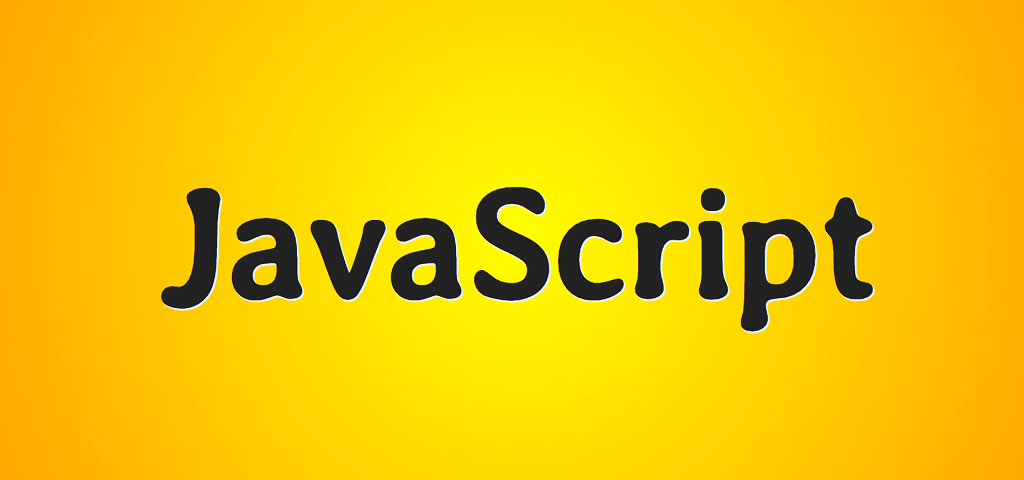
Good day, friends!
Here is a list of the top 100 JavaScript basics questions from this repository with short answers and links to Ilya Kantor's Modern JavaScript Tutorial (JSR) and MDN.
This list, along with 300+ practice questions, are available in my app .
The application implements a mechanism for memorizing the studied question, and also provides offline work.
I apologize for any possible errors and typographical errors. Any form of feedback is appreciated.
Edition from 14.09.
See the continuation here .
1. How to create an object?
There are several ways to do this. Some of them are:
Object literal:
const object = {}
Object constructor (not recommended):
const object = new Object()
Object.create ()
method When using this method, an object is passed to it as an argument, which will become the prototype of the new object.
// -
const object = Object.create(null)
Constructor
function We create a constructor function and use the "new" operator to create an instance of this function - an object:
function Person (name) {
const object = {}
object.name = name
object.age = 30
return object
}
const user = new Person('')
Class:
class Person {
constructor(name) {
this.name = name
}
}
const user = new Person('')
JSR
MDN
2. What are prototypes?
Prototypes are used to create new objects based on existing ones. This technique is called prototypal inheritance. The prototype of an instance of an object is available through Object.getPrototypeOf (object) or the __proto__ property ([[Prototype]] internal hidden property).

JSR
MDN
3. What is the difference between call (), apply () and bind ()?
The difference between these methods is easiest to explain with examples.
The call () method calls a function with the specified this value and the arguments separated by commas.
const employee1 = { firstName: '', lastName: '' }
const employee2 = { firstName: '', lastName: '' }
function invite (greet1, greet2) {
console.log(\`${greet1}, ${this.firstName} ${this.lastName}. ${greet2}\`)
}
invite.call(employee1, '', ' ?') // , . ?
invite.call(employee2, '', ' ?') // , . ?
The apply () method calls a function with the specified this value and array arguments.
const employee1 = { firstName: '', lastName: '' }
const employee2 = { firstName: '', lastName: '' }
function invite (greet1, greet2) {
console.log(\`${greet1}, ${this.firstName} ${this.lastName}. ${greet2}\`)
}
invite.apply(employee1, ['', ' ?']) // , . ?
invite.apply(employee2, ['', ' ?']) // , . ?
The bind () method returns a new function with the specified this value and allows you to pass an array or any number of arguments separated by commas to it.
const employee1 = { firstName: '', lastName: '' }
const employee2 = { firstName: '', lastName: '' }
function invite (greet1, greet2) {
console.log(\`${greet1}, ${this.firstName} ${this.lastName}. ${greet2}\`)
}
const inviteEmployee1 = invite.bind(employee1)
const inviteEmployee2 = invite.bind(employee2)
inviteEmployee1('', ' ?') // , . ?
inviteEmployee2('', ' ?') // , . ?
Thus, the call () and apply () methods call the function after it has been bound to an object. The difference between the two is the way the arguments are passed. This difference is easy to remember with the first letters of the methods: call is a comma (comma, c), apply is an array (array, a). The bind () method returns a new function bound to the specified object.
JSR - Call / Apply
JSR - Bind
MDN - Call
MDN - Apply
MDN - Bind
4. What is JSON and what methods does it have?
JSON is a textual data format based on JavaScript object syntax invented by Douglas Crockford. It is used to transfer data over the network and usually has the extension .json and the MIME type application / json.
Parsing: Converts a JSON string to an object.
JSON.parse(text)
Stringification: Converts an object to a JSON string for transmission over the network.
