The necessary information on the NXOpen library can be found on the official website .
The NX root folder contains the default libraries we need, as well as examples:
- C: \ Program Files \ Siemens \ NX 12.0 \ NXBIN with jar extension
- C: \ Program Files \ Siemens \ NX 12.0 \ UGOPEN with jar extension
- C: \ Program Files \ Siemens \ NX 12.0 \ UGOPEN \ SampleNXOpenApplications \ Java.
To simplify writing code, you can use the log of your actions to a text file as a basis. By default, Unigraphics NX writes in Visual Basic, but in the settings you can change it to Java or any other from the list of available ones:
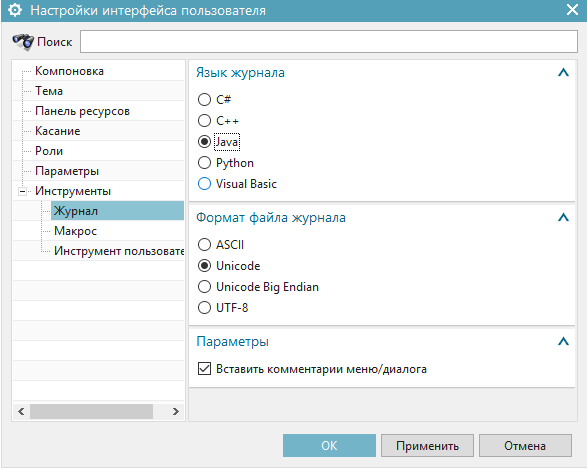
Here is an example of logging to a text file.
Code Listing
// NX 12.0.1.7
// Journal created by Aleksandr on Sun Nov 3 19:49:32 2019 RTZ 2 ()
//
import nxopen.*;
public class journalTT
{
public static void main(String [] args) throws NXException, java.rmi.RemoteException
{
nxopen.Session theSession = (nxopen.Session)nxopen.SessionFactory.get("Session");
nxopen.cae.FemPart workFemPart = ((nxopen.cae.FemPart)theSession.parts().baseWork());
nxopen.cae.FemPart displayFemPart = ((nxopen.cae.FemPart)theSession.parts().baseDisplay());
// ----------------------------------------------
// : ->->2D ...
// ----------------------------------------------
int markId1;
markId1 = theSession.setUndoMark(nxopen.Session.MarkVisibility.VISIBLE, "");
nxopen.cae.FEModel fEModel1 = ((nxopen.cae.FEModel)workFemPart.findObject("FEModel"));
nxopen.cae.MeshManager meshManager1 = ((nxopen.cae.MeshManager)fEModel1.find("MeshManager"));
nxopen.cae.Mesh2d nullNXOpen_CAE_Mesh2d = null;
nxopen.cae.Mesh2dBuilder mesh2dBuilder1;
mesh2dBuilder1 = meshManager1.createMesh2dBuilder(nullNXOpen_CAE_Mesh2d);
nxopen.cae.MeshCollector nullNXOpen_CAE_MeshCollector = null;
mesh2dBuilder1.elementType().destinationCollector().setElementContainer(nullNXOpen_CAE_MeshCollector);
mesh2dBuilder1.elementType().setElementTypeName("CQUAD4");
nxopen.Unit unit1 = ((nxopen.Unit)workFemPart.unitCollection().findObject("MilliMeter"));
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("quad mesh overall edge size", "10", unit1);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("target minimum element edge length", false);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("surface curvature threshold", "5.05", unit1);
nxopen.Unit nullNXOpen_Unit = null;
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("small feature value", "1", nullNXOpen_Unit);
theSession.setUndoMarkName(markId1, " 2D ");
nxopen.DisplayableObject [] objects1 = new nxopen.DisplayableObject[1];
nxopen.cae.CAEBody cAEBody1 = ((nxopen.cae.CAEBody)workFemPart.findObject("CAE_Body(14)"));
nxopen.cae.CAEFace cAEFace1 = ((nxopen.cae.CAEFace)cAEBody1.findObject("CAE_Face(14)"));
objects1[0] = cAEFace1;
boolean added1;
added1 = mesh2dBuilder1.selectionList().add(objects1);
nxopen.DisplayableObject [] objects2 = new nxopen.DisplayableObject[1];
nxopen.cae.CAEBody cAEBody2 = ((nxopen.cae.CAEBody)workFemPart.findObject("CAE_Body(3)"));
nxopen.cae.CAEFace cAEFace2 = ((nxopen.cae.CAEFace)cAEBody2.findObject("CAE_Face(3)"));
objects2[0] = cAEFace2;
boolean added2;
added2 = mesh2dBuilder1.selectionList().add(objects2);
nxopen.DisplayableObject [] objects3 = new nxopen.DisplayableObject[1];
nxopen.cae.CAEBody cAEBody3 = ((nxopen.cae.CAEBody)workFemPart.findObject("CAE_Body(2)"));
nxopen.cae.CAEFace cAEFace3 = ((nxopen.cae.CAEFace)cAEBody3.findObject("CAE_Face(2)"));
objects3[0] = cAEFace3;
boolean added3;
added3 = mesh2dBuilder1.selectionList().add(objects3);
nxopen.DisplayableObject [] objects4 = new nxopen.DisplayableObject[1];
nxopen.cae.CAEBody cAEBody4 = ((nxopen.cae.CAEBody)workFemPart.findObject("CAE_Body(1)"));
nxopen.cae.CAEFace cAEFace4 = ((nxopen.cae.CAEFace)cAEBody4.findObject("CAE_Face(1)"));
objects4[0] = cAEFace4;
boolean added4;
added4 = mesh2dBuilder1.selectionList().add(objects4);
int markId2;
markId2 = theSession.setUndoMark(nxopen.Session.MarkVisibility.INVISIBLE, "2D ");
theSession.deleteUndoMark(markId2, null);
int markId3;
markId3 = theSession.setUndoMark(nxopen.Session.MarkVisibility.INVISIBLE, "2D ");
mesh2dBuilder1.setAutoResetOption(false);
mesh2dBuilder1.elementType().setElementDimension(nxopen.cae.ElementTypeBuilder.ElementType.SHELL);
mesh2dBuilder1.elementType().setElementTypeName("CQUAD4");
nxopen.cae.DestinationCollectorBuilder destinationCollectorBuilder1;
destinationCollectorBuilder1 = mesh2dBuilder1.elementType().destinationCollector();
destinationCollectorBuilder1.setElementContainer(nullNXOpen_CAE_MeshCollector);
destinationCollectorBuilder1.setAutomaticMode(true);
mesh2dBuilder1.propertyTable().setIntegerPropertyValue("meshing method", 0);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("quad mesh overall edge size", "10", unit1);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("block decomposition option bool", false);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("mapped mesh option bool", false);
mesh2dBuilder1.propertyTable().setIntegerPropertyValue("fillet num elements", 3);
mesh2dBuilder1.propertyTable().setIntegerPropertyValue("num elements on cylinder circumference", 6);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("element size on cylinder height", "1", unit1);
mesh2dBuilder1.propertyTable().setIntegerPropertyValue("quad only option", 0);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("mesh individual faces option bool", false);
