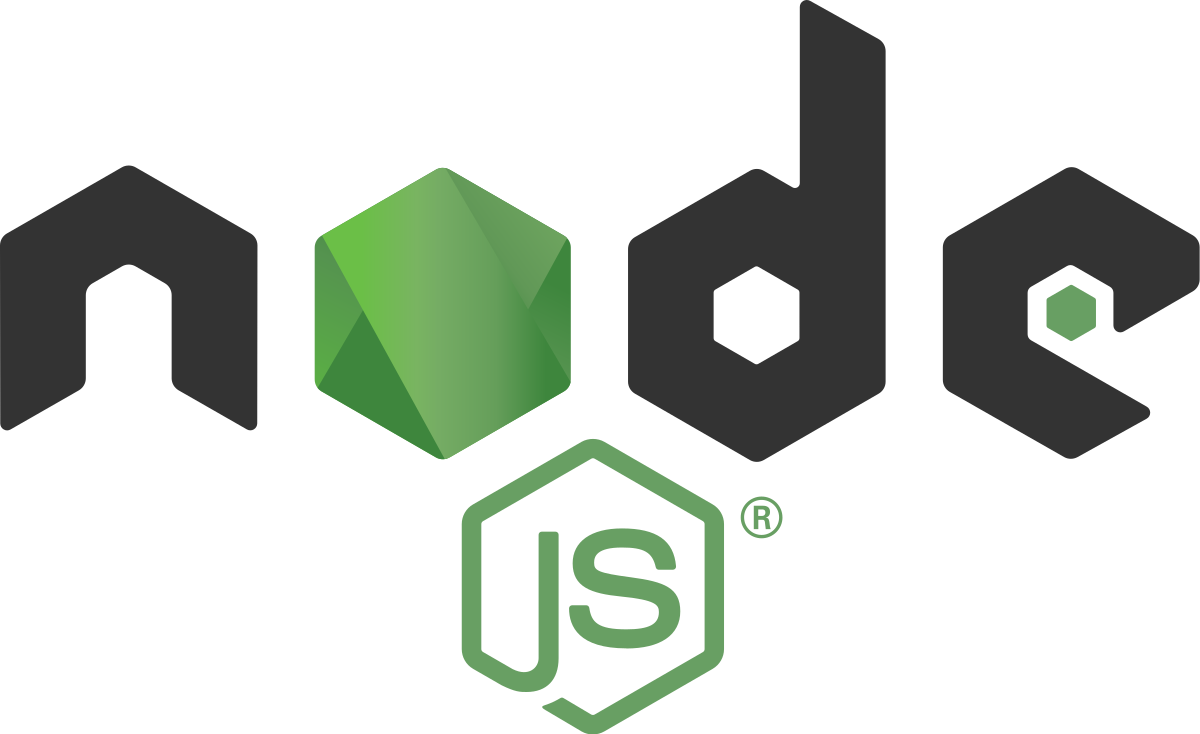
Good day, friends!
I present to you the translation of this Node.js guide .
Other parts:
Part 1
Part 2
Part 3
Part 4
Introduction to Node.js
Node.js is an open and cross-platform JavaScript runtime. This is a great solution for almost any project.
Node.js launches the JavaScript engine V8, the core of Google Chrome, outside the browser. This makes Node.js very performant.
Node.js applications run as a single process, without creating a new thread for each request. Node.js provides a set of asynchronous I / O primitives in the standard library that protect JavaScript from blocking, and typically libraries in Node.js are written using non-blocking paradigms, making blocking behavior the exception rather than the rule.
When Node.js performs an I / O operation, such as reading (data) from the network, accessing a database or file system, instead of blocking the stream and waiting for the CPU cycles to complete, Node.js will continue to perform the operation after receiving a response.
This allows Node.js to handle thousands of requests concurrently through a single server without the need for a stream consistency system that can be a source of serious bugs.