JSON.stringify(object)
JSR
MDN
5. What does the Array.slice () method do?
The slice () method returns the selected array elements as a new array. It returns items starting at the index specified in the first argument and ending with, but not including, the index specified in the second optional argument. If the second argument is absent, then all elements starting at the index specified in the first argument will be retrieved.
const arrayIntegers = [1, 2, 3, 4, 5]
const arrayIntegers1 = arrayIntegers.slice(0, 2) // [1, 2]
const arrayIntegers2 = arrayIntegers.slice(2, 3) // [3]
const arrayIntegers3 = arrayIntegers.slice(4) // [5]
Note that this method does not modify the original array, but only returns a subset of it as a new array.
JSR
MDN
6. What does the Array.splice () method do?
The splice () method is used to add or remove elements to or from an array. The first argument specifies the starting position for adding or removing elements, the second optional argument specifies the number of elements to remove. Each subsequent argument (third, etc.) is added to the array:
let arrayOriginal1 = [1, 2, 3, 4, 5]
let arrayOriginal2 = [1, 2, 3, 4, 5]
let arrayOriginal3 = [1, 2, 3, 4, 5]
let array1 = arrayOriginal1.splice(0, 2) // [1, 2]; = [3, 4, 5]
let array2 = arrayOriginal2.slice(3) // [4, 5]; = [1, 2, 3]
let array3 = arrayOriginal3.slice(3, 1, 'a', 'b', 'c') // [4]; = [1, 2, 3, 'a', 'b', 'c']
Note that the splice () method modifies the original array and returns an array of the extracted elements.
JSR
MDN
7. What is the difference between slice () and splice ()?
The main differences are as follows:
Slice | Splice |
---|---|
Doesn't change the original array | Modifies the original array |
Returns a subarray of the original array | Returns the removed elements as an array |
Used to retrieve elements from an array | Serves for adding / removing elements to / from an array |
JSR
MDN - Slice
MDN - Splice
8. How do objects and maps compare?
Objects are similar to maps in that they both allow you to set keys on values, retrieve values, delete keys, and determine if a value is present by key. For this reason, objects used to be used as maps. However, there are some differences between them that make the use of cards more preferable in certain cases.
- Object keys can only be strings and symbols, and map keys can be any values, including functions and objects
- Map keys are ordered, but object keys are not. So when iterating, the map keys are returned in the order they were added
- You can get the size of the map using the size property and the number of object properties is manually defined
- A map is an iterable entity, and to iterate over an object, you must first get its keys somehow, and then iterate over them
- When using an object as a map, keep in mind that any object has a prototype, so the map's own keys may overlap with user-defined keys. Therefore, Object.create (null) should be used to create a map object, but now this method is rarely used.
- Object is inferior to the map in terms of performance when it comes to quickly adding / removing keys
JSR
MDN
9. What is the difference between the "==" and "===" operators?
JavaScript provides two ways to compare values: strict (===,! ==) and abstract (==,! ==). In a strict comparison, the values ββare compared as they are, and in a lax comparison, if necessary, an implicit conversion (casting) of value types is performed. Strict operators use the following rules for comparing different types of values:
- Two strings are strictly equal when they have the same character set, the same length, and the same characters at the same positions
- Two numbers are strictly equal if their values ββare equal. There are two special cases:
- NaN equals nothing, including NaN
- Positive and negative zeros are equal to each other
- Boolean values ββare strictly equal when both are true or false, i.e. true or false
- Two objects are strictly equal if they refer to the same object (memory location)
- null === undefined returns false and null == undefined returns true
A few examples:
0 == false // true
0 === false // false
1 == "1" // true
1 === "1" // false
null == undefined // true
null === undefined // false
'0' == false // true
'0' === false // false
[] == [] //
[] === [] // false,
{} == {} //
{} === {} // false,
JSR
MDN
10. What are lambda or arrow functions?
Arrow functions are a shorthand way of writing functional expressions. They don't have their own this, arguments, super and new.target. These functions serve as a good alternative to functions that have no methods, but cannot be used as constructors.
function regularSum (x, y) {
return x + y
}
const arrowSum = (x, y) => x + y
JSR
MDN
11. Why are functions called first-class objects?
In JavaScript, functions are first class objects. This means that functions can be used like regular variables.
For example, a function can be passed as an argument to another function, returned as a value from another function, and assigned to a variable. The following example assigns a function to a handler:
const handler = () => console.log(' - ')
document.addEventListener('click', handler)
JSR
MDN
12. What is a first-order function?
A first-order function is a function that does not take another function as an argument and does not return a function as a value:
const firstOrder = () => console.log(' - ')
JSR
MDN
13. What is a higher order function?
A higher-order function is a function that takes another function as an argument, or returns another function as a value:
const firstOrderFun = () => console.log(' - ')
const higherOrder = returnFirstOrderFun => returnFirstOrderFun()
higherOrder(firstOrderFunc)
JSR
MDN
14. What is a unary function?
A unary function (monad function) is a function that takes only one argument:
const unaryFun = a => console.log(a + 10) // 10
JSR
MDN
15. What is currying?
Currying is the process of converting a function with multiple parameters into multiple functions with one parameter. This process is named after the mathematician Haskell Curry. Currying turns one n-ary function into several unary functions (reduces the arity of the function):
const sum = (a, b, c) => a + b + c
const currySum = a => b => c => a + b + c
currySum(1) // : b => c => 1 + b + c
currySum(1)(2) // : c => 3 + c
currySum(1)(2)(3) // 6
Currying is used to enable code reuse (partial function application) and to create a composition of functions.
