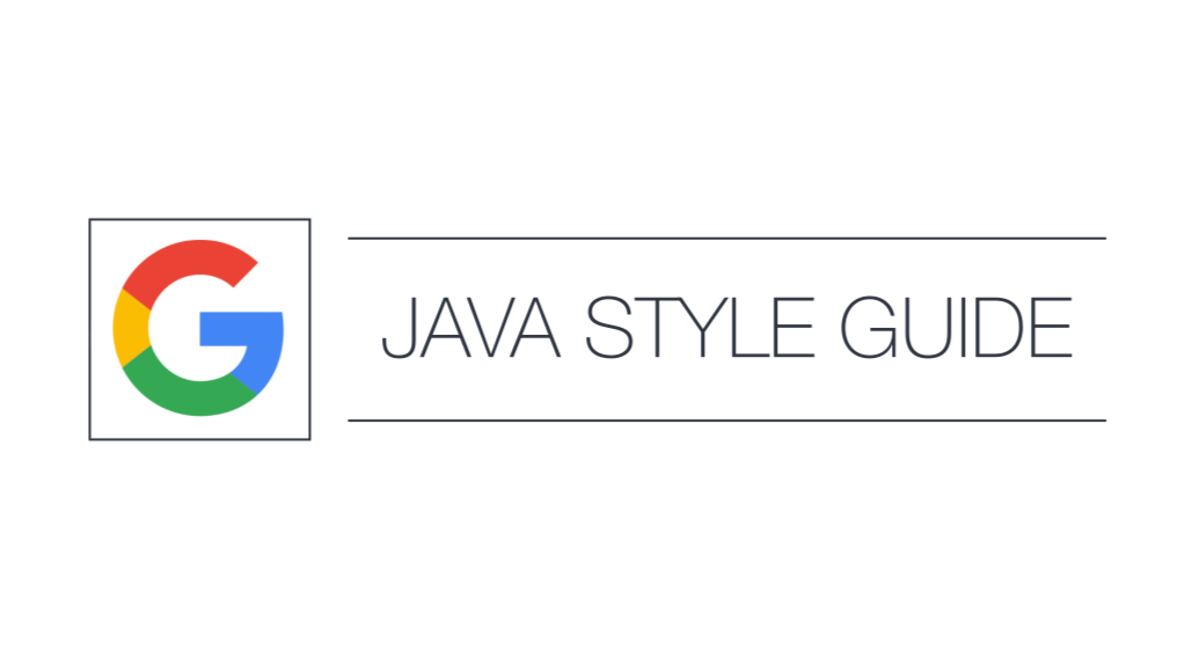
1. Introduction
This document describes the standards for coding in the Java programming language at Google. Java source code is considered to be in compliance with these standards if, and only if, it meets all the rules described in this document.
Topics covered in this tutorial are not only about the aesthetic side of code formatting, but other types of coding conventions or standards as well. However, this document concentrates primarily on defining the strict rules that we follow everywhere, and does not provide recommendations that can be incorrectly implemented (both by humans and by machine tools).
1.1 Terminology
Throughout this guide, the following terms are defined:
- The term class is used to mean a "regular" class, enumeration, interface or annotation type (@interface)
- The term class member is used to refer to a nested class, field, method, or constructor, that is, for all high-level class members except initialization blocks and comments.
- The term comment always refers to implementation comments. We do not use the phrase "documentation comments", but instead use the term "Javadoc"
The rest of the terminology will be provided as needed throughout this manual.
1.2 Guide note
The code examples in this document do not demonstrate the only correct stylistic approach to its formatting, although they are used in our tutorial.
2. Source file. The basics
2.1 File name
The source file name consists of the name of one particular top-level class that resides in it. The name is case sensitive and ends with a .java extension
2.2 File encoding: UTF-8
Files with code are encoded in UTF-8.
2.3 Special characters
2.3.1 Space characters
Apart from the end-of-line character sequence, the horizontal ASCII space character (0x20) is the only delimiter character found in the source file. It means that:
- All other whitespace characters in character and string literals are escaped
- Tab characters are not used for indentation
2.3.2 Special escape sequences
For each character for which there is a special escape sequence (\ b, \ t, \ n, \ f, \ r, \ ", \ 'and \\), it is preferable to use it instead of its corresponding octal value (for example, \ 012 ) or Unicode code (e.g. \ u000a).
