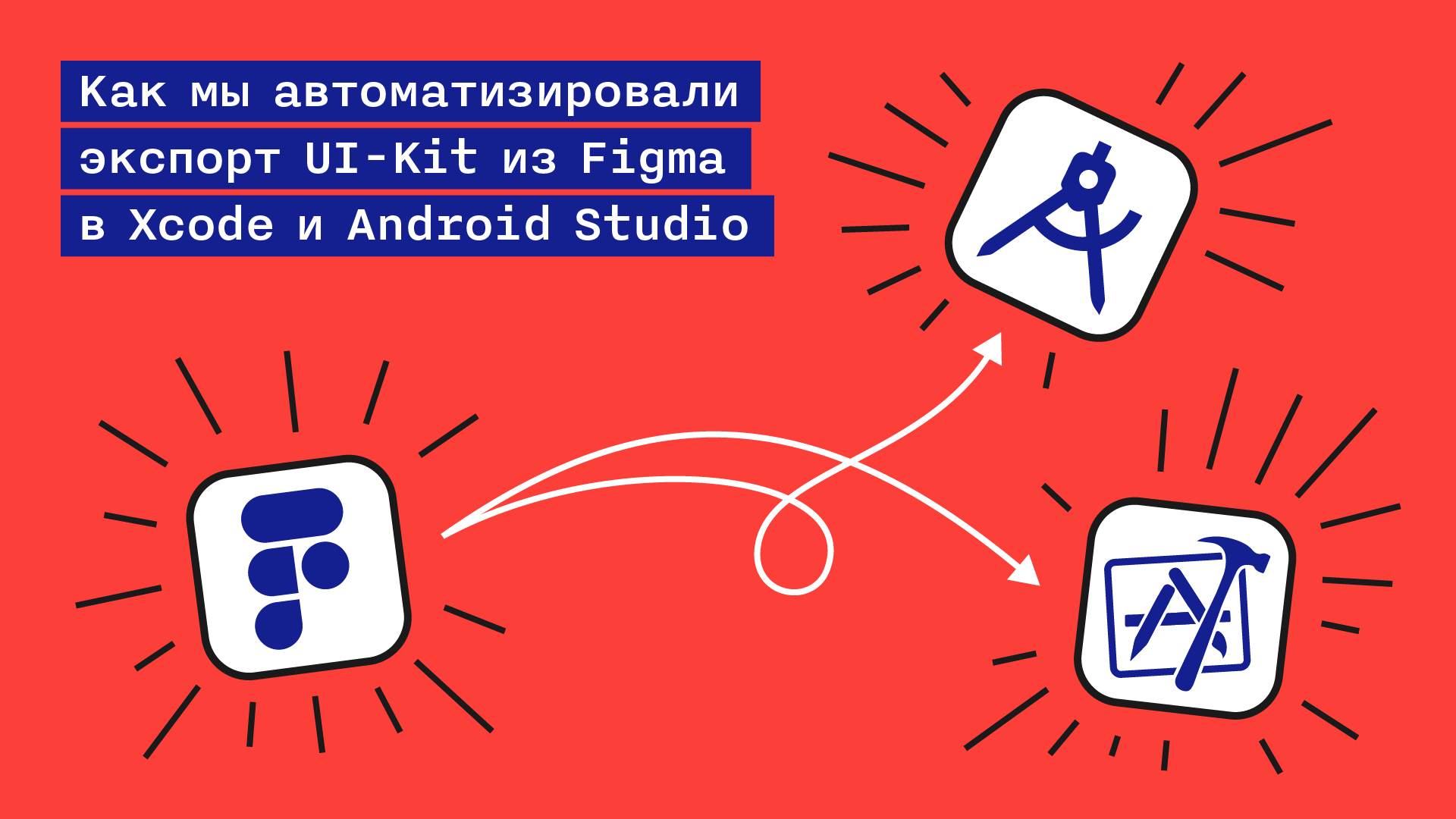
If you are an iOS or Android developer and the design of your project is developed in Figma, then most likely you encounter problems when exporting resources: you cannot unload colors, and it is inconvenient to export icons and pictures. In this article, I will tell you how you can make your life easier and automate the export of the UI-Kit from Figma directly to Xcode and Android Studio.
More and more mobile development teams are switching to Figma. Before, many (and we too) used a bundle of Sketch + Zeplin or Figma + Zeplin. And it was convenient. But when we added support for the dark theme on one of the projects, the situation got worse. Zeplin does not support the dark theme, so you have to look for workarounds that make you think: is Zeplin needed now? In this case, Zeplin is a waste of the designer's time maintaining and synchronizing projects in two tools, as well as additional costs for the company.
After ditching Zeplin and moving to Figma, we (the developers) began to experience pain exporting the UI-Kit from Figma.
Figma, unlike Zeplin, does not interact with Xcode or Android Studio in any way. Plugins prevent Figma from tightly integrating with them. We found a command line utility that exported colors and text styles, but it didn't work for us - it didn't support exporting images and a dark theme. That is why I wrote my utility.
But a little more detail on the problems.
Why is it inconvenient for mobile developers to export assets from Figma
Problem # 1: not being able to export colors
What do we - developers see when we open the UI-Kit in Figma? In the simplest case, several circles of different colors.

But if the project is large and supports a dark theme, then the palette will contain many more colors:
Planning to add dark theme support? See how much larger a palette can get

Figma doesn't have the ability to export colors. The most you can do is select the color and copy the HEX value. And if the designer changes something, then in the case of a large palette, the developer will have to spend a lot of time to compare the palette in the code and in Figma.
The color name also does not always work well to copy. Some designers use the "/" character in the style name, but it is not supported in iOS and Android. This symbol is needed to group colors into groups.
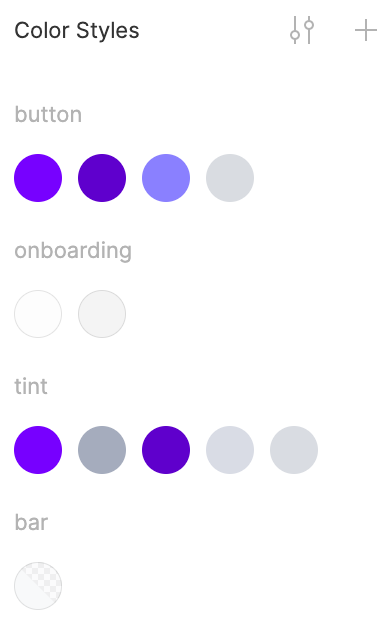
Example. The color in Figma is named background / primary . For Android developers, you need to specify a color called background_primary , and for iOS developers, backgroundPrimary .
Another problem with colors is the dark theme. No designer tool (Figma, Zeplin, Sketch) allows you to have a dark and light palette at the same time. There are two approaches here: create a separate file with a dark palette, or store all the colors in one file, but name them with a postfix, for example, background_primary_day, background_primary_night.
What we want as developers is to be able to export the color palette from Figma directly to Xcode or Android Studio projects.
Problem # 2: inconvenient export of icons using standard tools
There are also several difficulties here.
Again, it happens that designers use the "/" character in the name of icons to group icons. For example, if the icon with the name ic / 24 / tab / profile is exported using the standard Figma tools, we will get such nesting.
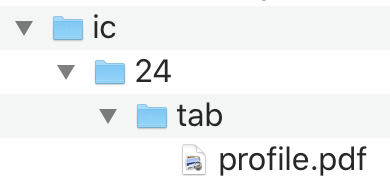
It is impossible to work with this. I have to rename the file. The file should be named ic24TabProfile.pdf . This is for iOS developers.
It is common for Android developers to name resources in the snake_case style . In this case, the file will be named ic_24_tab_profile.xml. But Figma doesn't do that.
Icons exported from Figma will have to be manually transferred to the project. And it's not enough for iOS developers to just migrate them. It is necessary to put down for each icon: Preserve Vector Data, Single Scale, Render as Template Image.