JSR
16. What is a pure function?
A pure function is a function whose return value depends only on the arguments passed, without side effects. Simply put, if you call a function n times with n arguments, and the function always returns the same value, then it is clean:
//
let numberArray = []
const impureAddNumber = number => numberArray.push(number)
//
const pureAddNumber = number => argNumberArray => argNumberArray.concat([number])
console.log(impureAddNumber(1)) // 1
console.log(numberArray) // [1]
console.log(pureAddNumber(2)(numberArray)) // [1, 2]
console.log(numberArray) // [1]
In the above example, impureAddNumber is not a pure function because the push () method returns the new length of the array, which is independent of the argument passed. The second function is pure because the concat () method concatenates the two arrays without side effects and returns a new array. Pure functions are essential for unit testing and don't need dependency injection. The absence of side effects improves the reliability of the application due to the weaker connections between its elements. One of the incarnations of this principle is the concept of immutability, introduced in ES6, which prefers const over let.
JSR
MDN
17. What is the "let" keyword used for?
The let keyword declares a block-scoped local variable. The scope of such a variable is limited by the block, operator or expression in which it is used. Variables declared with the "var" keyword have the global or function scope in which they are defined:
let counter = 1
if (counter === 1) {
let counter = 2
console.log(counter) // 2
}
console.log(counter) // 1 ( counter, , )
JSR
MDN
18. What is the difference between let and var?
The main differences are as follows:
var | let |
---|---|
Available since JavaScript | Introduced in ES6 |
Has global or functional scope | Has block scope |
Variables are hoisted to the beginning of the scope | Variables are also hoisted, but not initialized (only declaration is hoisted, not value assignment) |
A few examples:
function userDetails(username) {
if (username) {
console.log(salary)
console.log(age)
let age = 30
var salary = 10000
}
console.log(salary) // 10000 ( )
console.log(age) // SyntaxError: "age" is not defined ( )
}
JSR
MDN - let
MDN - var
19. Why was the word "let" chosen as the keyword?
Let is a mathematical operator that was used by early programming languages ββsuch as Scheme and Basic. Nowadays let is used by a large number of programming languages, so this word is the closest alternative to the abbreviation "var" (variable).
JSR
MDN
20. How to override a variable in a switch block?
If you try to override a variable declared with the "let" keyword in a switch block, you will get an error:
let counter = 1
switch(x) {
case 0:
let name
break
case 1:
let name // SyntaxError
break
}
To solve this problem, you need to create a new block inside the case - a new lexical scope:
let counter = 1
switch(x) {
case 0: {
let name
break
}
case 1: {
let name
break
}
}
JSR
MDN
21. What is a temporary dead zone?
If you try to access variables declared with the keyword "let" or "const" (but not "var") before they are defined (that is, before they are assigned a value within the current scope), a ReferenceError exception will be thrown. ). In other words, the time dead zone is the time between the creation of the context (scope) of the variable and its definition:
function someMethod () {
console.log(counter1) // undefined
console.log(counter2) // ReferenceError
var counter1 = 1
const counter2 = 2
}
MDN
22. What is an Immediately Invoked Function Expression (IIFE)?
IIFE is a function that is called immediately after definition. The syntax for such a function may look like this (one of the options, the most common):
(function () {
//
})()
// ,
(() => {
//
})()
The main reason for using an IIFE is to keep variables private, since variables declared inside the IIFE cannot be accessed from the outside environment:
(function () {
var message = 'IIFE'
console.log(message)
})()
console.log(message) // SyntaxError: "message" is not defined
JSR
MDN
23. What are the benefits of using modules?
Among others, the following can be mentioned:
- Improving readability and easier code maintenance
- Reusable code
- Keeping the Global Namespace Clean
JSR
MDN
24. What is memorization or memoization?
Memoization is a way to improve the performance of a function by storing previously obtained results of that function in the cache. Each time the function is called, the argument passed to it becomes the cache index. If the data is in the cache, it is returned without re-executing the function. Otherwise, the function is executed and the result is written to the cache:
const memoAdd = () => {
let cache = {}
return value => {
if (value in cache) {
console.log(' ')
return cache[value] // , cache.value , JavaScript .
} else {
console.log(' ')
let result = value + 20
cache[value] = result
return result
}
}
}
// memoAdd
const add = memoAdd()
console.log(add(20)) // 40
console.log(add(20)) // 40
25. What is hoisting?
Hoisting is the process of moving variables and function expressions to the beginning of their scope before executing code. Remember: only the variables and expressions themselves are hoisted, not their initialization (i.e. the variable declaration is hoisted, not the assignment of a value to it):
console.log(message) // undefined
var message = '!'
For the interpreter, this code looks like this:
var message
console.log(message)
message = '!'
JSR
MDN
26. What is a class?
Classes introduced in ES6 are syntactic sugar (wrapper, abstraction, or add-on) for prototype inheritance (for constructor function prototype). Example of a constructor function:
function Bike(model, color) {
this.model = model
this.color = color
}
Bike.prototype.getDetails = function () {
return ' ' + this.model + ' ' + this.color + ' .'
