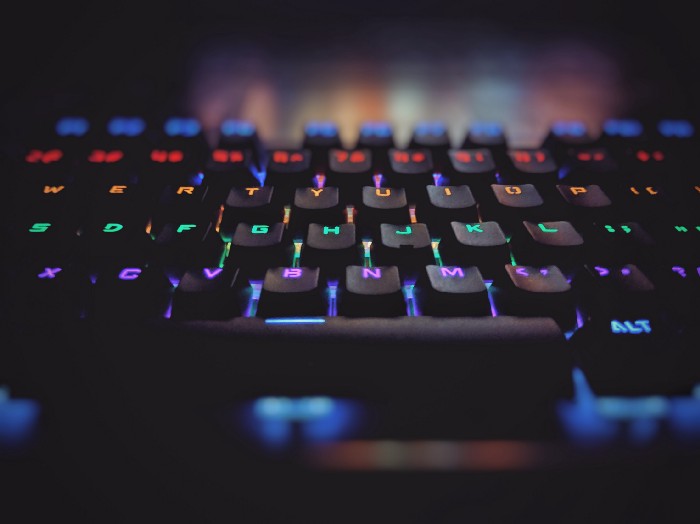
Photo from the Unsplash website . Author: Vipul Jha
Python is convenient in that it allows you to simultaneously return several values from a function at once. To do this, you need to use an operator
return
and return a data structure with several values - for example, a list of the total number of working hours for each week.
def hours_to_write(happy_hours):
week1 = happy_hours + 2
week2 = happy_hours + 4
week3 = happy_hours + 6
return [week1, week2, week3]
print(hours_to_write(4))
# [6, 8, 10]
Data structures in Python are used to store collections of data that can be returned by a statement
return
. In this article, we'll look at ways to return multiple values using similar structures (dictionaries, lists, and tuples), as well as using classes and data classes (Python 3.7+).
Method 1: return values using dictionaries
Dictionaries contain combinations of elements, which are key-value pairs (
key:value
), enclosed in curly braces ( {}
).
Dictionaries, in my opinion, are the best option for the job if you know the key to access the values. The following is a dictionary where the key is the person's name and the corresponding value is age.
people={
'Robin': 24,
'Odin': 26,
'David': 25
}
Now let's move on to the function that returns a dictionary with key-value pairs.
# A Python program to return multiple values using dictionary
# This function returns a dictionary
def people_age():
d = dict();
d['Jack'] = 30
d['Kim'] = 28
d['Bob'] = 27
return d
d = people_age()
print(d)
# {'Bob': 27, 'Jack': 30, 'Kim': 28}
Method 2: return values using lists
Lists are similar to arrays formed using square brackets, but they can contain different types of elements. Lists are also different from tuples because they are mutable data types. That is, any list can change .
Lists are one of the most versatile data structures in Python because they don't have to be uniform (they can include strings, numbers, and elements). Sometimes lists are even used in conjunction with stacks or queues.
# A Python program to return multiple values using list
def test():
str1 = "Happy"
str2 = "Coding"
return [str1, str2];
list = test()
print(list)
# ['Happy', 'Coding']
Here is an example that returns a list with natural numbers.
def natural_numbers(numbers = []):
for i in range(1, 16):
numbers.append(i)
return numbers
print(natural_numbers())
# [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15]
Method 3: return values using tuples
Tuples are ordered immutable objects in Python that are commonly used to store collections of heterogeneous data.
Tuples resemble lists, but they cannot be changed after they have been declared. Also, as a rule, tuples are faster to work than lists. You can create a tuple by separating the items with commas:
x, y, z
or (x, y, z)
.
In this example, a tuple is used to store data about an employee (name, work experience in years, and company name).
Bob = ("Bob", 7, "Google")
And here is an example of writing a function to return a tuple.
# A Python program to return multiple values using tuple
# This function returns a tuple
def fun():
str1 = "Happy"
str2 = "Coding"
return str1, str2; # we could also write (str1, str2)
str1, str2= fun()
print(str1)
print(str2)
# Happy
Coding
Note that we have omitted the parentheses in the statement
return
, since it is sufficient to simply separate each element with a comma to return a tuple (as shown above).
Don't forget that you can create a tuple using a comma instead of parentheses. Parentheses are only required when empty tuples are used or you need to avoid syntactic imprecision.
For a better understanding of tuples, refer to the official Python 3 documentation (the documentation is in English - Ed. ).
Below is an example of a function that uses parentheses to return a tuple.
def student(name, class):
return (name, class)
print(student("Brayan", 10))
# ('Brayan', 10)
Again, tuples are easy to confuse with lists (after all, both are a container of elements). However, there is a fundamental difference to keep in mind: tuples cannot be changed, but lists can.
Method 4: return values using objects
Everything is the same here as in C / C ++ or Java. You can simply form a class (called a struct in C) to store multiple traits and return an object of the class.
# A Python program to return multiple values using class
class Intro:
def __init__(self):
self.str1 = "hello"
self.str2 = "world"
# This function returns an object of Intro
def message():
return Intro()
x = message()
print(x.str1)
print(x.str2)
# hello
world
Method 5: returning values using data classes (Python 3.7+)
Data classes in Python 3.7+ help return a class with automatically added unique methods, a module,
typing
and other useful tools.
from dataclasses import dataclass
@dataclass
class Item_list:
name: str
perunit_cost: float
quantity_available: int = 0
def total_cost(self) -> float:
return self.perunit_cost * self.quantity_available
book = Item_list("better programming.", 50, 2)
x = book.total_cost()
print(x)
print(book)
# 100
Item_list(name='better programming.', perunit_cost=50,
quantity_available=2)
For a better understanding of data classes, refer to the official Python 3 documentation (documentation is in English - Ed. ).
Output
The purpose of this article is to familiarize you with ways to return multiple values from a function in Python. And, as you can see, there are really many of these ways.
Learn materiel and constantly develop your programming skills. Thank you for attention!