general information
In previous articles, we talked about how to use the HUAWEI ML Kit to create a smile detection function and an applet for document photos. In this article, I will show you how to implement the bank card recognition feature so that users can link a bank card with a minimal investment of time.
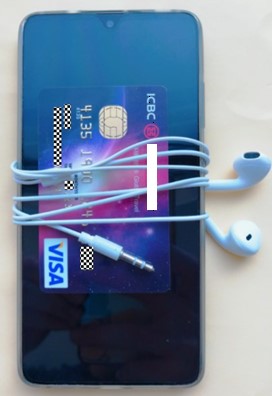
Purpose of the function of recognition of bank cards
Before we start developing, let's look at what the bank card recognition function is for. It is most relevant for applications with payment functions such as banking applications and online shopping. These applications often have a number of common requirements:
- Linking a bank card
Users can link their bank cards to make fast online payments.
- Bank transfers
Users can transfer money between accounts at the same bank or between different banks.
- Real name authentication and identity verification
Users can quickly authenticate with their real name and verify their identity based on their bank card information.
To use each of these options, the user must enter their bank card details, including the card number and expiration date. You know, of course, that it is easy to make mistakes and waste a lot of time when entering data manually. But thanks to the HUAWEI ML Kit bank card recognition service, users will be able to enter their data quickly and without errors.
Application of the bank card recognition service
The bank card recognition service allows the camera to recognize bank cards and read key data such as card number and expiration date. Supported by an ID recognition service, it offers a number of popular features such as identity verification and credit card number entry.
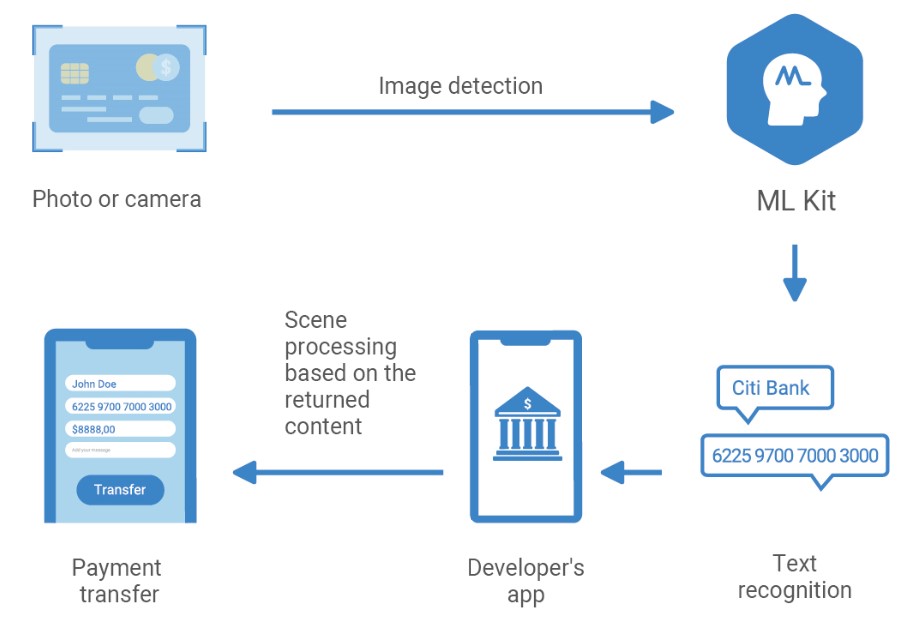
We offer a bank card recognition plugin that you can integrate to make this service available in your application. The plugin will handle the camera data for you.
Development start
1. Preparation
Add 1.1 Huawei's Maven repository to a file on the project level build.gradle
Open the file build.gradle in the root folder of your Android Studio project and add the address of the Maven repository.
buildscript {
repositories {
maven {url 'http://developer.huawei.com/repo/'}
} }allprojects {
repositories {
maven { url 'http://developer.huawei.com/repo/'}
}}
1.2 Add SDK dependencies to app-level file build.gradle
dependencies{
implementation 'com.huawei.hms:ml-computer-vision-bcr:1.0.3.303'
implementation 'com.huawei.hms:ml-computer-card-bcr-plugin:1.0.3.300'
implementation 'com.huawei.hms:ml-computer-card-bcr-model:1.0.3.300' }
1.3 Enable automatic app update to the latest model
To have your app automatically update the machine learning model after downloading from the HUAWEI AppGallery, add the following data to the AndroidManifest.xml file:
<manifest
...
<meta-data
android:name="com.huawei.hms.ml.DEPENDENCY"
android:value= "bcr"/>
<!--If multiple models are required,set the parameter as follows:
android:value="object,ocr,face,label,icr,bcr,imgseg"-->
... </manifest>
1.4 Request access rights to Camera and Memory in AndroidManifest.xml file
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
2. Code development
2.1 Create callback functions that are called after receiving the recognition result
Override the onSuccess, onCanceled, onFailure and onDenied functions :
- onSuccess : Called after recognition completes. MLBcrCaptureResult displays the recognition result.
- onCanceled : Called if the user canceled the recognition.
- onFailure : Called when recognition fails.
- onDenied : Called if the recognition request was denied for any reason (for example, if the camera is not available).
private MLBcrCapture.Callback callback = new MLBcrCapture.Callback() {
@Override
public void onSuccess(MLBcrCaptureResult bankCardResult){
}
@Override
public void onCanceled(){
}
@Override
public void onFailure(int retCode, Bitmap bitmap){
}
@Override
public void onDenied(){
} };
2.2 Set the recognition parameters for the captureFrame API call of the recognizer Recognition
data is returned by the callback function created in 2.1.
private void startCaptureActivity(MLBcrCapture.Callback callback) {
MLBcrCaptureConfig config = new MLBcrCaptureConfig.Factory()
.setOrientation(MLBcrCaptureConfig.ORIENTATION_AUTO)
.create();
MLBcrCapture bankCapture = MLBcrCaptureFactory.getInstance().getBcrCapture(config);
bankCapture.captureFrame(this, callback); }
2.3 Call the method specified in clause 2.2 with the callback of the recognition button
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.detect:
startCaptureActivity(callback);
break;
default:
break;
} }
Try it yourself
Let's take a look at how the bank card recognition feature works.
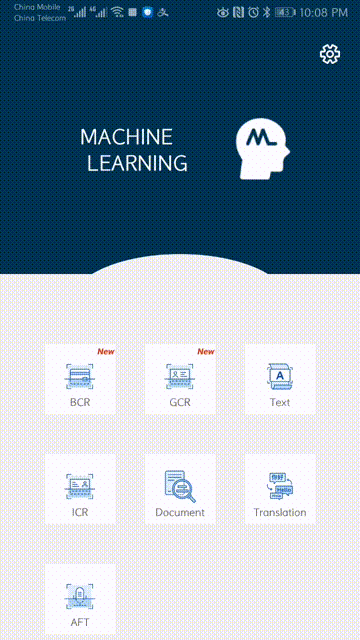
Source
We've uploaded the source to GitHub, so feel free to make changes.
github.com/HMS-Core/hms-ml-demo
More details you can visit
See the sample code for recognizing bank cards in MLKit-Sample \ module-text \ src \ main \ java \ com \ mlkit \ sample \ activity \ BankCardRecognitionActivity.java.
Please visit our official website for details.
New examples
We will share with you other useful features of the HUAWEI ML Kit. Stay tuned!