}
Same example using class:
class Bike {
constructor (color, model) {
this.color = color
this.model = model
}
getDetails () {
return \` ${this.model} ${this.color} .\`
}
}
JSR
MDN
27. What is a closure?
A closure is a combination of a function and its lexical environment. Simply put, a closure is when an inner function has access to variables declared in an outer function. A closure has a chain of three scopes:
- Own scope
- External function scope
- Global scope
const welcome = name => {
const greet = message => {
console.log(\`${message}, ${name}!\`)
}
return greet
}
const fun = welcome('')
fun('') // , !
JSR
MDN
28. What is a module?
Modules are small pieces of independent, reusable code that underlie many design patterns. Most modules are exported as objects, functions, or constructors.
JSR
MDN
29. What is scope?
Scope defines the availability of variables, functions and objects in different places in the code during its execution. In other words, scope is the visibility of variables and other resources in the current context of code execution.
MDN
30. What is a service worker?
A service worker is a script that runs independently of the web page on which it runs and the user's actions. In fact, the service worker acts as a proxy server between the application and the browser. The main features of service workers are the following: ensuring the application is offline, periodic background synchronization, push notifications, intercepting and processing network requests, and programmatically managing the cache.
MDN
31. How do you interact with the Document Object Model (DOM) using service workers?
Service workers do not have direct access to the DOM. However, they can interact with the page through the postMessage interface, and the page can modify the DOM.
MDN - ServiceWorker
MDN - postMessage
32. How to reuse information when restarting a service worker?
One of the problems with service workers is that they stop executing when they are not in use and restart when needed. This prevents you from adding fetch and message event handlers globally. To reuse information, it is necessary to ensure that service workers interact with an indexed database (IndexedDB) or local storage (local storage).
MDN
33. What is an indexed database (IndexedDB)?
IndexedDB is a low-level API for storing large amounts of structured data, including files and blobs, on the client side. This interface uses indexes to improve the speed of data retrieval.
JSR
MDN
34. What is Web Storage?
Web storage is an interface that allows you to store data as key / value pairs locally, i.e. in the user's browser, in a more convenient way than using cookies. Web storage provides two storage mechanisms:
- Local storage (local stotage) - stores the data of the current user for an unlimited amount of time
- Session storage - stores data throughout the current session, i.e. when closing the browser tab, the data will be lost
JSR
MDN
35. What is postMessage?
postMessage is a way of communicating between different sources of a window object (for example, a page and the popup it generates (a pop-up window) or a page and an embedded iframe). Typically, scripts on one page do not have access to another page if that page follows the Common Origin or Single Source Policy (source - protocol, host and port).
MDN - postMessage
36. What are cookies?
A cookie is a small piece of data that is stored on a user's computer for later use by a browser. Cookies are stored as key / value pairs:
document.cookie = 'username='
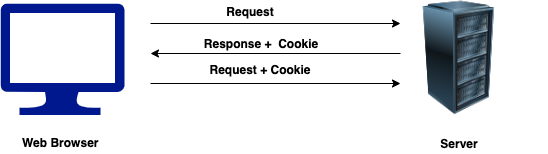
JSR
MDN
37. Why do we need cookies?
Cookies are used to save information about the user (it is not recommended to use them to store confidential information). Usually, this process consists of two stages:
- On the first visit to the page, the user profile is saved in a cookie
- On revisiting the page, the user profile is retrieved from the cookie
JSR
MDN
38. What are the capabilities of cookies?
By default, cookies are deleted when the browser is closed, however this can be changed by setting the expires in UTC:
document.cookie = 'username=; expires=Sat, 5 Sep 2020 12:00:00 UTC'
By default, cookies belong to the current page, however this can also be changed by setting the path:
document.cookie = 'username=; path=/services'
JSR
MDN
39. How do I delete cookies?
You can delete cookies by setting the elapsed time as the lifetime. In this case, you do not need to define a cookie value:
document.cookie = 'username=; expires=Sat, 05 Jun 2020 00:00:00 UTC; path=/;'
Please note that in this case it is necessary to determine the path to delete the correct cookie. Some browsers do not allow you to delete cookies without specifying this parameter.
JSR
MDN
40. What is the difference between cookies, local storage and session storage?
The main differences are as follows:
Criterion | Cookies | Local storage | Session storage |
---|---|---|---|
Availability | Both on the server and on the client | Client only | Client only |
Lifetime | Installed with expires | Until user deletes | Until the browser tab is closed |
Encryption support | Supported | Not supported | Not supported |
Maximum data size | 4 Kb | About 5 MB (depending on the browser) | About 5 MB (depending on the browser) |
JSR - Cookies
MDN - Cookie
JSR - LocalStorage, SessionStotage
MDN - Web Storage
41. What is the main difference between local and session storage?
Local storage is the same as session storage, except that in the first, the data is saved even when you close and restart the browser, and in the second, the data is deleted at the end of the session (closing the browser tab).
