj)
obj.mission = 'impossible' // TypeError: Cannot assign to read only property 'mission' of object '#<Object>'
delete obj.mission // TypeError: Cannot delete property 'mission' of #<Object>
Note that in non-strict mode, no exception is thrown, the code simply does not execute.
JSR
MDN
166. Why do we need the freeze () method?
The object-oriented programming paradigm states that an interface containing a certain number of elements must be immutable, i.e. it must be impossible to extend, modify or use elements outside the current context. This method is an alias for the final keyword in some other programming languages.
JSR
MDN
167. How to capitalize the first letter of each word in a line?
One way to do this is as follows:
const capitilize = str => str.replace(
/[-]S+/gi,
txt => txt[0].toUpperCase() + txt.slice(1).toLowerCase()
)
console.log(capitilize(', , ')) // , ,
168. How do I know if JavaScript is disabled on a page?
You can use the noscript tag for this. The code inside this tag will only be executed if JavaScript is disabled on the page:
console.log('JavaScript ')
<noscript>
<p> JavaScript, </p>
</noscript>
In order to disable JavaScript in Chrome, go to settings -> section "Privacy and security" -> Site settings -> section "Content" -> JavaScript.
MDN
169. What operators are supported by JavaScript?
Operators are used to work with values ββor operands. JavaScript supports the following operators:
- Arithmetic: + (addition, casting to a number, concatenation), - (subtraction), * (multiplication), / (division),% (modulo, with remainder), ++ (increment), - (decrement), * * (exponentiation)
- comparison operators: == (abstract, loose equality),! = (abstract inequality), === (strict equality, identity check),! == (strict inequality),>,> =, <, <=
- logical: && (and), || (or),! (not) (!!! (double negation) is not a separate operator)
- assignment operators: =, + =, - =, * =, / =,% =
- ternary:? ...: (if ... else)
- typeof operator: defines the type of the operand
- bitwise: & (and), | (or), ^ (exclusive or), ~ (not), << (left shift), >> (right shift), >>> (right shift with zero padding)
- new:? .. ββ(optional chain), ?? (null merge)
//
const obj = {
foo: {
baz: {
qux: 'bar'
}
}
}
//
console.log(obj.foo.bar.baz.qux) // TypeError: Cannot read property 'baz' of undefined
if (
obj.foo !== undefined &&
obj.foo.bar !== undefined &&
obj.foo.bar.baz !== undefined
) {
console.log(obj.foo.bar.baz.qux) //
}
//
console.log(obj?.foo?.bar?.baz?.qux) // undefined
// null
console.log(
0 || 'default null', // 'default null'
0 ?? 'default null', // 0
'' || 'default string', // default string
'' ?? 'default string', // ''
)
JSR - JSR Operators
- Logical Operators
JSR - Comparison Operators
JSR - Conditional
JSR Operators - Bitwise
MDN Operators - Optional Sequence
Operator MDN - Null Merge Operator
170. What is the rest operator ... (other parameters) used for?
The rest operator is an alternative to the arguments object and returns an array of the arguments passed to the function:
const sum = (...rest) => rest.reduce((acc, cur) => acc + cur)
console.log(sum(1, 2, 3)) // 6
Please note that the rest operator must be passed as the last argument:
const fun = (x, ...rest, y) => console.log(rest) // SyntaxError: Rest parameter must be last formal parameter
JSR
MDN
171. What is the spread operator used for?
The spread operator is used to expand (unpack, expand) iterable entities (arrays, strings). Unpacking means converting, for example, an array of numbers to a set of simple values:
const sum = (x, y, z) => x + y + z
const nums = [1, 2, 3]
console.log(sum(...nums)) // 6
JSR
MDN
172. How to determine if an object is frozen?
In order to determine if an object is frozen, i.e. is it immutable (immutable) the isFrozen () method is used:
const obj = {
prop: ' JavaScript!'