2.3.3 Non-ASCII Characters
For non-ASCII characters, use the Unicode character (for example, ∞) or an equivalent escape sequence (for example, \ u221e). The choice is made in favor of those symbols that make the code more understandable and readable.
When using Unicode escape characters and in cases where valid Unicode characters are used, we strongly recommend that such code be accompanied by explanatory comments.
Example | Note |
---|---|
String unitAbbrev = "μs" | Excellent: perfectly perceived even without comment |
String unitAbbrev = "\ u03bcs" // "μs" | Allowed, but no reason to do so |
String unitAbbrev = "\ u03bcs" // Greek letter mu, "s" | Allowed but awkward and can lead to errors |
String unitAbbrev = "\ u03bcs" | Bad: the reader has no idea what it is |
return '\ ufeff' + content // byte order sign | Good: use escaping for non-printable characters and comment as needed |
Never make your code less readable just for fear that some programs will not be able to correctly process non-ASCII characters. If this happens, such programs do not work correctly and need to be fixed.
3. Source file structure
The source file consists of the following elements (in the order shown):
- License or copyright information, if any
- Package declaration
- Declaring imports
- Class declaration
Exactly one blank line separates each section present.
3.1 License or copyright information, if any
License or copyright information must be included in the file to which it refers.
3.2 Package declaration
The package is declared without a line break. The line width limitation (Section 4.4) does not apply to a package declaration.
3.3 Import declarations
3.3.1 Wildcard in import declarations
The * (wildcard) character is not used when declaring imports, static or not.
3.3.2 Line break
Imports are declared without line breaks. They are not subject to the line width limit.
3.3.3 Ordering and spacing
Imports are ordered as follows:
- All static imports are placed and grouped in one block
- All non-static imports are placed in another block
If both static and non-static imports are declared, then these blocks must be separated by an empty line. There should not be any other blank lines between them.
Within each block, the imported classes are listed in the ASCII sort order.
3.3.4 Static import of nested classes
Static imports are not used for static nested classes. Such classes are imported with a regular import declaration.
3.4 Class declaration
3.4.1 Exactly one top-level class is declared
Each top-level class is located in its own source file.
3.4.2 Ordering the contents of a class
The order in which you place the members and initialization blocks of a class can have a big impact on how easy your code is to understand. Either way, there is no simple and correct recipe for this; different classes can arrange their content differently.
The important thing is that each class has a logical ordering of content so that the programmer reading the code can explain it.
3.4.2.1 Overloaded code should not be split
When a class has multiple constructors or methods with the same name, they must be placed sequentially without inserting other code in between.
4. Formatting
Terminology
The body of a class, method, or constructor is block construction .
Note that according to Section 4.8.3.1, any array initializer can also be considered a block construct.
4.1 Curly braces
4.1.1 Curly braces are used wherever they may be used
Curly braces are used in if, else, for, while, and do-while, even if the body of the expression is empty or contains only one line of code.
4.1.2 Nonblank Blocks: K&R Style
Curly braces are placed according to the style of Kernighan and Ritchie ("Egyptian brackets") for non-empty blocks and block structures (for clarity, we decided to add a little code demonstrating these rules - translator's note):
- No line break is done before the opening parenthesis:
//
if (true) {
//
if (true)
{
- A line break is done after the opening parenthesis:
//
while (true) {
// code
//
while (true) { // code
- A line break is done before the closing parenthesis:
//
for () {
// code
}
//
while (true) { /* code */ }
//
if (true) {
/* code */ }
- A line break occurs after a closing parenthesis only if that parenthesis ends an expression or body of a method, constructor, or non-anonymous class. A line break is not done after a parenthesis if it is followed by an else, catch or semicolon
Examples of correctly executed rules:
return () -> {
while (condition()) {
method();
}
};
return new MyClass() {
@Override public void method() {
if (condition()) {
try {
something();
} catch (ProblemException e) {
recover();
}
} else if (otherCondition()) {
somethingElse();
} else {
lastThing();
}
}
};
Some exceptions for enumerations are given in Section 4.8.1
4.1.3 Empty blocks can be compressed
An empty block or empty block construct can follow the K & R style (as described in Section 4.1.2). It is also possible for such a block to be closed immediately after opening, without characters or line breaks inside {}. This rule does not apply when the block is part of a multi-block expression that contains an if-else or try-catch-finally.
Examples:
//
void doNothing() {}
//
void doNothingElse() {
}
// :
try {
doSomething();
} catch (Exception e) {}
4.2 Two spaces for indentation
Each time a new block or block construct is opened, the offset to the right is increased by two spaces. When a block ends, the start of the next line of code is shifted to the previous offset level. The offset level applies to both the block and the comments in that block (see the example in Section 4.1.2).
4.3 One expression per line
Each expression ends with a line break.
4.4 Limiting line width to 100 characters
Java code is limited to 100 characters in line width. A "character" refers to any of the Unicode elements. Except as described below, each line exceeding the width limit must be wrapped as explained in Section 4.5.
Exceptions:
- , (, URL Javadoc JSNI- )
- package import (. 3.2 3.3)
- ,
4.5
When code that might otherwise be on the same line is split across multiple lines, this phenomenon is called line break.
There is no generally accepted unambiguous formula that determines exactly how line breaks should be done in every situation. Very often there are several ways to break a line with the same piece of code.
Usually wrapping is done to avoid line width overflow. But even if the code would remain within the allowed width, then, at the author's discretion, it can be wrapped to a new line.
Allocating a helper method or local variable can solve the line width overflow problem without wrapping the code
4.5.1 Where to transfer
The first guideline for line breaks says: it is preferable to do the line break at a higher syntactic level. Also:
1. When a line is broken on a non-assignment statement, the break is made before the character.
This rule also applies to the following "operator-like" characters:
- dividing point "."
- double colon of reference method "::"
- ampersand in parentheses generic <T extends Foo & Bar>
- delimiter in catch block (FooException | BarException e)
2. When a string is wrapped in an assignment statement, the wrap is usually done after the character, but another solution
is acceptable. This also applies to the colon for for-each loop.
3. The name of the method or constructor when the line breaks remains attached to the opening parenthesis "("
4. The comma "," when the line breaks remains with the element that precedes it
5. The line is never wrapped directly at the arrow of the lambda expression, except when its body consists of a single expression without curly braces:
MyLambda<String, Long, Object> lambda =
(String label, Long value, Object obj) -> {
...
};
Predicate<String> predicate = str ->
longExpressionInvolving(str);
The main purpose of a line break is to achieve clarity in the code, but not necessarily the smallest number of lines
4.5.2 Offset line continuation by 4 or more spaces
When a line breaks, each subsequent substring (each line continuation) is shifted by at least 4 spaces relative to the previous one.
When there are several line continuations, the offset can vary within 4 spaces at the request of the author. As a general rule, two line extensions can have the same offset if and only if they start with syntactically parallel elements.
Section 4.6.3 provides guidance on using varying amounts of spaces to align code points relative to previous lines.
4.6 Spaces and indents
4.6.1 Indentation
One empty line is always placed:
1. Between successive members or class initializers: fields, constructors, methods, nested classes, static and dynamic initialization blocks
- exception: an empty line between two consecutive fields (no code between them) is optional. Empty lines are used to group fields logically if necessary
- exception: blank lines between enum class constants (see Section 4.8.1)
2. Consistent with other sections of this document (eg Section 3 and Section 3.3) The
blank line can also be used throughout to improve code readability, eg between expressions to organize code into logical subsections. An empty string before the first member of a class, or an initialization block, or after the last member, or an initialization block of a class is discouraged, but not prohibited.
Multiple consecutive blank lines are permitted, but not necessary or encouraged.
4.6.2 Spaces
Apart from the requirements of the language itself or other rules of this document, as well as not counting literals and comments (including Javadoc), single spaces from the ASCII table can only appear in the following places:
1. When separating any reserved word such as if, for or catch, and an opening parenthesis "(" that follows
2. When separating any reserved word such as else or catch, and a closing curly brace "}" that follows it
3. Before any opening curly brace " {", Except for two situations:
- @SomeAnnotation ({a, b})
- String [] [] x = {{"foo"}}; - space between {{not required according to clause 8 below
4. On either side of any binary or ternary operator
This rule also applies to the following operators:
- ampersand inside angle brackets: <T extends Foo & Bar>
- a delimiter in a catch block containing multiple exceptions: catch (FooException | BarException e)
- colon ":" in for-each
- arrow in lambda expression: (String str) -> str.length ()
But this rule does not apply to operators:
- double colon "::" of the reference method, which is written as Object :: toString
- the separating dot ".", which is written as object.toString ()
5. After ",:;" or the closing parenthesis ")" when casting to type
6. On either side of the double forward slash "//" when creating a comment on the same line of code. Multiple spaces are allowed but not required here
7. Between the type declaration and the variable name:
List<String> list
8. Optional: inside the parentheses of the array initializer
new int [] {5, 6} and new int [] {5, 6} are both valid
9. Between the type annotation and [] or ...
This rule does not require the presence or absence of spaces in beginning or end of a line; it only applies to internal spaces.
4.6.3 Horizontal alignment is never required
Terminology
Horizontal alignment is the practice of adding a varying number of extra spaces in your code to make certain elements appear below other elements from the previous line.
This practice is permitted but is not required by this guide. There is also no need to maintain alignment in those parts of the code where it has already been applied.
Example with and without alignment:
private int x; //
private Color color; //
private int x; // ,
private Color color; //
Alignment improves the readability of the code, but creates problems with maintaining such code in the future. Let's say you only want to change one line. This change may corrupt the formatting of previously accepted code, which is acceptable. But, most likely, the programmer (perhaps you) will start adjusting the number of spaces on adjacent lines, which may trigger a whole series of corrections. Changing one line can trigger a “blast wave” of mindless labor (at worst). Or, at best, it will distort information in the version history, impair code readability, and exacerbate merge conflicts.
4.7 Grouping brackets are recommended
You should only omit grouping brackets if the author of the code and the reviewer agree that there is no reasonable likelihood that the code will be misinterpreted without parentheses, nor would the parentheses make it easier to read. There is no reason to believe that anyone who reads the code has memorized the entire Java operator precedence table.
4.8 Special designs
4.8.1 Enumeration classes
After each comma that follows an enumeration constant, a line break is optional. Extra blank lines (usually just one) are also allowed. Here's an example of such code:
private enum Answer {
YES {
@Override public String toString() {
return "yes";
}
},
NO,
MAYBE
}
The code of an enumeration class without methods and comments describing its constants can be represented as an array initializer (see Section 4.8.3.1):
private enum Suit { CLUBS, HEARTS, SPADES, DIAMONDS }
Since enums are classes, all other rules that apply to classes should apply to them.
4.8.2 Variable declarations
4.8.2.1 One variable per declaration
Each variable (field or local) is declared only one at a time: declarations such as int a, b; are not used.
Exception : Multiple variable declarations are allowed in the header of a for loop.
4.8.2.2 Declare variables when you need them
Local variables do not need to be declared at the beginning of a block or block construction. Conversely, local variables need to be declared just before where they are first used to minimize their scope. Usually local variables are initialized at the time of declaration, or immediately after it.
4.8.3 Arrays
4.8.3.1 Array initializers can be "block"
Any array can be initialized as if it were a block construct. For example, all of the following code is valid (the list of examples is not complete):
new int[] {
0, 1, 2, 3
}
new int[] {
0, 1,
2, 3
}
new int[]
{0, 1, 2, 3}
new int[] {
0,
1,
2,
3
}
4.8.3.2 No C-style array declarations
The square brackets are placed after the type, not after the variable: String [] args, not String args [].
4.8.4 The switch statement
Terminology
One or more groups of statements are located within a switch block. Each group consists of one or more labels (both case FOO: and default :) followed by one or more statements (or, in the case of the last group, none or more).
4.8.4.1 Offset
As with any other block, the contents of the switch block are offset by 2 spaces.
A line break is made after the switch block label, and the offset is increased by 2 spaces, just as when the block was opened. Each next label returns to the previous level of offset, as when closing a block.
4.8.4.2 The through passage is commented
Within a block, each group of statements either terminates ahead of schedule with a switch (using break, continue, return, or throwing an exception), or is marked with a comment to indicate that code execution will or can continue in the next group. Any comment conveying the idea of a through passage (usually // fall through) is sufficient. This comment is not required in the last group of the switch block. Example:
switch (input) {
case 1:
case 2:
prepareOneOrTwo();
// fall through
case 3:
handleOneTwoOrThree();
break;
default:
handleLargeNumber(input);
}
Please note that the comment is not placed after case 1, but only at the end of the statement group.
4.8.4.3 Always use default
The switch statement must contain the default label, even if there is no code in it.
Exception : A switch block for an enum type may not use default if it contains explicit cases covering all possible values of that type. This allows the IDE or other static analysis tools to issue a warning that some cases are not covered.
4.8.5 Annotations
Annotations applied to a class, method, or constructor follow immediately after the doc block. Each annotation is indicated on its own line (that is, one annotation per line). These line breaks are not line breaks (see Section 4.5), so the indentation level is not increased. Example:
@Override
@Nullable
public String getNameIfPresent() { ... }
Exception : a single annotation without parameters can be displayed along with the first signature line, for example:
@Override public int hashCode() { ... }
Annotations applied to a field also appear immediately after the doc block, but in this case, multiple annotations (possibly parameterized) can be listed on the same line, for example:
@Partial @Mock DataLoader loader;
There are no special rules for formatting annotations for parameters, local variables, or types.
4.8.6 Comments
This section is dedicated to implementation comments. Javadoc is discussed separately in Section 7.
Any line break can be preceded by an arbitrary number of spaces, followed by an implementation comment. This comment makes the line non-empty.
4.8.6.1 Block comment style
The indentation level of a block comment is the same as the surrounding code. Block comments can be either / *… * / or //… For multi-line comments like / *… * /, subsequent lines must start with a *, aligned with a * from the previous line.
/*
* This is // And so /* Or you can
* okay. // is this. * even do this. */
*/
Comments are not enclosed in rectangles represented by asterisks or other symbols.
When writing multi-line comments, use the / * ... * / style if you want the automatic code formatter to break the line as needed (in a paragraph style). Most formatter cannot do this with single line comment blocks // ...
4.8.7 Modifiers
Class and field modifiers, if present, are displayed in the order recommended by the Java language specification:
public protected private abstract default static final transient volatile synchronized native strictfp
4.8.8 Numeric literals
The long type uses an uppercase L, not a lowercase letter (so as not to be confused with the number 1). For example, 300_000_000L instead of 300_000_000l.
5. Naming
5.1 General rules for all identifiers
Identifiers use only ASCII letters and numbers and, in some cases noted below, underscores.
Thus, every valid identifier name matches the regular expression \ w + (an alphanumeric character that occurs one or more times).
Names that use special suffixes or prefixes, such as name_, mName, s_name, or kName, do not conform to the style of this tutorial.
5.2 Rules for different types of identifiers
5.2.1 Package names
Package names must be written in lowercase, without camelCase or underscores.
Correct: com.example.deepspace
Incorrect: com.example.deepSpace or com.example.deep_space
5.2.2 Class names
Class names are written in the UpperCamelCase style (capitalized first letter).
Class names are usually nouns or noun phrases. For example, Character or ImmutableList.
Interface names can also be nouns or noun phrases (for example, List), but sometimes they can also be adjectives or adjective combinations (for example, Readable).
There are no specific rules or even well-established conventions for naming annotation types.
Test classes have a name that begins with the name of the class they are testing and ends with the word Test. For example, HashTest or HashIntegrationTest.
5.2.3 Method names
Method names are written in the lowerCamelCase style.
Method names are usually verbs or verb phrases. For example sendMessage or stop.
Underscores can be used in JUnit test method names to separate logical components in the name. Moreover, each component is written in the lowerCamelCase style. The typical pattern is:
<methodUnderTest>_<state>, , pop_emptyStack
There is no single correct way to name test methods.
5.2.4 Constant names
Constants are named in the CONSTANT_CASE style: all letters are uppercase, each word separated from the next by an underscore. But what exactly is a constant?
Constants are static final fields whose content is immutable, and methods that have no visible side effects. This applies to primitives, Strings, immutable types, and immutable collections of immutable types. If any observable state of an object can change, it is not a constant. The simple intention to never modify an object is not enough.
Examples:
//
static final int NUMBER = 5;
static final ImmutableList<String> NAMES = ImmutableList.of("Ed", "Ann");
static final ImmutableMap<String, Integer> AGES = ImmutableMap.of("Ed", 35, "Ann", 32);
static final Joiner COMMA_JOINER = Joiner.on(','); // because Joiner is immutable
static final SomeMutableType[] EMPTY_ARRAY = {};
enum SomeEnum { ENUM_CONSTANT }
//
static String nonFinal = "non-final";
final String nonStatic = "non-static";
static final Set<String> mutableCollection = new HashSet<String>();
static final ImmutableSet<SomeMutableType> mutableElements = ImmutableSet.of(mutable);
static final ImmutableMap<String, SomeMutableType> mutableValues =
ImmutableMap.of("Ed", mutableInstance, "Ann", mutableInstance2);
static final Logger logger = Logger.getLogger(MyClass.getName());
static final String[] nonEmptyArray = {"these", "can", "change"};
Constant names are usually nouns or noun phrases.
5.2.5 Names of non-constant fields
The names of fields that are not constants (static or not) are written in the lowerCamelCase style.
The names of such fields are usually nouns or noun phrases. For example, computedValues or index.
5.2.6 Parameter names
Parameter names are written in the lowerCamelCase style.
In public methods, one-character parameter names should be avoided.
5.2.7 Local variable names
Local variable names are written in the lowerCamelCase style.
Even though they are final and immutable, local variables are not considered constants and should not be written in the same style as constants.
5.2.8 Names of type variables
Each type variable is named according to one of two styles:
- Single capital letter, which can be followed by a regular number (for example, E, T, X, T2)
- A name in the form of a class name (see Section 5.2.2) followed by an uppercase letter T (examples: RequestT, FooBarT).
5.3 Camel style (camelCase)
Sometimes there is more than one way to convert an English phrase to camel style, such as in the case of abbreviations or atypical expressions like “IPv6” or “iOS”.
To improve predictability, this guide sets out the following (exemplary) schema.
Starting with the original form of the name:
1. Convert the phrase to regular ASCII and remove all apostrophes. For example, "Müller's algorithm" can be converted to "Muellers algorithm"
2. Divide the result into words, discarding spaces and any remaining punctuation (usually hyphens):
- recommendation: if any word already has a common form in the usual “camel” style, divide it into its component parts (for example, “AdWords” is converted to “ad words”). Note that a word like “iOS” is not really really camel-style; it does not conform to any conventions, so this recommendation does not apply.
3. Now convert everything to lower case (including abbreviations), then convert to upper case the first character:
- ... in every word to achieve the UpperCamelCase style, or
- ... in every word except the first to achieve the lowerCamelCase style
4. Finally, concatenate all the words into a single identifier.
Note that the case of the original words is almost completely ignored.
Examples:
Original form | Right | Wrong |
---|---|---|
"XML HTTP request" | XmlHttpRequest | XMLHTTPRequest |
"New customer ID" | newCustomerId | newCustomerID |
"Inner stopwatch" | innerStopwatch | innerStopWatch |
"Supports IPv6 on iOS?" | supportsIpv6OnIos | supportsIPv6OnIOS |
"YouTube importer" | YouTubeImporter
YoutubeImporter * |
* Allowed but not recommended.
Note : some words in English use a hyphen ambiguously: for example, both "nonempty" and "non-empty" are correct, so the method names checkNonempty and checkNonEmpty are also correct.
6. Programming practice
6.1 Always use @Override annotation
The method is marked with @Override annotation whenever it is actually overridden. This applies both to a method of a descendant class that overrides a method of a parent class, and an interface method that overrides a method of a super-interface.
Exception : the annotation can be omitted if the parent method is marked with @Deprecated annotation.
6.2 Don't ignore caught exceptions
It is very rare that there are situations when you do not need to take any action in response to a caught exception (a typical solution is to log it or, if it is considered "impossible", to throw the exception as an AssertionError).
Below is an example with an explanatory comment when it is really appropriate not to take any action in the catch block:
try {
int i = Integer.parseInt(response);
return handleNumericResponse(i);
} catch (NumberFormatException ok) {
// it's not numeric; that's fine, just continue
}
return handleTextResponse(response);
Exception : In tests, a caught exception may be ignored and uncommented if the test name is expected or if the name begins with expected. The following is a very common idiom showing that the code under test throws an exception of the expected type, so no comments are needed here:
try {
emptyStack.pop();
fail();
} catch (NoSuchElementException expected) {
}
6.3 For static members, use the class name
It is necessary to refer to a member of a static class through the class name, and not by reference to a class object or by an expression that returns this object:
Foo aFoo = ...;
Foo.aStaticMethod(); //
aFoo.aStaticMethod(); //
somethingThatYieldsAFoo().aStaticMethod(); //
6.4 Don't use finalizers
It is extremely rare that you need to override the Object.finalize method.
Hint :
Don't do this. If you really need it, first read and really thoroughly understand Effective Java Item 7, “Avoid Finalizers,” and then don't.
7. Javadoc
7.1 Formatting
7.1.1 Main form
The simple formatting of Javadoc blocks follows this example:
/**
* Multiple lines of Javadoc text are written here,
* wrapped normally...
*/
public int method(String p1) { ... }
... or in one line:
/** An especially short bit of Javadoc. */
The simple form is always applicable. The single line form can be applied when the entire Javadoc block (including comment markers) can fit on one line. Note that this only applies when there are no tags such as @return in the block.
7.1.2 Paragraphs
One blank line, that is, a line containing only an aligned leading asterisk (*), appears between paragraphs and before the block tag group, if any. Every paragraph except the first contains a <p> immediately before the first word, no space after.
7.1.3 Block tags
All block tags are in this order: @param, @return, @throws, @deprecated, and these four types are never present with empty descriptions. If a block tag does not fit on one line, continuation lines are indented four (or more) spaces from @.
7.2 Final snippet
Each Javadoc block starts with a short summary snippet. This snippet is very important: it is the only text that appears in a specific context, such as class and method indexes.
This snippet is a noun or verb phrase, not a full sentence. It does not start with A {@code Foo} is a ... or This method returns ..., nor does it form a complete affirmative sentence like Save the record. However, this passage is capitalized and punctuated as if it were a complete sentence.
Hint : It is a common mistake to write a simple Javadoc like / ** @return the customer ID * /. This is incorrect and should be corrected to / ** Returns the customer ID. * /.
7.3 When Javadoc Is Applied
Javadoc is present in at least every public class and every public and protected member of such class, except in some cases, described below.
Additional Javadoc may be present, as explained in Section 7.3.4, Optional Javadoc.
7.3.1 Exception: methods that describe themselves
Javadoc is optional for simple and obvious methods like getFoo, in cases where you really can't say more than "Returns foo".
Important : It is inappropriate to refer to this exception to justify omitting relevant information that the general reader might need.
For example, for a method named getCanonicalName, do not omit the documentation (with the justification that the method name only says / ** Returns the canonical name. * /) If the average person reading the code may not even suspect what the term "canonical name" means !
7.3.2 Exception: Override
The Javadoc does not always accompany a method that overrides a method from a super class (or super interface).
7.3.4 Optional Javadoc
Other classes and members are accompanied by Javadoc as needed or desired.
Whenever an implementation comment will be used to define the general purpose or behavior of a class or member, that comment is written as Javadoc (using / **).
The optional Javadoc does not have to follow the formatting rules of Sections 7.1.2, 7.1.3, and 7.2, although this is of course recommended.
This translation is also available on our blog.