JSR
MDN
42. How do I access the web storage?
The window object provides WindowLocalStorage and WindowSessionStorage objects that have localStorage and sessionStorage properties, respectively. These properties create an instance of a Storage object, with which you can write, retrieve, and delete data for a specific domain and storage type (session or local):
//
localStorage.setItem('data', document.querySelector('.data').value)
//
localStorage.getItem('data')
JSR
MDN
43. What methods does session storage provide?
Session storage provides methods for reading, writing and deleting data:
//
sessionStorage.setItem('key', 'value')
//
const data = sessionStorage.getItem('key')
//
sessionStorage.removeItem('key')
//
sessionStorage.clear()
JSR
MDN
44. What event occurs when working with the web storage?
When the storage changes in the context of another document, the storage event is raised:
window.onstorage = function () {}
An example of handling this event:
window.onstorage = event => {
console.log(\`${event.key} .
: ${event.oldValue}.
: ${event.newValue}.\`)
}
This event allows you to implement a kind of chat.
JSR
MDN
45. What is web storage used for?
Web storage is more secure and can store more data than cookies without affecting performance. In addition, no data is sent to the server (in the case of cookies, the data is included in the request and response headers each time the client accesses the server). Therefore, this way of storing data is preferable to cookies.
JSR
MDN
46. ββHow do I determine if a browser supports Web Storage?
Before using web storage, it is recommended to check the browser support for this interface:
if (typeof(Storage) !== 'undefined') {
//
} else {
// -
}
//
if ('Storage' in window) {
console.log('ok')
} else {
console.warn(' ok')
}
According to CanIUse , web storage support is 98% today.
JSR
MDN
47. How do I determine if a browser supports service workers?
Before using service workers, it is recommended to check browser support for this interface:
if (typeof(Worker) !== undefined) {
//
} else {
// -
}
//
if ('Worker' in window) {
console.log('ok')
} else {
console.warn(' ok')
}
According to CanIUse , service worker support is 94% today.
MDN
48. Give an example of a web worker
To use the web worker, you need to do the following.
Create a file for the worker, for example get-current-time.js:
const getCurrentTime = () => {
let time = new Date().toLocaleTimeString()
postMessage(time)
setTimeout(() => getCurrentTime(), 1000)
}
getCurrentTime()
The postMessage () method is used to send messages to the page.
Create a worker object:
const worker = new Worker('get-current-time.js')
After that, we process the receipt of messages from the worker:
<output></output>
<button></button>
worker
.addEventListener('message', event => document.querySelector('output')
.textContent = event.data)
The worker will continue to process the message event even after the external script has completed its work, so it must be forcedly stopped:
document.querySelector('button')
.addEventListener('click', () => worker.terminate())
If you set the worker to undefined, you can reuse it:
worker = undefined
MDN
49. What are the limitations of web workers for working with the DOM
Since web workers are created in a separate file, they do not have access to the following objects:
- window
- Document
- Parent object - the object that started the worker
MDN
50. What is a promise?
A promise (communication) is an object that is either executed with some value or rejected with an error. Promises are resolved either after a certain time has elapsed or after a certain event occurs. A promise can have one of three states: pending, fulfilled, and rejected.
Promise syntax:
const promise = new Promise((resolve, reject) => {
//
})
// , ,
const promise = Promise.resolve(value)
promise.then(value => {
//
})
An example of using a promise:
const promise = new Promise(resolve => {
const timer = setTimeout(() => {
resolve(' !')
clearTimeout(timer)
}, 5000);
}, reject => {
reject('- ')
})
promise
.then(value => console.log(value))
.catch(error => console.error(error))
.finally(() => console.log(' ')) // " !" 5 " "
Promise Resolution Algorithm:

JSR
MDN
51. Why are promises needed?
Promises are used to work with asynchronous code. They are an alternative to callback functions, avoiding the so-called "callback hell", making the code cleaner and more readable.
JSR
MDN
52. Name three possible states of a promise
Promises have three states:
- Pending: the stage before starting the operation
- Fulfilled: operation completed successfully
- Rejected: The operation failed. Exception is thrown
JSR
MDN
53. What is a callback function?
A callback is a function that is passed to another function as an argument. This function (internal) is called within the parent (external) to perform an operation when a specific event occurs. Let's look at a simple example:
function callback(name) {
alert(\`, ${name}!\`)
}
function outer(cb) {
const name = prompt(', ')
cb(name)
}
outer(callback)
In the above example, the outer function asks for the user's name and stores it in the name variable. This function then passes name to the callback function, which outputs a greeting with the user's name.
JSR
MDN
54. Why do we need callbacks?
Callbacks are needed because JavaScript is an event-driven language. This means that, for example, instead of waiting for a response to a request or for a particular event to be processed, JavaScript continues to respond to other events. Consider an example in which one function accesses the interface and the other prints a message to the console:
function first () {
// API
setTimeout(() => console.log(' '), 1000)
}
function second () {
console.log(' ')
}
first()
second()
// " ", " "
As you can see, JavaScript does not wait for the first function to complete, but continues to execute the code. Therefore, callbacks are used to simulate asynchrony, preventing blocking of the main program thread.
JSR
MDN
55. What is callback hell?
Callback hell is an anti-pattern where multiple callback functions are nested within each other to implement asynchronous logic. This code structure is difficult to understand and maintain. It might look like this:
function first () {
return function second () {
return function third () {
return function fourth () {
// ..