}
Object.freeze(obj)
console.log(Object.isFrozen(obj)) // true
MDN
173. How to determine equality of values ββusing an object?
The is () method can be used for this:
Object.is(' ', ' ') // true
Object.is(0.1 + 0.2, 0.3) // false
Object.is(window, window) // true
Object.is(+0, -0) // false
const objA = {}
const objB = objA
Object.is(objA, objB) // true
Object.is({}, {}) // false
The values ββare equal if:
- both are undefined
- both are null
- both are true or false
- both are strings of the same length with the same characters
- both refer to the same object
- both are numbers, +0, -0 or NaN
JSR
MDN
174. How to create a copy of an object?
One of the most reliable ways to do this is by using the assign () method:
const objA = {a: 1, b: 2}
const objB = Object.assign(objA)
console.log(objB) // {a: 1, b: 2}
console.log(objA === objB) // true
Also, this method allows you to combine objects, excluding duplicates:
const objA = {a: 1, b: 2}
const objB = {b: 2, c: 3}
const objC = Object.assign(objA, objB)
console.log(objC) {a: 1, b: 2, c: 3}
You can use the JSON.parse-JSON.stringify binding to copy simple objects:
const objA = {a: 1, b: 2}
const objB = JSON.parse(JSON.stringify(objA))
console.log(objB) // {a: 1, b: 2}
JSR
MDN
175. What is a proxy?
The Proxy object "wraps" around another object and can intercept (and, if desired, independently process) various actions with it, for example, reading / writing properties and others.
const handler = {
get: (obj, prop) => prop in obj
? obj[prop]
: 0
}
// new Proxy(, )
const p = new Proxy({}, handler)
p.a = 1
p.b = true
console.log(p.a, p.b) // 1 true
console.log( 'c' in p, p.c) // false 0
176. What is the seal () method used for?
This method "seals" the object, preventing adding / removing properties. It also sets configurable: false for all existing properties. However, the values ββof the properties of such an object can be changed. The isSealed () method is used to check if an object is sealed.
'use strict'
const obj = {
prop: ' JavaScript!'
}
Object.seal(obj)
obj.prop = ' , '
console.log(Object.isSealed(obj)) // true
delete obj.prop // TypeError: Cannot delete property 'prop' of #<Object>
console.log(obj.prop) // ,
Note that in lax mode, an attempt to delete a property on a sealed object will silently fail.
JSR
MDN
177. What is the difference between freeze () and seal () methods?
The Object.seal () method, unlike the Object.freeze () method, allows you to modify the existing properties of an object.
'use strict'
const objA = {
prop: ''
}
Object.freeze(objA)
objA.prop = ' ' // TypeError: Cannot assign to read only property 'prop' of object '#<Object>'
const objB = {
prop: ' '
}
Object.seal(objB)
objB.prop = ''
console.log(objB.prop) //
178. How to get enumerated key / value pairs of an object?
The Object.entries () method returns an array of enumerated object key / value pairs as subarrays in the same order as the for ... in loop:
const obj = {
x: 1,
y: 2
}
console.log(Object.entries(obj)) // [["x", 1], ["y", 2]]
for (let [key, value] of Object.entries(obj)) {
console.log(\`${key}: ${value}\`) // x: 1 y: 2
}
JSR
MDN
179. What is the main difference between the Object.keys (), Object.values ββ() and Object.entries () methods?
The Object.keys () method returns the keys of an object, the Object.values ββ() method returns the values ββof its properties, and Object.entries () returns an array of key / value pairs:
const user = {
name: '',
age: 30
}
console.log(Object.keys(user)) // ["name", "age"]
console.log(Object.values(user)) // ["", 30]
console.log(Object.entries(user)) // [["name", ""], ["age", 30]]
JSR
MDN - Object.keys ()
MDN - Object.values ββ()
MDN - Object.entries ()
180. How to create an object with a specific prototype without using a constructor function and classes?
The Object.create () method can be used for this:
const firstUser = {
name: '',
sayHi() {
console.log(\`, ${this.name}!\`)
}
}
const secondUser = Object.create(firstUser)
secondUser.name = ''
secondUser.sayHi() // , !