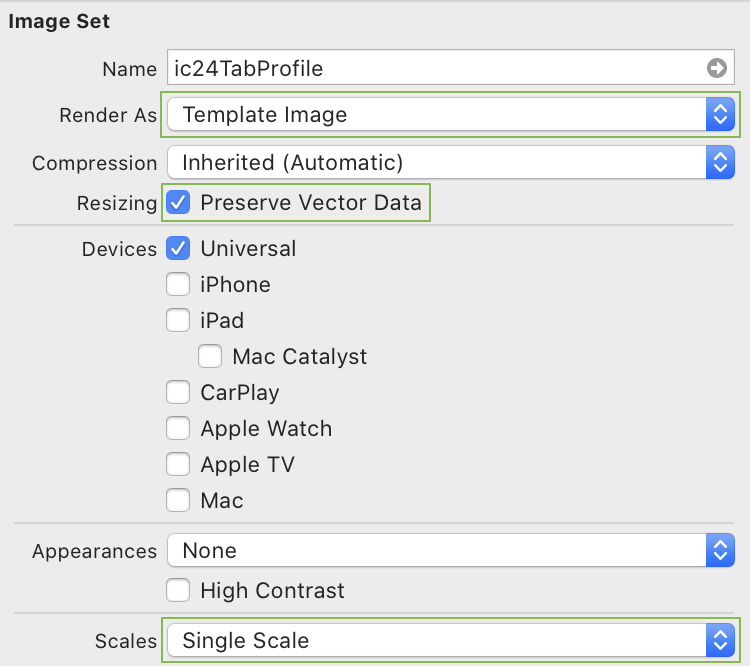
What we want as developers is to be able to export all Figma icons directly to Xcode or Android Studio projects.
Problem # 3: inconvenient export of illustrations using standard tools
Icons are small vector images, often black and white, and can be recolored (by the system or developer) and resized. If you enable accessibility, then some icons grow with the text. Their main problem is the names: the "/" character, which I mentioned above, and the fact that iOS developers use camelCase names, and Android developers use snake_case.
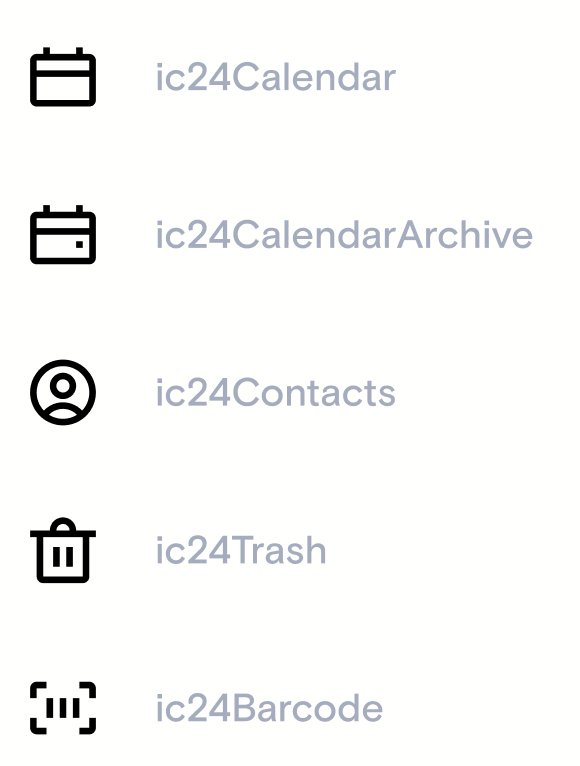
Illustrations are large color images that differ in dark and light themes. In a dark theme, colors should be muted. If the illustration is too light, then standing out against the dark, it will "hit" with bright light in the eyes.
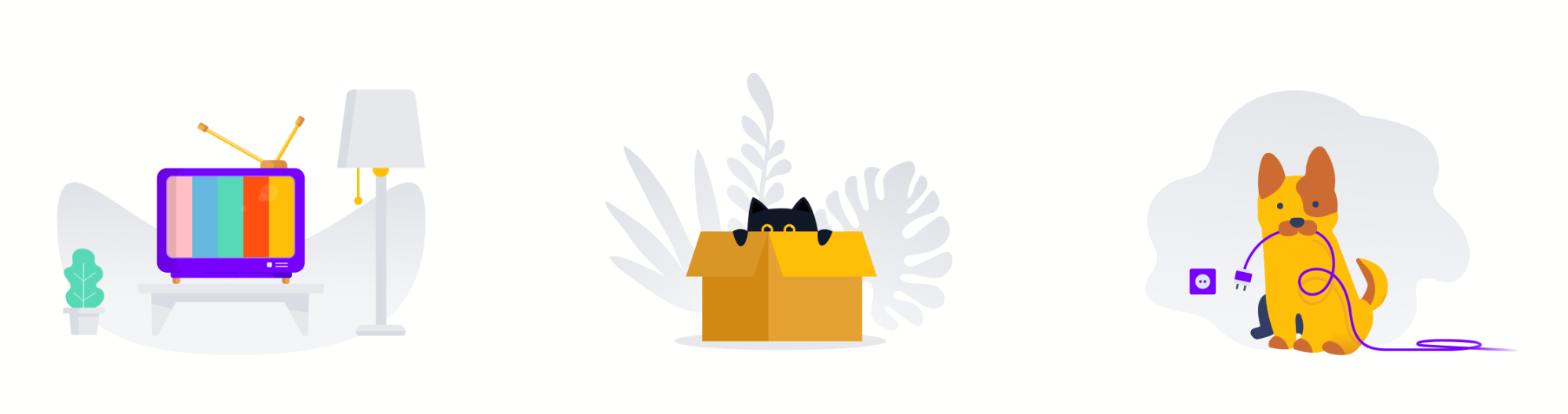
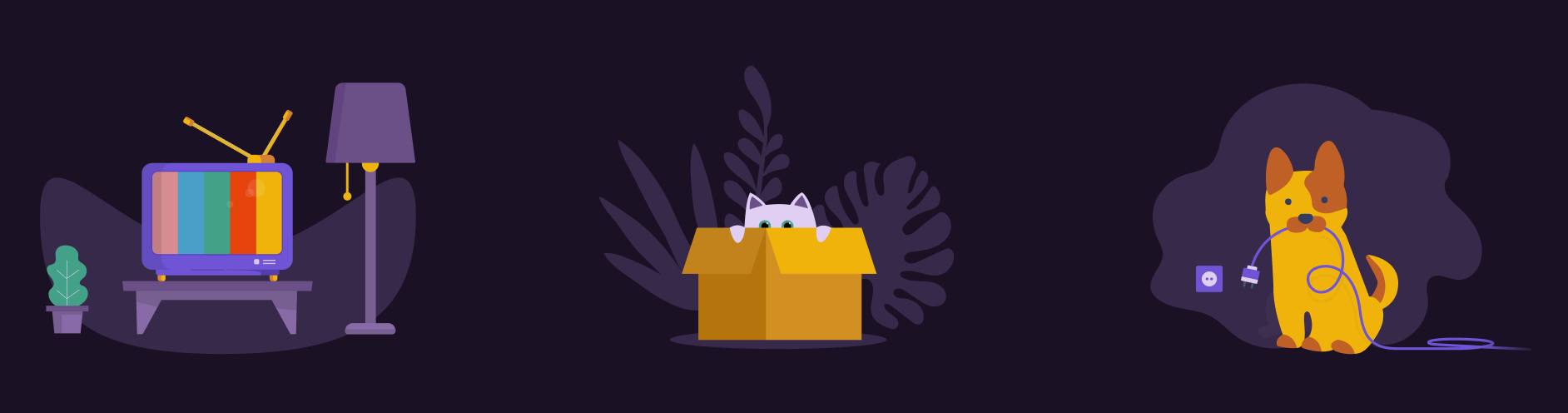
Illustrations have the same problems as icons, but there are also a couple of new ones.