}
}
}
}
This approach to coding is considered bad practice, except in cases of currying, partial application, or composition of functions.
JSR
MDN
56. What are server-sent events (SSE)?
Server Sent Events is a push notification technology that allows browsers to receive updated data from the server over an HTTP connection without sending a request. This is one of the ways of communication between the client and the server, when messages are sent only by the server. This technology is used to update Facebook / Twitter, store prices, news feeds, etc.
JSR
MDN
57. How to receive messages (notifications or events) sent by the server?
The EventSource object is used for this:
if('EventSource' in window) {
const source = new EventSource('sse.js')
source.addEventListener('message', event => document.querySelector('output')
.textContent = event.data)
}
JSR
MDN
58. How can I check the browser support for SSE?
This is done like this:
if (typeof EventSource !== 'undefined') {
//
} else {
// SSE
}
//
('EventSource' in window)
? console.log('ok')
: console.warn('! ok')
According to CanIUse, 95% of browsers are currently supported by SSE.
JSR
MDN
59. What events occur when working with SSE?
Here is a list of these events:
Event | Description |
---|---|
open | Occurs when a connection to the server is opened |
message | Occurs when receiving a message from the server |
error | Thrown when an exception is thrown |
JSR
MDN
60. What are the basic rules for working with promises?
The basic rules for working with promises are as follows:
- A promise is an object containing a built-in or standard then () method
- The stage of waiting for a promise, usually ends with a stage of its fulfillment or rejection
- The status of a fulfilled or rejected promise should not change once it has been resolved
- After resolving a promise, its value should not change either
JSR
MDN
61. What is a callback in a callback?
You can nest callbacks within each other in order to sequentially perform certain operations:
loadScript('/script1.js', script => {
console.log(\` ${script} \`)
loadScript('/script2.js', script => {
console.log(\` ${script} \`)
loadScript('/script3.js', script => {
console.log(\` ${script} \`)
})
})
})
JSR
MDN
62. What is a promise chain?
Sequential execution of multiple asynchronous tasks using promises is called a promise chain. Let's consider an example:
new Promise((resolve, reject) => {
const id = setTimeout(() => {
resolve(1)
clearTimeout(id)
}, 1000)
}).then(result => {
console.log(result) // 1
return result * 2
}).then(result2 => {
console.log(result2) // 2
return result2 * 3
}).then(result3 => {
console.log(result3) // 6
}).catch(error => console.error(error))
Execution algorithm:
- The first promise resolves with a value of 1
- After that, the first then () method prints this value to the console and returns it multiplied by 2
- The second then () prints the result of the first then () to the console (2) and returns the result multiplied by 3
- The last then () prints the result of the second then () to the console (6)
- The catch block is used to handle errors
JSR
MDN
63. What is Promise.all ()?
Promise.all () is a promise that takes an array of other promises as an argument and returns the results of fulfilled promises or an error if one is rejected. Syntax:
Promise.all([Promise1, Promise2, Promise3])
.then(results => console.log(results))
.catch(error => console.error(error))
Remember, the order in which the results are fired depends on the order of the promises in the array.
JSR
MDN
64. What is Promise.race ()?
Promise.race () returns the result of the first fulfilled or rejected promise passed as an array of promises:
const promise1 = new Promise((resolve, reject) => setTimeout(resolve, 500, ''))
const promise2 = new Promise((resolve, reject) => setTimeout(resolve, 100, ''))
Promise.race([promise1, promise2]).then(value => console.log(value)) // ""
JSR
MDN
65. What is strict regime?
To enable strict mode, use the 'use strict' (or 'use strict') statement at the beginning of all code or an individual function. Strict mode was introduced in ES5. In this mode, some actions are prohibited and more exceptions are thrown.
JSR
MDN
66. Why do you need a strict regime?
Strict mode allows you to write more secure code by preventing many errors from occurring. For example, it disallows the accidental creation of global variables (without the keyword, variable = value), assignment of a value to a read-only property, a property that can only be obtained with a getter, a non-existent property, and a non-existent variable or object. In non-strict mode, no exception is thrown in all of these cases.
JSR
MDN
67. How do I enable strict security?
Strict mode is enabled by the 'use strict' (or 'use strict') statement at the beginning of a code or function. Usually, this instruction is indicated at the very beginning of the script, i.e. in the global namespace:
'use strict'
x = 3.14 // ReferenceError: "x" is not defined
If 'use strict' is specified in a function, then strict mode applies only to that function:
x = 3.14 //
f() // ReferenceError: "y" is not defined
function f () {
'use strict'
y = 3.14
}
JSR
MDN
68. What is double negation used for?
Double negation (!!) converts the value to boolean. If the value is false, then false is returned, otherwise - true:
const x = 1
console.log(x) // 1
console.log(!!x) // true
const y = ''
console.log(y) // ''
console.log(!!str) // false
Note:!!! Is not a separate operator, but two operators! ..
MDN
JSR
69. What is the delete operator used for?
This operator is used to delete properties of objects and the values ββof these properties:
'use strict'
const user = {
name: '',
age: 30
}
delete user.age
console.log(user) // { name: "" }
delete user // SyntaxError: Delete of an unqualified identifier in strict mode
Note that in non-strict mode, an attempt to delete an object will silently fail.