JSR
MDN
181. What is WeakSet used for?
WeakSet is used to store a collection of loosely referenced objects. In other words, it serves as additional storage for objects used by other code. Such objects are automatically removed by the garbage collector when they become unreachable (unused), i.e. when only the key remains in the WeakSet.
const ws = new WeakSet()
let user = {}
ws.add(user)
console.log(ws.has(user)) // true
user = null
console.log(ws.has(user)) // false,
JSR
MDN
182. What is the difference between Set and WeakSet?
Their main difference is that the objects stored in the WeakSet are weakly referenced, i.e. are automatically deleted as soon as they become unreachable. Other differences are as follows:
- Set can store any value, and WeakSet can only store objects
- WeakSet has no size property
- WeakSet doesn't have clear (), keys (), values ββ(), forEach () methods
- WeakSet is not an iterable entity
JSR
MDN
183. What methods are available in the WeakSet?
WeakSet has the following methods:
- add (): adds an object to the collection
- delete (): removes an object from a collection
- has (): determines if an object is in the collection
- length (): returns the length of the collection
const ws = new WeakSet()
const objA = {}
const objB = {}
ws.add(objA)
ws.add(objB)
console.log(ws.has(objA)) // true
console.log(ws.lenghth()) // 2
ws.delete(objA)
console.log(ws.has(objA)) // false
JSR
MDN
184. What is WeakMap used for?
WeakMap is used to store key / value pairs where keys are weakly referenced. In other words, it serves as an additional repository for keys used by other code. Such keys are automatically removed by the garbage collector when they become unreachable (unused), i.e. when only the key remains in the WeakMap.
const wm = new WeakMap()
let user = {}
wm.set(user, 'user')
console.log(wm.has(user)) // true
user = null
console.log(wm.has(user)) // false,
JSR
MDN
185. What is the difference between Map and WeakMap?
Their main difference is that the keys stored in the WeakMap are weakly referenced, i.e. are automatically deleted as soon as they become unreachable. Other differences are as follows:
- Any values ββcan be used as keys in Map, and only objects can be used in WeakMap
- WeakMap has no size property
- WeakMap does not have clear (), keys (), values ββ(), entries (), forEach () methods
- WeakMap is not an iterable entity
JSR
MDN
186. What methods are available in WeakMap?
WeakMap has the following methods:
- set (): adds a key / value pair to an object
- delete (): deletes a value by key
- has (): determines if a value is present by key
- get (): returns the value by key
const wm = new WeakMap()
const firstUser = {}
const secondUser = {}
wm.set(firstUser, '')
wm.set(secondUser, '')
console.log(wm.has(firstUser)) // true
console.log(wm.get(firstUser)) //
wm.delete(secondUser)
console.log(wm.has(secondUser)) // false
JSR
MDN
187. How to encode a URL?
You can use the encodeURI () method for this. This method converts all special characters except / ?: @ = + $ #
const url = 'https://ru.wikipedia.org/wiki/,__'
const encoded = encodeURI(url)
console.log(encoded) // https://ru.wikipedia.org/wiki/%D0%9B%D0...
JSR
MDN
188. How to decode a URL?
You can use the decodeURI () method for this:
const url = 'https://ru.wikipedia.org/wiki/%D0%9B%D0...'
const decoded = decodeURI(url)
console.log(decoded) // https://ru.wikipedia.org/wiki/,__
JSR
MDN
189. How to print the page content?
You can use the global print () method for this. This method opens a special dialog box with print settings:
<button></button>
document.querySelector('button')
.addEventListener('click', () => print())
MDN
190. What is an anonymous function?
An anonymous function is an unnamed function. Such functions are often assigned to variables and also used as callbacks:
const sayHi = function () {
console.log('')
}
sayHi() //
//
const sayBye = () => console.log('')
sayBye() //
window.addEventListener('click', function () {
console.log(' , ')
})
//
window.addEventListener('contextmenu', e => {
e.preventDefault()
console.log(' - ')
})
JSR
MDN
191. What is the priority of using local and global variables?
Local variables take precedence over globals of the same name:
let question = ' '
function toBe () {
question = ''
console.log(question)
}
toBe() //
JSR
MDN
192. What are accessors?
Accessors or computed properties are getters and setters. Getters are used to get the values ββof an object's properties, and setters are used to set them:
class User {
constructor (name, age) {
this.name = name
this.age = age
}
#access = false
get access () {
return this.#access
}
set access (bool) {
this.#access = bool
}
}
const user = new User('', 30)
console.log(user.#access) // SyntaxError: Private field '#access' must be declared in an enclosing class
console.log(user.access) // false
user.access = true
console.log(user.access) // true
Getters and setters are properties, and methods are functions:
class User {
constructor (name, age) {
this.name = name
this.age = age
}
#access = false
getAccess () {
return this.#access
}
setAccess(bool) {
this.#access = bool
}
}
const user = new User('', 30)
console.log(user.#access) // SyntaxError: Private field '#access' must be declared in an enclosing class
console.log(user.getAccess()) // false
user.setAccess(true)