Android developers export Figma icons and illustrations as SVG files, and then, using Android Studio's built-in tools, convert the SVG files to vector drawable XML files. If you need to export 50 icons or illustrations, it will take a long time to convert them all. This can be automated.
iOS developers export illustrations as bitmaps at three scales. If the application supports a dark theme, then there will be 6 images in total. This is how it looks in an iOS project:
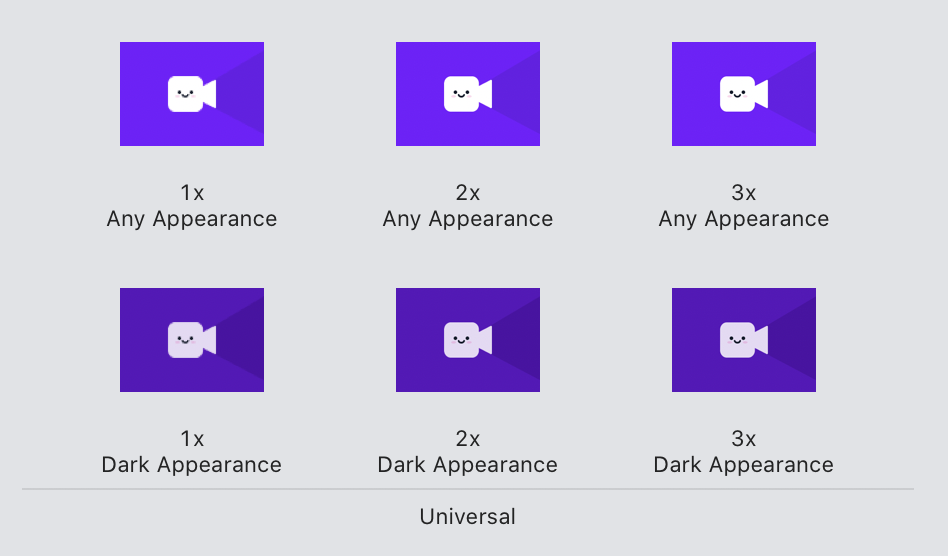
Now, let's imagine that we have unloaded 50 illustrations from Figma. It turns out that we have 50 * 6 = 300 PNG-images, which must be manually transferred to the project via drag & drop, and then all of them, again, must be manually renamed. Do you want to do this? - no. There are more important things to do ...
What we want as developers is to be able to export all Figma illustrations directly to Xcode or Android Studio projects.
Why Zeplin didn't help:
- it does not support dark theme;
- does not allow you to have several colors with the same HEX value, but different names: if you name the colors according to the place of their application, it may turn out that two colors will have different names, but the same HEX values. For example, backgroundPrimaryPressed (main background color when pressed) and backgroundSecondary (background color) must have the same HEX value. Zeplin won't let you do that. There is a workaround - change the HEX value to the lowest possible value. Example # F4F5F8 and # F4F5F7;
- requires additional designer resources to synchronize layouts and UI kit with Figma;
- it costs extra money. Figma costs $ 12 per designer per month for an organization. If you buy Zeplin, that's another $ 10.75 per designer per month;
How we automated the export of assets from Figma
Realizing that this is no longer possible to live like this, I looked to see if Figma has any API or the ability to extend with plugins to automate export, and it turned out that it does. I had two paths: write a Figma plugin or use the Figma API .
The Figma plugin works directly in the Figma app. Using the Figma API, you can write a console utility. The plugin can not only read information from Figma files, but also make changes to them. Because of this, Figma plugins require the developer to have permission to edit the file. Figma API can only read information from Figma files.
The plugin is written in JavaScript. Using the Figma API, you can write a wrapper around anything. But Figma plugin cannot work with the file system on the developer's (user's) computer. That is why I didnβt do it.
I am an iOS developer, so I decided to make a console utility in Swift. I started with a prototype that should export a color palette from Figma directly to an Xcode project. In a couple of weeks it was ready. It was something. I ran my utility and after a few seconds I got the whole palette in Xcode. This is how a perfect Figma export should work :)

After a couple of months, my utility for exporting assets from Figma was ready. You can find a link to it at the end of the article.
How the export process works
Colors
The developer calls the command
figma-export colors
. If it's an iOS project, the colors are exported to the Assets.xcassets folder .
Additionally, a Color.swift file is created so that you can use colors directly from the code.
import UIKit
extension UIColor {
static var backgroundSecondaryError: UIColor { return UIColor(named: #function)! }
static var backgroundSecondarySuccess: UIColor { return UIColor(named: #function)! }
static var backgroundVideo: UIColor { return UIColor(named: #function)! }
...
}
If it is an Android project, the colors are exported to values ββ/ colors.xml and values-night / colors.xml if dark theme is supported.
values ββ/ colors.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="button">#7701FF</color>
<color name="button_ripple">#8A80FF</color>
<color name="button_disabled">#D9DCE1</color>
<color name="text_primary">#FFFFFC</color>
<color name="text_primary_pressed">#A680FE</color>
<color name="text_primary_disabled">#FFFFFE</color>
<color name="text_secondary">#101828</color>
<color name="text_tertiary">#A5ACBD</color>
...
values-night / colors.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="button">#6F01EC</color>
<color name="button_ripple">#7D6AF0</color>
<color name="button_disabled">#3F334B</color>
<color name="text_primary">#E6D9F6</color>
<color name="text_primary_pressed">#E6D9F3</color>
<color name="text_primary_disabled">#544761</color>
<color name="text_secondary">#E6D9F5</color>
<color name="text_tertiary">#7B6F98</color>
...
Icons
The developer calls the command If this is an iOS project, the icons will be exported as PDF files with the Render as Template Image parameter to the Assets.xcassets folder . If icons will be used in the UITabBar , then you can optionally specify Preserve Vector Data to support Accessibility. If it is an Android project, the icons will be exported to the drawable folder as vector xml files. Under the hood, the utility converts SVG files to XML using the official vd-tool (vector-drawable-tool), which is used by Android Studio.
figma-export icons.
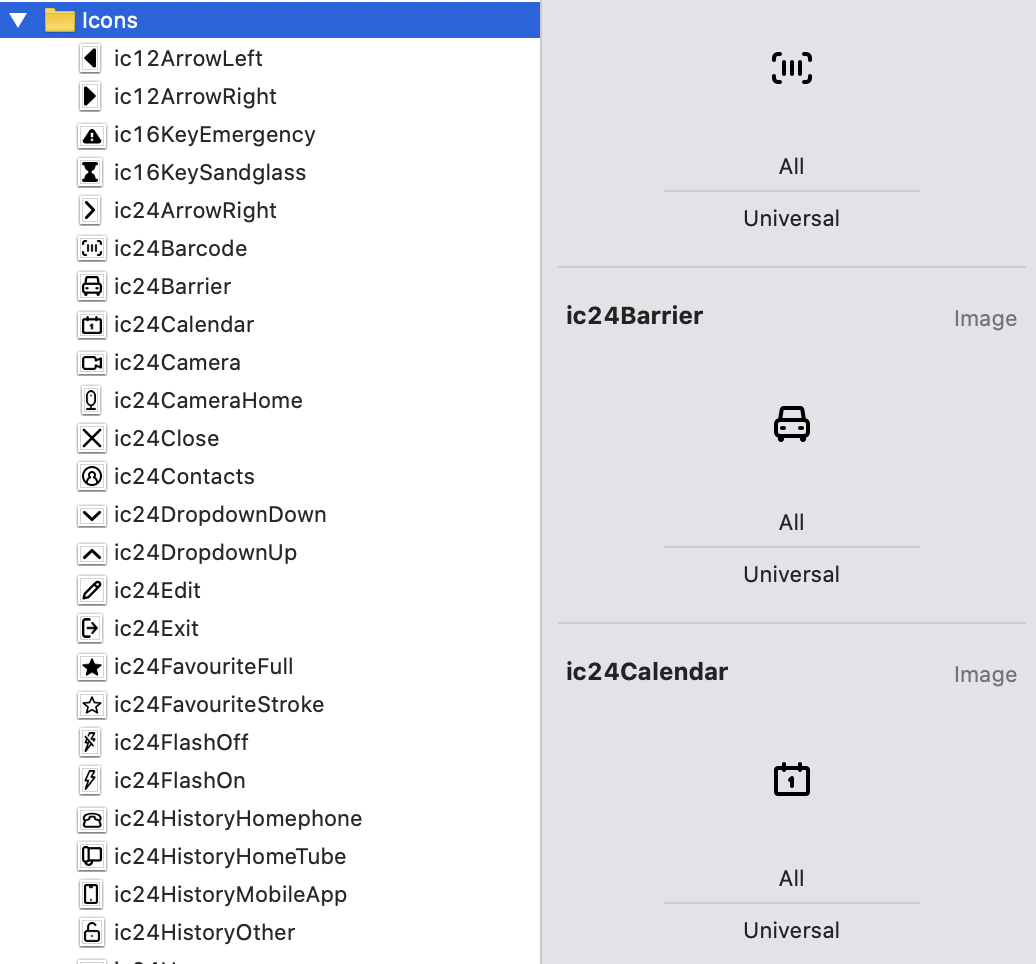
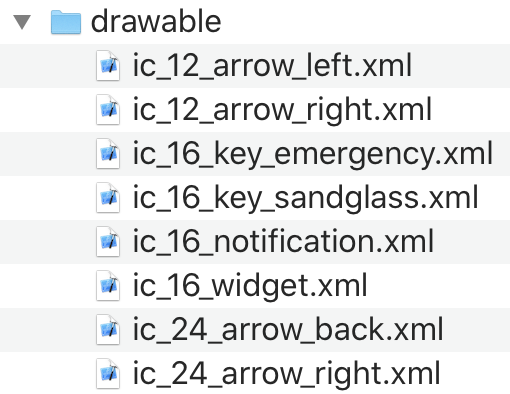
Illustrations
The developer calls the command. Everything is the same, only the pictures are exported as PNG files. If it is an Android project, the illustrations will be exported to the drawable and drawable-night folder as vector xml files.
figma-export images.
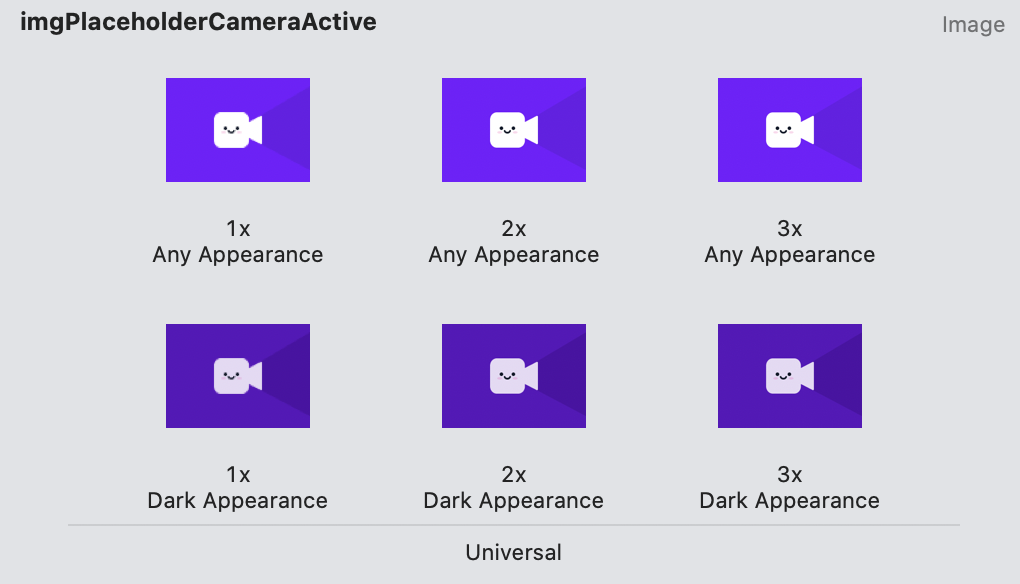
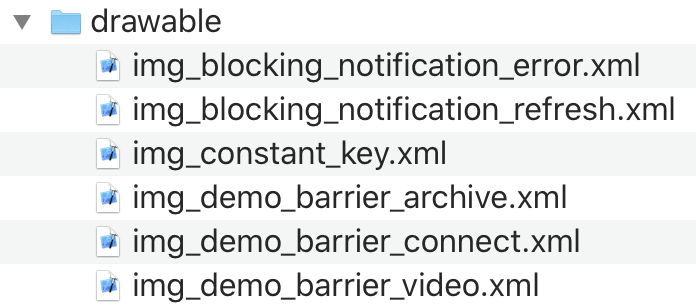
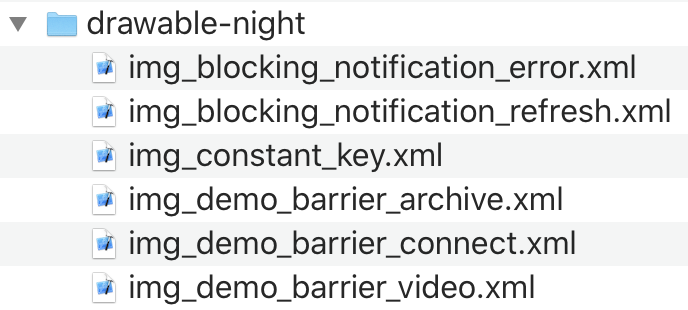
How export can be configured
FigmaExport has a lot of settings that are stored in the config file and passed when the utility starts.
./figma-export colors -i figma-export.yaml
The configuration file specifies the parameters for iOS, for Android and general parameters. It also contains the identifier of the Figma file, where the colors, icons and pictures are located. The file ID can be found in the address bar if you open it in a browser.
For example, here is the address of our UI-Kit: www.figma.com/file/GVHjNNE8PKKRf1KtnMPY9m/RTC-Key-UI-kit
The identifier of the lightFileId file in our case: GVHjNNE8PKKRf1KtnMPY9m
An example of a configuration file for an iOS project:
---
figma:
lightFileId: shPilWnVdJfo10YFo12345
darkFileId: KfF6DnJTWHGZzC9Nm12345
ios:
# Path to the Assets.xcassets directory
xcassetsPath: "./Resources/Assets.xcassets"
# Parameters for exporting colors
colors:
# Should be generate color assets instead of pure swift code
useColorAssets: True
# Name of the folder inside Assets.xcassets where to place colors (.colorset directories)
assetsFolder: Colors
# Path to Color.swift file where to export colors for accessing colors from the code (e.g. UIColor.backgroundPrimary)
colorSwift: "./Sources/Presentation/Common/Color.swift"
# Color name style: camelCase or snake_case
nameStyle: camelCase
# Parameters for exporting icons
icons:
# Name of the folder inside Assets.xcassets where to place icons (.imageset directories)
assetsFolder: Icons
# Icon name style: camelCase or snake_case
nameStyle: camelCase
# [optional] Enable Preserve Vector Data for specified icons
preservesVectorRepresentation:
- ic24TabMain
- ic24TabHistory
- ic24TabProfile
# Parameters for exporting images
images:
# Name of the folder inside Assets.xcassets where to place images (.imageset directories)
assetsFolder: Illustrations
# Image name style: camelCase or snake_case
nameStyle: camelCase
An example of a config file for an Android project:
---
figma:
lightFileId: shPilWnVdJfo10YFo12345
darkFileId: KfF6DnJTWHGZzC9Nm12345
android:
mainRes: "./main/res"
How to organize a Figma file for automated resource export
In order for the UI-Kit to be automatically unloaded, the following rules must be observed.
General
- If a color, icon or illustration is unique for iOS or Android in its properties, the description field should contain βiosβ or βandroidβ . If a color, icon or illustration should not be available for export, then their description property will have βnoneβ . Thus, FigmaExport will determine what should be exported to an iOS project, what is an Android project, and what should not be exported at all.
Example. The share icon looks different on iOS and Android. The screenshots below indicate that the ic24ShareIos icon will be exported only to an iOS project. ios is specified in the Component description property, and the ic24ShareAndroid icon will be exported only to an Android project;
- Icons and illustrations should be components.
- Color styles and components must be published in the Team Library.
- Only icons and illustrations added to the Icons and Illustrations frames are exported.
Examples
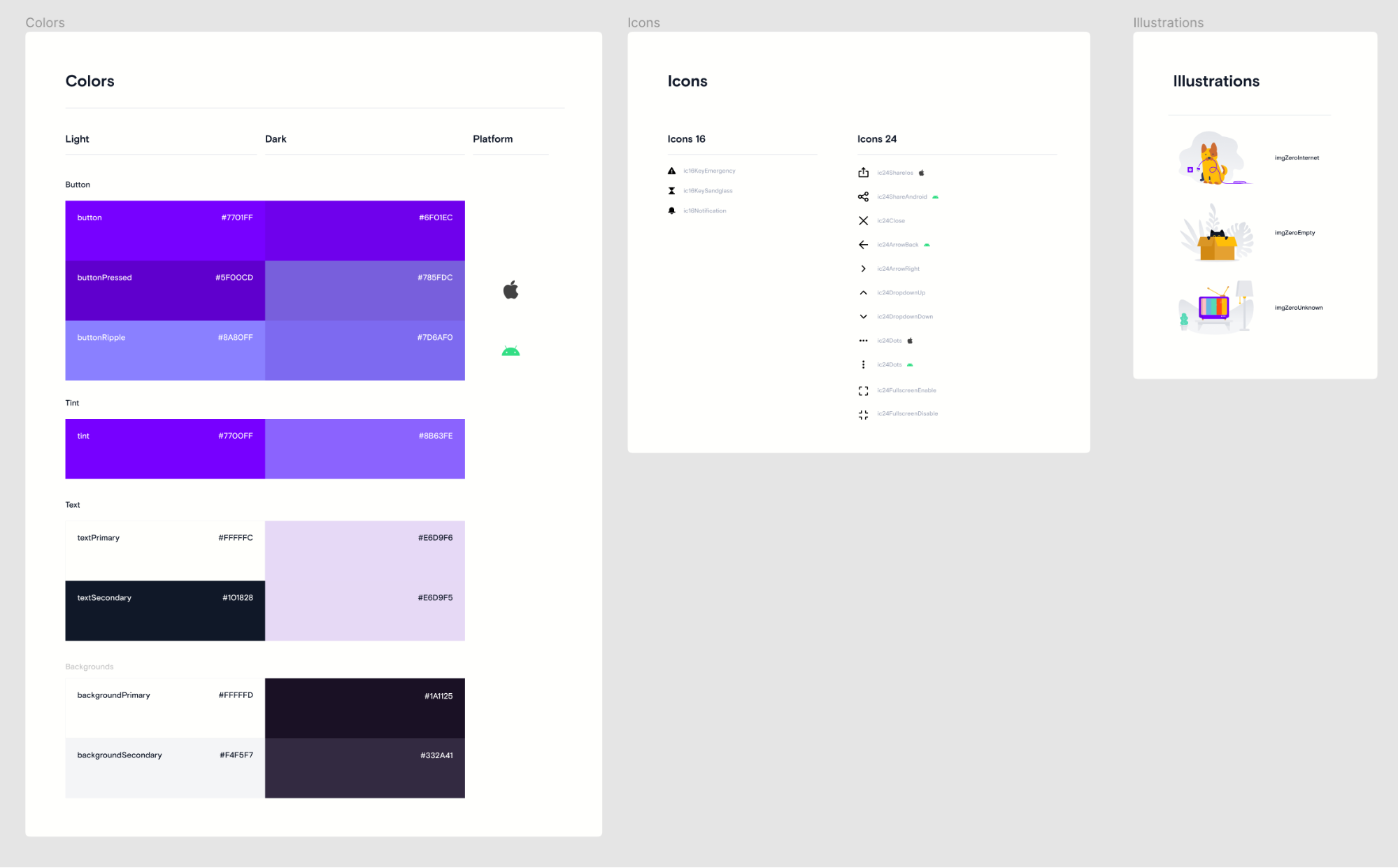
Figma-file with UI-Kit (light theme)
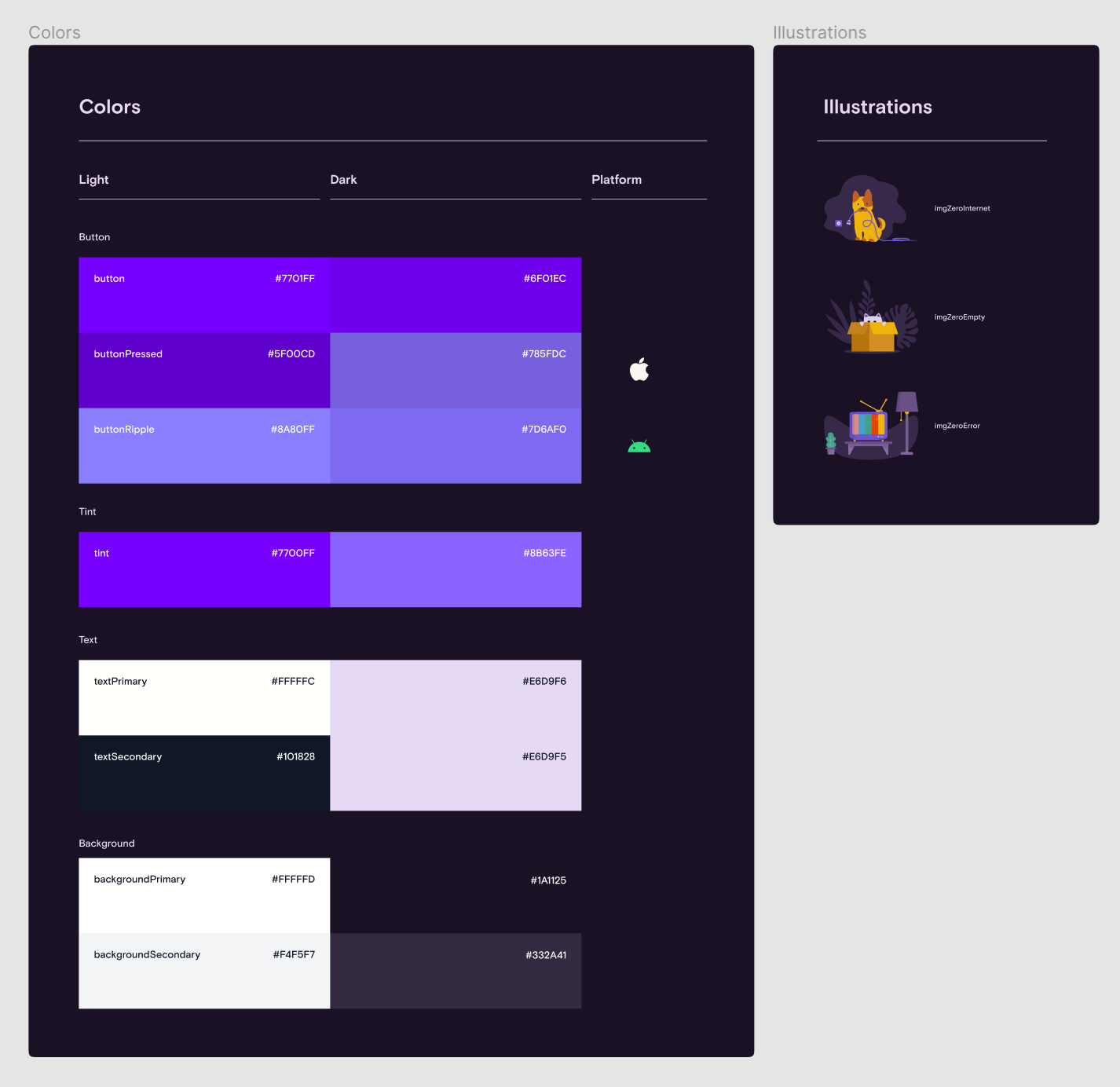
Figma-file with UI-Kit (dark theme)
Resource names
Colors, icons and illustrations can be called any names containing letters az, AZ and symbols "_" and "/".
Since variable names in the code cannot have the β/β symbol, FigmaExport automatically replaces it with the β_β symbol. Then the resulting string is converted to camelCase for iOS or snake_case for Android.
Color name | iOS | Android |
---|---|---|
button / pressed | buttonPressed | button_pressed |
background / primaryPressed | backgroundPrimaryPressed | background_primary_pressed |
Icon name | iOS | Android |
---|---|---|
ic / 24 / sound_off
|
ic24SoundOff
|
ic_24_sound_off |
Title of illustration | iOS | Android |
---|---|---|
img / demo / camera_archive
|
imgDemoCameraArchive
|
img_demo_camera_archive
|
For consistency and convenience, you can, for example, name all icons in your own format - ic / size / name . An example is ic / 24 / open . And the illustrations are img / group / title . An example is img / zero / nointernet. You can read about colors in the article "Application designer: how to create and transfer a dark theme" .
In the config file figma-export.yaml, you can use regular expressions to enable name validation before export. If any resource has the wrong name, FigmaExport will report it.
common:
colors:
# RegExp pattern for color name validation before exporting
nameValidateRegexp: '^[a-zA-Z_]+$' # RegExp pattern for: background, background_primary, widget_primary_background
icons:
# RegExp pattern for icon name validation before exporting
nameValidateRegexp: '^(ic)_(\d\d)_([a-z0-9_]+)$' # RegExp pattern for: ic_24_icon_name, ic_24_icon
images:
# RegExp pattern for image name validation before exporting
nameValidateRegexp: '^(img)_([a-z0-9_]+)$' # RegExp pattern for: img_image_name
Dark theme
If your project supports a dark theme, you need to create a separate file with UI components, which will contain a dark color palette and dark illustrations.
Colors
In the Figma file, colors must be styled as color styles and published to the Team Library.
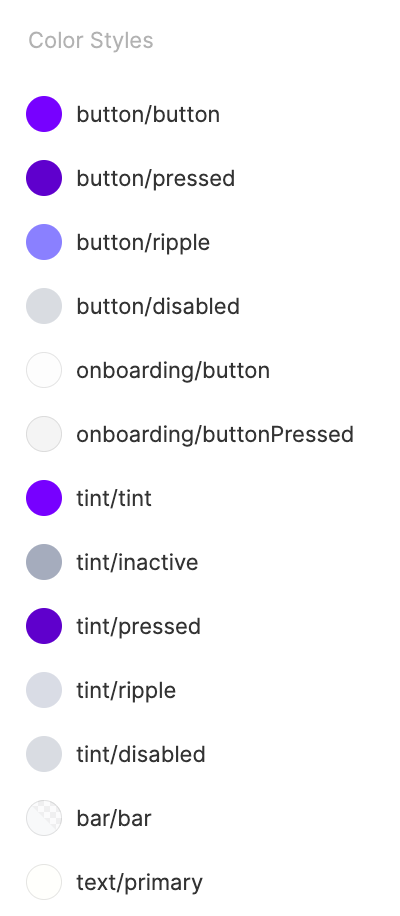
For the convenience of developers, it is advisable to make a table of all colors, which will indicate what color and where it is used.
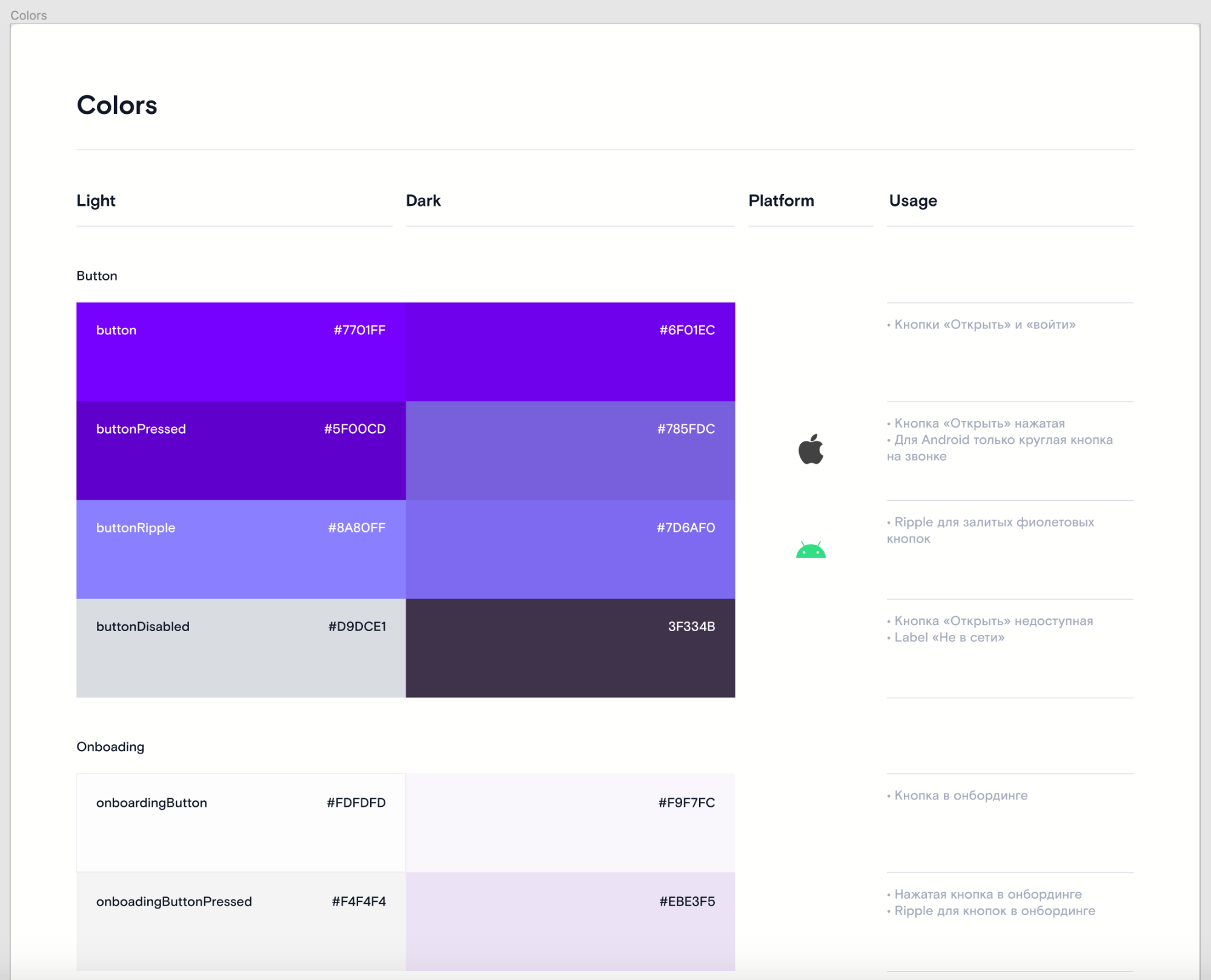
Icons
The Figma file must have a Frame named Icons. This Frame should contain components for each icon. Example:
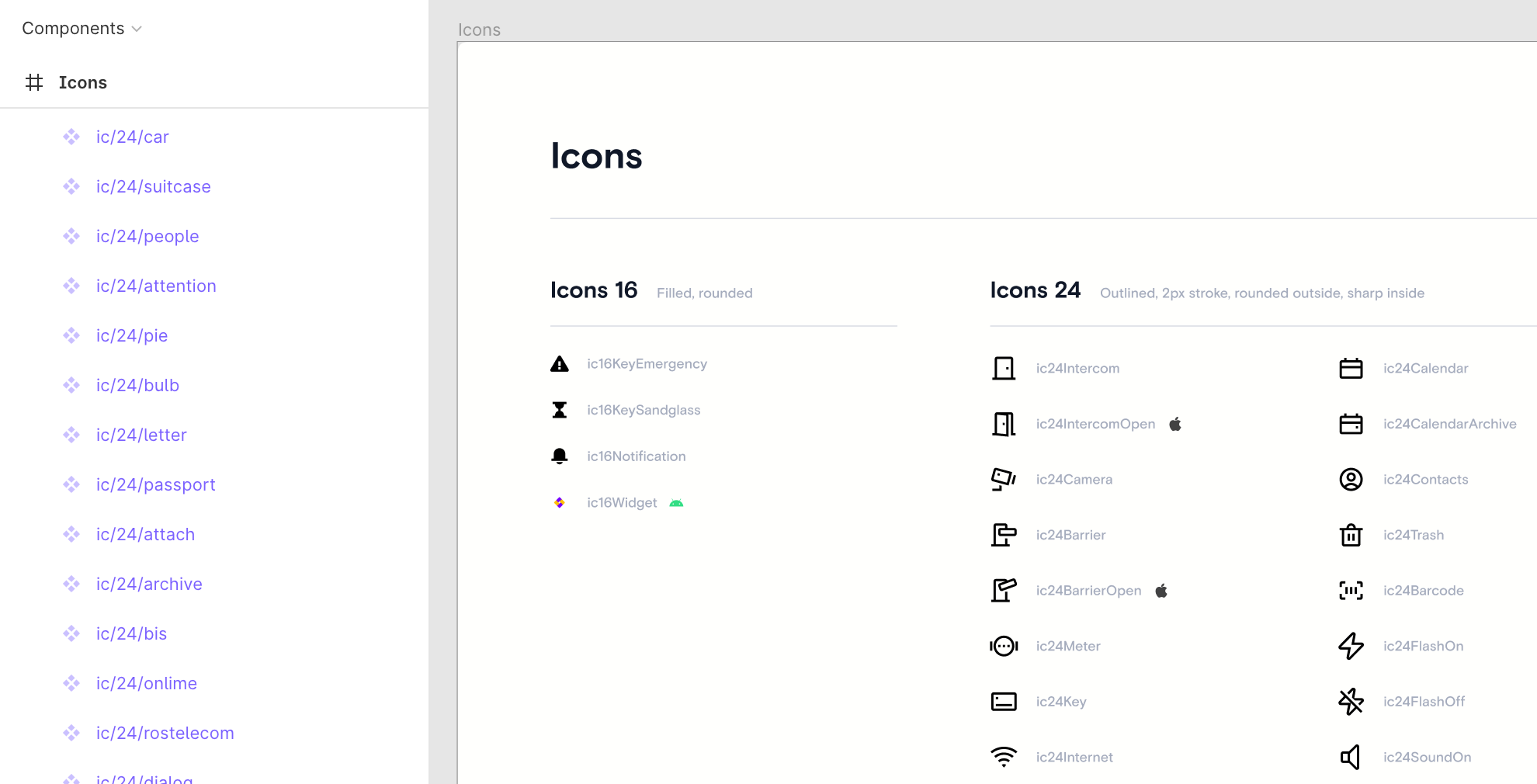
Illustrations
The Figma file should have a Frame named Illustrations, which contains the components for each illustration. Example:
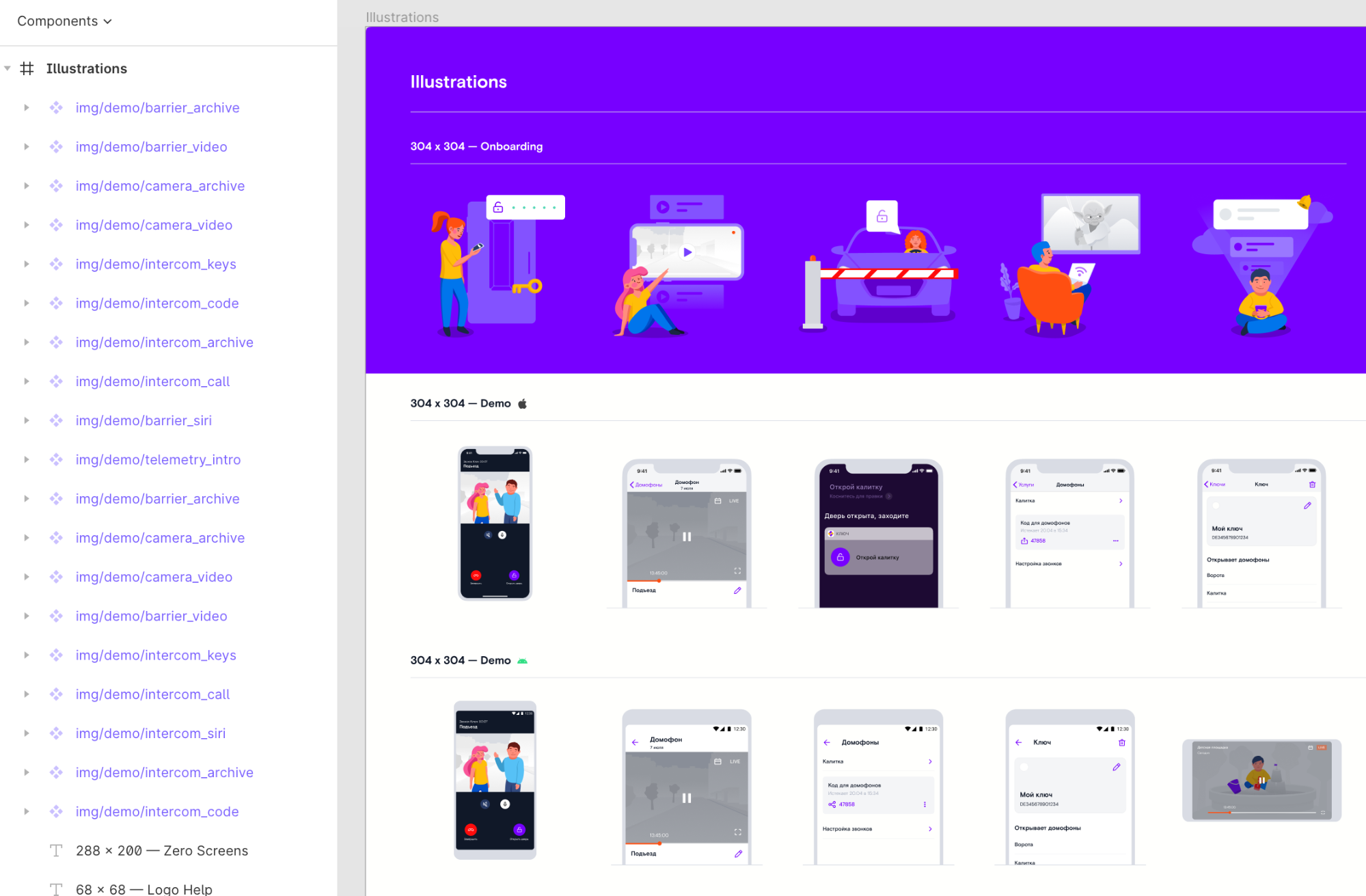
Outcome
Thanks to FigmaExport, we got rid of a lot of problems when working with Figma. Exporting resources now takes seconds. A few examples of how using the utility made our life easier.
1. One of the projects had 52 icons. The designer decided that they all need to be brought into the same style. After he updated them, we had to update them at home.
How would we update them manually: we exported all the icons from Figma as a zip file, renamed 52 files, transferred the icons to the project, put down all the necessary properties, made sure that they had not forgotten anything. This would take at least an hour.
How we updated them from FigmaExport: we ran the figma-export icons command .After 10 seconds, all the changes were tightened, we launched the application on the simulator and saw that all the icons were replaced.
2. At one of the sprints, we decided to release a new feature. In the UI-Kit, the designer added four new icons, removed two old icons, and added two new colors.
Instead of spending one hour of time discussing with the designer what he changed in the UI-Kit, manual export of icons and colors, we just ran the figma-export colors and figma-export icons command and through Git we saw what was removed, what was added, and what changed. And they immediately began to make up layouts using new icons and colors.
3. We made a feature in which it was necessary to make advanced onboarding with 4 sections, each of which has up to 7 pages with pictures.