mesh2dBuilder1.propertyTable().setIntegerPropertyValue("midnodes", 0);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("geometry tolerance option bool", false);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("geometry tolerance", "0", unit1);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("target maximum element edge length bool", false);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("maximum element edge length", "1", unit1);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("target minimum element edge length", false);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("split poor quads bool", false);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("move nodes off geometry bool", false);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("max quad warp option bool", false);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("max quad warp", "5", nullNXOpen_Unit);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("max jacobian", "5", nullNXOpen_Unit);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("target element skew bool", false);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("element skew", "30", nullNXOpen_Unit);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("max included angle quad option bool", false);
nxopen.Unit unit2 = ((nxopen.Unit)workFemPart.unitCollection().findObject("Degrees"));
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("max included angle quad", "150", unit2);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("min included angle quad option bool", false);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("min included angle quad", "30", unit2);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("max included angle tria option bool", false);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("max included angle tria", "150", unit2);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("min included angle tria option bool", false);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("min included angle tria", "30", unit2);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("mesh transition bool", false);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("mesh size variation", "50", nullNXOpen_Unit);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("surface curvature threshold", "5.05", unit1);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("alternate feature abstraction bool", false);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("minimum feature element size", "0.001", unit1);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("small feature tolerance", "10", nullNXOpen_Unit);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("small feature value", "1", nullNXOpen_Unit);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("suppress hole option bool", false);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("hole diameter tolerance", "0", unit1);
mesh2dBuilder1.propertyTable().setIntegerPropertyValue("hole suppresion point type", 0);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("merge edge toggle bool", false);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("edge angle", "15", unit2);
mesh2dBuilder1.propertyTable().setBooleanPropertyValue("quad mesh edge match toggle", false);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("quad mesh edge match tolerance", "0.02", unit1);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("quad mesh smoothness tolerance", "0.01", unit1);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("quad mesh surface match tolerance", "0.001", unit1);
mesh2dBuilder1.propertyTable().setIntegerPropertyValue("quad mesh transitional rows", 3);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("min face angle", "20", unit2);
mesh2dBuilder1.propertyTable().setIntegerPropertyValue("mesh time stamp", 0);
mesh2dBuilder1.propertyTable().setBaseScalarWithDataPropertyValue("quad mesh node coincidence tolerance", "0.0001", unit1);
mesh2dBuilder1.propertyTable().setIntegerPropertyValue("mesh edit allowed", 0);
mesh2dBuilder1.propertyTable().setIntegerPropertyValue("transition edge seeding", 0);
mesh2dBuilder1.propertyTable().setIntegerPropertyValue("cylinder curved end num elements", 6);
int id1;
id1 = theSession.newestVisibleUndoMark();
int nErrs1;
nErrs1 = theSession.updateManager().doUpdate(id1);
nxopen.cae.Mesh [] meshes1 ;
meshes1 = mesh2dBuilder1.commitMesh();
theSession.deleteUndoMark(markId3, null);
theSession.setUndoMarkName(id1, "2D ");
mesh2dBuilder1.destroy();
// ----------------------------------------------
// : -> ->3
// ----------------------------------------------
// ----------------------------------------------
// : ->->
// ----------------------------------------------
}
public static final int getUnloadOption() { return nxopen.BaseSession.LibraryUnloadOption.IMMEDIATELY; }
}
}
Now it builds a mesh only on those surfaces that are specified in the code, and if there are fewer or more of them, and their names differ, then nx will give an error when running our code. Therefore, we need to modify the code by adding try / catch exceptions to it and come up with a way so that we do not bind to the number of polygonal faces.
I did it like this:
try {
for (int i = 1; i < 20; i++) {
obj="object"+i;
cAEB="cAEBody"+i;
cAEF="cAEFace"+i;
adde="added"+i;
//Object objects = new Object();
nxopen.DisplayableObject [] obj = new nxopen.DisplayableObject[1];
nxopen.cae.CAEBody cAEB = ((nxopen.cae.CAEBody)workFemPart.findObject(("CAE_Body"+"("+i+")")));
nxopen.cae.CAEFace cAEF = ((nxopen.cae.CAEFace)cAEB.findObject("CAE_Face"+"("+i+")"));
obj[0] = cAEF;
boolean adde;
adde = mesh2dBuilder1.selectionList().add(obj);
}
} catch (Exception ex) {
Here is the final result in the program:
