general information
In our last article, we covered how to quickly integrate the HMS Core Scan SDK and compared this SDK to other open source barcode scanning tools. If you haven't read this article yet, you can find it here .
We scan barcodes every day to pay for purchases, subscribe to social media accounts, and receive product information. Today I want to show you how to implement barcode scanning functionality in your shopping app.
Scenario
With this feature, users can scan a barcode to get product information and a link to make a purchase.

Action diagram

Training
Open build.gradle file in gradle folder

Go to allprojects> repositories and configure the Maven repository address for the HMS Core SDK.
allprojects {
repositories {
google()
jcenter()
maven {url 'http://developer.huawei.com/repo/'}
}
}
Go to buildscript> repositories and configure the Maven repository address for the HMS Core SDK.
buildscript {
repositories {
google()
jcenter()
maven {url 'http://developer.huawei.com/repo/'}
}
}
Add dependencies
Open build.gradle file in app folder .

Integrate HMS Core SDK.
dependencies{
implementation 'com.huawei.hms:scan:1.1.3.301'
}
Assign permissions and specify features.
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-feature android:name="android.hardware.camera" />
<uses-feature android:name="android.hardware.camera.autofocus" />
Since SDK integration uses the default View mode, specify the right to use the scan screen in the AndroidManifest.xml file in the application folder.
<activity android:name="com.huawei.hms.hmsscankit.ScanKitActivity" />
Implementation
Two functions are available for implementation: adding a product and searching for a product. You can link a product by scanning a barcode and taking a photo. Users can then search for that product by scanning the barcode.
Submit a request for a dynamic rights request
private static final int PERMISSION_REQUESTS = 1;
@Override
public void onCreate(Bundle savedInstanceState) {
// Checking camera permission
if (!allPermissionsGranted()) {
getRuntimePermissions();
}
}
Open the add product screen
Click Add Product to open a screen where you can add a product.
public void addProduct(View view) {
Intent intent = new Intent(MainActivity.this, AddProductActivity.class);
startActivityForResult(intent, REQUEST_ADD_PRODUCT);
}
Scan barcode to record product information
Scan the barcode using the HUAWEI Scan Kit's Default View.
private void scanBarcode(int requestCode) {
HmsScanAnalyzerOptions options = new HmsScanAnalyzerOptions.Creator().setHmsScanTypes(HmsScan.ALL_SCAN_TYPE).create();
ScanUtil.startScan(this, requestCode, options);
}
Save the scan result in a callback function
@Override
public void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (data == null) {
return;
}
if ((requestCode == this.REQUEST_CODE_SCAN_ALL)
&& (resultCode == Activity.RESULT_OK)) {
HmsScan obj = data.getParcelableExtra(ScanUtil.RESULT);
if (obj != null && obj.getOriginalValue() != null) {
this.barcode = obj.getOriginalValue();
}
} else if ((requestCode == this.REQUEST_TAKE_PHOTO)
&& (resultCode == Activity.RESULT_OK)) {
……
}
}
Scan the barcode to find a product
Click on Query Product and open the screen where you can find the product. Display the result with a callback function.
public void queryProduct(View view) {
HmsScanAnalyzerOptions options = new HmsScanAnalyzerOptions.Creator().setHmsScanTypes(HmsScan.ALL_SCAN_TYPE).create();
ScanUtil.startScan(this, REQUEST_QUERY_PRODUCT, options);
}
@Override
public void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (data == null) {
return;
}
if ((requestCode == this.REQUEST_ADD_PRODUCT) && (resultCode == Activity.RESULT_OK)) {
barcodeToProduct.put(data.getStringExtra(Constant.BARCODE_VALUE), data.getStringExtra(Constant.IMAGE_PATH_VALUE));
} else if ((requestCode == this.REQUEST_QUERY_PRODUCT) && (resultCode == Activity.RESULT_OK)) {
HmsScan obj = data.getParcelableExtra(ScanUtil.RESULT);
String path = "";
if (obj != null && obj.getOriginalValue() != null) {
path = barcodeToProduct.get(obj.getOriginalValue());
}
if (path != null && !path.equals("")) {
loadCameraImage(path);
showPictures();
}
}
}
Try it yourself!
Click Add Product . On the screen that appears, scan the product barcode and take a photo of the product. Click Query Product and scan the product barcode. Since the product has been registered in the system, information about it will be returned.
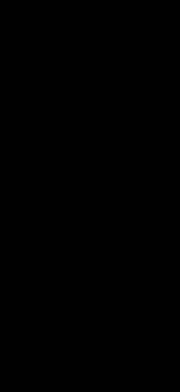
github.com/HMS-Core/hms-scan-demo/tree/master/Scan-Shopping
New examples
We'll be sharing with you examples of other amazing features available in the HUAWEI Scan Kit. Follow the news!