A significant advantage of Node.js is that millions of developers writing JavaScript code for the browser now have the ability to write server-side code in addition to the client code without having to learn a completely different language (programming).
In Node.js, the new ECMAScript standards can be used seamlessly, you don't have to wait for all users to update their browsers - you decide which version of ECMAScript to use by changing the Node.js version, you can also add experimental features by running Node.js with (corresponding) flags.
A huge number of libraries
Npm, with its simple structure, contributes to the rapid growth of the Node.js ecosystem; today, over 1 million open packages are registered with npm, which you can use for free.
Node.js application example
The most common use case for Node.js is to create a web server:
const http = require('http')
const hostname = '127.0.0.1'
const port = process.env.PORT
const server = http.createServer((req, res) => {
res.statusCode = 200
res.setHeader('Content-Type', 'text/plain')
res.end('Hello World!\n')
})
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`)
})
The first thing we do is plug in the http module .
Node.js has a fantastic standard library that includes top-notch networking support.
The method
createServer()
http
creates a new HTTP server and returns it.
The server is set to listen on a specific port and host. When the server is ready, a callback function is triggered, in this case, indicating that the server is running.
When a new request is received, a request event is fired containing two objects: a request (an http.IncomingMessage object (an incoming message)) and a response (an http.ServerResponse object (a server response)).
These objects are required to handle an HTTP call.
The first object contains request details. In our example, it is not used, but you can access the request headers and its data.
The second object is used to provide data to the requestor.
In this case, through
res.statusCode = 200
we set the statusCode property to 200 as an indicator of successful completion of the request.
We set the Content-Type header (content or content type)
res.setHeader('Content-Type', 'text/plain')
and close the response by adding content as an argument to
end()
:
res.end('Hello World\n')
Node.js frameworks and tools
Node.js is a low-level platform. Thousands of different libraries have been created to meet the needs of developers.
Over time, many of them have become very popular. Here is a list of some of them:
- AdonisJs: - , . Adonis — - Node.js.
- Express: -. , , .
- Fastify: -, . Fastify -.
- hapi: , .
- koa: Express . - , Express.
- Loopback.io: .
- Meteor: -, JavaScript, . - meteor , , React, Vue Angular. .
- Micro: HTTP-.
- NestJS: TypeScript Node.js- , .
- Next.js: React- .
- Nx: NestJS, Express, React, Angular .. Nx , .
- Socket.io: .
Node.js
Believe it or not, Node.js is only 10 years old.
By comparison, JavaScript has been around for 24 years, and the web for 30.
10 years is a short time for technology, but sometimes it seems like Node.js has always been.
I got to know Node.js when only 2 years had passed since its inception, but even then, despite the limited information, it felt like a great future awaited it.
In this section, we'll take a look at the big picture of Node.js history.
A bit of history
JavaScript is a programming language invented by Netscape as a scripting tool for manipulating web pages in the Netscape Navigator browser .
Part of Netscape's business model has been selling web servers that include the Netscape LiveWire framework, which can create dynamic pages using server-side JavaScript. Unfortunately, Netscape LiveWire failed and server-side JavaScript was not popular until Node.js.
One of the key factors in the popularity of Node.js is the time (of its appearance). JavaScript had been recognized as a serious (programming) language a few years earlier thanks to "Web 2.0" applications (such as Flickr, Gmail, and others) that showed the world what the modern web might look like.
JavaScript engines also got significantly better as browsers sought to improve performance for the benefit of users. The major browser development teams have worked hard to make sure JavaScript is better supported and executed as quickly as possible. The engine that uses Node.js, V8 (also known as Chrome V8 - Chromium's open JavaScript engine) came out the winner in this competition.
Node.js appeared at the right time and place. Fortunately, this is not the only reason for its popularity. The project contained a large number of innovative ideas and approaches to server-side JavaScript development, which many developers liked.
2009
- Node.js appeared
- Npm appeared
2010
- Express
- Socket.io
2011
- npm version 1.0
- Node.js: LinkedIn, Uber .
- hapi
2012
- Node.js
2013
- - Node.js: Ghost
- Koa
2014
- : Node.js io.js ( — git) ES6
2015
- Node.js Foundation
- IO.js Node.js ( — git)
- npm ()
- Node.js 4 ( 1, 2 3 )
2016
- left-pad
- Yarn
- Node.js 6
2017
- npm
- Node.js 8
- HTTP/2
- V8 Node.js , Node.js JS Chrome
- 3 npm
2018
- Node.js 10
- Experimental support for ES modules with the .mjs extension
How do I install Node.js?
Node.js can be installed in various ways.
Distributions for major platforms are available on the official website .
A very convenient way to install Node.js is to use a package manager. Each operating system has its own.
On macOS, this is Homebrew , which makes it easy to install Node.js using the command line:
brew install node
The list of package managers for Linux, Windows and other systems is here .
nvm
Is a popular way to run Node.js. It allows you to easily switch between Node.js versions, install new versions for testing, and undo installations in case something goes wrong.
It is also very useful when testing code in older versions of Node.js.
For more details
nvm
follow this link .
My advice is to use the official installer if you're just starting out and haven't used Homebrew before.
After installing Node.js, you get access to the executable program
node
on the command line.
How well do you need to know JavaScript to work with Node.js?
As a beginner, it is difficult to determine your programming level.
It is also difficult to determine where JavaScript ends and Node.js starts, and vice versa.
Personally, I would suggest getting a good grasp of the following basic JavaScript concepts before diving into Node.js:
- Syntax or lexical structure
- Expressions (essentially the same syntax)
- Data types)
- Variables
- Functions
- This keyword
- Arrow functions
- Cycles
- Area of visibility
- Arrays
- Template or string literals
- A semicolon (probably cases of its mandatory use, for example, when working with IIFE)
- Strict regime
- ECMAScript 6, 2016, 2017
Mastering these concepts is the beginning of the path of a professional full-stack developer.
The following concepts are also key to understanding asynchronous programming, which is a fundamental part of Node.js:
- Asynchronous programming and callback functions (callbacks)
- Timers (counters)
- Promises (promises)
- Async / await
- Short circuits
- Event loop (call stack)
Difference between Node.js and the browser
JavaScript can be used in both the browser and Node.js.
However, creating browser applications is very different from creating Node.js applications.
Despite the fact that JavaScript is used in both cases, there are some key differences that determine the specifics of the development.
From the point of view of the JavaScript frontend (front-end developer - the client-side of the application), developing applications in Node.js has a significant advantage, expressed in the fact that everywhere, both on the client and on the server, the same programming language is used - JavaScript.
This is a great opportunity to become a full stack: we all know how difficult it is to fully, deeply learn a new programming language, and in this case there is no such need.
The only thing that needs to be studied is the ecosystem.
In the browser, most of the time we have to deal with the DOM and other web APIs like cookies. Of course they don't exist in Node.js. Node.js lacks window, document, and other browser-specific objects.
On the other hand, browsers lack the great APIs that Node.js provides through modules, such as file system access.
Another big difference is that in Node.js, you control the code execution environment. Unless you are developing an open source application that anyone can deploy anywhere, you know which version of Node.js is used to run the application. This is very convenient, unlike the browser runtime, because users decide which browser to use.
This means that you can write code in JavaScript supported by your version of Node.js.
Because JavaScript is evolving very dynamically, browsers don't always have time to quickly implement new language features, and users don't always update in a timely manner, which is why you often have to maintain old JavaScript on the web.
To transpile code to ES5, you can use Babel; in Node.js, this is not necessary.
Another difference is that Node.js uses the CommonJS modular system, and browsers support ES-modules.
In practice, this means that in Node.js we use
require()
, and in the browser import
.
Thank you for your attention, friends. If you find errors and typos, do not hesitate to write in a personal, I will be grateful.
To be continued…