console.log(user.getAccess()) // true
JSR
193. How to define a property in an object constructor?
The Object.defineProperty () method can be used for this. This method allows you to add new properties to the object and modify existing ones, as well as change the settings for accessing the object:
'use strict'
const obj = {}
Object.defineProperty(obj, 'prop', {
value: 1,
writable: false
})
console.log(obj.prop) // 1
obj.prop = 2 // TypeError: Cannot assign to read only property 'prop' of object '#<Object>'
In lax mode, an attempt to change a read-only property will silently fail.
JSR
MDN
194. What are the features of getters and setters?
The main features of getters and setters are as follows:
- They have a simpler syntax compared to methods.
- Used to define computed properties - accessors
- Enables the same relationship between properties and methods
- Can provide better data quality
- Allows you to perform tasks behind the scenes when it comes to encapsulation
JSR
195. Can getters and setters be added to an object using the Object.defineProperty () method?
Quite:
const obj = {counter: 0}
Object.defineProperty(obj, 'increment', {
get() {return ++this.counter}
})
Object.defineProperty(obj, 'decrement', {
get() {return --this.counter}
})
Object.defineProperty(obj, 'sum', {
set(val) {return this.counter += val}
})
Object.defineProperty(obj, 'sub', {
set(val) {return this.counter -= val}
})
obj.sum = 10
obj.sub = 5
console.log(obj) // {counter: 5}
console.log(obj.increment) // 6
console.log(obj.decrement) // 5
JSR
MDN
196. What is switch ... case used for?
switch ... case is an alternative to if ... else and is a more visual way of executing code depending on the passed condition:
const calc = (x, y, operator) => {
let result
try {
switch (operator) {
case '+':
result = x + y
break
case '-':
result = x - y
break
case '*':
result = x * y
break
case '/':
result = x / y
break
default:
throw new Error(' ')
}
if (isNaN(result)) {
throw new Error(' ')
}
console.log(result)
return result
} catch (e) {
console.error(e.message)
}
}
calc(1, 2, '+') // 3
calc(3, 4, '*') // 12
calc('a', 1, '-') //
calc(5, 6, 'x') //
JSR
MDN
197. Name the rules for using switch ... case
When using the switch ... case construction, you must adhere to the following rules:
- the condition can be a number or a string
- duplicate values ββare not allowed
- the default statement is optional. If no case is found, the default block is executed
- break is used to stop the loop
- break is also optional, but without it, loop execution will continue
JSR
MDN
198. Name the primitive data types.
The primitive data types ("primitives") in JavaScript are the following values:
- number : , Β±253
- bigint
- string . ,
- boolean true/false
- null β , null
- undefined β , undefined
- symbol
MDN
Habr β JavaScript?
Habr β JavaScript ?
JavaScript ?
Medium β Advanced JavaScript ES6 β Temporal Dead Zone, Default Parameters And Let vs Var β Deep dive!
JavaScript:
Μ JavaScript
Medium β JavaScript.
Habr β JavaScript
Medium β Understanding Prototypes in JavaScript
Medium β apply(), call() bind(), JavaScript
JavaScript:
Medium β JavaScript Classes: An In-Depth look (Part 1)
Medium β JavaScript slice(), splice() split()
JavaScript
JavaScript- .map(), .filter() .reduce()
reduce() JavaScript
Μ Μ ECMAScript 2019, for-of
ES2020,
DigitalOcean β map set JavaScript
Medium β JavaScript
Medium β What is Memoization in Javascript?
Redd β Debounce vs Throttle: Definitive Visual Guide
JavaScript
Habr β 5 JSON.stringify()
Habr β () «» JavaScript
Habr β JavaScript- : . 1
Habr β -
GoogleDevelopers β Service Workers: an Introduction
Habr β
WebDevBlog β IndexedDB
GoogleDevelopers β Working with IndexedDB
Habr β Web Storage API:
Habr β -
Medium β A Simple Introduction to Web Workers in JavaScript
Habr β JavaScript,
Habr β Async/Await
Habr β CORS: