In the previous post, we figured out how to create a bot, initialized an instance of the class Bot
and got acquainted with the methods of sending messages using it.
In this article, I continue this topic, so I recommend starting reading this article only after reading the first part .
This time we will figure out how to revive our bot and add command support to it, as well as get to know the class Updater
.
In the course of the article, we will write several simple bots, the latter will, according to a given date and country code, determine whether a day in a given country is a weekend or a work day according to the production calendar. But, as before, the purpose of this article is to familiarize you with the package interface telegram.bot
for solving your own problems.
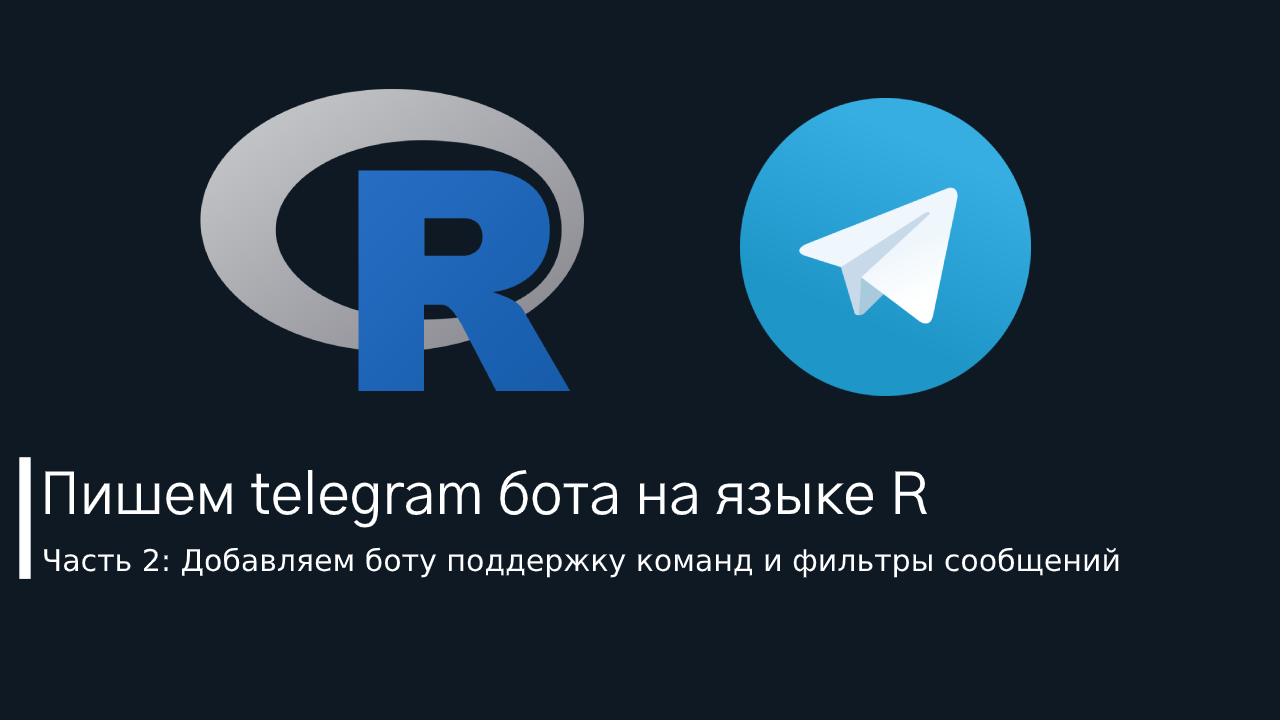
All articles from the series "Writing a telegram bot in the R language"
- We create a bot and send messages to telegram with it
- Add command support and message filters to the bot
- How to add keyboard support to your bot
- Building a consistent, logical dialogue with the bot
- Bot user rights management
Content
Updater
Updater
— , , Dispetcher
. Updater
, ( getUpdates()
), Dispetcher
.
Dispetcher
, .. Handler
.
Handlers —
Dispetcher
. telegram.bot
:
- MessageHandler —
- CommandHandler —
- CallbackQueryHandler — Inline
- ErrorHandler —
,
, , , /
.
, .. /hi
.
library(telegram.bot)
# Updater
updater <- Updater(' ')
#
say_hello <- function(bot, update) {
#
user_name <- update$message$from$first_name
#
bot$sendMessage(update$message$chat_id,
text = paste0(" , ", user_name, "!"),
parse_mode = "Markdown")
}
#
hi_hendler <- CommandHandler('hi', say_hello)
#
updater <- updater + hi_hendler
#
updater$start_polling()
, ' ' , BotFather ( ).
start_polling()
Updater
, , .
, /hi
.
/hi
, .
.
-
Updater
; - , .. .
say_hello()
. , — bot update, — args. bot, , , , . update , , ,getUpdates()
. args , ; - , .. - . , - .
/hi
,hi_hendler <- CommandHandler('hi', say_hello)
.CommandHandler()
,hi
, . ,say_hello
, ; -
Updater
. , ,+
, ..updater <- updater + hi_hendler
.add_handler()
,Dispatcher
, :updater$dispatcher$add_handler()
; -
start_polling()
.
, , , - , . — MessageHandler.
MessageHandler . . /hi
, , : , , , , .
- , .. . .
library(telegram.bot)
# Updater
updater <- Updater(' ')
#
##
say_hello <- function(bot, update) {
#
user_name <- update$message$from$first_name
#
bot$sendMessage(update$message$chat_id,
text = paste0(" , ", user_name, "!"),
parse_mode = "Markdown",
reply_to_message_id = update$message$message_id)
}
#
MessageFilters$hi <- BaseFilter(function(message) {
# , : , , , ,
grepl(x = message$text,
pattern = '||||',
ignore.case = TRUE)
}
)
#
hi_hendler <- CommandHandler('hi', say_hello) # hi
hi_txt_hnd <- MessageHandler(say_hello, filters = MessageFilters$hi)
#
updater <- updater +
hi_hendler +
hi_txt_hnd
#
updater$start_polling()
, ' ' , BotFather ( ).
, :
, , . reply_to_message_id, sendMessage()
, id . id : update$message$message_id
.
, — BaseFilter()
:
#
MessageFilters$hi <- BaseFilter(
#
function(message) {
# ,
grepl(x = message$text,
pattern = '||||',
ignore.case = TRUE)
}
)
, MessageFilters, . MessageFilters hi, .
BaseFilter()
. , — , TRUE FALSE. , , grepl()
, ||||
TRUE.
hi_txt_hnd <- MessageHandler(say_hello, filters = MessageFilters$hi)
. MessageHandler()
— , , — . MessageFilters$hi
.
, , hi_txt_hnd.
updater <- updater +
hi_hendler +
hi_txt_hnd
, telegram.bot
MessageFilters , :
- all —
- text —
- command — , ..
/
- reply — ,
- audio —
- document —
- photo —
- sticker —
- video —
- voice —
- contact —
- location —
- venue —
- game —
|
— , &
. , , :
handler <- MessageHandler(callback,
MessageFilters$video | MessageFilters$photo | MessageFilters$document
)
, , . , .
, .
API isdayoff.ru.
library(telegram.bot)
# Updater
updater <- Updater(' ')
#
##
check_date <- function(bot, update, args) {
#
day <- args[1] #
country <- args[2] #
#
if ( !grepl('\\d{4}-\\d{2}-\\d{2}', day) ) {
# Send Custom Keyboard
bot$sendMessage(update$message$chat_id,
text = paste0(day, " - , --"),
parse_mode = "Markdown")
} else {
day <- as.Date(day)
# POSIXtl
y <- format(day, "%Y")
m <- format(day, "%m")
d <- format(day, "%d")
}
#
##
## ru
if ( ! country %in% c('ru', 'ua', 'by', 'kz', 'us') ) {
# Send Custom Keyboard
bot$sendMessage(update$message$chat_id,
text = paste0(country, " - , : ru, by, kz, ua, us. ."),
parse_mode = "Markdown")
country <- 'ru'
}
# API
# HTTP
url <- paste0("https://isdayoff.ru/api/getdata?",
"year=", y, "&",
"month=", m, "&",
"day=", d, "&",
"cc=", country, "&",
"pre=1&",
"covid=1")
#
res <- readLines(url)
#
out <- switch(res,
"0" = " ",
"1" = " ",
"2" = " ",
"4" = "covid-19",
"100" = " ",
"101" = " ",
"199" = " ")
#
bot$sendMessage(update$message$chat_id,
text = paste0(day, " - ", out),
parse_mode = "Markdown")
}
#
date_hendler <- CommandHandler('check_date', check_date, pass_args = TRUE)
#
updater <- updater + date_hendler
#
updater$start_polling()
, ' ' , BotFather ( ).
, check_date
, .
, , , . , , .
, pass_args = TRUE
CommandHandler()
, , bot, update — args. , . , .
, .
— .
:
- R. RStudio File, Save As....
- bin, R Path, .
- , 1 :
R CMD BATCH C:\Users\Alsey\Documents\my_bot.R
. C:\Users\Alsey\Documents\my_bot.R . , , .. . , txt bat. - Windows, ,
%windir%\system32\taskschd.msc /s
. . - " ...".
- "" , " ".
- "", "". " " "", bat , .
- , .
- , "".
, , , .
, , . .