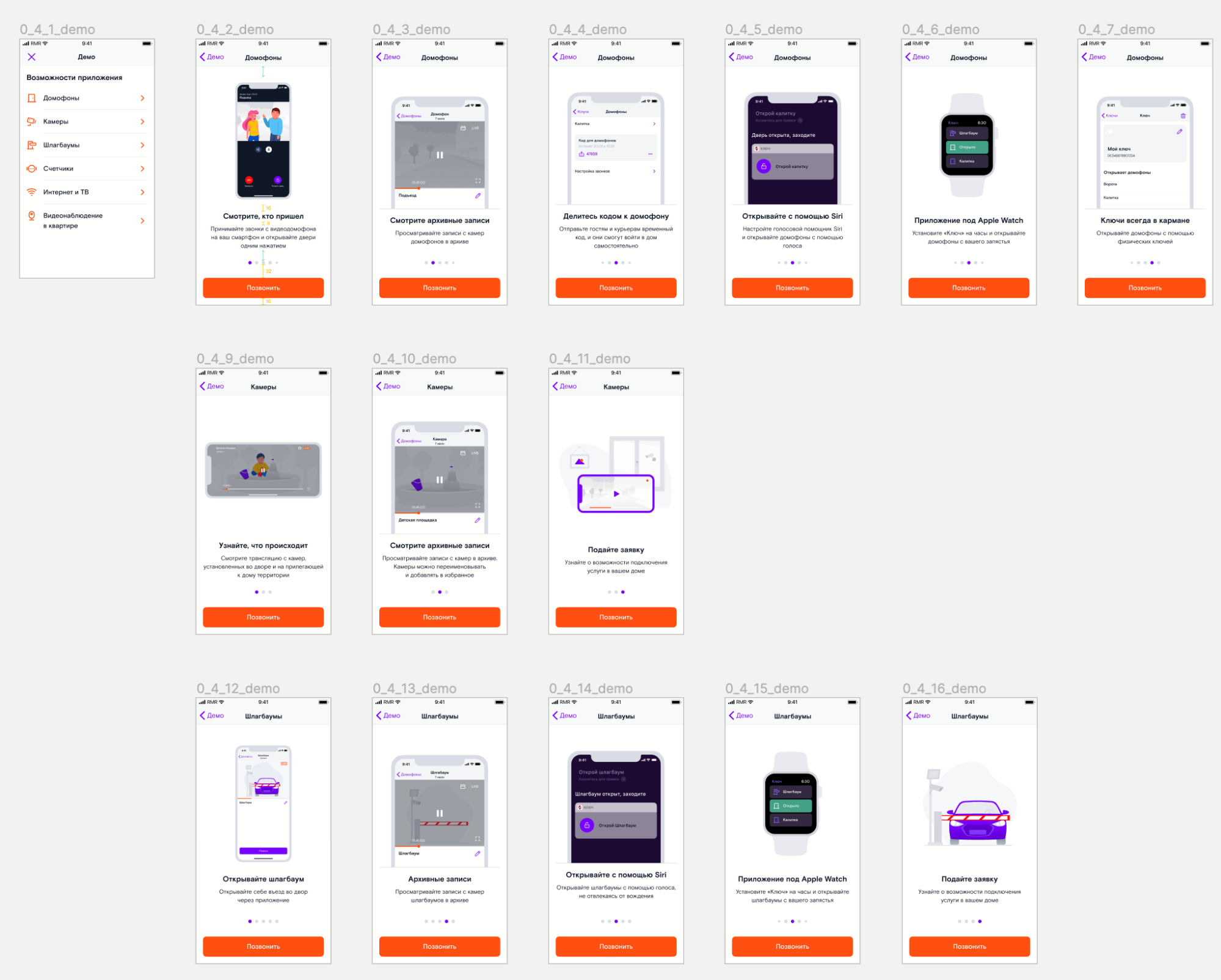
Manual export would take a long time. Having executed the figma-export images command in less than a minute, we got all these images in the project, ready to use.
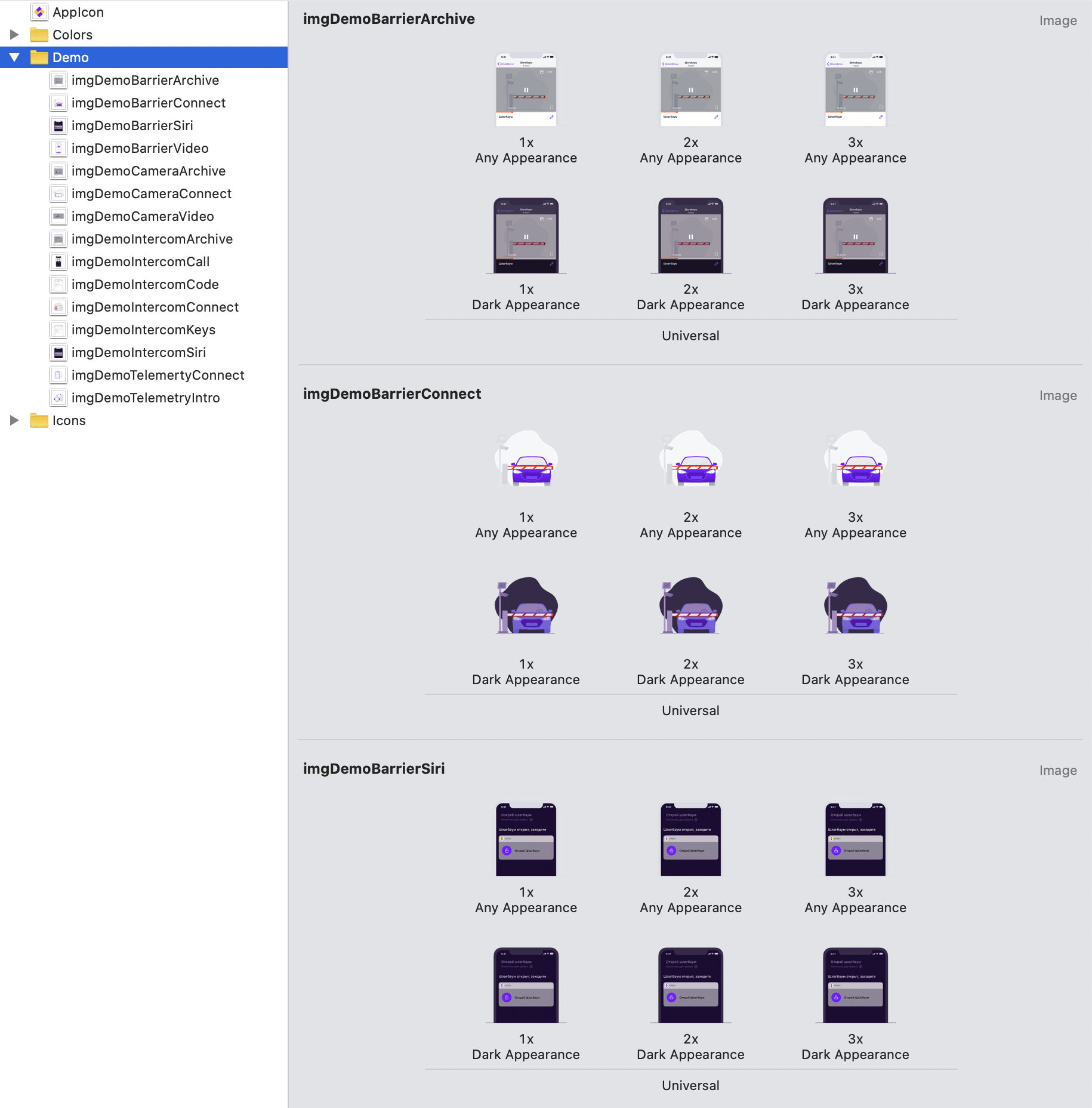
Few cons
- It is necessary to agree with the designer about how to store colors, icons, pictures in the UI-Kit so that they can be automatically unloaded.
- The export only works for those components that are added to the Team Library, so designers must have a paid Figma subscription.
Plans:
- add the ability to export text styles,
- add the ability to export bitmap images for Android,
- add SwiftUI support.
Special thanks to Artur Abrarov and Katya Rokityan for helping to finalize the UI-Kit.
Utility link
Download FigmaExport on GitHub .
I will be glad if you try my utility. Questions, wishes, feedback - write to me at d.subbotin@redmadrobot.com