Since an array is also an object, applying delete to an element of the array will delete its value and write undefined to it, i.e. the index of the deleted element of the array will be preserved and the length of the array will not change.
JSR
MDN
70. What is the typeof operator used for?
This operator is used to define the type of a variable or expression:
typeof 1 // number
typeof [] // object
typeof '' // string
typeof (1 + 2) // number
typeof null // object
typeof NaN // number
JSR
MDN
71. What is undefined?
undefined is the undefined (but not missing) standard value (default value) of a variable that has not been assigned a value, as well as an undeclared variable. This is one of the primitive data types:
let name
console.log(typeof name) // undefined
console.log(typeof age) // undefined
This value can be assigned to a variable explicitly:
user = undefined
JSR
MDN
72. What is null?
null is a value representing the absence of a value set explicitly. This is one of the primitive data types. Using null, you can remove the value of a variable:
const user = null
console.log(typeof user) // object
JSR
MDN
73. What is the difference between null and undefined?
The main differences are as follows:
Null | Undefined |
---|---|
Assigned as indicator of missing value | Is the default for variables that have not been assigned a value or for undeclared variables |
Type - object | Type - undefined |
Primitive type meaning null, no value, or no reference | Primitive type meaning no value has been assigned to a variable |
Indicates the absence of a variable value | Indicates the absence of a variable or its ambiguity |
JSR - undefined
JSR - null
MDN - undefined
MDN - null
74. What is eval?
The eval () function evaluates the string passed to it. A string can be an expression, a variable, one or more operators:
console.log(eval('1 + 2')) // 3
//
const curryCalc = x => operator => y =>
new Promise((resolve, reject) =>
resolve(eval(\`x${operator}y\`))
).then(
result => console.log(result),
error => console.error(' !')
)
curryCalc(1)('+')(2) // 3
curryCalc(4)('-')(3) // 1
curryCalc(5)('x')(6) // !
Not recommended for use.
JSR
MDN
75. How to access browser history?
Information about the history of movements between pages in the browser contains the history property of the window object. To go to the previous or next page, use the back (), next () or go () methods:
const goBack = () => {
history.back()
//
history.go(-1)
}
const goForward = () => history.forward()
MDN
76. What data types exist in JavaScript?
There are 8 main types in JavaScript:
- number for any number: integer or floating point, integer values ββare limited to Β± 2 53
- bigint for integers of arbitrary length
- string for strings. A string can contain one or more characters, there is no separate character type
- boolean for true / false
- null for unknown values ββ- a distinct type with a single null value
- undefined for unassigned values ββ- a separate type having one undefined value
- object for more complex data structures
- symbol for unique identifiers
JSR
MDN
77. What does isNaN () do?
The isNaN () function converts the value to a number and checks if it is NaN.
isNaN('hello') // true
isNaN(100) // false
A more robust version of this functionality is the Number.isNaN () method introduced in ES6.
JSR
MDN
78. What is the difference between undeclared and undefined variables?
The main differences are as follows:
Undeclared | Undefined |
---|---|
Do not exist in the program | Have been declared without being assigned a value |
An access attempt ends with an error | When trying to access it returns undefined |
Climbs (floats) to the beginning of the current scope | Also ascends, but without an assigned value, i.e. with the value undefined (only the declaration is hoisted, but not initialization) |
JSR
MDN
79. What are global variables?
In the browser, global functions and variables declared with the "var" keyword, or without the keyword (in lax mode), become properties of the global window object (does not work in modules). Such variables are accessible from anywhere in the program. It is not recommended to use global variables. If you need to create a global variable, it is better to do it explicitly:
window.currentUser = {
name: ''
}
//
globalThis.currentUser = {
name: ''
}
console.log(currentUser.name) //
JSR
80. What problems does the creation of global variables entail?
Creating global variables pollutes the global namespace, which can cause conflicts between variable names. It also makes it harder to debug and test your code.
JSR
81. What is NaN?
The global NaN property is a Not-a-Number value. More precisely, NaN indicates that the value is incorrect, but still a number. Therefore typeof NaN returns number.
parseInt('bla') // NaN
Math.sqrt(-1) // NaN
MDN
82. What does isFinite () do?
The global function isFinite () converts the argument to a number and returns true if it is an ordinary (finite) number, i.e. not NaN, Infinity (positive infinity), -Infinity (negative infinity). Otherwise, false is returned.
isFinite(Infinity) // false
isFinite(-Infinity) // false
isFinite(NaN) // false
isFinite(100) // true
There is also a Number.isFinite () method, which, unlike isFinite (), does not convert the argument to a number before checking.
MDN
JSR
83. What is event flow / propagation?
The flow of events (event propagation) is the order in which the event occurs on the page. When you click on an element nested within other elements, before the event reaches the target element, it will sequentially go through all of its ancestors, starting from the global window object. There are three stages of event propagation:
- Top to bottom - capture or dive stage
- Target stage
- Bottom up - the stage of ascent or ascent (not to be confused with raising variables - hoisting)
JSR
84. What is event bubbling?
Bubbling is the stage of propagation of an event, when the event is first registered on the target element, and then up the chain from the ancestors of this element to the topmost (outer) element - the global window object.
JSR
85. What is immersion or event capture?
Immersion is the stage in which an event is fired, when it first registers on the topmost (outer) element (the global window object) and then descends down the chain of ancestors to the target element.
JSR
86. How to submit a form for processing?
This can be done in different ways:
function submitForm() {
document.forms[0].submit()
}
form.onsubmit = function(event) {
event.preventDefault()
//
}
form.addEventListener('submit', event => {
event.preventDefault()
//
})
Any button on a form is submit by default, i.e. serves to submit a form.
JSR
MDN
87. How can I get information about the operating system?
This information is contained in the global navigator object. Some of this information can be obtained through its platform property:
console.log(navigator.platform)
MDN
88. What is the difference between DOMContentLoaded and load events?
The DOMContentLoaded event is raised when the original HTML document has been fully loaded and parsed without waiting for stylesheets, images, or frames to fully load. The load event fires after the page has fully loaded, including any additional resources.
JSR
MDN - DOMContentLoaded
MDN - load
89. What is the difference between native, host (owned by the code runtime) and custom objects?
Native objects are part of the language and are defined in the ECMAScript specification. Such objects are, for example, Number, String, Function, Object, Math, RegExp, Date, etc. Host objects are provided by the browser or another runtime such as Node.js. Such objects are, for example, window, document (DOM), XMLHttpRequest, Web API (call stack, task queue), etc. User objects are any objects created in code, for example, an object containing information about the user:
const user = {
name: '',
age: 30
}
JSR
MDN
90. What tools are used for postponing code?
Such means are:
- In-browser developer tools like Chrome DevTools
- Debugger expression
- Good old console.log ()
JSR
MDN - debugger
MDN - Console
91. What are the advantages and disadvantages of promises versus callbacks?
Benefits:
- Prevent the hell of callbacks
- Lets you execute asynchronous code sequentially using then ()
- Allow asynchronous code to execute in parallel using Promise.all ()
- Solves many callback problems (calling too late or too early, multiple calls instead of one, error hiding)
disadvantages
- Code gets harder to write
- To provide support for older browsers, a polyfill is needed (there are almost no such browsers left today)
JSR - Promises
JSR - MDN Callbacks
- Promise
MDN - Callback
92. What is the difference between an attribute and a property of an element?
When the browser loads the page, it parses the HTML and generates DOM objects from it. For element nodes, most standard HTML attributes automatically become properties of DOM objects. Those. an element's attribute is specified in the markup, and its property is specified in the DOM. For example, for a body tag with an id = "page" attribute, the DOM object will have a body.id = "page" property.
<input type="text" value=" !">
// : type value
const input = document.querySelector('input')
//
console.log(input.getAttribute('value'))
//
console.log(input.value)
//
input.setAttribute('value', ' !')
//
input.value = ' !'
Note that the ECMAScript specification also defines attributes for object properties β [[Value]], [[Writable]], [[Enumerable]], and [[Configurable]].
JSR
93. What is a same-origin policy (SOP)?
Shared origin (same source) policy blocks access to data from another source. The source is a combination of protocol, host, and port. By default, cross-origin resource sharing (CORS) is prohibited, i.e. data is provided only in response to a request from the same source in which it is located. This behavior can be changed using special HTTP headers.
JSR
MDN - SOP
MDN - CORS
94. What does void 0 do?
The void operator evaluates the passed expression and returns undefined. Usually, when we click on a link, the browser loads a new page or reloads the current one. You can avoid this by using the expression "void (0)":
<a href="javascript:void(0)" onclick="alert('!')"> !
</a>
Page reloads can also be prevented with a simple stub:
<a href="#"> </a>
// "#" URL
MDN
95. Is JavaScript a compiled or interpreted programming language?
JavaScript itself is an interpreted programming language. The engine parses the code, interprets each line and executes it. However, modern browsers use a technology called just-in-time (JIT compilation) where code is compiled (optimized) before execution. This increases the preparation time for code execution, but significantly speeds up the execution itself. For example, V8, the engine used in Chrome and Node.js, uses the Ignition interpreter to parse code and the TurboFan compiler to optimize the code.
JSR
MDN
96. Is JavaScript case sensitive?
Yes, JavaScript is case sensitive. Therefore, keywords, names of variables, functions and objects must be identical when using them. For example, const somename and const someName are different variables, typeof (1) is number, and typeOf 1 is ReferenceError: typeOf is not defined.
JSR
MDN
97. Are Java and JavaScript related?
No, they are two different programming languages. However, they both belong to object-oriented languages ββand, like many other languages, use similar syntax (if, else, for, switch, break, continue, etc.). Basically, Java to JavaScript is a marketing ploy.
JSR
MDN
98. What is an event?
An event is a browser reaction to a specific action. This action can be a user action, for example, clicking a button or entering text, loading a page, receiving a response to a request, etc. (The actions that trigger events are not necessarily user-specific). Events are logged for further processing.
button.onclick = () => alert('!')
input.addEventListener('change', function() {
p.textContent = this.value
})
window.onload = () => console.log(' ')
MDN
JSR
99. Who Invented JavaScript?
JavaScript was created by Brendan Eich during his time at Netscape Communications. The language was originally called Mocha, then it was renamed LiveScript and was intended for both client-side programming and server-side programming (where it should be called LiveWire).
JSR
MDN